android 自定义View研究(二) — 自定义控件添加属性
2013-08-26 13:46
465 查看
前一篇讲到了自定义View,有些时候为了提高复用性,可能需要给 自定义的View添加某些属性,比如 字体大小、字体颜色等等,这一篇就跟大家探讨如何 自定义View并添加属性 。
工程目录结构如下:
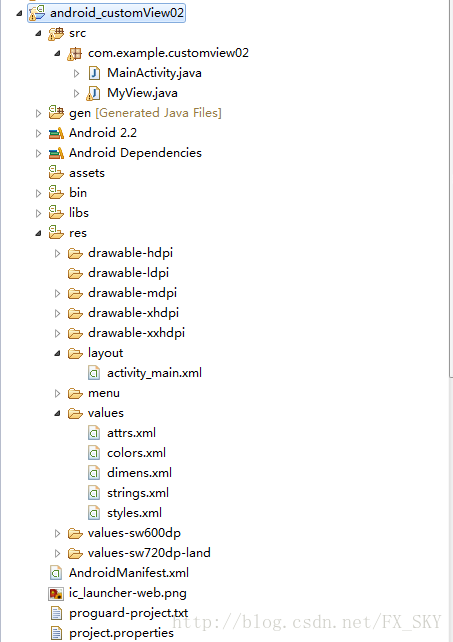
运行效果图:
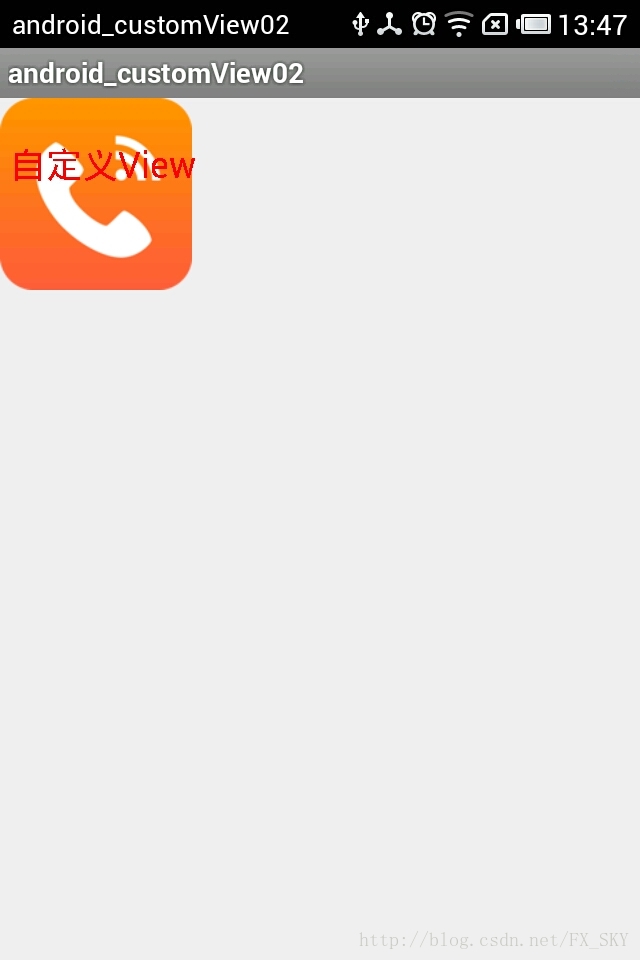
1、在values 目录下新建 attrs.xml
2、在 布局文件中对属性进行设置
注意:如果使用自定义属性,那么在应用xml文件中需要加上新的schemas,
比如这里是xmlns:my="http://schemas.android.com/apk/res/com.example.customview02" ,其中xmlns后的“my”是自定义的属性的前缀,res后的是我们自定义View所在的包
3、通过TypedArray 获取 属性值
最后是 MainActivity.java
自定义控件属性详解可以参考这篇文章:http://www.cnblogs.com/bill-joy/archive/2012/04/26/2471831.html
工程下载地址:http://download.csdn.net/detail/fx_sky/6013031
工程目录结构如下:
运行效果图:
1、在values 目录下新建 attrs.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="MyView"> <attr name="textColor" format="color"/> <attr name="textSize" format="dimension"/> <attr name="text" format="string"/> <attr name="background" format="reference"/> <attr name="focusable" format="boolean"/> </declare-styleable> </resources>
2、在 布局文件中对属性进行设置
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:my="http://schemas.android.com/apk/res/com.example.customview02" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" > <com.example.customview02.MyView android:layout_width="wrap_content" android:layout_height="wrap_content" my:text="@string/custom_view" my:textColor="@color/red" my:textSize="18sp" my:background="@drawable/logo" my:focusable="true"/> </RelativeLayout>
注意:如果使用自定义属性,那么在应用xml文件中需要加上新的schemas,
比如这里是xmlns:my="http://schemas.android.com/apk/res/com.example.customview02" ,其中xmlns后的“my”是自定义的属性的前缀,res后的是我们自定义View所在的包
3、通过TypedArray 获取 属性值
package com.example.customview02; import android.content.Context; import android.content.res.TypedArray; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.Canvas; import android.graphics.Paint; import android.util.AttributeSet; import android.view.View; /** * 这个是自定义的TextView. * 至少需要重载构造方法和onDraw方法 * 对于自定义的View如果没有自己独特的属性,可以直接在xml文件中使用就可以了 * 如果含有自己独特的属性,那么就需要在构造函数中获取属性文件attrs.xml中自定义属性的名称 * 并根据需要设定默认值,放在在xml文件中没有定义。 * 如果使用自定义属性,那么在应用xml文件中需要加上新的schemas, * 比如这里是xmlns:my="http://schemas.android.com/apk/res/com.example.customview02" * 其中xmlns后的“my”是自定义的属性的前缀,res后的是我们自定义View所在的包 * * @author Administrator * */ public class MyView extends View { private Paint mPaint; private String content = null; private int resourceId; public MyView(Context context) { super(context); // TODO Auto-generated constructor stub } public MyView(Context context, AttributeSet attrs) { super(context, attrs); mPaint = new Paint(); //TypedArray是一个用来存放由context.obtainStyledAttributes获得的属性的数组 //在使用完成后,一定要调用recycle方法 //属性的名称是styleable中的名称+“_”+属性名称 TypedArray a = context.obtainStyledAttributes(attrs, R.styleable.MyView); int textColor = a.getColor(R.styleable.MyView_textColor, 0XFF00FF00); //提供默认值,放置未指定 float textSize = a.getDimensionPixelSize(R.styleable.MyView_textSize, 20); //获取字体大小 content = a.getString(R.styleable.MyView_text); boolean focusable = a.getBoolean(R.styleable.MyView_focusable, false); // Drawable drawable = array.getDrawable(R.styleable.MyView_background); resourceId = a.getResourceId(R.styleable.MyView_background, R.drawable.ic_launcher); mPaint.setColor(textColor); mPaint.setTextSize(textSize); a.recycle(); //一定要调用,否则这次的设定会对下次的使用造成影响 } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); Bitmap mBitmap = BitmapFactory.decodeResource(getResources(), resourceId); canvas.drawBitmap(mBitmap, 0, 0, mPaint); canvas.drawText(content, 10, 100, mPaint); } }
最后是 MainActivity.java
package com.example.customview02; import android.app.Activity; import android.os.Bundle; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } }
自定义控件属性详解可以参考这篇文章:http://www.cnblogs.com/bill-joy/archive/2012/04/26/2471831.html
工程下载地址:http://download.csdn.net/detail/fx_sky/6013031
相关文章推荐
- Android 自定义View并添加属性
- Android 自定义View并添加属性(转)
- Android自定义View及自定义控件属性declare-styleable:自定义控件的属性(attr.xml,TypedArray)的使用
- android 自定义view添加自定义xml属性
- 为android自定义View控件添加自定义的属性
- Android自定义控件之自定义属性的添加 -(2)
- 【android自定义控件】自定义View属性
- Android中如何使用自定义view 自定义控件属性及动态自定义控件
- Android 自定义View并添加属性
- Android 自定义View并添加属性
- [Android_Develop]自定义View添加XML属性
- Android自定义View 属性添加
- Android 自定义View并添加属性
- Android 自定义View并添加属性介绍
- android中如何使用自定义view,自定义控件属性,及动态自定义控件
- Android 自定义View并添加属性
- android自定义控件(7)-获取自定义ImageView的src属性
- 自定义View添加自定义属性详细
- Android控件构架与自定义控件详解(二)自定义View
- Android自定义控件-完全自定义(继承View ,ViewGroup)