Android应用中通过AIDL机制实现进程间的通讯实例
2013-03-27 10:10
811 查看
在Android中,每个应用程序都有自己的进程,当需要在不同的进程之间传递对象时,该如何实现呢?显然,Java中是不支持跨进程内存共享的,因此要传递对象,需要把对象解析成操作系统能够理解的数据格式,以达到跨界对象访问的目的。在Android中,则采用AIDL(Android Interface Definition Language :接口定义语言)方式实现。
AIDL (Android Interface Definition Language)是一种IDL语言,用于生成可以在Android设备上两个进程之间进行进程间通信(IPC)的代码。如果在一个进程中(例如Activity)要调用另一个进程中(例如Service)对象的操作,就可以使用AIDL生成可序列化的参数。
AIDL IPC机制是面向接口的,它是使用代理类在客户端和实现端传递数据。
使用AIDL实现IPC
使用AIDL实现IPC服务的步骤是:
1.创建.aidl文件-该文件(YourInterface.aidl)定义了客户端可用的方法和数据的接口。
2.在makefile文件中加入.aidl文件-(Eclipse中的ADT插件提供管理功能)Android包括名为AIDL的编译器,位于tools/文件夹。
3.实现接口-AIDL编译器从AIDL接口文件中利用Java语言创建接口,该接口有一个继承的命名为Stub的内部抽象类(并且实现了一些IPC调用的附加方法),要做的就是创建一个继承于YourInterface.Stub的类并且实现在.aidl文件中声明的方法。
4.向客户端公开接口-如果是编写服务,应该继承Service并且重载Service.onBind(Intent)以返回实现了接口的对象实例
创建.aidl文件
AIDL使用简单的语法来声明接口,描述其方法以及方法的参数和返回值。这些参数和返回值可以是任何类型,甚至是其他AIDL生成的接口。重要的是必须导入所有非内置类型,哪怕是这些类型是在与接口相同的包中。下面是AIDL能支持的数据类型:
1.Java编程语言的主要类型 (int, boolean等)—
不需要 import语句。
2.以下的类 (不需要import语句):
String
List -列表中的所有元素必须是在此列出的类型,包括其他AIDL生成的接口和可打包类型。List可以像一般的类(例如List<String>)那样使用,另一边接收的具体类一般是一个ArrayList,这些方法会使用List接口。
Map - Map中的所有元素必须是在此列出的类型,包括其他AIDL生成的接口和可打包类型。一般的maps(例如Map<String,Integer>)不被支持,另一边接收的具体类一般是一个HashMap,这些方法会使用Map接口。
CharSequence -该类是被TextView和其他控件对象使用的字符序列。
3.通常引引用方式传递的其他AIDL生成的接口,必须要import语句声明
4.实现了Parcelable protocol以及按值传递的自定义类,必须要import
语句声明。
通过对上面的基本了解,下面我就以一个具体的实例来说明Android中如何通过AIDL机制来实现两个进程中实现通讯:(情景假设:例如A应用通过服务Service方式向B应用提供通过书籍编号来查询书籍名称的服务)
A应用程序结构图如下:

通过上面的结构图可以看到,在A应用程序中创建一个 aidl 的接口,然后系统在 gen 目录下自动生成相应的 java 文件。
其中 IBook.aidl 文件的源码:
[html]
view plaincopyprint?
package com.andyidea.aidl; interface IBook { String queryBook(int bookNo); }
其中 BookService.java 类中的源码如下:
[html]
view plaincopyprint?
package com.andyidea.service;
import com.andyidea.aidl.IBook;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.RemoteException;
/**
* 查询书籍的服务
* @author Andy
*
*/
public class BookService extends Service {
private String[] bookNames = {"Java编程思想","设计模式","Android开发设计"};
private IBinder mIBinder =
new BookBinder();
@Override
public IBinder onBind(Intent intent) {
// TODO Auto-generated method stub
return mIBinder;
}
/**
* 服务中交互的方法
* @param bookNo
* @return
*/
public String queryBookName(int bookNo){
if(bookNo > 0 && bookNo
<= bookNames.length){
return bookNames[bookNo-1];
}
return null;
}
private class BookBinder extends IBook.Stub{
@Override
public String queryBook(int bookNo) throws RemoteException {
return queryBookName(bookNo);
}
}
}
[html]
view plaincopyprint?
<?xml
version="1.0"
encoding="utf-8"?>
<manifest
xmlns:android="http://schemas.android.com/apk/res/android"
package="com.andyidea.service"
android:versionCode="1"
android:versionName="1.0"
>
<uses-sdk
android:minSdkVersion="8"
/>
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
>
<span
style="color: rgb(255, 0, 0);">
<service
android:name=".BookService">
<intent-filter>
<action
android:name="com.andyidea.aidl.bookservice"/>
</intent-filter>
</service></span>
</application>
</manifest>
B应用程序结构图如下:
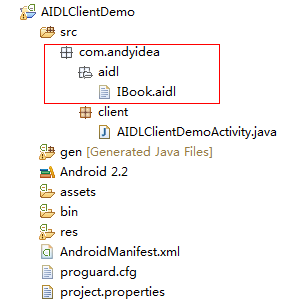
我们看到B应用程序也要和服务端同样的 .aidl 文件,我们可以从A应用程序中把该 aidl 文件中拷贝过来就是了,呵。由于B应用中 .aidl 文件和 A应用中的 .aidl 文件源码一样,我在这里就不列出来了。
其中AIDLClientDemoActivity.java源码如下:【注:其中该客户端类要通过 bindService 方式来启动另外一个进程的服务,这样才能实现和服务进行交互。如果通过startService方式来启动服务,则不能与服务进行交互】
[html]
view plaincopyprint?
package com.andyidea.client;
import android.app.Activity;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.andyidea.aidl.IBook;
public class AIDLClientDemoActivity extends Activity {
private EditText numberText;
private TextView resultView;
private Button query;
private IBook bookQuery;
private BookConnection bookConn =
new BookConnection();
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
numberText = (EditText) this.findViewById(R.id.number);
resultView = (TextView) this.findViewById(R.id.resultView);
query = (Button)findViewById(R.id.query);
Intent service =
new Intent("com.andyidea.aidl.bookservice");
bindService(service, bookConn, BIND_AUTO_CREATE);
query.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String number =
numberText.getText().toString();
int num =
Integer.valueOf(number);
try {
resultView.setText(bookQuery.queryBook(num));
} catch (RemoteException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onDestroy() {
unbindService(bookConn);
super.onDestroy();
}
private final class BookConnection implements ServiceConnection{
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
// TODO Auto-generated method stub
bookQuery = IBook.Stub.asInterface(service);
}
@Override
public void onServiceDisconnected(ComponentName name) {
// TODO Auto-generated method stub
bookQuery = null;
}
}
}
[html]
view plaincopyprint?
<?xml
version="1.0"
encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="书籍编号"
/>
<EditText
android:id="@+id/number"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/query"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="查询"/>
<TextView
android:id="@+id/resultView"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
</LinearLayout>

通过上面的截图,我们输入书籍编号 1,就可以查询出相应的书籍名称,到此,我们就可以了解了 Android应用中如何通过AIDL机制实现两个进程的通讯。
AIDL (Android Interface Definition Language)是一种IDL语言,用于生成可以在Android设备上两个进程之间进行进程间通信(IPC)的代码。如果在一个进程中(例如Activity)要调用另一个进程中(例如Service)对象的操作,就可以使用AIDL生成可序列化的参数。
AIDL IPC机制是面向接口的,它是使用代理类在客户端和实现端传递数据。
使用AIDL实现IPC
使用AIDL实现IPC服务的步骤是:
1.创建.aidl文件-该文件(YourInterface.aidl)定义了客户端可用的方法和数据的接口。
2.在makefile文件中加入.aidl文件-(Eclipse中的ADT插件提供管理功能)Android包括名为AIDL的编译器,位于tools/文件夹。
3.实现接口-AIDL编译器从AIDL接口文件中利用Java语言创建接口,该接口有一个继承的命名为Stub的内部抽象类(并且实现了一些IPC调用的附加方法),要做的就是创建一个继承于YourInterface.Stub的类并且实现在.aidl文件中声明的方法。
4.向客户端公开接口-如果是编写服务,应该继承Service并且重载Service.onBind(Intent)以返回实现了接口的对象实例
创建.aidl文件
AIDL使用简单的语法来声明接口,描述其方法以及方法的参数和返回值。这些参数和返回值可以是任何类型,甚至是其他AIDL生成的接口。重要的是必须导入所有非内置类型,哪怕是这些类型是在与接口相同的包中。下面是AIDL能支持的数据类型:
1.Java编程语言的主要类型 (int, boolean等)—
不需要 import语句。
2.以下的类 (不需要import语句):
String
List -列表中的所有元素必须是在此列出的类型,包括其他AIDL生成的接口和可打包类型。List可以像一般的类(例如List<String>)那样使用,另一边接收的具体类一般是一个ArrayList,这些方法会使用List接口。
Map - Map中的所有元素必须是在此列出的类型,包括其他AIDL生成的接口和可打包类型。一般的maps(例如Map<String,Integer>)不被支持,另一边接收的具体类一般是一个HashMap,这些方法会使用Map接口。
CharSequence -该类是被TextView和其他控件对象使用的字符序列。
3.通常引引用方式传递的其他AIDL生成的接口,必须要import语句声明
4.实现了Parcelable protocol以及按值传递的自定义类,必须要import
语句声明。
通过对上面的基本了解,下面我就以一个具体的实例来说明Android中如何通过AIDL机制来实现两个进程中实现通讯:(情景假设:例如A应用通过服务Service方式向B应用提供通过书籍编号来查询书籍名称的服务)
A应用程序结构图如下:

通过上面的结构图可以看到,在A应用程序中创建一个 aidl 的接口,然后系统在 gen 目录下自动生成相应的 java 文件。
其中 IBook.aidl 文件的源码:
[html]
view plaincopyprint?
package com.andyidea.aidl; interface IBook { String queryBook(int bookNo); }
package com.andyidea.aidl; interface IBook { String queryBook(int bookNo); }
其中 BookService.java 类中的源码如下:
[html]
view plaincopyprint?
package com.andyidea.service;
import com.andyidea.aidl.IBook;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.RemoteException;
/**
* 查询书籍的服务
* @author Andy
*
*/
public class BookService extends Service {
private String[] bookNames = {"Java编程思想","设计模式","Android开发设计"};
private IBinder mIBinder =
new BookBinder();
@Override
public IBinder onBind(Intent intent) {
// TODO Auto-generated method stub
return mIBinder;
}
/**
* 服务中交互的方法
* @param bookNo
* @return
*/
public String queryBookName(int bookNo){
if(bookNo > 0 && bookNo
<= bookNames.length){
return bookNames[bookNo-1];
}
return null;
}
private class BookBinder extends IBook.Stub{
@Override
public String queryBook(int bookNo) throws RemoteException {
return queryBookName(bookNo);
}
}
}
package com.andyidea.service; import com.andyidea.aidl.IBook; import android.app.Service; import android.content.Intent; import android.os.IBinder; import android.os.RemoteException; /** * 查询书籍的服务 * @author Andy * */ public class BookService extends Service { private String[] bookNames = {"Java编程思想","设计模式","Android开发设计"}; private IBinder mIBinder = new BookBinder(); @Override public IBinder onBind(Intent intent) { // TODO Auto-generated method stub return mIBinder; } /** * 服务中交互的方法 * @param bookNo * @return */ public String queryBookName(int bookNo){ if(bookNo > 0 && bookNo <= bookNames.length){ return bookNames[bookNo-1]; } return null; } private class BookBinder extends IBook.Stub{ @Override public String queryBook(int bookNo) throws RemoteException { return queryBookName(bookNo); } } }同时别忘了在 Manifest.xml中配置该服务对象(标红色的部分),建议采用隐式方式激活该服务,适合不同的进程的意图。
[html]
view plaincopyprint?
<?xml
version="1.0"
encoding="utf-8"?>
<manifest
xmlns:android="http://schemas.android.com/apk/res/android"
package="com.andyidea.service"
android:versionCode="1"
android:versionName="1.0"
>
<uses-sdk
android:minSdkVersion="8"
/>
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
>
<span
style="color: rgb(255, 0, 0);">
<service
android:name=".BookService">
<intent-filter>
<action
android:name="com.andyidea.aidl.bookservice"/>
</intent-filter>
</service></span>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.andyidea.service" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" /> <application android:icon="@drawable/ic_launcher" android:label="@string/app_name" > <service android:name=".BookService"> <intent-filter> <action android:name="com.andyidea.aidl.bookservice"/> </intent-filter> </service> </application> </manifest>以上我们已经实现了A应用程序提供服务的功能,下面我们来实现B应用(或者其它需要用到A应用提供服务的应用程序)
B应用程序结构图如下:
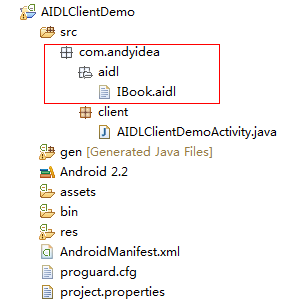
我们看到B应用程序也要和服务端同样的 .aidl 文件,我们可以从A应用程序中把该 aidl 文件中拷贝过来就是了,呵。由于B应用中 .aidl 文件和 A应用中的 .aidl 文件源码一样,我在这里就不列出来了。
其中AIDLClientDemoActivity.java源码如下:【注:其中该客户端类要通过 bindService 方式来启动另外一个进程的服务,这样才能实现和服务进行交互。如果通过startService方式来启动服务,则不能与服务进行交互】
[html]
view plaincopyprint?
package com.andyidea.client;
import android.app.Activity;
import android.content.ComponentName;
import android.content.Intent;
import android.content.ServiceConnection;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import com.andyidea.aidl.IBook;
public class AIDLClientDemoActivity extends Activity {
private EditText numberText;
private TextView resultView;
private Button query;
private IBook bookQuery;
private BookConnection bookConn =
new BookConnection();
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
numberText = (EditText) this.findViewById(R.id.number);
resultView = (TextView) this.findViewById(R.id.resultView);
query = (Button)findViewById(R.id.query);
Intent service =
new Intent("com.andyidea.aidl.bookservice");
bindService(service, bookConn, BIND_AUTO_CREATE);
query.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String number =
numberText.getText().toString();
int num =
Integer.valueOf(number);
try {
resultView.setText(bookQuery.queryBook(num));
} catch (RemoteException e) {
e.printStackTrace();
}
}
});
}
@Override
protected void onDestroy() {
unbindService(bookConn);
super.onDestroy();
}
private final class BookConnection implements ServiceConnection{
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
// TODO Auto-generated method stub
bookQuery = IBook.Stub.asInterface(service);
}
@Override
public void onServiceDisconnected(ComponentName name) {
// TODO Auto-generated method stub
bookQuery = null;
}
}
}
package com.andyidea.client; import android.app.Activity; import android.content.ComponentName; import android.content.Intent; import android.content.ServiceConnection; import android.os.Bundle; import android.os.IBinder; import android.os.RemoteException; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import com.andyidea.aidl.IBook; public class AIDLClientDemoActivity extends Activity { private EditText numberText; private TextView resultView; private Button query; private IBook bookQuery; private BookConnection bookConn = new BookConnection(); /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); numberText = (EditText) this.findViewById(R.id.number); resultView = (TextView) this.findViewById(R.id.resultView); query = (Button)findViewById(R.id.query); Intent service = new Intent("com.andyidea.aidl.bookservice"); bindService(service, bookConn, BIND_AUTO_CREATE); query.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { String number = numberText.getText().toString(); int num = Integer.valueOf(number); try { resultView.setText(bookQuery.queryBook(num)); } catch (RemoteException e) { e.printStackTrace(); } } }); } @Override protected void onDestroy() { unbindService(bookConn); super.onDestroy(); } private final class BookConnection implements ServiceConnection{ @Override public void onServiceConnected(ComponentName name, IBinder service) { // TODO Auto-generated method stub bookQuery = IBook.Stub.asInterface(service); } @Override public void onServiceDisconnected(ComponentName name) { // TODO Auto-generated method stub bookQuery = null; } } }其中界面布局文件 main.xml 源码:
[html]
view plaincopyprint?
<?xml
version="1.0"
encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="书籍编号"
/>
<EditText
android:id="@+id/number"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/query"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="查询"/>
<TextView
android:id="@+id/resultView"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="书籍编号" /> <EditText android:id="@+id/number" android:layout_width="fill_parent" android:layout_height="wrap_content"/> <Button android:id="@+id/query" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="查询"/> <TextView android:id="@+id/resultView" android:layout_width="fill_parent" android:layout_height="wrap_content"/> </LinearLayout>至此,我们已经完成了B应用程序的代码实现部分,我们要先把A应用程序部署到机器上,然后我们再运行B应用程序。下面我们通过截图来看下程序运行的结果:

通过上面的截图,我们输入书籍编号 1,就可以查询出相应的书籍名称,到此,我们就可以了解了 Android应用中如何通过AIDL机制实现两个进程的通讯。
相关文章推荐
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- (4.1.18.6)Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android应用中通过AIDL机制实现进程间的通讯实例
- Android之通过AIDL机制实现进程间的通讯实例
- AIDL机制实现进程间的通讯实例
- Android开源项目之Music (二)--- AIDL实现IPC进程间通讯
- Android IPC机制(三)在Android Studio中使用AIDL实现跨进程方法调用
- Android四大组件应用系列——使用ContentProvider实现跨进程通讯
- Android AIDL实现两个APP间的跨进程通信实例
- Android:使用AIDL实现进程间通讯