中介者模式(Mediator Pattern)
2013-01-31 09:27
302 查看
设计模式 - 吕震宇
.NET设计模式系列文章
薛敬明的专栏
乐在其中设计模式(C#)
乐在其中设计模式(C#) - 中介者模式(Mediator Pattern)
[align=center]乐在其中设计模式(C#) - 中介者模式(Mediator Pattern)[/align]作者:webabcd
介绍
用一个中介对象来封装一系列的对象交互。中介者使各对象不需要显式地相互引用,从而使其耦合松散,而且可以独立地改变它们之间的交互。
示例
有一个Message实体类,某个对象对它的操作有Send()和Insert()方法,现在用一个中介对象来封装这一系列的对象交互。
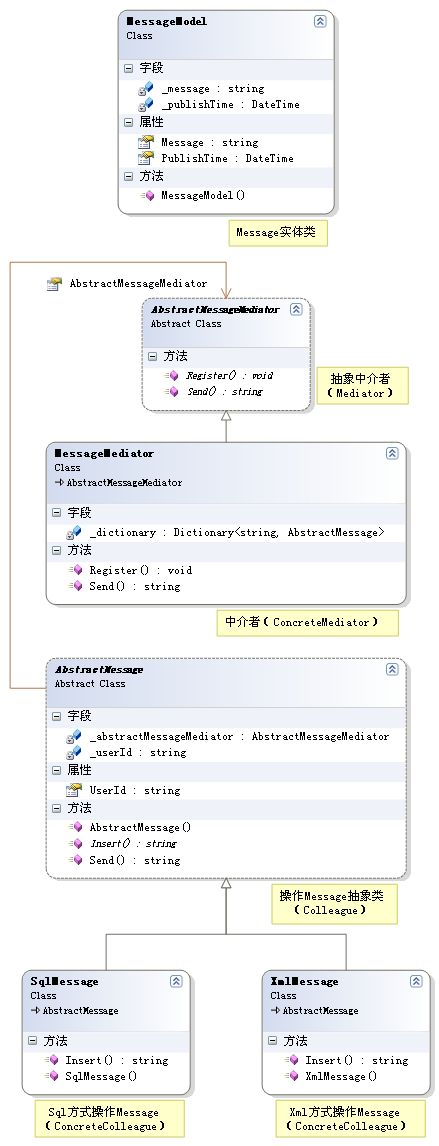
MessageModel

using System;

using System.Collections.Generic;

using System.Text;


namespace Pattern.Mediator

{

/// <summary>

/// Message实体类

/// </summary>

public class MessageModel

{

/// <summary>

/// 构造函数

/// </summary>

/// <param name="msg">Message内容</param>

/// <param name="pt">Message发布时间</param>

public MessageModel(string msg, DateTime pt)

{

this._message = msg;

this._publishTime = pt;

}


private string _message;

/// <summary>

/// Message内容

/// </summary>

public string Message

{

get { return _message; }

set { _message = value; }

}


private DateTime _publishTime;

/// <summary>

/// Message发布时间

/// </summary>

public DateTime PublishTime

{

get { return _publishTime; }

set { _publishTime = value; }

}

}

}

AbstractMessageMediator

using System;

using System.Collections.Generic;

using System.Text;


namespace Pattern.Mediator

{

/// <summary>

/// 抽象中介者(Mediator)

/// </summary>

public abstract class AbstractMessageMediator

{

/// <summary>

/// 注册一个操作Message的对象

/// </summary>

/// <param name="AbstractMessage">AbstractMessage</param>

public abstract void Register(AbstractMessage AbstractMessage);


/// <summary>

/// 发送Message

/// </summary>

/// <param name="from">来自UserId</param>

/// <param name="to">发送到UserId</param>

/// <param name="mm">Message实体对象</param>

/// <returns></returns>

public abstract string Send(string from, string to, MessageModel mm);

}

}

MessageMediator

using System;

using System.Collections.Generic;

using System.Text;


namespace Pattern.Mediator

{

/// <summary>

/// 中介者(ConcreteMediator)

/// </summary>

public class MessageMediator : AbstractMessageMediator

{

private Dictionary<string, AbstractMessage> _dictionary = new Dictionary<string, AbstractMessage>();


/// <summary>

/// 注册一个操作Message的对象

/// </summary>

/// <param name="abstractMessage">AbstractMessage</param>

public override void Register(AbstractMessage abstractMessage)

{

if (!_dictionary.ContainsKey(abstractMessage.UserId))

{

_dictionary.Add(abstractMessage.UserId, abstractMessage);

}


abstractMessage.AbstractMessageMediator = this;

}


/// <summary>

/// 发送Message

/// </summary>

/// <param name="from">来自UserId</param>

/// <param name="to">发送到UserId</param>

/// <param name="mm">Message实体对象</param>

/// <returns></returns>

public override string Send(string from, string to, MessageModel mm)

{

AbstractMessage abstractMessage = _dictionary[to];

if (abstractMessage != null)

{

return abstractMessage.Insert(from, mm);

}

else

{

return null;

}

}

}

}

AbstractMessage

using System;

using System.Collections.Generic;

using System.Text;


namespace Pattern.Mediator

{

/// <summary>

/// 操作Message抽象类(Colleague)

/// </summary>

public abstract class AbstractMessage

{

private AbstractMessageMediator _abstractMessageMediator;

private string _userId;


/// <summary>

/// 构造函数

/// </summary>

/// <param name="userId">UserId</param>

public AbstractMessage(string userId)

{

this._userId = userId;

}


/// <summary>

/// UserId

/// </summary>

public string UserId

{

get { return _userId; }

}


/// <summary>

/// 中介者

/// </summary>

public AbstractMessageMediator AbstractMessageMediator

{

get { return _abstractMessageMediator; }

set { _abstractMessageMediator = value; }

}


/// <summary>

/// 发送Message(由客户端调用)

/// </summary>

/// <param name="to">发送到UserId</param>

/// <param name="mm">Message实体对象</param>

/// <returns></returns>

public string Send(string to, MessageModel mm)

{

return _abstractMessageMediator.Send(_userId, to, mm);

}


/// <summary>

/// 接受Message(由中介者调用)

/// </summary>

/// <param name="from">来自UserId</param>

/// <param name="mm">Message实体对象</param>

/// <returns></returns>

public abstract string Insert(string from, MessageModel mm);

}

}

SqlMessage

using System;

using System.Collections.Generic;

using System.Text;


namespace Pattern.Mediator

{

/// <summary>

/// Sql方式操作Message(ConcreteColleague)

/// </summary>

public class SqlMessage : AbstractMessage

{

/// <summary>

/// 构造函数

/// </summary>

/// <param name="userId">UserId</param>

public SqlMessage(string userId)

: base(userId)

{


}


/// <summary>

/// 接受Message(由中介者调用)

/// </summary>

/// <param name="from">来自UserId</param>

/// <param name="mm">Message实体对象</param>

/// <returns></returns>

public override string Insert(string from, MessageModel mm)

{

return "Sql方式插入Message(" + from + "发送给" + base.UserId + ")"

+ " - 内容:" + mm.Message

+ " - 时间:" + mm.PublishTime.ToString();

}

}

}

XmlMessage

using System;

using System.Collections.Generic;

using System.Text;


namespace Pattern.Mediator

{

/// <summary>

/// Xml方式操作Message(ConcreteColleague)

/// </summary>

public class XmlMessage : AbstractMessage

{

/// <summary>

/// 构造函数

/// </summary>

/// <param name="userId">UserId</param>

public XmlMessage(string userId)

: base(userId)

{


}


/// <summary>

/// 接受Message(由中介者调用)

/// </summary>

/// <param name="from">来自UserId</param>

/// <param name="mm">Message实体对象</param>

/// <returns></returns>

public override string Insert(string from, MessageModel mm)

{

return "Xml方式插入Message(" + from + "发送给" + base.UserId + ")"

+ " - 内容:" + mm.Message

+ " - 时间:" + mm.PublishTime.ToString();

}

}

}

Test

using System;

using System.Data;

using System.Configuration;

using System.Collections;

using System.Web;

using System.Web.Security;

using System.Web.UI;

using System.Web.UI.WebControls;

using System.Web.UI.WebControls.WebParts;

using System.Web.UI.HtmlControls;


using Pattern.Mediator;


public partial class Mediator : System.Web.UI.Page

{

protected void Page_Load(object sender, EventArgs e)

{

AbstractMessageMediator messageMediator = new MessageMediator();


AbstractMessage user1 = new SqlMessage("user1");

AbstractMessage user2 = new SqlMessage("user2");

AbstractMessage user3 = new XmlMessage("user3");

AbstractMessage user4 = new XmlMessage("user4");


messageMediator.Register(user1);

messageMediator.Register(user2);

messageMediator.Register(user3);

messageMediator.Register(user4);


Response.Write(user1.Send("user2", new MessageModel("你好!", DateTime.Now)));

Response.Write("<br />");

Response.Write(user2.Send("user1", new MessageModel("我不好!", DateTime.Now)));

Response.Write("<br />");

Response.Write(user1.Send("user2", new MessageModel("不好就不好吧。", DateTime.Now)));

Response.Write("<br />");

Response.Write(user3.Send("user4", new MessageModel("吃了吗?", DateTime.Now)));

Response.Write("<br />");

Response.Write(user4.Send("user3", new MessageModel("没吃,你请我?", DateTime.Now)));

Response.Write("<br />");

Response.Write(user3.Send("user4", new MessageModel("不请。", DateTime.Now)));

Response.Write("<br />");

}

}

运行结果
Sql方式插入Message(user1发送给user2) - 内容:你好! - 时间:2007-5-19 23:43:19
Sql方式插入Message(user2发送给user1) - 内容:我不好! - 时间:2007-5-19 23:43:19
Sql方式插入Message(user1发送给user2) - 内容:不好就不好吧。 - 时间:2007-5-19 23:43:19
Xml方式插入Message(user3发送给user4) - 内容:吃了吗? - 时间:2007-5-19 23:43:19
Xml方式插入Message(user4发送给user3) - 内容:没吃,你请我? - 时间:2007-5-19 23:43:19
Xml方式插入Message(user3发送给user4) - 内容:不请。 - 时间:2007-5-19 23:43:19
参考
http://www.dofactory.com/Patterns/PatternMediator.aspx
OK
[源码下载]
设计模式22:Mediator Pattern (调解者模式)
英文原文:http://www.dofactory.com/Patterns/PatternMediator.aspx一、Mediator Pattern (调解者模式)的定义
Define an object that encapsulates how a set of objects interact. Mediator promotes loose coupling by keeping objects from referring to each other explicitly, and it lets you vary their interaction independently.定义一个对象将一系列对象如何相互作用的操作进行封装。调解者通过避免一个对象明确指向其余的对象来促使自由耦合,同时能够让你单独的改变他们之间的相互作用。
二、UML类图

Mediator (IChatroom)
定义一个接口用于与同事级对象进行通信
ConcreteMediator (Chatroom)
通过与同事级对象进行对等来实现相互协作行为
知道并且维持本身的同事级对象
Colleague classes (Participant)
每个同事级对象都知道自己的调节者对象
每个同事级对象在与另外的同事级对象进行通信时必须要与调节者进行通信。
三、调解者模式实例代码
[c-sharp]view plaincopy
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Mediator_Pattern
{
/// <summary>
/// MainApp startup class for Structural
/// Mediator Design Pattern.
/// </summary>
class MainApp
{
/// <summary>
/// Entry point into console application.
/// </summary>
static void Main()
{
ConcreteMediator m = new ConcreteMediator();
ConcreteColleague1 c1 = new ConcreteColleague1(m);
ConcreteColleague2 c2 = new ConcreteColleague2(m);
m.Colleague1 = c1;
m.Colleague2 = c2;
c1.Send("How are you?");
c2.Send("Fine, thanks");
// Wait for user
Console.ReadKey();
}
}
/// <summary>
/// The 'Mediator' abstract class
/// </summary>
abstract class Mediator
{
public abstract void Send(string message,
Colleague colleague);
}
/// <summary>
/// The 'ConcreteMediator' class
/// </summary>
class ConcreteMediator : Mediator
{
private ConcreteColleague1 _colleague1;
private ConcreteColleague2 _colleague2;
public ConcreteColleague1 Colleague1
{
set { _colleague1 = value; }
}
public ConcreteColleague2 Colleague2
{
set { _colleague2 = value; }
}
public override void Send(string message,
Colleague colleague)
{
if (colleague == _colleague1)
{
_colleague2.Notify(message);
}
else
{
_colleague1.Notify(message);
}
}
}
/// <summary>
/// The 'Colleague' abstract class
/// </summary>
abstract class Colleague
{
protected Mediator mediator;
// Constructor
public Colleague(Mediator mediator)
{
this.mediator = mediator;
}
}
/// <summary>
/// A 'ConcreteColleague' class
/// </summary>
class ConcreteColleague1 : Colleague
{
// Constructor
public ConcreteColleague1(Mediator mediator)
: base(mediator)
{
}
public void Send(string message)
{
mediator.Send(message, this);
}
public void Notify(string message)
{
Console.WriteLine("Colleague1 gets message: "
+ message);
}
}
/// <summary>
/// A 'ConcreteColleague' class
/// </summary>
class ConcreteColleague2 : Colleague
{
// Constructor
public ConcreteColleague2(Mediator mediator)
: base(mediator)
{
}
public void Send(string message)
{
mediator.Send(message, this);
}
public void Notify(string message)
{
Console.WriteLine("Colleague2 gets message: "
+ message);
}
}
}
四、另外一个使用调解者模式的实例代码
This real-world code demonstrates the Mediator pattern facilitating loosely coupled communication between different Participants registering with a Chatroom. The Chatroom is the central hub through which all communicationtakes place. At this point only one-to-one communication is implemented in the Chatroom, but would be trivial to change to one-to-many.
[c-sharp]
view plaincopy
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Mediator_Pattern
{
/// <summary>
/// MainApp startup class for Real-World
/// Mediator Design Pattern.
/// </summary>
class MainApp
{
/// <summary>
/// Entry point into console application.
/// </summary>
static void Main()
{
// Create chatroom
Chatroom chatroom = new Chatroom();
// Create participants and register them
Participant George = new Beatle("George");
Participant Paul = new Beatle("Paul");
Participant Ringo = new Beatle("Ringo");
Participant John = new Beatle("John");
Participant Yoko = new NonBeatle("Yoko");
chatroom.Register(George);
chatroom.Register(Paul);
chatroom.Register(Ringo);
chatroom.Register(John);
chatroom.Register(Yoko);
// Chatting participants
Yoko.Send("John", "Hi John!");
Paul.Send("Ringo", "All you need is love");
Ringo.Send("George", "My sweet Lord");
Paul.Send("John", "Can't buy me love");
John.Send("Yoko", "My sweet love");
// Wait for user
Console.ReadKey();
}
}
/// <summary>
/// The 'Mediator' abstract class
/// </summary>
abstract class AbstractChatroom
{
public abstract void Register(Participant participant);
public abstract void Send(
string from, string to, string message);
}
/// <summary>
/// The 'ConcreteMediator' class
/// </summary>
class Chatroom : AbstractChatroom
{
private Dictionary<string, Participant> _participants =
new Dictionary<string, Participant>();
public override void Register(Participant participant)
{
if (!_participants.ContainsValue(participant))
{
_participants[participant.Name] = participant;
}
participant.Chatroom = this;
}
public override void Send(
string from, string to, string message)
{
Participant participant = _participants[to];
if (participant != null)
{
participant.Receive(from, message);
}
}
}
/// <summary>
/// The 'AbstractColleague' class
/// </summary>
class Participant
{
private Chatroom _chatroom;
private string _name;
// Constructor
public Participant(string name)
{
this._name = name;
}
// Gets participant name
public string Name
{
get { return _name; }
}
// Gets chatroom
public Chatroom Chatroom
{
set { _chatroom = value; }
get { return _chatroom; }
}
// Sends message to given participant
public void Send(string to, string message)
{
_chatroom.Send(_name, to, message);
}
// Receives message from given participant
public virtual void Receive(
string from, string message)
{
Console.WriteLine("{0} to {1}: '{2}'",
from, Name, message);
}
}
/// <summary>
/// A 'ConcreteColleague' class
/// </summary>
class Beatle : Participant
{
// Constructor
public Beatle(string name)
: base(name)
{
}
public override void Receive(string from, string message)
{
Console.Write("To a Beatle: ");
base.Receive(from, message);
}
}
/// <summary>
/// A 'ConcreteColleague' class
/// </summary>
class NonBeatle : Participant
{
// Constructor
public NonBeatle(string name)
: base(name)
{
}
public override void Receive(string from, string message)
{
Console.Write("To a non-Beatle: ");
base.Receive(from, message);
}
}
}
四.中介者模式案例分析(Example)
1、场景
实现一个聊天室功能,聊城室就是一个中介者,参与聊天的人就是同事对象,如下图所示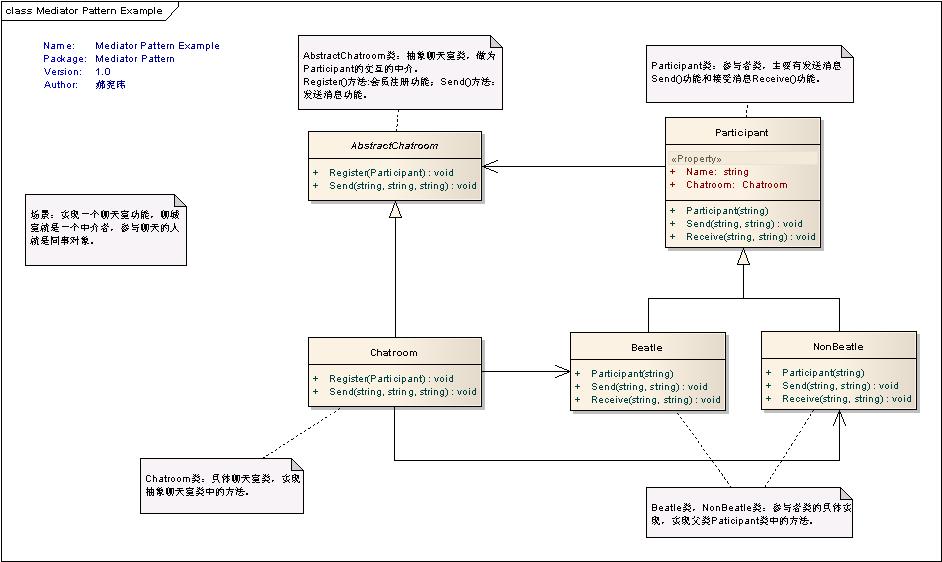
AbstractChatroom类:抽象聊天室类,做为Participant的交互的中介。
Register()方法:会员注册功能;Send()方法:发送消息功能。
Chatroom类:具体聊天室类,实现抽象聊天室类中的方法。
Participant类:参与者类,主要有发送消息Send()功能和接受消息Receive()功能。
Beatle类,NonBeatle类:参与者类的具体实现,实现父类Paticipant类中的方法。
2、代码
1、抽象聊天室类AbstractChatroom及其具体聊天室Chatroom |
/// <summary> /// The 'Mediator' abstract class /// </summary> abstract class AbstractChatroom { public abstract void Register(Participant participant); public abstract void Send(string from, string to, string message); } /// <summary> /// The 'ConcreteMediator' class /// </summary> class Chatroom : AbstractChatroom { private Dictionary<string, Participant> _participants =new Dictionary<string, Participant>(); public override void Register(Participant participant) { if (!_participants.ContainsValue(participant)) { _participants[participant.Name] = participant; } participant.Chatroom = this; } public override void Send(string from, string to, string message) { Participant participant = _participants[to]; if (participant != null) { participant.Receive(from, message); } } } |
2、参与者Participant类及其实现Beatle、NonBeatle |
/// <summary> /// The 'AbstractColleague' class /// </summary> class Participant { private Chatroom _chatroom; private string _name; // Constructor public Participant(string name) { this._name = name; } // Gets participant name public string Name { get { return _name; } } // Gets chatroom public Chatroom Chatroom { set { _chatroom = value; } get { return _chatroom; } } // Sends message to given participant public void Send(string to, string message) { _chatroom.Send(_name, to, message); } // Receives message from given participant public virtual void Receive(string from, string message) { Console.WriteLine("{0} to {1}: '{2}'",from, Name, message); } } /// <summary> /// A 'ConcreteColleague' class /// </summary> class Beatle : Participant { // Constructor public Beatle(string name) : base(name) { } public override void Receive(string from, string message) { Console.Write("To a Beatle: "); base.Receive(from, message); } } /// <summary> /// A 'ConcreteColleague' class /// </summary> class NonBeatle : Participant { // Constructor public NonBeatle(string name) : base(name) { } public override void Receive(string from, string message) { Console.Write("To a non-Beatle: "); base.Receive(from, message); } } |
3、客户端代码 |
static void Main(string[] args) { // Create chatroom Chatroom chatroom = new Chatroom(); // Create participants and register them Participant George = new Beatle("George"); Participant Paul = new Beatle("Paul"); Participant Ringo = new Beatle("Ringo"); Participant John = new Beatle("John"); Participant Yoko = new NonBeatle("Yoko"); chatroom.Register(George); chatroom.Register(Paul); chatroom.Register(Ringo); chatroom.Register(John); chatroom.Register(Yoko); // Chatting participants Yoko.Send("John", "Hi John!"); Paul.Send("Ringo", "All you need is love"); Ringo.Send("George", "My sweet Lord"); Paul.Send("John", "Can't buy me love"); John.Send("Yoko", "My sweet love"); // Wait for user Console.ReadKey(); } |
3、运行结果
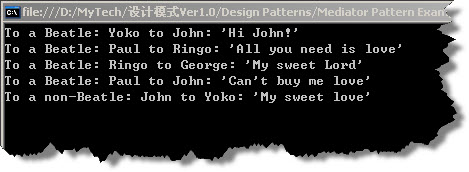
五、总结(Summary)
中介者模式(Mediator Pattern),定义一个中介对象来封装系列对象之间的交互。中介者使各个对象不需要显示地相互引用,从而使其耦合性松散,而且可以独立地改变他们之间的交互。中介者模式一般应用于一组对象以定义良好但是复杂的方法进行通信的场合,以及想定制一个分布在多个类中的行为,而不想生成太多的子类的场合。相关文章推荐
- java设计模式——中介者模式(Mediator Pattern)
- 第17章 中介者模式(Mediator Pattern)
- Java Mediator Pattern(中介者模式)
- 中介者模式(Mediator pattern)
- 中介者模式(Mediator Pattern)
- 极速理解设计模式系列:15.中介者模式(Mediator Pattern)
- Mediator Pattern(中介者模式)
- Mediator Design Pattern 中介者模式
- 设计模式之中介者模式---Mediator Pattern
- Java设计模式十二: 中介者模式(Mediator Pattern)
- 行为型---中介者模式(Mediator Pattern)
- 23种设计模式--中介者模式-Mediator Pattern
- 23种设计模式-10.中介者模式(Mediator Pattern)
- 极速理解设计模式系列:15.中介者模式(Mediator Pattern)
- C#设计模式之十七中介者模式(Mediator Pattern)【行为型】
- Mediator Pattern --中介者模式原理及实现(C++)
- 中介者模式(Mediator Pattern)。
- 中介者模式(Mediator pattern)
- 我所理解的设计模式(C++实现)——中介者模式(Mediator Pattern)
- 大话设计模式二十三章经(三) 中介者模式(Mediator Pattern)