Php设计模式之【外观模式Facade Pattern】
2012-05-21 11:31
821 查看
【案例】
黑枣设计公司开发一套流程图开发工具。使用这套开发工具在画一个箭头时,用户先画一个三角,再画一个坚杠就可以了。不过用户反馈画箭头太麻烦,希望能精简操作。
<代码模拟>
【分析OOA】
在应用进程中的一个步骤抱很许多复杂的逻辑步骤和方法调用时,创建一个外观模式的对象,使用外观模式隐藏了自调用对象的复杂性问题。我们可以新建一个箭头类,箭头类将代码中的step1\step2封装起来。对于用户来说,不用先画三角再画竖杠,直接调用箭头类就可以了。
【设计OOD】
<UML>
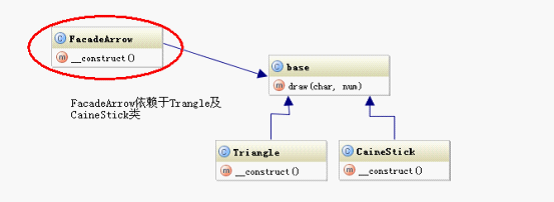
<说明>
外观类FacedeArrow:这个外观类为用户提供一个画箭头的简单接口,该类依赖于Triangle三角及CaineStick竖杠类
【编程 OOP】
<代码>
【测试用例Test Case】
<代码>
【输出】
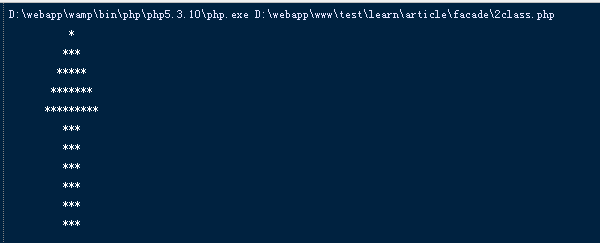
小结:
【模式实现要点】
外观模式是软件工程中常用的一种软件设计模式。它为子系统中的一组接口提供一个统一的高层接口。使用子系统更容易使用。
【优点】
外观模式作为结构型模式中的一个简单又实用的模式,外观模式通过封装细节来提供大粒度的调用,直接的好处就是,封装细节,提供了应用写程序的可维护性和易用性。
【适用场景】
外观模式一般应用在系统架构的服务层中,当我们是多个不同类型的客户端应用程序时,比如一个系统既可以在通过Web的形式访问,也可以通过客户端应用程序的形式时,可能通过外观模式来提供远程服务,让应用程序进行远程调用,这样通过外观形式提供服务,那么不管是什么样的客户端都访问一致的外观服务,那么以后就算是我们的应用服务发生变化,那么我们不需要修改没一个客户端应用的调用,只需要修改相应的外观应用即可。
********************************************
* 作者:叶文涛
* 标题:Php设计模式之【外观模式[Facade Pattern】
* 参考:
*《设计模式:可复用面向对象软件基础 》(美)Erich Gamma 等著
*《Head First设计模式》Eric Freeman等著
* http://www.cnblogs.com/hegezhou_hot/archive/2010/12/06/1897398.html
******************转载请注明网址来源 ***************
黑枣设计公司开发一套流程图开发工具。使用这套开发工具在画一个箭头时,用户先画一个三角,再画一个坚杠就可以了。不过用户反馈画箭头太麻烦,希望能精简操作。
<代码模拟>
class base { public function draw($char = '*', $num = 1) { echo str_repeat($char, $num); } } class Triangle extends base { public function __construct() { $this->draw(' ', 10); $this->draw('*', 1); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 8); $this->draw('*', 5); $this->draw("\n", 1); $this->draw(' ', 7); $this->draw('*', 7); $this->draw("\n", 1); $this->draw(' ', 6); $this->draw('*', 9); $this->draw("\n", 1); } } class CaineStick extends base { public function __construct() { $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); } } class testDriver { public function run() { $step1 = new Triangle(); $step2 = new CaineStick(); } } $test = new testDriver(); $test->run();
【分析OOA】
在应用进程中的一个步骤抱很许多复杂的逻辑步骤和方法调用时,创建一个外观模式的对象,使用外观模式隐藏了自调用对象的复杂性问题。我们可以新建一个箭头类,箭头类将代码中的step1\step2封装起来。对于用户来说,不用先画三角再画竖杠,直接调用箭头类就可以了。
【设计OOD】
<UML>
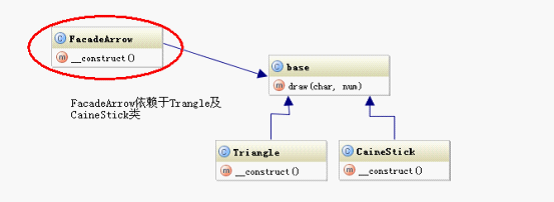
<说明>
外观类FacedeArrow:这个外观类为用户提供一个画箭头的简单接口,该类依赖于Triangle三角及CaineStick竖杠类
【编程 OOP】
<代码>
class base { public function draw($char = '*', $num = 1) { echo str_repeat($char, $num); } } class Triangle extends base { public function __construct() { $this->draw(' ', 10); $this->draw('*', 1); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 8); $this->draw('*', 5); $this->draw("\n", 1); $this->draw(' ', 7); $this->draw('*', 7); $this->draw("\n", 1); $this->draw(' ', 6); $this->draw('*', 9); $this->draw("\n", 1); } } class CaineStick extends base { public function __construct() { $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); $this->draw(' ', 9); $this->draw('*', 3); $this->draw("\n", 1); } } //箭头类 class FacadeArrow extends base { public function __construct(){ $step1 = new Triangle(); $step2 = new CaineStick(); } }
【测试用例Test Case】
<代码>
class testDriver { public function run() { $step1 = new FacadeArrow(); } } $test = new testDriver(); $test->run();
【输出】
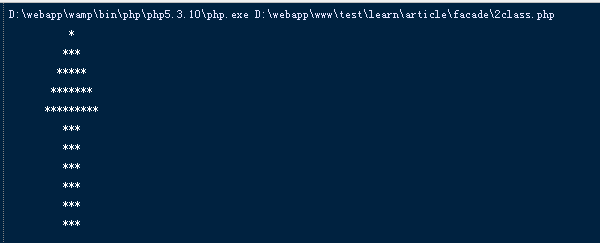
小结:
【模式实现要点】
外观模式是软件工程中常用的一种软件设计模式。它为子系统中的一组接口提供一个统一的高层接口。使用子系统更容易使用。
【优点】
外观模式作为结构型模式中的一个简单又实用的模式,外观模式通过封装细节来提供大粒度的调用,直接的好处就是,封装细节,提供了应用写程序的可维护性和易用性。
【适用场景】
外观模式一般应用在系统架构的服务层中,当我们是多个不同类型的客户端应用程序时,比如一个系统既可以在通过Web的形式访问,也可以通过客户端应用程序的形式时,可能通过外观模式来提供远程服务,让应用程序进行远程调用,这样通过外观形式提供服务,那么不管是什么样的客户端都访问一致的外观服务,那么以后就算是我们的应用服务发生变化,那么我们不需要修改没一个客户端应用的调用,只需要修改相应的外观应用即可。
********************************************
* 作者:叶文涛
* 标题:Php设计模式之【外观模式[Facade Pattern】
* 参考:
*《设计模式:可复用面向对象软件基础 》(美)Erich Gamma 等著
*《Head First设计模式》Eric Freeman等著
* http://www.cnblogs.com/hegezhou_hot/archive/2010/12/06/1897398.html
******************转载请注明网址来源 ***************
相关文章推荐
- 设计模式 - Facade Pattern(外观模式)
- 设计模式——外观模式(FacadePattern)
- 设计模式 - Facade Pattern(外观模式)
- 如何让孩子爱上设计模式 ——11.外观模式(Facade Pattern)
- 我读设计模式之外观模式(Facade Pattern)
- 解读设计模式----外观模式(Facade Pattern),谈阿牛讨媳妇故事
- 外观模式(Facade Pattern) - 最易懂的设计模式解析
- 设计模式笔记10:外观模式(Facade Pattern)
- 设计模式(十一)外观模式(Facade Pattern)
- 设计模式学习--适配器模式(Adapter Pattern)+外观模式(Facade Pattern)
- 设计模式(七)The Adapter and Facade Pattern 适配器模式与外观模式
- 【设计模式】 外观模式(Facade Pattern) - 最易懂的设计模式解析
- 乐在其中设计模式(C#) - 外观模式(Facade Pattern)
- 设计模式学习笔记---外观模式Facade pattern(Java版)
- 设计模式笔记10:外观模式(Facade Pattern)
- 设计模式之外观模式(Facade Pattern)
- 设计模式外观模式(FacadePattern)
- php设计模式之Proxy(代理模式)和Facade(外观)设计模式
- C#设计模式之十外观模式(Facade Pattern)【结构型】
- php设计模式 Facade(外观模式)