2012腾讯实习生内推面试题
2012-04-25 13:57
288 查看
1.链表逆置
[cpp]
view plaincopy
#include <iostream>
#include <ctime>
using namespace std;
struct node
{
int value;
node * next;
};
node* reverse(node * );
node * make_link();
void display(node *);
int main()
{
node* head = make_link();
display(head);
head = reverse(head);
display(head);
return 0;
}
node* reverse(node *head)
{
node *pre, *cur, *post;
if(!head || !head->next)
return head;
pre = head;
cur = pre->next;
while(cur)
{
post = cur->next;
cur->next = pre;
pre = cur;
cur = post;
}
head->next = NULL;
return pre;
}
node * make_link()
{
srand(time(NULL));
node *head = new node();
node *cur = head;
for(int i= 0; i < 10; i++)
{
cur->value = rand() % 10;
cur->next = new node();
cur = cur->next;
}
return head;
}
void display(node *head)
{
node *cur = head;
while(cur)
{
cout << cur->value << " " ;
cur = cur->next;
}
cout << endl;
}
2.链表合并,将两个有序的链表合并为一个
[cpp]
view plaincopy
#include <iostream>
#include <ctime>
using namespace std;
struct node
{
int value;
node * next;
};
node * make_link();
void display(node *);
void sort(node *);
node * merge(node *,node *);
int main()
{
node* head1 = make_link();
display(head1);
sort(head1);
node* head2 = make_link();
display(head2);
sort(head2);
node *head = merge(head1,head2);
display(head);
return 0;
}
node * make_link()
{
srand(time(NULL));
node *head = new node();
node *cur = head;
for(int i= 0; i < 10; i++)
{
cur->value = rand() % 10;
cur->next = new node();
cur = cur->next;
}
return head;
}
void display(node *head)
{
node *cur = head;
while(cur)
{
cout << cur->value << " " ;
cur = cur->next;
}
cout << endl;
}
void sort(node *head)
{
//cout << "sorting" << endl;
node *cur = head;
while(cur)
{
node *min = cur;
node *cur2 = cur->next;
while(cur2)
{
if(cur2->value < min->value)
{
min = cur2;
}
cur2 = cur2->next;
}
int tem = cur->value;
cur->value = min->value;
min->value = tem;
cur = cur->next;
}
}
node * merge(node *h1,node *h2)
{
node *mcur = new node();
node *cur1 = h1 , *cur2 = h2;
while(cur1 && cur2){
if(cur1->value < cur2->value){
mcur->next = cur1;
mcur = mcur->next;
cur1 = cur1->next;
}else{
mcur->next = cur2;
mcur = mcur->next;
cur2 = cur2->next;
}
}
if(cur1)
{
mcur->next = cur1;
}else
{
mcur->next = cur2;
}
return h1->value < h2->value ? h1 : h2;
}
3.一棵树是否某条路径结点之和等于给定值。并描述算法复杂度
[cpp]
view plaincopy
//基本思想:递归
#include <iostream>
#include <ctime>
using namespace std;
struct node
{
int value;
node *left;
node *right;
};
node * build_tree();
bool find(node *, int);
int main(void)
{
node *root = build_tree();
int t;
cin >> t;
cout << find(root,t);
return 0;
}
node * build_tree()
{
int a;
cin >> a;
if(a == 0)
{
return NULL;
}
node *root = new node();
root->value = a;
root->left = build_tree();
root->right = build_tree();
return root;
}
bool find(node *root,int v) //时间复杂度:
//平均要查找n条路径,路径上的平均节点数为树的高度——lg(n),对每个节点的操作为常量时间,所以时间复杂度为 n * lg(n)
{
if(root == NULL)
return false;
if(root->value == v)
return true;
else
return find(root->left, v-root->value) || find(root->right, v-root->value);
}
4.你熟悉的排序算法并描述算法复杂度。
[cpp]
view plaincopy
//快排:分难合易
//基本思想:先用partition()函数找到中位数,再分别对小于和大于中位数的那部分进行快速排序
#include <iostream>
using namespace std;
int partition(int a[], int b, int e)
{
int i = -1;
for(int j = 0; j < e; j++)
{
if(a[j] < a[e])
{
i++;
int tem = a[i];
a[i] = a[j];
a[j] = tem;
}
}
int tem = a[i + 1];
a[i + 1] = a[e];
a[e] = tem;
return i + 1;
}
void qsort(int a[], int b, int e)//时间复杂度:
{
if(b < e)
{
int m = partition(a,b,e);
qsort(a,b,m-1);
qsort(a,m+1,e);
}
}
int main()
{
int a[] = {2,9,4,1,6,8};
qsort(a, 0, 5);
for(int i = 0; i < 6; i++)
{
cout << a[i] << " ";
}
cout << endl;
return 0;
}
[cpp]
view plaincopy
//归并排序:分易合难
#include <iostream>
using namespace std;
void display(int a[], int size)
{
for(int i= 0; i < size; i++)
{
cout << a[i] << " ";
}
cout << endl;
}
void merge(int *a, int p, int q, int r)
{
int n1= q- p + 1;
int n2 = r - q;
int f[100], s[100];
for(int i = 0; i < n1; i++)
{
f[i] = a[p+i];
}
for(int i = 0; i < n2; i++)
{
s[i] = a[q + 1 + i];
}
f[n1] = 9999;//哨兵
s[n2] = 9999;
int i = 0, j = 0;
for(int k = p; k <= r; k++)
{
if(f[i] < s[j])
{
a[k] = f[i++];
}
else{
a[k] = s[j++];
}
}
}
void merge_sort(int *a, int b, int e)
{
if(b < e)
{
int p = (b + e) / 2;
merge_sort(a,b,p);
merge_sort(a,p+1,e);
merge(a,b,p,e);
}
}
int main()
{
int a[] = {9,3,5,7,6,8,10,22,21,34};
display(a, 10);
merge_sort(a, 0, 9);
display(a, 10);
return 0;
}
[cpp]
view plaincopy
//推排序
//a[0]存放数组大小,既方便得到数组大小,又方便堆中节点的父/子下标的计算
//基本思想:先从底往上建大根堆,然后每次将数组最后一个元素与堆的根元素交换,并将堆的大小减一,再保持堆的性质。 heapsort<-build_heap<-max_heapify
#include <iostream>
using namespace std;
void exchange(int &a,int &b)
{
int tem = a;
a = b;
b = tem;
}
void display(int a[])
{
for(int i= 1; i <= a[0]; i++)
{
cout << a[i] << " ";
}
cout << endl;
}
void max_heapify(int *a, int i)
{
int max = i;
int l = a[0];
if(2 * i <= l && a[2 * i] > a[max]){
max = 2 * i;
}
if(2 * i + 1 <= l && a[2 * i + 1] > a[max])
{
max = 2 * i + 1;
}
if(max != i)
{
exchange(a[i],a[max]);
max_heapify(a, max);
}
}
void build_max_heap(int *a)
{
int len = a[0];
for(int i = len/2; i > 0; i--)
{
max_heapify(a,i);
}
}
void heapsort(int a[])
{
int len = a[0];
for(int i = len;i > 1; i--)
{
exchange(a[i],a[1]);
a[0]--;
max_heapify(a,1);
}
a[0] = len;
}
int main()
{
int a[] = {9,3,5,7,6,8,10,22,21,34};
display(a);
build_max_heap(a);
display(a);
heapsort(a);
display(a);
return 0;
}
[cpp]
view plaincopy
//选择排序
//基本思想:
#include <iostream>
using namespace std;
void selectionSort(int a[], int n)
{
for(int i = 0; i < n - 1; i++)
{
int index = i;
for(int j = i + 1; j < n; j ++)
{
if(a[j] < a[i])
index = j;
}
int tem = a[i];
a[i] = a[index];
a[index] = tem;
}
}
int main()
{
int a[] = {2,1,7,5,4,3};
selectionSort(a, 6);
for(int i = 0; i < 6; i++)
{
cout << a[i] << endl;
}
}
[cpp]
view plaincopy
//插入排序
//基本思想:
#include <iostream>
using namespace std;
void insertionSort(int a[], int n)
{
for(int i = 1; i < n; i++)
{
int tem = a[i];
int j;
for(j = i - 1; j >= 0; j--)
{
if(a[j] > tem)
{
a[j + 1] = a[j];
}else
{
break;
}
}
a[j + 1] = tem;
}
}
int main()
{
int a[] = {2,1,7,5,4,3};
insertionSort(a, 6);
for(int i = 0; i < 6; i++)
{
cout << a[i] << endl;
}
}
5.二叉树与B+树的区别
ISAM树与B+树的区别
溢出时,ISAM树采用溢出页的方式解决,B+树则动态调整自身的结构(此外B+树的叶子页之间用指针链起来)
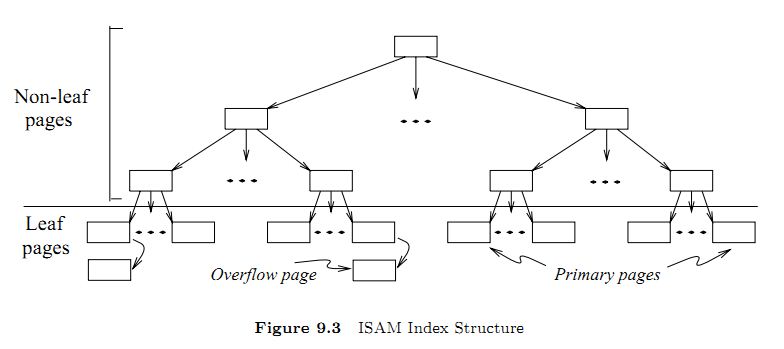

6.一个html文件有一千个<a>,写一个js程序,当点击某一个<a>时,能输出其对应的序号
7.用js实现继承,实现多继承
8.一棵要能快速查找,需要什么性质,怎么保持这个性质
9.hash表
[cpp]
view plaincopy
#include <iostream>
#include <ctime>
using namespace std;
struct node
{
int value;
node * next;
};
node* reverse(node * );
node * make_link();
void display(node *);
int main()
{
node* head = make_link();
display(head);
head = reverse(head);
display(head);
return 0;
}
node* reverse(node *head)
{
node *pre, *cur, *post;
if(!head || !head->next)
return head;
pre = head;
cur = pre->next;
while(cur)
{
post = cur->next;
cur->next = pre;
pre = cur;
cur = post;
}
head->next = NULL;
return pre;
}
node * make_link()
{
srand(time(NULL));
node *head = new node();
node *cur = head;
for(int i= 0; i < 10; i++)
{
cur->value = rand() % 10;
cur->next = new node();
cur = cur->next;
}
return head;
}
void display(node *head)
{
node *cur = head;
while(cur)
{
cout << cur->value << " " ;
cur = cur->next;
}
cout << endl;
}
2.链表合并,将两个有序的链表合并为一个
[cpp]
view plaincopy
#include <iostream>
#include <ctime>
using namespace std;
struct node
{
int value;
node * next;
};
node * make_link();
void display(node *);
void sort(node *);
node * merge(node *,node *);
int main()
{
node* head1 = make_link();
display(head1);
sort(head1);
node* head2 = make_link();
display(head2);
sort(head2);
node *head = merge(head1,head2);
display(head);
return 0;
}
node * make_link()
{
srand(time(NULL));
node *head = new node();
node *cur = head;
for(int i= 0; i < 10; i++)
{
cur->value = rand() % 10;
cur->next = new node();
cur = cur->next;
}
return head;
}
void display(node *head)
{
node *cur = head;
while(cur)
{
cout << cur->value << " " ;
cur = cur->next;
}
cout << endl;
}
void sort(node *head)
{
//cout << "sorting" << endl;
node *cur = head;
while(cur)
{
node *min = cur;
node *cur2 = cur->next;
while(cur2)
{
if(cur2->value < min->value)
{
min = cur2;
}
cur2 = cur2->next;
}
int tem = cur->value;
cur->value = min->value;
min->value = tem;
cur = cur->next;
}
}
node * merge(node *h1,node *h2)
{
node *mcur = new node();
node *cur1 = h1 , *cur2 = h2;
while(cur1 && cur2){
if(cur1->value < cur2->value){
mcur->next = cur1;
mcur = mcur->next;
cur1 = cur1->next;
}else{
mcur->next = cur2;
mcur = mcur->next;
cur2 = cur2->next;
}
}
if(cur1)
{
mcur->next = cur1;
}else
{
mcur->next = cur2;
}
return h1->value < h2->value ? h1 : h2;
}
3.一棵树是否某条路径结点之和等于给定值。并描述算法复杂度
[cpp]
view plaincopy
//基本思想:递归
#include <iostream>
#include <ctime>
using namespace std;
struct node
{
int value;
node *left;
node *right;
};
node * build_tree();
bool find(node *, int);
int main(void)
{
node *root = build_tree();
int t;
cin >> t;
cout << find(root,t);
return 0;
}
node * build_tree()
{
int a;
cin >> a;
if(a == 0)
{
return NULL;
}
node *root = new node();
root->value = a;
root->left = build_tree();
root->right = build_tree();
return root;
}
bool find(node *root,int v) //时间复杂度:
//平均要查找n条路径,路径上的平均节点数为树的高度——lg(n),对每个节点的操作为常量时间,所以时间复杂度为 n * lg(n)
{
if(root == NULL)
return false;
if(root->value == v)
return true;
else
return find(root->left, v-root->value) || find(root->right, v-root->value);
}
4.你熟悉的排序算法并描述算法复杂度。
[cpp]
view plaincopy
//快排:分难合易
//基本思想:先用partition()函数找到中位数,再分别对小于和大于中位数的那部分进行快速排序
#include <iostream>
using namespace std;
int partition(int a[], int b, int e)
{
int i = -1;
for(int j = 0; j < e; j++)
{
if(a[j] < a[e])
{
i++;
int tem = a[i];
a[i] = a[j];
a[j] = tem;
}
}
int tem = a[i + 1];
a[i + 1] = a[e];
a[e] = tem;
return i + 1;
}
void qsort(int a[], int b, int e)//时间复杂度:
{
if(b < e)
{
int m = partition(a,b,e);
qsort(a,b,m-1);
qsort(a,m+1,e);
}
}
int main()
{
int a[] = {2,9,4,1,6,8};
qsort(a, 0, 5);
for(int i = 0; i < 6; i++)
{
cout << a[i] << " ";
}
cout << endl;
return 0;
}
[cpp]
view plaincopy
//归并排序:分易合难
#include <iostream>
using namespace std;
void display(int a[], int size)
{
for(int i= 0; i < size; i++)
{
cout << a[i] << " ";
}
cout << endl;
}
void merge(int *a, int p, int q, int r)
{
int n1= q- p + 1;
int n2 = r - q;
int f[100], s[100];
for(int i = 0; i < n1; i++)
{
f[i] = a[p+i];
}
for(int i = 0; i < n2; i++)
{
s[i] = a[q + 1 + i];
}
f[n1] = 9999;//哨兵
s[n2] = 9999;
int i = 0, j = 0;
for(int k = p; k <= r; k++)
{
if(f[i] < s[j])
{
a[k] = f[i++];
}
else{
a[k] = s[j++];
}
}
}
void merge_sort(int *a, int b, int e)
{
if(b < e)
{
int p = (b + e) / 2;
merge_sort(a,b,p);
merge_sort(a,p+1,e);
merge(a,b,p,e);
}
}
int main()
{
int a[] = {9,3,5,7,6,8,10,22,21,34};
display(a, 10);
merge_sort(a, 0, 9);
display(a, 10);
return 0;
}
[cpp]
view plaincopy
//推排序
//a[0]存放数组大小,既方便得到数组大小,又方便堆中节点的父/子下标的计算
//基本思想:先从底往上建大根堆,然后每次将数组最后一个元素与堆的根元素交换,并将堆的大小减一,再保持堆的性质。 heapsort<-build_heap<-max_heapify
#include <iostream>
using namespace std;
void exchange(int &a,int &b)
{
int tem = a;
a = b;
b = tem;
}
void display(int a[])
{
for(int i= 1; i <= a[0]; i++)
{
cout << a[i] << " ";
}
cout << endl;
}
void max_heapify(int *a, int i)
{
int max = i;
int l = a[0];
if(2 * i <= l && a[2 * i] > a[max]){
max = 2 * i;
}
if(2 * i + 1 <= l && a[2 * i + 1] > a[max])
{
max = 2 * i + 1;
}
if(max != i)
{
exchange(a[i],a[max]);
max_heapify(a, max);
}
}
void build_max_heap(int *a)
{
int len = a[0];
for(int i = len/2; i > 0; i--)
{
max_heapify(a,i);
}
}
void heapsort(int a[])
{
int len = a[0];
for(int i = len;i > 1; i--)
{
exchange(a[i],a[1]);
a[0]--;
max_heapify(a,1);
}
a[0] = len;
}
int main()
{
int a[] = {9,3,5,7,6,8,10,22,21,34};
display(a);
build_max_heap(a);
display(a);
heapsort(a);
display(a);
return 0;
}
[cpp]
view plaincopy
//选择排序
//基本思想:
#include <iostream>
using namespace std;
void selectionSort(int a[], int n)
{
for(int i = 0; i < n - 1; i++)
{
int index = i;
for(int j = i + 1; j < n; j ++)
{
if(a[j] < a[i])
index = j;
}
int tem = a[i];
a[i] = a[index];
a[index] = tem;
}
}
int main()
{
int a[] = {2,1,7,5,4,3};
selectionSort(a, 6);
for(int i = 0; i < 6; i++)
{
cout << a[i] << endl;
}
}
[cpp]
view plaincopy
//插入排序
//基本思想:
#include <iostream>
using namespace std;
void insertionSort(int a[], int n)
{
for(int i = 1; i < n; i++)
{
int tem = a[i];
int j;
for(j = i - 1; j >= 0; j--)
{
if(a[j] > tem)
{
a[j + 1] = a[j];
}else
{
break;
}
}
a[j + 1] = tem;
}
}
int main()
{
int a[] = {2,1,7,5,4,3};
insertionSort(a, 6);
for(int i = 0; i < 6; i++)
{
cout << a[i] << endl;
}
}
5.二叉树与B+树的区别
ISAM树与B+树的区别
溢出时,ISAM树采用溢出页的方式解决,B+树则动态调整自身的结构(此外B+树的叶子页之间用指针链起来)
6.一个html文件有一千个<a>,写一个js程序,当点击某一个<a>时,能输出其对应的序号
7.用js实现继承,实现多继承
8.一棵要能快速查找,需要什么性质,怎么保持这个性质
9.hash表
相关文章推荐
- 腾讯2012实习生招聘面试题(部分)
- 腾讯2012实习生招聘面试题:矩阵中A移动到B一共有多少走法
- 腾讯2012实习生笔试题
- 腾讯2012实习生笔试题+答案解析
- 2012百度实习生面试题一道,打乱100个数的顺序,越乱越好
- 腾讯2012实习生面试
- 腾讯2012实习生笔试题2+答案解析
- 腾讯2012实习生笔试题+答案解析
- 腾讯2012实习生笔试题+答案解析
- 2016腾讯实习生面试题(前端、后台、开发、算法)
- 编写C++中的两个类 一个只能在栈中分配空间 一个只能在堆中分配(腾讯2012面试题)
- 2012腾讯春季实习生面试经历(二)
- 腾讯2012实习生笔试题2+答案解析
- 腾讯实习生入职报到注意点(2012)
- 2012腾讯春季实习生面试经历(二)
- 腾讯2012实习生笔试题+答案解析
- 腾讯2012实习生招聘笔试题
- 腾讯校园招聘前端实习生面试题及答案(1)
- 【笔试面试题】腾讯2013实习生面试算法题及参考答案
- 【LeetCode】Longest Palindromic Substring && 【九度】题目1528:最长回文子串(腾讯2013年实习生招聘二面面试题)