jsp分页技术的例子实现和总结
2011-09-07 22:16
579 查看
在jsp开发中,在前台的数据展示页面,经常遇到这样的情况,很多条的数据,有几十上百条,而只显示10条,然后翻页显示下面的十条,如下图所示:
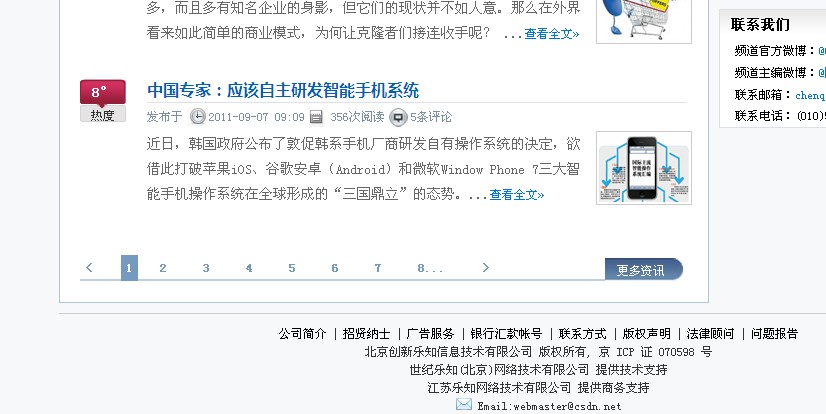
处理方式:一:首先sql语句,使用not in的方式处理,
在dao类里写一个方法
public List<> getAllByPage(int pageSize,pageNum){
......
String sql="select top"+pageSize +" * from 表名x where 字段A not in (select top "+pageSize*(pageNum-1)+" 字段A from 表名x)";
.......
rerun list;
}
其中,pageSize是页面要显示的条数,pageNum是要显示的页码,其中的sql语句基本是固定的,返回一个list对象。
二:在Biz里写一个方法,调用Dao里的这个getAllByPage方法,传入的也是两个参数,返回的也是list结果集。
三:在jsp页面以url传参的方式进行页面处理和数据的显示。
使用request对象获取传入的值,然后进行强制类型转换之后就可以作为参数,传入到Biz业务类的方法中去处理,返回的就是list结果集,然后使用表达式的方式在页面中进行显示就可以了。
综上:这就是分页显示数据的一个方法,
下面是一个小小的例子。
问题需求:开发一个小的留言板页面,页面能够以分页的形式显示所有留言信息,下放是一个发表留言的表单。
所有代码如下:
数据库如图:
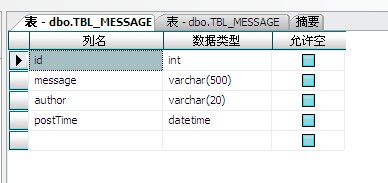
实体类(entity)如下:
dao类如下:(包括链接jdbc数据库)
Biz类如下(调用dao的相关方法来实现业务处理):
测试index页面代码如下:
处理表单提交的jsp页面doPost.jsp如下:
页面效果如下:
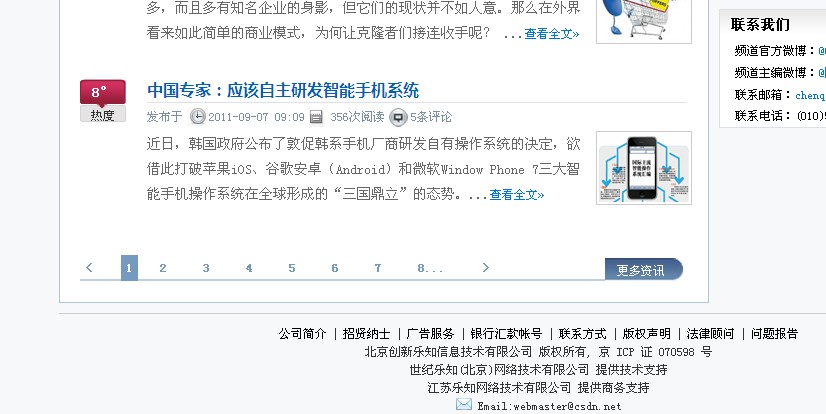
处理方式:一:首先sql语句,使用not in的方式处理,
在dao类里写一个方法
public List<> getAllByPage(int pageSize,pageNum){
......
String sql="select top"+pageSize +" * from 表名x where 字段A not in (select top "+pageSize*(pageNum-1)+" 字段A from 表名x)";
.......
rerun list;
}
其中,pageSize是页面要显示的条数,pageNum是要显示的页码,其中的sql语句基本是固定的,返回一个list对象。
二:在Biz里写一个方法,调用Dao里的这个getAllByPage方法,传入的也是两个参数,返回的也是list结果集。
三:在jsp页面以url传参的方式进行页面处理和数据的显示。
使用request对象获取传入的值,然后进行强制类型转换之后就可以作为参数,传入到Biz业务类的方法中去处理,返回的就是list结果集,然后使用表达式的方式在页面中进行显示就可以了。
综上:这就是分页显示数据的一个方法,
下面是一个小小的例子。
问题需求:开发一个小的留言板页面,页面能够以分页的形式显示所有留言信息,下放是一个发表留言的表单。
所有代码如下:
数据库如图:
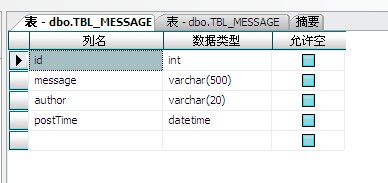
实体类(entity)如下:
package com.rz.entity; import java.util.Date; public class Message { private int id; //留言id private String message; //留言信息 private String author; //留言作者 private Date postTime; //留言时间 public int getId() { return id; } public void setId(int id) { this.id = id; } public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public Date getPostTime() { return postTime; } public void setPostTime(Date postTime) { this.postTime = postTime; } }
dao类如下:(包括链接jdbc数据库)
package com.rz.dao; import com.rz.entity.*; import java.sql.*; import java.util.*; public class MessageDao { private String driver="com.microsoft.sqlserver.jdbc.SQLServerDriver"; private String url="jdbc:sqlserver://localhost:1433;DataBaseName=message"; private String name="sa"; private String password="lishiyuzuji"; /** * 保存一条记录 * @param message * @return */ public int save(Message message){ int line=0; //保存记录的行数 Connection con=null; PreparedStatement pstat=null; try{ con=getConn(); pstat=con.prepareStatement("insert into TBL_MESSAGE(message,author,postTime) values(?,?,getdate())"); pstat.setString(1,message.getMessage()); pstat.setString(2,message.getAuthor()); line=pstat.executeUpdate(); }catch(SQLException e){ e.printStackTrace(); }finally{ closeAll(null,pstat,con); } return line; } /** * 分页显示信息 * @param pageSize 每页显示的信息数量 * @param pageNum 定位到那一页 * @return */ public List<Message> listByPage(int pageSize,int pageNum){ Connection con=null; PreparedStatement pstat=null; ResultSet res=null; List<Message> list=new ArrayList<Message>(); try{ con=getConn(); pstat=con.prepareStatement("select top "+pageSize+ " * from TBL_MESSAGE where id not in (select top "+pageSize*(pageNum-1)+ " id from TBL_MESSAGE order by postTime) order by postTime"); res=pstat.executeQuery(); while(res.next()){ Message message=new Message(); message.setId(res.getInt(1)); message.setMessage(res.getString(2)); message.setAuthor(res.getString(3)); message.setPostTime(res.getDate(4)); list.add(message); } }catch(SQLException e){ e.printStackTrace(); }finally{ closeAll(res,pstat,con); } return list; } /** * 获得记录的总条数 * @return 返回查询到的所有记录的总条数 */ public int getAllUserCount(){ Connection con=null; PreparedStatement pstat=null; ResultSet res=null; int ret = 0; try{ con=getConn(); pstat=con.prepareStatement("select count(*) from TBL_MESSAGE"); res=pstat.executeQuery(); if(res.next()){ ret=res.getInt(1); } }catch(SQLException e){ e.printStackTrace(); }finally{ closeAll(res,pstat,con); } return ret; } /** * 连接数据库 */ public Connection getConn(){ Connection con=null; try{ Class.forName(driver); con=DriverManager.getConnection(url,name,password); }catch(ClassNotFoundException e){ e.printStackTrace(); }catch(SQLException e){ e.printStackTrace(); } return con; } /** * 关闭数据库连接,而且三者是有顺序的,先关闭ResultSet连接,接着关闭Statement连接,最后关闭Connection连接 * 并且还要抛出异常。数据库连接使用完毕以后需要关闭连接的,否则,连接数据库的“通道”就会继续增加,而数据库的连接 * 能力也是有限的,大约是200个,因此需要在使用完数据库后将连接关闭掉。 * @param res * @param stat * @param conn */ public void closeAll(ResultSet res,PreparedStatement pstat,Connection con){ try{ if(res!=null){ res.close(); res=null; } if(pstat!=null){ pstat.close(); pstat=null; } if(con!=null){ con.close(); } }catch(SQLException e){ e.printStackTrace(); } } }
Biz类如下(调用dao的相关方法来实现业务处理):
package com.rz.Biz; import java.util.*; import com.rz.dao.*; import com.rz.entity.*; public class MessageBiz { /** * 保存一条信息 * @param message */ public void saveMessage(Message message){ MessageDao messageDao=new MessageDao(); messageDao.save(message); } /** * 根据页码来查询数据 * @param page * @return */ public List<Message> listByPage(int pageSize,int pageNum){ MessageDao messageDao=new MessageDao(); return messageDao.listByPage(pageSize,pageNum); } /** * 返回查询到的记录的总条数 * @return */ public int getAllUserCount(){ MessageDao messageDao=new MessageDao(); return messageDao.getAllUserCount(); } }
测试index页面代码如下:
<%@ page language="java" import="java.util.*,com.rz.Biz.*, com.rz.entity.*" contentType="text/html; charset=utf-8"%> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <title>留言板系统</title> <script> function check(){ var name=document.form.text.value; var pass=document.form.textarea.value; if(name.length==0){ alert("用户名不得为空!"); return false; } if(pass.length==0){ alert("留言信息不能为空!"); return false; } return true; } </script> </head> <body style="text-align:center;"> <h1>Beta留言板</h1> <div style="width:800px; border:#CCCCCC solid 1px;"> <% String p=request.getParameter("p"); int pageNum=1; //定义页数 int pageSize=10; //定义每一页显示的个数 if(p!=null){ //判断传入的输入值是否为空,并且进行强制类型转换 pageNum=Integer.parseInt(p); } MessageBiz messageBiz=new MessageBiz(); List<Message> list=messageBiz.listByPage(pageSize,pageNum); //根据传入的两个参数,返回一个list集合 for(int i=list.size()-1;i>=0;i--){ Message message=new Message(); message=(Message)list.get(i); %> <div style="width:800px;"> <div style="background-color:#CCCCCC; text-align:left;">作者:<%=message.getAuthor() %> <%=message.getPostTime() %> </div> <div style="text-align:right;"><%=i+1+(pageNum-1)*pageSize %>#</div> <div><%=message.getMessage() %> </div> </div> <% } //获得最大页码 int maxPage; int reCount=messageBiz.getAllUserCount(); if(reCount%pageSize==0) maxPage=reCount/pageSize; else maxPage=(reCount/pageSize)+1; int backPage=(pageNum==1) ? 1 :pageNum-1; int nextPage=(pageNum==maxPage) ? maxPage : pageNum+1; %> </div> <div style="width:800px; height:30px; border:#000033 solid 0px; margin-top:12px; text-align:right;"> <div style="width:50px; height:15px; border:#CCCCCC solid 1px; display:inline; cursor:pointer;"> <a href="index.jsp?p=<%=backPage %>">上一页</a> </div> <div style="width:50px; height:15px; border:#CCCCCC solid 1px; display:inline; margin-left:12px; margin-right:20px; cursor:pointer;"> <a href="index.jsp?p=<%=nextPage %>">下一页</a> </div> </div> <div style=" width:800px; height:300px; border:#333333 solid 0px;"> <form name="form" action="doPost.jsp" method="post" onSubmit="return check()"> <table> <tr> <td>用户名:</td> <td><input type="text" name="text"/></td> </tr> <tr> <td>留言信息:</td> <td><textarea name="textarea" style="width:400px; height:200px;"></textarea></td> </tr> <tr><td><input type="submit" value="提交"/></td></tr> </table> </form> </div> </body> </html>
处理表单提交的jsp页面doPost.jsp如下:
<%@ page language="java" import="java.util.*,com.rz.Biz.*, com.rz.entity.*" contentType="text/html; charset=utf-8"%> <% request.setCharacterEncoding("utf-8"); String author=request.getParameter("text"); String message=request.getParameter("textarea"); Message messages=new Message(); messages.setAuthor(author); messages.setMessage(message); MessageBiz messageBiz=new MessageBiz(); messageBiz.saveMessage(messages); response.sendRedirect("index.jsp"); %>
页面效果如下:
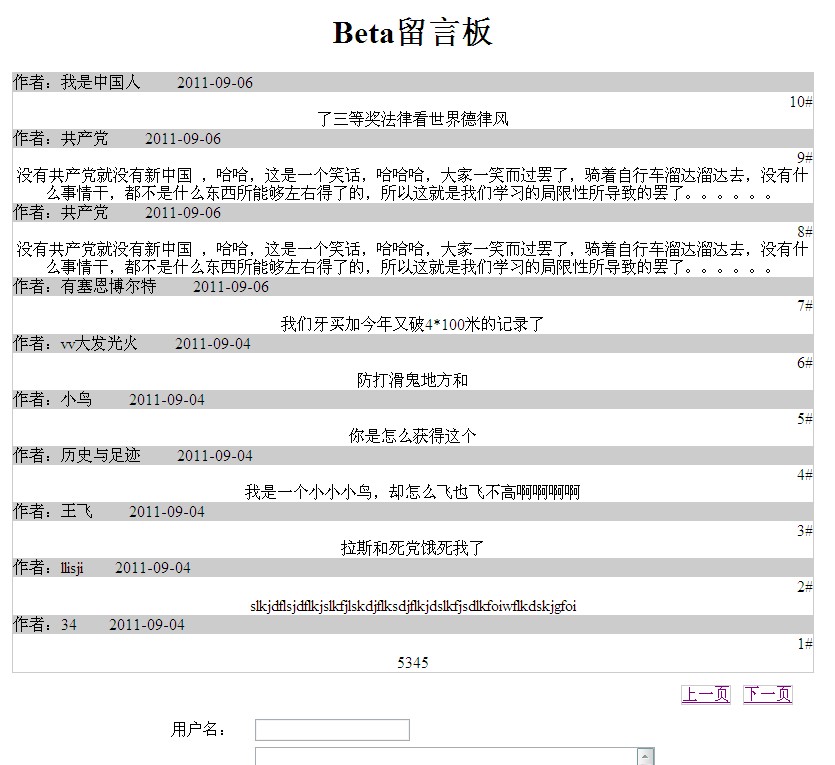
相关文章推荐
- JSP分页技术实现 (附代码)
- JSP分页技术实现
- JSP分页技术实现[转]
- JSP分页技术实现
- 01.关于使用Hibernate技术实现分页显示的思考总结
- JSP分页技术实现
- 说下jsp分页技术是怎么样实现的???
- JSP+Servlet技术实现分页 首页 下一页 每一页显示10条页码 下一页 尾页 第页/共页 (利用PageBean实现)
- JSP分页实现(以留言板为例子)
- JSP分页技术的实现(利用当前页进行前后加减,并利用href进行当前页面传值,传值当然是那个当前值变量)
- JSP分页技术实现
- 很好的jsp+servlet+javaBean实现数据分页代码例子
- jsp+servlet实现简单商品上传、商品浏览、与商品分页技术
- JSP分页技术实现
- JSP分页技术实现
- jsp读取数据库实现分页技术简析
- JSP分页技术实现
- JAVA_WEB项目(结合Servlet+jsp+ckEditor编辑器+jquery easyui技术)实现新闻发布管理系统第四篇:前台首页,新闻分类(体育新闻,科技新闻等),新闻列表分页的实现
- JSP分页技术实现
- 利用纯JSP技术实现分页效果