JSP+Servlet技术实现分页 首页 下一页 每一页显示10条页码 下一页 尾页 第页/共页 (利用PageBean实现)
2017-08-25 09:40
1211 查看
前端JSP页面的代码:
商品详情显示的代码:
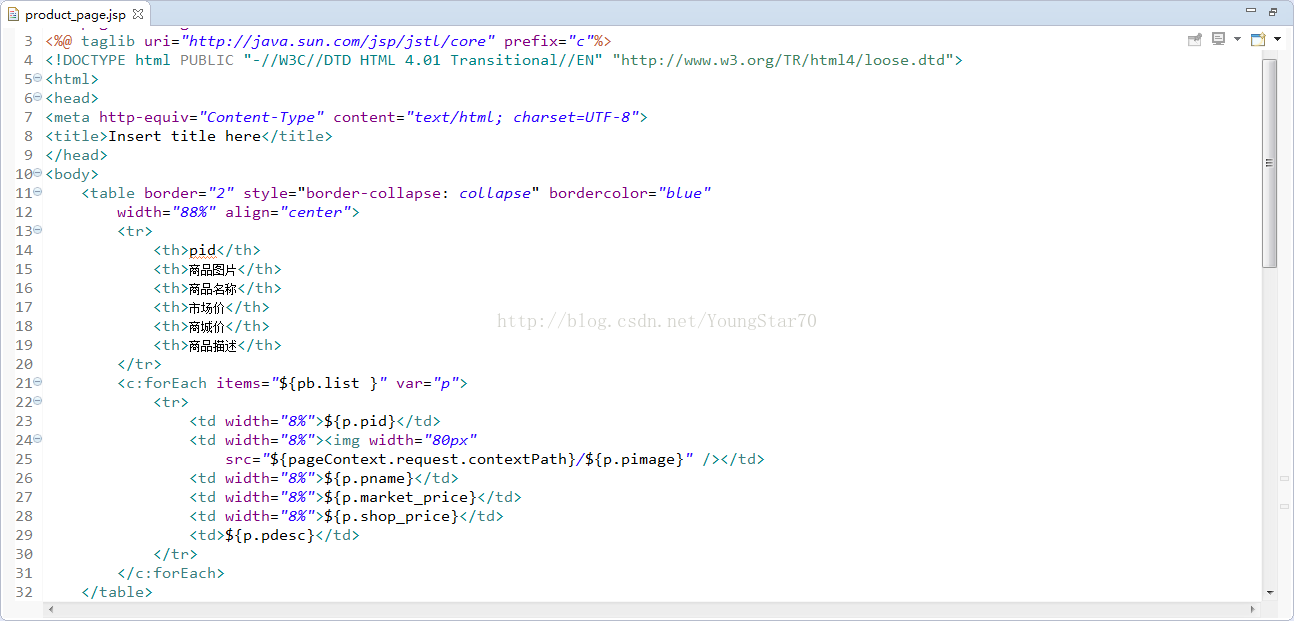
首页 上一页的代码

每一页显示10条页码的代码:
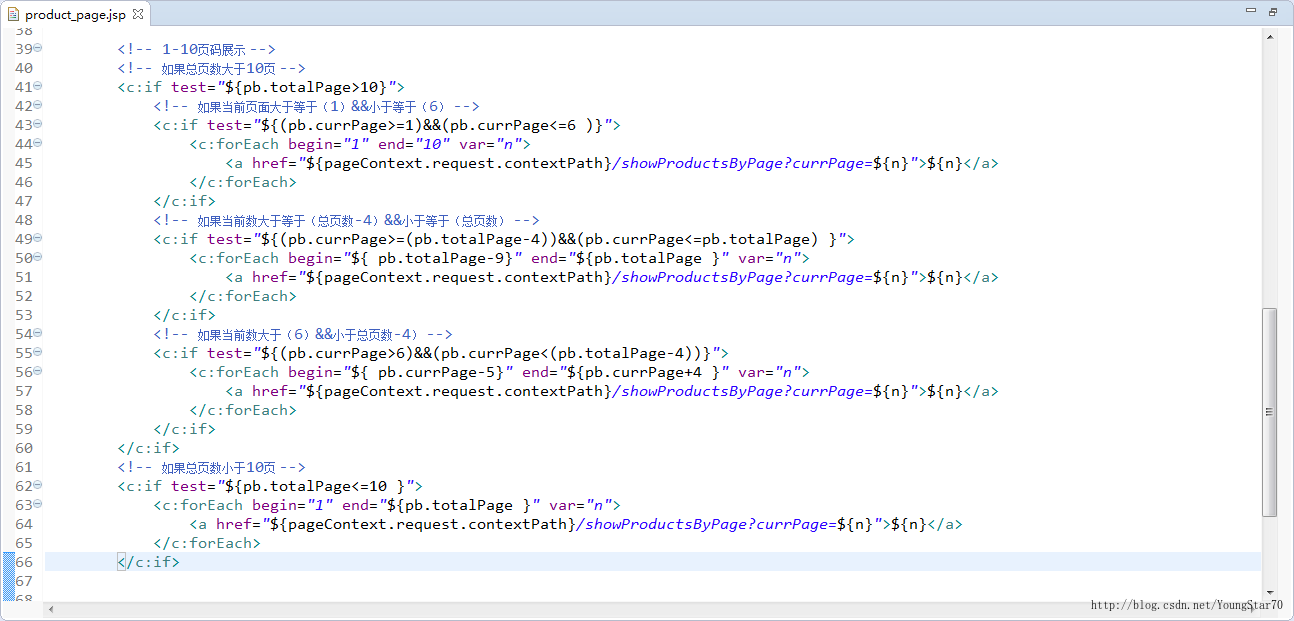
下一页 尾页 第页/共页的代码:

分页效果显示:
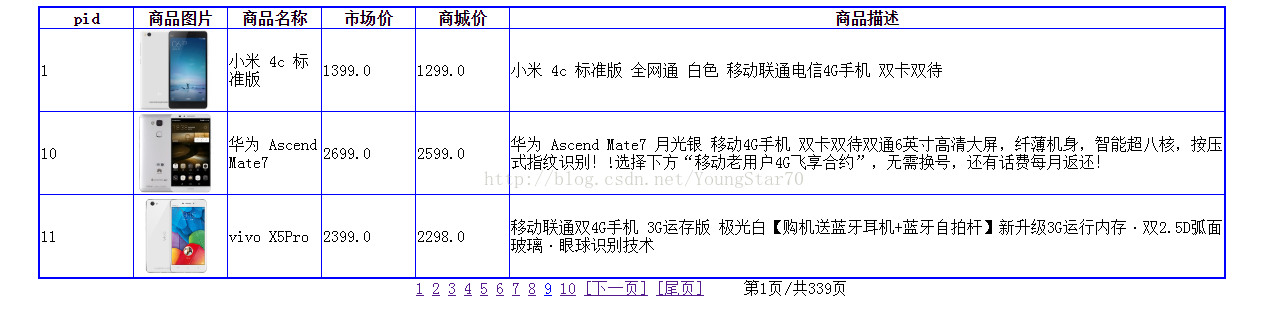
后端的代码:
PageBean的代码:
ShowProductsByPageServlet的代码:
ProductService的代码:
ProductDao的代码:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <table border="2" style="border-collapse: collapse" bordercolor="blue" width="88%" align="center"> <tr> <th>pid</th> <th>商品图片</th> <th>商品名称</th> <th>市场价</th> <th>商城价</th> <th>商品描述</th> </tr> <c:forEach items="${pb.list }" var="p"> <tr> <td width="8%">${p.pid}</td> <td width="8%"><img width="80px" src="${pageContext.request.contextPath}/${p.pimage}" /></td> <td width="8%">${p.pname}</td> <td width="8%">${p.market_price}</td> <td width="8%">${p.shop_price}</td> <td>${p.pdesc}</td> </tr> </c:forEach> </table> <center> <c:if test="${pb.currPage!=1 }"> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=1">[首页]</a> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=${pb.currPage-1}">[上一页]</a> </c:if> <!-- 1-10页码展示 --> <!-- 如果总页数大于10页 --> <c:if test="${pb.totalPage>10}"> <!-- 如果当前页面大于等于(1)&&小于等于(6) --> <c:if test="${(pb.currPage>=1)&&(pb.currPage<=6 )}"> <c:forEach begin="1" end="10" var="n"> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=${n}">${n}</a> </c:forEach> </c:if> <!-- 如果当前数大于等于(总页数-4)&&小于等于(总页数) --> <c:if test="${(pb.currPage>=(pb.totalPage-4))&&(pb.currPage<=pb.totalPage) }"> <c:forEach begin="${ pb.totalPage-9}" end="${pb.totalPage }" var="n"> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=${n}">${n}</a> </c:forEach> </c:if> <!-- 如果当前数大于(6)&&小于总页数-4) --> <c:if test="${(pb.currPage>6)&&(pb.currPage<(pb.totalPage-4))}"> <c:forEach begin="${ pb.currPage-5}" end="${pb.currPage+4 }" var="n"> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=${n}">${n}</a> </c:forEach> </c:if> </c:if> <!-- 如果总页数小于10页 --> <c:if test="${pb.totalPage<=10 }"> <c:forEach begin="1" end="${pb.totalPage }" var="n"> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=${n}">${n}</a> </c:forEach> </c:if> <c:if test="${pb.currPage!=pb.totalPage }"> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=${pb.currPage+1}">[下一页]</a> <a href="${pageContext.request.contextPath}/showProductsByPage?currPage=${pb.totalPage}">[尾页]</a> </c:if> 第${pb.currPage }页/共${pb.totalPage }页 </center> </body> </html>
商品详情显示的代码:
首页 上一页的代码
每一页显示10条页码的代码:
下一页 尾页 第页/共页的代码:
分页效果显示:
后端的代码:
PageBean的代码:
package com.itheima.domain; import java.util.List; public class PageBean<T> { private List<T> list; // 当前页内容 查询 private int currPage; // 当前页码 传递 private int pageSize; // 每页显示的条数 固定 private int totalCount; // 总条数 查询 private int totalPage; // 总页数 计算 public List<T> getList() { return list; } public void setList(List<T> list) { this.list = list; } public int getCurrPage() { return currPage; } public void setCurrPage(int currPage) { this.currPage = currPage; } public int getPageSize() { return pageSize; } public void setPageSize(int pageSize) { this.pageSize = pageSize; } public int getTotalCount() { return totalCount; } public void setTotalCount(int totalCount) { this.totalCount = totalCount; } public int getTotalPage() { return (int) Math.ceil(totalCount * 1.0 / pageSize); } public PageBean() { } public PageBean(List<T> list, int currPage, int pageSize, int totalCount) { super(); this.list = list; this.currPage = currPage; this.pageSize = pageSize; this.totalCount = totalCount; } }
ShowProductsByPageServlet的代码:
package com.itheima.web.servlet; import java.io.IOException; import java.sql.SQLException; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.itheima.domain.PageBean; import com.itheima.domain.Product; import com.itheima.service.ProductService; /** * 分页展示数据 */ public class ShowProductsByPageServlet extends HttpServlet { private static final long serialVersionUID = 1L; protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { int currPage = Integer.parseInt(request.getParameter("currPage")); int pageSize = 3; PageBean<Product> bean = null; try { bean = new ProductService().showProductsByPage(currPage, pageSize); } catch (SQLException e) { e.printStackTrace(); } request.setAttribute("pb", bean); request.getRequestDispatcher("/product_page.jsp").forward(request, response); } protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doGet(request, response); } }
ProductService的代码:
/** * 分页查询商品 * @param currPage 第几页 * @param pageSize 每页显示的条数 * @return * @throws SQLException */ public PageBean<Product> showProductsByPage(int currPage, int pageSize) throws SQLException { ProductDao dao=new ProductDao(); List<Product> list=dao.findProductByPage(currPage,pageSize); int totalCount=dao.getCount(); return new PageBean<>(list, currPage, pageSize, totalCount); }
ProductDao的代码:
public List<Product> findProductByPage(int currPage, int pageSize) throws SQLException { QueryRunner qr=new QueryRunner(DataSourceUtils.getDataSource()); String sql="select * from product limit ?,?"; return qr.query(sql, new BeanListHandler<>(Product.class),(currPage-1)*pageSize,pageSize); } public int getCount() throws SQLException { QueryRunner qr=new QueryRunner(DataSourceUtils.getDataSource()); String sql="select count(*) from product"; return ((Long)qr.query(sql, new ScalarHandler<>())).intValue(); }
相关文章推荐
- GridView自带分页 1总页数 首页 下一页 上一页 尾页 X 页 go 实现方法 .
- JAVA_WEB项目(结合Servlet+jsp+ckEditor编辑器+jquery easyui技术)实现新闻发布管理系统第四篇:前台首页,新闻分类(体育新闻,科技新闻等),新闻列表分页的实现
- jsp servlet javaBean实现oracle数据库分页显示
- (jsp 和 servlet功能篇) jsp+servlet+javaBean 实现分页代码(mysql 数据库)
- 利用ajax技术实现通用分页,在jsp页面呈现
- jsp+servlet+javabean实现数据分页
- 关于MVC中使用JqGrid插件分页时无法显示分页按钮(首页、上一页、下一页、最后一页)的原因
- JSP分页技术的实现(利用当前页进行前后加减,并利用href进行当前页面传值,传值当然是那个当前值变量)
- jsp+servlet+javaBean实现分页
- JSP+JavaBean+Servlet实现各类列表分页功能
- 分页对 首页,上一页,下一页,尾页按钮的处理
- 分页实现-----首页、上一页、下一页、最后一页
- jsp+Javabean+servlet实现分页查询
- Servlet+JavaBean+JSP真假分页技术详解
- JSP+JavaBean+Servlet实现分页
- GridView分页 记录总数:15 总页数:2 当前页:1 首页 上一页 下一页 尾页 GO
- JSP+servlet+javabean实现分页
- repeater 自定义分页 首页 上一页 下一页 尾页
- Shop项目--4. 分页显示商品的,与分页跳转,上一页,下一页product_list.jsp
- GridView自带分页 1/总页数 首页 下一页 上一页 尾页 X 页 go 实现方法