POJ 2299 Ultra-QuickSort
2010-07-08 19:25
274 查看
Ultra-QuickSort
Description
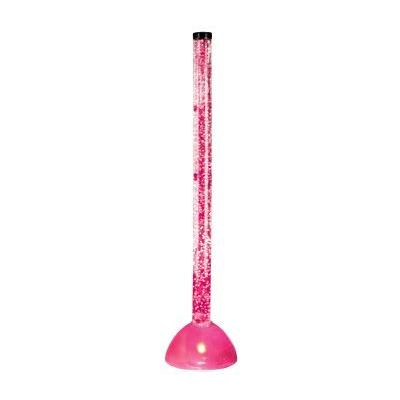
In this problem, you have to analyze a particular sorting algorithm. The algorithm processes a sequence of n distinct integers by swapping two adjacent sequence elements until the sequence is sorted in ascending order. For the input sequence
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
Sample Output
Source
Waterloo local 2005.02.05
合并排序写的程序
#include<stdio.h>
int a[500005],t[500005];
__int64 cnt;
void merge_sort(int x,int y)
{
if(y-x>1)
{
int m=x+(y-x)/2;
int p=x,q=m,i=x;
merge_sort(x,m);
merge_sort(m,y);
while(p<m||q<y)
{
if(q>=y||(p<m&&a[p]<=a[q])) t[i++]=a[p++];
else {t[i++]=a[q++];cnt+=m-p;}//要是是后面的数加到前面去,那么就产生了逆序数
}
for(i=x;i<y;i++) a[i]=t[i];
}
}
int main()
{
int n;
while(scanf("%d",&n)==1&&n)
{
for(int i=0;i<n;i++) scanf("%d",&a[i]);
cnt=0;
merge_sort(0,n);
printf("%I64d/n",cnt);
}
return 0;
}
两个正确的树状数组+离散化写的程序
#include<cstdio>
#include<algorithm>
#include<cstring>
using namespace std;
struct t
{
int num,id;
}a[500005];
int b[500005],n;//离散化后放重新排序的数组
__int64 tree[500005];
__int64 cnt;
bool cmp(t a,t b)
{
return a.num<b.num;
}
inline int Lowbit(int x)
{
return x&(-x);
}
void Update(int x)
{
for(int i=x;i<=n;i+=Lowbit(i))
tree[i]++;
}
__int64 Getsum(int x)
{
__int64 temp=0;
for(int i=x;i>0;i-=Lowbit(i))
temp+=tree[i];
return temp;
}
int main()
{
while((scanf("%d",&n),n)!=0)
{
cnt=0;
memset(tree,0,sizeof(tree));
memset(b,0,sizeof(b));
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].num);
//a[i].num=1000000000-a[i].num;
a[i].id=i;
}
sort(a+1,a+n+1,cmp);
b[a[1].id]=1;
for(int i=2;i<=n;i++) //离散化
{
if(a[a[i].id].num!=a[a[i-1].id].num)
b[a[i].id]=i;
else
b[a[i].id]=b[a[i-1].id];
}
/*for(int i=1;i<=n;i++)
{
cnt+=Getsum(b[i]);
Update(b[i]);
}*/
for(int i=1;i<=n;i++)
{
Update(b[i]);
cnt+=(Getsum(n)-Getsum(b[i]));
}
printf("%I64d/n",cnt);
}
return 0;
}
#include<cstdio>
#include<algorithm>
#include<cstring>
using namespace std;
struct t
{
int num,id;
}a[500005];
int b[500005],n;//离散化后放重新排序的数组
__int64 tree[500005];
__int64 cnt;
bool cmp(t a,t b)
{
return a.num<b.num;
}
inline int Lowbit(int x)
{
return x&(-x);
}
void Update(int x)
{
for(int i=x;i>0;i-=Lowbit(i))
tree[i]++;
}
__int64 Getsum(int x)
{
__int64 temp=0;
for(int i=x;i<=n;i+=Lowbit(i))
temp+=tree[i];
return temp;
}
int main()
{
while((scanf("%d",&n),n)!=0)
{
cnt=0;
memset(tree,0,sizeof(tree));
memset(b,0,sizeof(b));
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].num);
// a[i].num=1<<31-1-a[i].num;
a[i].id=i;
}
sort(a+1,a+n+1,cmp);
b[a[1].id]=1;
for(int i=2;i<=n;i++) //离散化
{
if(a[a[i].id].num!=a[a[i-1].id].num)
b[a[i].id]=i;
else
b[a[i].id]=b[a[i-1].id];
}
for(int i=1;i<=n;i++)
{
cnt+=Getsum(b[i]);
Update(b[i]);
}
/* for(int i=1;i<=n;i++)
{
Update(b[i]);
cnt+=(Getsum(n)-Getsum(b[i]));
}*/
printf("%I64d/n",cnt);
}
return 0;
}
错误的程序,还是不知道哪错了
#include<cstdio>
#include<algorithm>
#include<cstring>
using namespace std;
struct t
{
int num,id;
}a[500005];
int b[500005],n;//离散化后放重新排序的数组
__int64 tree[500005];
__int64 cnt;
bool cmp(t a,t b)
{
return a.num<b.num;
}
inline int Lowbit(int x)
{
return x&(-x);
}
void Update(int x)
{
for(int i=x;i<=n;i+=Lowbit(i))
tree[i]++;
}
__int64 Getsum(int x)
{
__int64 temp=0;
for(int i=x;i>0;i-=Lowbit(i))
temp+=tree[i];
return temp;
}
int main()
{
while((scanf("%d",&n),n)!=0)
{
cnt=0;
memset(tree,0,sizeof(tree));
memset(b,0,sizeof(b));
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].num);
a[i].num=1<<31-1-a[i].num;
a[i].id=i;
}
sort(a+1,a+n+1,cmp);
b[a[1].id]=1;
for(int i=2;i<=n;i++) //离散化
{
if(a[a[i].id].num!=a[a[i-1].id].num)
b[a[i].id]=i;
else
b[a[i].id]=b[a[i-1].id];
}
for(int i=1;i<=n;i++)
{
cnt+=Getsum(b[i]);
Update(b[i]);
}
/* for(int i=1;i<=n;i++)
{
Update(b[i]);
cnt+=(Getsum(n)-Getsum(b[i]));
}*/
printf("%I64d/n",cnt);
}
return 0;
}
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 15420 | Accepted: 5435 |
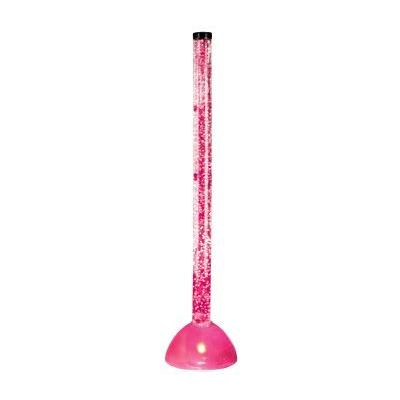
In this problem, you have to analyze a particular sorting algorithm. The algorithm processes a sequence of n distinct integers by swapping two adjacent sequence elements until the sequence is sorted in ascending order. For the input sequence
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
Source
Waterloo local 2005.02.05
合并排序写的程序
#include<stdio.h>
int a[500005],t[500005];
__int64 cnt;
void merge_sort(int x,int y)
{
if(y-x>1)
{
int m=x+(y-x)/2;
int p=x,q=m,i=x;
merge_sort(x,m);
merge_sort(m,y);
while(p<m||q<y)
{
if(q>=y||(p<m&&a[p]<=a[q])) t[i++]=a[p++];
else {t[i++]=a[q++];cnt+=m-p;}//要是是后面的数加到前面去,那么就产生了逆序数
}
for(i=x;i<y;i++) a[i]=t[i];
}
}
int main()
{
int n;
while(scanf("%d",&n)==1&&n)
{
for(int i=0;i<n;i++) scanf("%d",&a[i]);
cnt=0;
merge_sort(0,n);
printf("%I64d/n",cnt);
}
return 0;
}
两个正确的树状数组+离散化写的程序
#include<cstdio>
#include<algorithm>
#include<cstring>
using namespace std;
struct t
{
int num,id;
}a[500005];
int b[500005],n;//离散化后放重新排序的数组
__int64 tree[500005];
__int64 cnt;
bool cmp(t a,t b)
{
return a.num<b.num;
}
inline int Lowbit(int x)
{
return x&(-x);
}
void Update(int x)
{
for(int i=x;i<=n;i+=Lowbit(i))
tree[i]++;
}
__int64 Getsum(int x)
{
__int64 temp=0;
for(int i=x;i>0;i-=Lowbit(i))
temp+=tree[i];
return temp;
}
int main()
{
while((scanf("%d",&n),n)!=0)
{
cnt=0;
memset(tree,0,sizeof(tree));
memset(b,0,sizeof(b));
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].num);
//a[i].num=1000000000-a[i].num;
a[i].id=i;
}
sort(a+1,a+n+1,cmp);
b[a[1].id]=1;
for(int i=2;i<=n;i++) //离散化
{
if(a[a[i].id].num!=a[a[i-1].id].num)
b[a[i].id]=i;
else
b[a[i].id]=b[a[i-1].id];
}
/*for(int i=1;i<=n;i++)
{
cnt+=Getsum(b[i]);
Update(b[i]);
}*/
for(int i=1;i<=n;i++)
{
Update(b[i]);
cnt+=(Getsum(n)-Getsum(b[i]));
}
printf("%I64d/n",cnt);
}
return 0;
}
#include<cstdio>
#include<algorithm>
#include<cstring>
using namespace std;
struct t
{
int num,id;
}a[500005];
int b[500005],n;//离散化后放重新排序的数组
__int64 tree[500005];
__int64 cnt;
bool cmp(t a,t b)
{
return a.num<b.num;
}
inline int Lowbit(int x)
{
return x&(-x);
}
void Update(int x)
{
for(int i=x;i>0;i-=Lowbit(i))
tree[i]++;
}
__int64 Getsum(int x)
{
__int64 temp=0;
for(int i=x;i<=n;i+=Lowbit(i))
temp+=tree[i];
return temp;
}
int main()
{
while((scanf("%d",&n),n)!=0)
{
cnt=0;
memset(tree,0,sizeof(tree));
memset(b,0,sizeof(b));
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].num);
// a[i].num=1<<31-1-a[i].num;
a[i].id=i;
}
sort(a+1,a+n+1,cmp);
b[a[1].id]=1;
for(int i=2;i<=n;i++) //离散化
{
if(a[a[i].id].num!=a[a[i-1].id].num)
b[a[i].id]=i;
else
b[a[i].id]=b[a[i-1].id];
}
for(int i=1;i<=n;i++)
{
cnt+=Getsum(b[i]);
Update(b[i]);
}
/* for(int i=1;i<=n;i++)
{
Update(b[i]);
cnt+=(Getsum(n)-Getsum(b[i]));
}*/
printf("%I64d/n",cnt);
}
return 0;
}
错误的程序,还是不知道哪错了
#include<cstdio>
#include<algorithm>
#include<cstring>
using namespace std;
struct t
{
int num,id;
}a[500005];
int b[500005],n;//离散化后放重新排序的数组
__int64 tree[500005];
__int64 cnt;
bool cmp(t a,t b)
{
return a.num<b.num;
}
inline int Lowbit(int x)
{
return x&(-x);
}
void Update(int x)
{
for(int i=x;i<=n;i+=Lowbit(i))
tree[i]++;
}
__int64 Getsum(int x)
{
__int64 temp=0;
for(int i=x;i>0;i-=Lowbit(i))
temp+=tree[i];
return temp;
}
int main()
{
while((scanf("%d",&n),n)!=0)
{
cnt=0;
memset(tree,0,sizeof(tree));
memset(b,0,sizeof(b));
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].num);
a[i].num=1<<31-1-a[i].num;
a[i].id=i;
}
sort(a+1,a+n+1,cmp);
b[a[1].id]=1;
for(int i=2;i<=n;i++) //离散化
{
if(a[a[i].id].num!=a[a[i-1].id].num)
b[a[i].id]=i;
else
b[a[i].id]=b[a[i-1].id];
}
for(int i=1;i<=n;i++)
{
cnt+=Getsum(b[i]);
Update(b[i]);
}
/* for(int i=1;i<=n;i++)
{
Update(b[i]);
cnt+=(Getsum(n)-Getsum(b[i]));
}*/
printf("%I64d/n",cnt);
}
return 0;
}
相关文章推荐
- POJ 2299 -Ultra-QuickSort-树状数组求逆序数
- POJ2299——Ultra-QuickSort(归并排序)
- [POJ] 2299 -> Ultra-QuickSort
- poj 2299 Ultra-QuickSort(树状数组求逆序数+离散化)
- poj 2299 Ultra-QuickSort
- poj2299——Ultra-QuickSort(归并排序)
- POJ 2299 Ultra-QuickSort
- POJ 2299 Ultra-QuickSort
- poj-2299-Ultra-QuickSort
- POJ 2299 Ultra-QuickSort(逆序对数,线段树/树状数组/归并排序)
- POJ 2299 Ultra-QuickSort(线段树+离散化)
- POJ 2299-Ultra-QuickSort (树状数组+离散化)
- POJ 2299 - Ultra-QuickSort 统计逆序对
- POJ 2299 Ultra-QuickSort
- POJ 2299 Ultra-QuickSort
- POJ2299-Ultra-QuickSort(树状数组+离散化)
- POJ2299-Ultra-QuickSort
- POJ 2299 Ultra-QuickSort
- POJ 2299 Ultra-QuickSort
- Poj 2299 Ultra-QuickSort (归排求逆序数)