13、C 语言中的变量和关键字
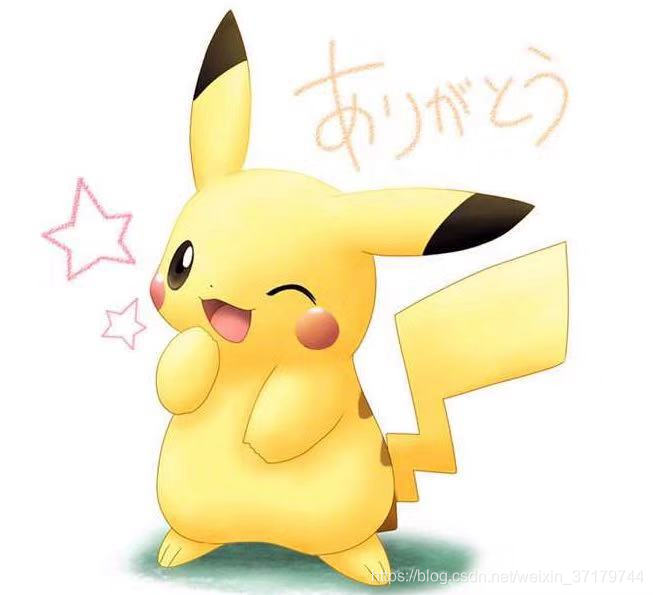
目录
13、C 语言中的变量和关键字
简单地说,变量是一个存储空间,其中分配了一些内存。基本上,用于存储某种形式数据的变量。不同类型的变量需要不同数量的内存,并且有一些可以应用于它们的特定操作集。
变量声明:
一个典型的变量声明语法为:
type variable_name; or for multiple variables: type variable1_name, variable2_name, variable3_name;
变量名可以由字母(大写和小写)、数字和下划线组成。但是,名称不能以数字开头。
变量申明与定义的不同:
变量声明是指在第一次使用变量之前首先声明或引入变量的部分。变量定义是为变量分配内存位置和值的部分。大多数情况下,变量声明和定义是一起完成的。请参阅以下 C 语言程序以获得更好的说明:
#include <stdio.h> int main() { // declaration and definition of variable 'a123' char a123 = 'a'; // This is also both declaration and definition as 'b' is allocated // memory and assigned some garbage value. float b; // multiple declarations and definitions int _c, _d45, e; // Let us print a variable printf("%c \n", a123); return 0; }
单独的声明和定义是否可以?对于外部变量和函数,这是可能的,更多细节参阅这里。
关键字是 C 语言中的特定保留词,每个保留词都有与之相关联的特定特征。几乎所有帮助我们使用 C 语言功能的单词都包含在关键字列表中。所以你可以想象,关键字列表不会太小!
下面是 C 语言的总共 32 个关键字列表:
auto break case char const continue default do double else enum extern float for goto if int long register return short signed sizeof static struct switch typedef union unsigned void volatile while
这些关键字中的大多数已经在 C 语言的各个小节中讨论过了,比如数据类型、存储类、控制语句、函数等。下面让我们讨论一些其他关键字,这些关键字允许我们使用 C 的基本功能:
const: const 可用于声明常量变量。常量变量是初始化时不能更改其值的变量。或者换句话说,分配给它们的值不能在程序中进一步被修改。具体语法为:
const data_type var_name = var_value;
注意:常量变量必须在声明期间初始化。const关键字也用于指针。
extern: extern 只是告诉我们变量是在别处定义的,而不是在使用它的同一块中定义的。基本上,该值是在不同的块中分配给它的,它也可以在不同的块中被覆盖/更改。因此,外部变量只不过是一个全局变量,它是用合法值初始化的,在该值被声明后以便在其他地方使用。它可以在任何函数/块中访问。此外,通过在任何函数/块中的声明/定义之前放置“extern”关键字,也可以将普通全局变量设置为 extern。这基本上意味着我们没有初始化一个新变量,而是只使用/访问全局变量。使用外部变量的主要目的是可以在作为大型程序一部分的两个不同文件之间访问它们。其语法为:
extern data_type var_name = var_value;
static: static 关键字用于声明静态变量,这些变量在用 C 语言编写程序时很常用。静态变量有一个属性,即使在超出其作用域后也能保持其值!因此,静态变量保留其作用域中最后一次使用的值。所以我们可以说,它们只初始化一次,直到程序结束。因此,没有分配新内存,因为它们没有重新声明。它们的作用域是定义它们的函数的局部作用域。全局静态变量可以被该文件中的任何位置访问到,因为它们的作用域是该文件。默认情况下,编译器为它们分配值 0。其语法为:
static data_type var_name = var_value;
void: voidb是一种特殊的数据类型。但是,是什么让它如此特别呢?void,顾名思义,是一个空数据类型。它意味着它什么都没有,或者它没有值。例如,当它用作函数的返回数据类型时,它只是表示函数不返回值。类似地,当它添加到函数参数中时,它表示该函数不接受任何参数。
注意:void 对于指针也有重要的用途。
typedef: 用于给已经存在的或甚至自定义的数据类型(如结构)赋予新名称。它有时非常方便,例如,在您定义的结构名称很长的情况下,或者只需要一个现有数据类型的简称。
下面的代码演示了上述几个关键词的示例:
#include <stdio.h> // declaring and initializing an extern variable extern int x = 9; // declaring and initializing a global variable // simply int z; would have initialized z with // the default value of a global variable which is 0 int z=10; // using typedef to give a short name to long long int // very convenient to use now due to the short name typedef long long int LL; // function which prints square of a no. and which has void as its // return data type void calSquare(int arg) { printf("The square of %d is %d\n",arg,arg*arg); } // Here void means function main takes no parameters int main(void) { // declaring a constant variable, its value cannot be modified const int a = 32; // declaring a char variable char b = 'G'; // telling the compiler that the variable z is an extern variable // and has been defined elsewhere (above the main function) extern int z; LL c = 1000000; printf("Hello World!\n"); // printing the above variables printf("This is the value of the constant variable 'a': %d\n",a); printf("'b' is a char variable. Its value is %c\n",b); printf("'c' is a long long int variable. Its value is %lld\n",c); printf("These are the values of the extern variables 'x' and 'z'" " respectively: %d and %d\n",x,z); // value of extern variable x modified x=2; // value of extern variable z modified z=5; // printing the modified values of extern variables 'x' and 'z' printf("These are the modified values of the extern variables" " 'x' and 'z' respectively: %d and %d\n",x,z); // using a static variable printf("The value of static variable 'y' is NOT initialized to 5 after the " "first iteration! See for yourself :)\n"); while (x > 0) { static int y = 5; y++; // printing value at each iteration printf("The value of y is %d\n",y); x--; } // print square of 5 calSquare(5); printf("Bye! See you soon. :)\n"); return 0; }
Output:
Hello World This is the value of the constant variable 'a': 32 'b' is a char variable. Its value is G 'c' is a long long int variable. Its value is 1000000 These are the values of the extern variables 'x' and 'z' respectively: 9 and 10 These are the modified values of the extern variables 'x' and 'z' respectively: 2 and 5 The value of static variable 'y' is NOT initialized to 5 after the first iteration! See for yourself :) The value of y is 6 The value of y is 7 The square of 5 is 25 Bye! See you soon. :)
This article is contributed by Ayush Jaggi.
- 点赞
- 收藏
- 分享
- 文章举报

- 黑马程序员----Java语言基础1(关键字、标识符、注释、常量和变量、运算符)
- 黑马程序员——C语言基础语法--关键字、数据、变量
- Go语言学习笔记 -- 变量、类型和关键字
- 02.Java语言基本语法之关键字、标识符、编码、变量、进制和运算符
- 基础快速过 之C语言 二:什么是关键字 标识符 系统保留字 变量和常量[各个语言通用]
- Java基础第一阶段——02_Java语言基础_关键字&变量&运算
- C语言基础-12-static和extern关键字2-对变量的作用
- 黑马程序员——OC语言学习——Xcode开发初始化、点语法、成员变量作用域、关键字@property和@synthesize
- Go语言学习笔记(二) [变量、类型、关键字]
- 黑马程序员--Java语言基础组成 —关键字、常量与变量
- C语言基础:关键字符号与变量
- 黑马程序员——Java语言基础(一)---关键字、标识符、注释、常量、变量、运算符
- DirectX 3D_基础之HLSL(高级着色语言) HLSL着色器程序的编制 HSLS变量 HLSL入口函数 HLSL程序编译 变量常量类型 设置方法 前缀 关键字 类型 语句 类型转换
- 黑马程序员—Java语言基础(关键字、标识符、注释、常量与变量、进制、数据类型、类型转换、代码示例)
- 黑马程序员—【Java基础篇】之语言基础———关键字、标识符、注释、常量和变量及运算符
- Java语言基础(关键字、标识符、注释、常量、变量、运算符)
- Go语言学习笔记(二) [变量、类型、关键字]
- 黑马程序员——Java语言基础(一)---关键字、标识符、注释、常量、变量、运算符
- C语言中关键字、标识符、注释、变量、运算符
- 黑马程序员——零基础学习iOS开发——03 c语言基础语法:关键字、标示符、注释、数据结构、变量、变量内存分析、scanf函数