Python全栈(一)基础之5.条件控制语句
文章目录
今天是10.24,一年一度的程序员节,是属于我们最独特的节日,首先祝各位程序猿/媛节日快乐,也祝我节日快乐,希望用我们的代码敲出不一样的天地,加油???
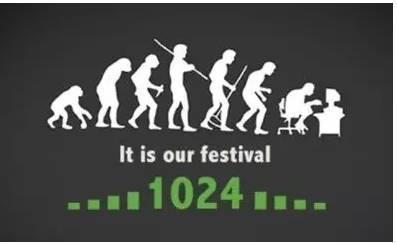
一、if语句
语法:
if 条件表达式: 代码块
代码块是保存一组代码,同一个代码块要么都执行,要么都不执行,代码块就是一种伪代码分组的机制。
执行流程:if语句在执行时,会先对条件表达式进行判断,
如果为True,则执行if后的语句,
如果为False,则不执行。
if True: print('hello')
number = 50 if number > 20: print('number is bigger than 20')
默认情况下if指挥控制紧跟其后的一条语句;
如果希望if可以控制多条语句,则可以在if后跟一个代码块。
if True: print('hello')print('你好')
※可以用逻辑运算符连接判断条件。
num = 30 if num > 20 and num < 40: print('num is bigger than 20 and less than 40')
二、input()函数
函数在执行时,程序会暂停、等待用户输入,直到用户输入,才会继续往下执行。
username = input('Please input your username:') if username == 'admin': print('Welcome')
先进行类型转换
salary = int(input('Please input your salary')) if salary <= 2000: print('You can be better')
三、if-else语句
语法:
if 条件表达式: 代码块 else: 代码块
执行流程:
if-else语句在执行时,先对if后的条件表达式进行求值判断,
如果为True,则执行if后的代码块,
如果为False,则执行else后的代码块。
salary = int(input('Please input your salary')) if salary <= 2000: print('You can be better')else: print('You are good')
四、if-elif-else语句
语法:
if 条件表达式: 代码块 elif 条件表达式: 代码块 elif 条件表达式: 代码块 elif 条件表达式: 代码块 else: 代码块
执行流程:
语句在执行时,会自上向下一次对条件表达式进行求值判断,
如果表达式的结果为True,则执行当前代码块,然后语句结束,
如果表达式的结果为False,则继续向下判断,直到找到True为止,
如果所有的表达式都是False,则执行else后的代码块。
语句中只会有一个代码块被执行。
salary = 30000 if salary >= 30000: print('Your salary is bigger than 30000') elif salary >= 20000: print('Your salary is bigger than 20000') elif salary >= 10000: print('Your salary is bigger than 10000') elif salary >= 5000: print('Your salary is bigger than 5000') else: print('You can be better')
没有else语句时,可能不会有执行结果。
salary = 2000 if salary >= 30000: print('Your salary is bigger than 30000') elif salary >= 20000: print('Your salary is bigger than 20000') elif salary >= 10000: print('Your salary is bigger than 10000') elif salary >= 5000: print('Your salary is bigger than 5000')
if-elif-else语句的表达式的数值一般采用从高到低写,而不能从低到高写。
salary = 30000 if salary >= 5000: print('Your salary is bigger than 5000') elif salary >= 10000: print('Your salary is bigger than 10000') elif salary >= 20000: print('Your salary is bigger than 20000') else: print('Your salary is bigger than 30000')
可能会总是打印“Your salary is bigger than 5000”,与我们要表达的意思不符,成为死代码。
判断月份属于哪个季节:
month = 3 if month > 12 or month < 1: print('not exists') elif month >=3 and month <= 5: print(month,'Spring') elif month >= 6 and month <= 8: print(month,'Summer') elif month >= 9 and month <= 11: print(month,'Autumn') else: print(month,'Winter')
五、if语句练习
1.判断奇偶数
num = int(input('Please input a number:')) if num % 2 == 0: print(num,'is an even') else: print(num,'is an odd')
2.检查年份是否为闰年
year = int(input('Please input a year:')) if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0: print('Leap year') else: print('Ordinary year')
3.狗与人的年龄对应
条件:狗前两年相当于人10.5岁,然后每增加1年就增加4岁
dog_age = float(input('Please input the age of dog:')) person_age = 0 if dog_age > 0: if dog_age <= 2: person_age = dog_age * 10.5 else: person_age = 10.5 * 2 person_age += (dog_age - 2) * 4 print('The dog of {} years old is equal to a person of {} years old'.format(dog_age,person_age)) else: print('Error')
※此段代码使用条件判断的嵌套。
六、while语句
循环:是指定的代码块重复执行指定的次数。
while循环:
语法:
while 条件表达式: 代码块 else: 代码块
执行流程:
语句在执行时,会对while后的条件表达式进行求值判断,
如果判断结果为True,则执行循环体(代码块),
循环体执行完毕,继续对条件表达式进行求值判断,以此类推,
直到判断结果为False,则循环中止。
while True: print('hello')
。
作为测试,执行之后,一直打印Hello,在几秒内打印了近20万行,如图所示。
此程序为无限循环,会一直打印,如不强行停止,会进入死循环,很消耗内存。
循环三要素:
初始化的表达式:通过初始化来定义变量,如
i = 0
条件表达式:用来循环执行的条件,如
while i < 20:
print(i)
更新表达式:修改初始化变量的值,如
i += 1
完整代码为:
i = 0 while i < 20: print(i) i += 1
且while语句可以和else语句结合使用。
i = 0 while i < 20: print(i) i += 1else: print('i is not less than 20')
七、while语句练习
1.求100(包括100)以内所有偶数之和
方法一:
i = 0 result = 0 while i < 100: i += 1 if i % 2 == 0: result += i print('The result is {}'.format(result))
方法二:
i = 0 result = 0 while i < 100: i += 2 result += i print('The result is {}'.format(result))
2.求100以内(包括100)所有9的倍数之和
方法一:
i = 0 result = 0 while (i+9) < 100: i += 9 result += i print('The result is {}'.format(result))
方法二:
i = 9 result = 0 while i < 100: result += i i += 9 print('The result is {}'.format(result))
- 点赞 4
- 收藏
- 分享
- 文章举报

- Python全栈(一)基础之6.条件控制语句练习
- Python基础之条件控制操作示例【if语句】
- python基础知识点数据类型转换、条件控制语句、循环语句、字符串、元组、字典、逻辑运算符
- python 基础知识第6讲:条件语句控制练习题集合
- Python基础6- 流程控制之if条件语句
- Python基础语法学习--条件控制与循环语句
- Python基础作业_第06章_条件控制和循环语句
- 9-Python3从入门到实战—基础之条件控制语句
- python 基础知识第5讲:条件控制语句
- 第一章:python基础语法| 字符编码| 条件语句...
- Python新手学习基础之条件语句——elif语句
- 学习笔记(03):Python数据分析与爬虫-Python中的流程控制语句:if条件判断
- python基础知识--条件,循环和其他语句
- Python基础教程(三):运算符、条件语句
- JS基础5-流程控制语句之条件(if、switch)
- Python基础,基本类型(整型,浮点数等)数据结构(List,dic(Map),Set,Tuple),控制语句(if,for,while,continue or break):来自学习资料
- python基础------if条件语句
- Python基础教程之第5章 条件, 循环和其他语句
- Python基础2:流程控制语句 while / for循环
- python基础之条件循环语句