codeforce gym 101726 problem D Poker
Poker is played with a standard 52-card deck (13 ranks and 4 suits). The ranks of the cards, in increasing order, are: 2, 3, ..., 10, Jack, Queen, King and Ace. Given a poker table, with two players, your task is to determine the winner.
Each player has two cards in his hand and there are five common cards to both players. Whichever player has the most valuable 5-card hand, choosing from his own cards and the one on the table, wins. A card on the table may be used by both players at the same time and a player may ignore zero, one or both of his own cards.
To determine the value of a 5-card hand, it is identified as one of the hand types listed below. If the same hand fits more than one type, the most valuable one is chosen. If both players' hands fit the same type, the tie is broken by some rule specific to that type.
The type list, from least to most valuable, and their respective tie-breaking rule are:
- Highest card: If the hand does not fit any of the other types. To break the tie, the five cards are compared, one by one, from highest to lowest rank, until one hand has a higher card then the other;
- One pair: Two cards of the same rank. The tie-breaker is similar the rule in Highest card, first comparing the pair, and then the other three cards;
- Two pairs: Two pairs of different rank. The tie-breaker is first by the most valuable pair, then by the second, then by the last card;
- Three of a kind: Three cards of the same rank. The tie-breaker is analogous to the one in One pair, first compare the triple, then the other two cards;
- Straight: Five cards in a sequence. In this case, the Ace may be either the highest rank (after the King) or the lowest (before the 2). The tie-breaker is done by the card of highest rank, considering that Ace has the lowest rank if it comes before a 2;
- Flush: Five cards of the same suit. The tie-breaker is done like in Highest card;
- Full House: A three of a kind with a pair. The tie-breaker is done by the rank of the three of a kind, and then by the pair;
- Four of a kind: Four cards of the same rank. The tie-breaker is done by the rank of the four of a kind, and then by the last card;
- Straight Flush: Straight and Flush at the same time. The tie-breaker is the same as in Straight.
Notice that it is possible for the tie to persist even after the tie-breaker rules are applied. The suits of a card are only used to define a Flush, and are not used in any tie-breaker rule.
On the first line, an integer T, the number of test cases.
Each test case is given in three lines. The first two lines have the description of two cards each, the cards of the first player, and of the second. The last line has the description of five cards, the cards on the table.
The description of each card is given by two characters, the rank and the suit, as given in the table.
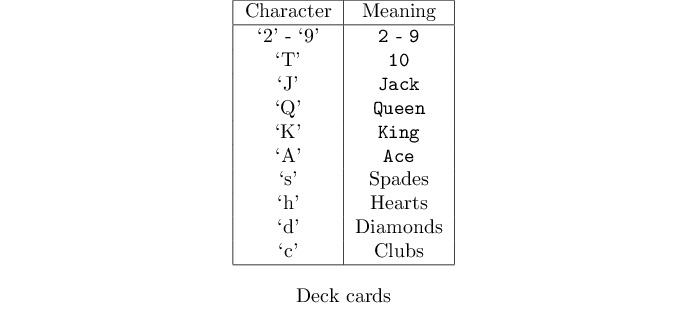
Limits
- 1 ≤ T ≤ 105
- For each test case, all cards are valid and distinct.
For each test, print 1 if the first player wins, 2 if the second player wins, and 0 if there is a tie, even after applying the tie-breaker rules.
Example input Copy3output Copy
Ts Js
Tc Jc
Qs Qc Ks Kc As
As 7d
Ah 8s
Ac Ad 9s Jh Kc
As 7d
Ah 8s
Ac Ad 6s 3h Kc
1
0
2
思路:按规则模拟即可。判断当前是什么类型优先判断是否满足等级高的类别。


1 #include <iostream> 2 #include <fstream> 3 #include <sstream> 4 #include <cstdlib> 5 #include <cstdio> 6 #include <cmath> 7 #include <string> 8 #include <cstring> 9 #include <algorithm> 10 #include <queue> 11 #include <stack> 12 #include <vector> 13 #include <set> 14 #include <map> 15 #include <list> 16 #include <iomanip> 17 #include <cctype> 18 #include <cassert> 19 #include <bitset> 20 #include <ctime> 21 22 using namespace std; 23 24 #define pau system("pause") 25 #define ll long long 26 #define pii pair<int, int> 27 #define pb push_back 28 #define mp make_pair 29 #define clr(a, x) memset(a, x, sizeof(a)) 30 31 const double pi = acos(-1.0); 32 const int INF = 0x3f3f3f3f; 33 const int MOD = 1e9 + 7; 34 const double EPS = 1e-9; 35 36 /* 37 #include <ext/pb_ds/assoc_container.hpp> 38 #include <ext/pb_ds/tree_policy.hpp> 39 40 using namespace __gnu_pbds; 41 tree<pli, null_type, greater<pli>, rb_tree_tag, tree_order_statistics_node_update> T; 42 */ 43 44 int t; 45 struct gg { 46 int v, co; 47 gg () {} 48 gg (int v, int co) : v(v), co(co) {} 49 void input() { 50 char c = getchar(); 51 while (!isalpha(c) && !isdigit(c)) c = getchar(); 52 if ('T' == c) { 53 v = 10; 54 } else if ('J' == c) { 55 v = 11; 56 } else if ('Q' == c) { 57 v = 12; 58 } else if ('K' == c) { 59 v = 13; 60 } else if ('A' == c) { 61 v = 14; 62 } else { 63 v = c - '0'; 64 } 65 co = getchar(); 66 } 67 bool operator < (const gg &g) const { 68 return v < g.v; 69 } 70 } g1[10], g2[10]; 71 struct hh { 72 int type; 73 gg g[5]; 74 void modify() { 75 sort(g, g + 5); 76 } 77 void output() { 78 printf("type = %d ", type); 79 for (int i = 0; i < 5; ++i) { 80 printf("%d %c ", g[i].v, g[i].co); 81 } 82 puts(""); 83 } 84 int is_flush() const { 85 for (int i = 1; i < 5; ++i) { 86 if (g[i].co != g[0].co) return 0; 87 } 88 return g[4].v; 89 } 90 int is_straight() const { 91 int f = 1; 92 for (int i = 3; ~i; --i) { 93 if (g[i].v != g[i + 1].v - 1) { 94 f = 0; 95 break; 96 } 97 } 98 if (f) return g[4].v; 99 f = 1; 100 if (14 == g[4].v) { 101 for (int i = 0; i < 4; ++i) { 102 if (g[i].v != i + 2) { 103 f = 0; 104 break; 105 } 106 } 107 } else { 108 f = 0; 109 } 110 return f ? 5 : 0; 111 } 112 int is_pair() const { 113 int i; 114 for (i = 4; i; --i) { 115 if (g[i].v == g[i - 1].v && (1 == i || g[i].v != g[i - 2].v) && (4 == i || g[i].v != g[i + 1].v)) { 116 break; 117 } 118 } 119 if (i) { 120 int res = g[i].v; 121 for (int j = 4; ~j; --j) { 122 if (i == j || i - 1 == j) continue; 123 res = res * 20 + g[j].v; 124 } 125 return res; 126 } 127 return 0; 128 } 129 int is_triple() const { 130 if (g[4].v == g[2].v) return g[4].v * 400 + g[1].v * 20 4000 + g[0].v; 131 if (g[3].v == g[1].v) return g[3].v * 400 + g[4].v * 20 + g[0].v; 132 if (g[2].v == g[0].v) return g[2].v * 400 + g[4].v * 20 + g[3].v; 133 return 0; 134 } 135 int is_fouple() const { 136 if (g[4].v == g[1].v) return g[4].v * 20 + g[0].v; 137 if (g[3].v == g[0].v) return g[3].v * 20 + g[4].v; 138 return 0; 139 } 140 int is_two_pair() const { 141 if (g[4].v == g[3].v && g[2].v == g[1].v) return g[4].v * 400 + g[2].v * 20 + g[0].v; 142 if (g[4].v == g[3].v && g[1].v == g[0].v) return g[4].v * 400 + g[1].v * 20 + g[2].v; 143 if (g[3].v == g[2].v && g[1].v == g[0].v) return g[3].v * 400 + g[1].v * 20 + g[4].v; 144 return 0; 145 } 146 pii is_house() const { 147 if (g[4].v == g[2].v && g[1].v == g[0].v) return pii(g[4].v, g[1].v); 148 if (g[4].v == g[3].v && g[2].v == g[0].v) return pii(g[2].v, g[4].v); 149 return pii(0, 0); 150 } 151 int get_type() const { 152 int ff = is_flush(); 153 int fs = is_straight(); 154 int fp = is_pair(); 155 int ft = is_triple(); 156 if (fs) { 157 return ff ? 9 : 5; 158 } else if (is_fouple()) { 159 return 8; 160 } else if (fp && ft) { 161 return 7; 162 } else if (ff) { 163 return 6; 164 } else if (ft) { 165 return 4; 166 } else if (is_two_pair()) { 167 return 3; 168 } else if (is_pair()) { 169 return 2; 170 } else { 171 return 1; 172 } 173 } 174 bool operator < (const hh h) const { 175 if (type != h.type) return type < h.type; 176 if (1 == type) { 177 for (int i = 4; ~i; --i) { 178 if (g[i].v != h.g[i].v) return g[i].v < h.g[i].v; 179 } 180 return false; 181 } else if (2 == type) { 182 return is_pair() < h.is_pair(); 183 } else if (3 == type) { 184 return is_two_pair() < h.is_two_pair(); 185 } else if (4 == type) { 186 return is_triple() < h.is_triple(); 187 } else if (5 == type) { 188 return is_straight() < h.is_straight(); 189 } else if (6 == type) { 190 for (int i = 4; ~i; --i) { 191 if (g[i].v != h.g[i].v) return g[i].v < h.g[i].v; 192 } 193 return false; 194 } else if (7 == type) { 195 return is_house() < h.is_house(); 196 } else if (8 == type) { 197 return is_fouple() < h.is_fouple(); 198 } else { 199 return is_straight() < h.is_straight(); 200 } 201 } 202 } h1, h2, th; 203 int main() { 204 scanf("%d", &t); 205 while (t--) { 206 g1[1].input(), g1[2].input(); 207 g2[1].input(), g2[2].input(); 208 for (int i = 1; i <= 5; ++i) { 209 g1[i + 2].input(); 210 g2[i + 2] = g1[i + 2]; 211 } 212 h1.type = h2.type = 0; 21 8000 3 for (int i = 1; i <= 7; ++i) { 214 for (int j = i + 1; j <= 7; ++j) { 215 for (int k = 1, l = 0; k <= 7; ++k) { 216 if (k == i || k == j) continue; 217 th.g[l++] = g1[k]; 218 } 219 th.modify(); 220 th.type = th.get_type(); 221 if (h1 < th) { 222 h1.type = th.type; 223 for (int i = 0; i < 5; ++i) { 224 h1.g[i] = th.g[i]; 225 } 226 } 227 } 228 } 229 for (int i = 1; i <= 7; ++i) { 230 for (int j = i + 1; j <= 7; ++j) { 231 for (int k = 1, l = 0; k <= 7; ++k) { 232 if (k == i || k == j) continue; 233 th.g[l++] = g2[k]; 234 } 235 th.modify(); 236 th.type = th.get_type(); 237 if (h2 < th) { 238 h2.type = th.type; 239 for (int i = 0; i < 5; ++i) { 240 h2.g[i] = th.g[i]; 241 } 242 } 243 } 244 } 245 //h1.output(), h2.output(); 246 if (h1 < h2) puts("2"); 247 else if (h2 < h1) puts("1"); 248 else puts("0"); 249 } 250 return 0; 251 } 252 /* 253 111 254 9h 8h 255 Jc 9d 256 9s 4d Ts Ad As 257 */View Code
转载于:https://www.cnblogs.com/BIGTOM/p/8969352.html
- codeforce gym 101726 problem C Ekaterinburg Pyramids
- codeforce gym 101726 problem B. Spy Duel
- codeforce gym 101726 problem A. Popularity on Facebook
- codeforce Gym 100500F Door Lock (二分)
- codeforce Gym 100500A Poetry Challenge(博弈,暴搜)
- 【codeforce Gym 100570B】【最短路SPFA】 ShortestPath Query 【询问单源最短路径,每条边有一个颜色,要求路径上相邻边的颜色不能相同】
- codeforce Gym 100500I Hall of Fame (水)
- codeforce Gym 100500H ICPC Quest (简单dp)
- codeforce Gym 100342J Triatrip (bitset)
- codeforce Gym 100203I I WIN (网络流)
- Codeforces Gym 100610 Problem E. Explicit Formula 水题
- Codeforces Gym 100623D Problem D. Deposits
- 【打渔】CodeForce Round 197 Div.2 Problem_A
- codeforce Gym 100342H Hard Test (思考题)
- Codeforces Gym 100610 Problem H. Horrible Truth 瞎搞
- Codeforces GYM 100960B: ForceField 题解
- codeforce Gym 100425E The Street Escalator(期望,线性递推)
- codeforce Gym 100570B ShortestPath Query (最短路SPFA)
- codeforce gym 100548H The Problem to Make You Happy
- Codeforces Gym 100610 Problem K. Kitchen Robot 状压DP