(八)上传和下载模块(单文件和多文件上传)
2018-03-28 18:35
218 查看
既然上面说到了邮件上传附件,那么干脆具体的写一下上传文件这个控件吧。
通常情况下,上传文件是指将本地文件上传到远程的服务器中,在asp.net中,上传文件通常由fileupload控件来实现。
首先改我们的配置文件,让fileupload上传大文件不会报错。加入
<system.webServer>
<security>
<requestFiltering >
<requestLimits maxAllowedContentLength="2147483647" ></requestLimits>
</requestFiltering><!--单位字节-->
</security>
</system.webServer>
修改 <httpRuntime maxRequestLength="2097152" executionTimeout="600" /><!--单位kb,默认值大小为4096,最大2097152,可以根据需求改, 指定上传文件的有效时间为10分钟-->
上传单个文件onefileupload.aspx.cs
namespace fileupload
{
public partial class oneuploading : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
try
{
if (FileUpload1.PostedFile.ContentLength <= 0)
{
Response.Write("上传的文件为空文件,请重新上传");
}
else
{
if (FileUpload1.HasFile)
{
if (FileUpload1.PostedFile.ContentLength < 2147483647)//单位字节,2g
{
Boolean fileOk = false;
String fileException = Path.GetExtension(FileUpload1.FileName).ToLower();//获取文件的扩展名
String[] allowException = { ".xls", ".doc", ".docx", ".xlsx ", ".mpp", ".rar", ".zip", ".vsd", ".txt", ".jpg", ".gif", ".bmp", ".png", ".bak", ".swf", ".avi", ".mp3", ".rm", ".wma", ".wmv", ".pdf" };//上传文件格式
for (int i = 0; i < allowException.Length; i++)
{
if (fileException == allowException[i])
fileOk = true;
}
if (fileOk)
{
string FilePath = Server.MapPath("~/file/");
if (!Directory.Exists(FilePath))
{
Directory.CreateDirectory(FilePath);
}
FileUpload1.SaveAs(FilePath + "\\" + FileUpload1.FileName);
Response.Write("上传成功!");
}
else
{
Response.Write("不支持此格式的文件上传!");
}
}
else
Response.Write("上传的文件过大!");
}
else
{
Response.Write("没有文件可以上传!");
}
}
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
}
}
}
对于多个文件上传,这里没有采用asp.net提供的fileupload控件,我采用了html的input(file),在增加控件的时候,由于asp.net的控件是有id的,不可以重复增加一个一样id的控件,修改每个控件的id比较难,所以选择html提供的。增加,删除,重置也一起采用html提供的input(button),用来写js。
下面是增加,删除和重置的js代码
<script type="text/javascript">
function delall() {
var div = document.getElementById("FileCollection");
while (div.hasChildNodes()) //当div下还存在子节点时 循环继续
{
div.removeChild(div.firstChild);
}
}
function addfilecontrol() {
var str = '<div><input id="File" type="file" runat="server"/><input id="Button3" type="button" value="删除" onclick="del()"/></div>';
document.getElementById("FileCollection").insertAdjacentHTML("beforeEnd", str);//在 element 元素的最后一个子元素后面。
}
function del() {
var parent = document.getElementById("FileCollection");
var divs = parent.getElementsByTagName("div");
var childs = parent.childNodes;
for (var i = 0; i < divs.length; i++) {
divs[i].setAttribute("index", i + 1);//获取div的索引
divs[i].onclick = function () {
parent.removeChild(childs[this.getAttribute("index")]);
4000
}
}
}
</script>
moreuploading.aspx
<body>
<form id="form1" runat="server">
<div><input id="File1" type="file" runat="server"/></div>
<div id="FileCollection"> </div>
<input id="Button1" type="button" value="增加(file)" onclick="addfilecontrol()" /> <asp:Button ID="Upload" runat="server" Text="上传" OnClick="Upload_Click" />
<input id="Button2" type="button" value="重置" onclick="delall()"/></form>
</body>
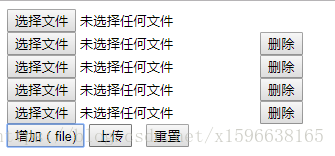
注意一点很重要的,<input id="File1" type="file" runat="server"/>必须要写runat="server",不然后台是遍历不到的。
下面是moreuploading.aspx.cs代码
namespace fileupload
{
public partial class moreuploading : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Upload_Click(object sender, EventArgs e)
{
HttpFileCollection files = HttpContext.Current.Request.Files;
try
{
for(int fileCount=0;fileCount<files.Count;fileCount++)
{
HttpPostedFile postedFile = files[fileCount];
string fileName = Path.GetFileName(postedFile.FileName);
if (postedFile.ContentLength <= 0){
int j= Convert.ToInt32(fileCount) + 1;
Response.Write("第" +j +"为空文件,此文件上传失败<br>");
}
else
{
if (fileName != String.Empty)
{
if (postedFile.ContentLength < 2147483647)
{
Boolean fileOk = false;
String fileException = Path.GetExtension(fileName).ToLower();
String[] allowException = { ".xls", ".doc", ".docx", ".xlsx ", ".mpp", ".rar", ".zip", ".vsd", ".txt", ".jpg", ".gif", ".bmp", ".png", ".bak", ".swf", ".avi", ".mp3", ".rm", ".wma", ".wmv", ".pdf", ".xml" };//上传文件格式
for (int i = 0; i < allowException.Length; i++)
{
if (fileException == allowException[i])
fileOk = true;
}
if (fileOk)
{
string FilePath = Server.MapPath("~/morefile/");
if (!Directory.Exists(FilePath))
{
Directory.CreateDirectory(FilePath);
}
postedFile.SaveAs(FilePath + "\\" + fileName);
Response.Write(fileName + "上传成功!<br>");
}
else
Response.Write(fileName + "扩展名为" + fileException + "不支持此格式的文件上传,此文件上传失败<br>");
}
else
Response.Write("第" + fileCount + 1 + "个上传的文件过大!上传失败<br>");
}
else
Response.Write("第" + fileCount + 1 + "个控件中没有可上传文件,上传失败<br>");
}
}
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
}
}
}
如果上传的文件有一个是上传失败的,那么只有它需要重新上传,别的文件没有出错的,会照常上传成功。
https://github.com/1126048156/upanddownfile.git
通常情况下,上传文件是指将本地文件上传到远程的服务器中,在asp.net中,上传文件通常由fileupload控件来实现。
首先改我们的配置文件,让fileupload上传大文件不会报错。加入
<system.webServer>
<security>
<requestFiltering >
<requestLimits maxAllowedContentLength="2147483647" ></requestLimits>
</requestFiltering><!--单位字节-->
</security>
</system.webServer>
修改 <httpRuntime maxRequestLength="2097152" executionTimeout="600" /><!--单位kb,默认值大小为4096,最大2097152,可以根据需求改, 指定上传文件的有效时间为10分钟-->
上传单个文件onefileupload.aspx.cs
namespace fileupload
{
public partial class oneuploading : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
try
{
if (FileUpload1.PostedFile.ContentLength <= 0)
{
Response.Write("上传的文件为空文件,请重新上传");
}
else
{
if (FileUpload1.HasFile)
{
if (FileUpload1.PostedFile.ContentLength < 2147483647)//单位字节,2g
{
Boolean fileOk = false;
String fileException = Path.GetExtension(FileUpload1.FileName).ToLower();//获取文件的扩展名
String[] allowException = { ".xls", ".doc", ".docx", ".xlsx ", ".mpp", ".rar", ".zip", ".vsd", ".txt", ".jpg", ".gif", ".bmp", ".png", ".bak", ".swf", ".avi", ".mp3", ".rm", ".wma", ".wmv", ".pdf" };//上传文件格式
for (int i = 0; i < allowException.Length; i++)
{
if (fileException == allowException[i])
fileOk = true;
}
if (fileOk)
{
string FilePath = Server.MapPath("~/file/");
if (!Directory.Exists(FilePath))
{
Directory.CreateDirectory(FilePath);
}
FileUpload1.SaveAs(FilePath + "\\" + FileUpload1.FileName);
Response.Write("上传成功!");
}
else
{
Response.Write("不支持此格式的文件上传!");
}
}
else
Response.Write("上传的文件过大!");
}
else
{
Response.Write("没有文件可以上传!");
}
}
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
}
}
}
对于多个文件上传,这里没有采用asp.net提供的fileupload控件,我采用了html的input(file),在增加控件的时候,由于asp.net的控件是有id的,不可以重复增加一个一样id的控件,修改每个控件的id比较难,所以选择html提供的。增加,删除,重置也一起采用html提供的input(button),用来写js。
下面是增加,删除和重置的js代码
<script type="text/javascript">
function delall() {
var div = document.getElementById("FileCollection");
while (div.hasChildNodes()) //当div下还存在子节点时 循环继续
{
div.removeChild(div.firstChild);
}
}
function addfilecontrol() {
var str = '<div><input id="File" type="file" runat="server"/><input id="Button3" type="button" value="删除" onclick="del()"/></div>';
document.getElementById("FileCollection").insertAdjacentHTML("beforeEnd", str);//在 element 元素的最后一个子元素后面。
}
function del() {
var parent = document.getElementById("FileCollection");
var divs = parent.getElementsByTagName("div");
var childs = parent.childNodes;
for (var i = 0; i < divs.length; i++) {
divs[i].setAttribute("index", i + 1);//获取div的索引
divs[i].onclick = function () {
parent.removeChild(childs[this.getAttribute("index")]);
4000
}
}
}
</script>
moreuploading.aspx
<body>
<form id="form1" runat="server">
<div><input id="File1" type="file" runat="server"/></div>
<div id="FileCollection"> </div>
<input id="Button1" type="button" value="增加(file)" onclick="addfilecontrol()" /> <asp:Button ID="Upload" runat="server" Text="上传" OnClick="Upload_Click" />
<input id="Button2" type="button" value="重置" onclick="delall()"/></form>
</body>
注意一点很重要的,<input id="File1" type="file" runat="server"/>必须要写runat="server",不然后台是遍历不到的。
下面是moreuploading.aspx.cs代码
namespace fileupload
{
public partial class moreuploading : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Upload_Click(object sender, EventArgs e)
{
HttpFileCollection files = HttpContext.Current.Request.Files;
try
{
for(int fileCount=0;fileCount<files.Count;fileCount++)
{
HttpPostedFile postedFile = files[fileCount];
string fileName = Path.GetFileName(postedFile.FileName);
if (postedFile.ContentLength <= 0){
int j= Convert.ToInt32(fileCount) + 1;
Response.Write("第" +j +"为空文件,此文件上传失败<br>");
}
else
{
if (fileName != String.Empty)
{
if (postedFile.ContentLength < 2147483647)
{
Boolean fileOk = false;
String fileException = Path.GetExtension(fileName).ToLower();
String[] allowException = { ".xls", ".doc", ".docx", ".xlsx ", ".mpp", ".rar", ".zip", ".vsd", ".txt", ".jpg", ".gif", ".bmp", ".png", ".bak", ".swf", ".avi", ".mp3", ".rm", ".wma", ".wmv", ".pdf", ".xml" };//上传文件格式
for (int i = 0; i < allowException.Length; i++)
{
if (fileException == allowException[i])
fileOk = true;
}
if (fileOk)
{
string FilePath = Server.MapPath("~/morefile/");
if (!Directory.Exists(FilePath))
{
Directory.CreateDirectory(FilePath);
}
postedFile.SaveAs(FilePath + "\\" + fileName);
Response.Write(fileName + "上传成功!<br>");
}
else
Response.Write(fileName + "扩展名为" + fileException + "不支持此格式的文件上传,此文件上传失败<br>");
}
else
Response.Write("第" + fileCount + 1 + "个上传的文件过大!上传失败<br>");
}
else
Response.Write("第" + fileCount + 1 + "个控件中没有可上传文件,上传失败<br>");
}
}
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
}
}
}
如果上传的文件有一个是上传失败的,那么只有它需要重新上传,别的文件没有出错的,会照常上传成功。
https://github.com/1126048156/upanddownfile.git
相关文章推荐
- 文件一键上传、汉字转拼音、excel文件上传下载功能模块的实现
- 实用模块:Asp.net实现的树形管理界面(支持zip文件上传和zip文件下载)
- 文件一键上传、汉字转拼音、excel文件上传下载功能模块的实现
- 文件一键上传、汉字转拼音、excel文件上传下载功能模块的实现
- python模块学习之paramiko远程执行命令,文件上传、下载
- python模块学习之paramiko远程执行命令,文件上传、下载
- 文件一键上传、汉字转拼音、excel文件上传下载功能模块的实现
- 使用paramiko模块远程登录并上传或下载文件
- Python paramiko模块 实现 ssh远程执行命令 上传下载文件 堡垒机模式下的远程命令执行
- 使用paramiko模块远程执行命令、上传文件和下载文件
- (九)上传和下载模块(下载文件)
- python paramiko模块实现sftp上传下载文件
- 如何使用 paramiko 模块来实现远程执行OS命令和文件上传下载?
- 利用python fabric模块写的批量操作远程主机脚本(命令执行,上传、下载文件)
- Java文件上传下载
- struts2中文件上传与下载
- 【Python】Python的urllib模块、urllib2模块进行网页下载文件
- ①Struts2之实现文件上传与下载
- 利用SecureCRT上传、下载文件(使用sz与rz命令)
- 文件上传和下载