Shiro入门
2018-03-15 15:15
134 查看
1、Shiro定义
Apache Shiro是一个强大灵活的开源安全框架,可以完全处理身份验证、授权、企业会话管理和加密。
2、Shiro目标是什么
Shiro开发团队称为“应用程序安全的四个基石即:认证,授权,会话管理和加密:
(1)认证:有时被称为登录,这是证明用户的行为(他们说自己是谁)。
(2)授权:访问控制的过程,即确定谁可以访问什么。
(3)会话管理:管理用户特定的会话,即使在非Web或EJB应用程序中。
(4)加密:使用加密算法保持数据安全,同时仍然易于使用。
3、主要概念
Subject:本质上是当前正在执行的用户的安全特定视图。
SecurityManager:Shiro的体系结构的核心,并且充当一种“伞”对象,协调其内部安全组件,它们一起形成对象图。但是,一旦为应用程序配置了SecurityManager及其内部对象图表,它通常会被放在一边,应用程序开发人员几乎将所有时间用在SubjectAPI上。
Realm:领域充当Shiro和应用程序安全数据之间的桥梁或连接器。当需要实际与用户帐户等安全相关数据进行交互以执行身份验证(登录)和授权(访问控制)时,Shiro将从为应用程序配置的一个或多个领域查找许多这些内容。
4、简单实例
(1)项目结构
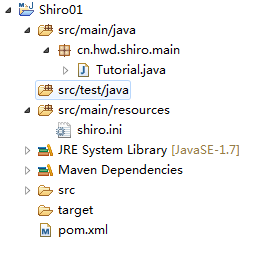
(2)pom.xml<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>cn.hwd</groupId>
<artifactId>Shiro01</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>Shiro01</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-core</artifactId>
<version>1.1.0</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.6.1</version>
</dependency>
</dependencies>
</project>(3)shiro.ini# =============================================================================
# Tutorial INI configuration
#
# Usernames/passwords are based on the classic Mel Brooks' film "Spaceballs" :)
# =============================================================================
# -----------------------------------------------------------------------------
# Users and their (optional) assigned roles
# username = password, role1, role2, ..., roleN
# -----------------------------------------------------------------------------
[users]
root = secret, admin
guest = guest, guest
presidentskroob = 12345, president
darkhelmet = ludicrousspeed, darklord, schwartz
lonestarr = vespa, goodguy, schwartz
# -----------------------------------------------------------------------------
# Roles with assigned permissions
# roleName = perm1, perm2, ..., permN
# -----------------------------------------------------------------------------
[roles]
admin = *
schwartz = lightsaber:*
goodguy = winnebago:drive:eagle5其中配置文件的编码使用ANSI,否则会报错。
(4)Tutorial.javapackage cn.hwd.shiro.main;
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.authc.AuthenticationException;
import org.apache.shiro.authc.IncorrectCredentialsException;
import org.apache.shiro.authc.LockedAccountException;
import org.apache.shiro.authc.UnknownAccountException;
import org.apache.shiro.authc.UsernamePasswordToken;
import org.apache.shiro.config.IniSecurityManagerFactory;
import org.apache.shiro.mgt.SecurityManager;
import org.apache.shiro.session.Session;
import org.apache.shiro.subject.Subject;
import org.apache.shiro.util.Factory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Tutorial {
private static final transient Logger log = LoggerFactory.getLogger(Tutorial.class);
public static void main(String[] args) {
log.info("My First Apache Shiro Application");
Factory<SecurityManager> factory = new IniSecurityManagerFactory("classpath:shiro.ini");
SecurityManager securityManager = factory.getInstance();
SecurityUtils.setSecurityManager(securityManager);
// get the currently executing user:
Subject currentUser = SecurityUtils.getSubject();
// Do some stuff with a Session (no need for a web or EJB container!!!)
Session session = currentUser.getSession();
session.setAttribute("someKey", "aValue");
String value = (String) session.getAttribute("someKey");
if (value.equals("aValue")) {
log.info("Retrieved the correct value! [" + value + "]");
}
// let's login the current user so we can check against roles and permissions:
if (!currentUser.isAuthenticated()) {
UsernamePasswordToken token = new UsernamePasswordToken("lonestarr", "vespa");
token.setRememberMe(true);
try {
currentUser.login(token);
} catch (UnknownAccountException uae) {
log.info("There is no user with username of " + token.getPrincipal());
} catch (IncorrectCredentialsException ice) {
log.info("Password for account " + token.getPrincipal() + " was incorrect!");
} catch (LockedAccountException lae) {
log.info("The account for username " + token.getPrincipal() + " is locked. " +
"Please contact your administrator to unlock it.");
}
// ... catch more exceptions here (maybe custom ones specific to your application?
catch (AuthenticationException ae) {
//unexpected condition? error?
}
}
//say who they are:
//print their identifying principal (in this case, a username):
log.info("User [" + currentUser.getPrincipal() + "] logged in successfully.");
//test a role:
if (currentUser.hasRole("schwartz")) {
log.info("May the Schwartz be with you!");
} else {
log.info("Hello, mere mortal.");
}
//test a typed permission (not instance-level)
if (currentUser.isPermitted("lightsaber:weild")) {
log.info("You may use a lightsaber ring. Use it wisely.");
} else {
log.info("Sorry, lightsaber rings are for schwartz masters only.");
}
//a (very powerful) Instance Level permission:
if (currentUser.isPermitted("winnebago:drive:eagle5")) {
log.info("You are permitted to 'drive' the winnebago with license plate (id) 'eagle5'. " +
"Here are the keys - have fun!");
} else {
log.info("Sorry, you aren't allowed to drive the 'eagle5' winnebago!");
}
//all done - log out!
currentUser.logout();
System.exit(0);
}
}(5)运行结果0 [main] INFO cn.hwd.shiro.main.Tutorial - My First Apache Shiro Application
100 [main] INFO org.apache.shiro.session.mgt.AbstractValidatingSessionManager - Enabling session validation scheduler...
320 [main] INFO cn.hwd.shiro.main.Tutorial - Retrieved the correct value! [aValue]
320 [main] INFO cn.hwd.shiro.main.Tutorial - User [lonestarr] logged in successfully.
320 [main] INFO cn.hwd.shiro.main.Tutorial - May the Schwartz be with you!
320 [main] INFO cn.hwd.shiro.main.Tutorial - You may use a lightsaber ring. Use it wisely.
320 [main] INFO cn.hwd.shiro.main.Tutorial - You are permitted to 'drive' the winnebago with license plate (id) 'eagle5'. Here are the keys - have fun!
Apache Shiro是一个强大灵活的开源安全框架,可以完全处理身份验证、授权、企业会话管理和加密。
2、Shiro目标是什么
Shiro开发团队称为“应用程序安全的四个基石即:认证,授权,会话管理和加密:
(1)认证:有时被称为登录,这是证明用户的行为(他们说自己是谁)。
(2)授权:访问控制的过程,即确定谁可以访问什么。
(3)会话管理:管理用户特定的会话,即使在非Web或EJB应用程序中。
(4)加密:使用加密算法保持数据安全,同时仍然易于使用。
3、主要概念
Subject:本质上是当前正在执行的用户的安全特定视图。
SecurityManager:Shiro的体系结构的核心,并且充当一种“伞”对象,协调其内部安全组件,它们一起形成对象图。但是,一旦为应用程序配置了SecurityManager及其内部对象图表,它通常会被放在一边,应用程序开发人员几乎将所有时间用在SubjectAPI上。
Realm:领域充当Shiro和应用程序安全数据之间的桥梁或连接器。当需要实际与用户帐户等安全相关数据进行交互以执行身份验证(登录)和授权(访问控制)时,Shiro将从为应用程序配置的一个或多个领域查找许多这些内容。
4、简单实例
(1)项目结构
(2)pom.xml<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion>
<groupId>cn.hwd</groupId>
<artifactId>Shiro01</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>Shiro01</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-core</artifactId>
<version>1.1.0</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.6.1</version>
</dependency>
</dependencies>
</project>(3)shiro.ini# =============================================================================
# Tutorial INI configuration
#
# Usernames/passwords are based on the classic Mel Brooks' film "Spaceballs" :)
# =============================================================================
# -----------------------------------------------------------------------------
# Users and their (optional) assigned roles
# username = password, role1, role2, ..., roleN
# -----------------------------------------------------------------------------
[users]
root = secret, admin
guest = guest, guest
presidentskroob = 12345, president
darkhelmet = ludicrousspeed, darklord, schwartz
lonestarr = vespa, goodguy, schwartz
# -----------------------------------------------------------------------------
# Roles with assigned permissions
# roleName = perm1, perm2, ..., permN
# -----------------------------------------------------------------------------
[roles]
admin = *
schwartz = lightsaber:*
goodguy = winnebago:drive:eagle5其中配置文件的编码使用ANSI,否则会报错。
(4)Tutorial.javapackage cn.hwd.shiro.main;
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.authc.AuthenticationException;
import org.apache.shiro.authc.IncorrectCredentialsException;
import org.apache.shiro.authc.LockedAccountException;
import org.apache.shiro.authc.UnknownAccountException;
import org.apache.shiro.authc.UsernamePasswordToken;
import org.apache.shiro.config.IniSecurityManagerFactory;
import org.apache.shiro.mgt.SecurityManager;
import org.apache.shiro.session.Session;
import org.apache.shiro.subject.Subject;
import org.apache.shiro.util.Factory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class Tutorial {
private static final transient Logger log = LoggerFactory.getLogger(Tutorial.class);
public static void main(String[] args) {
log.info("My First Apache Shiro Application");
Factory<SecurityManager> factory = new IniSecurityManagerFactory("classpath:shiro.ini");
SecurityManager securityManager = factory.getInstance();
SecurityUtils.setSecurityManager(securityManager);
// get the currently executing user:
Subject currentUser = SecurityUtils.getSubject();
// Do some stuff with a Session (no need for a web or EJB container!!!)
Session session = currentUser.getSession();
session.setAttribute("someKey", "aValue");
String value = (String) session.getAttribute("someKey");
if (value.equals("aValue")) {
log.info("Retrieved the correct value! [" + value + "]");
}
// let's login the current user so we can check against roles and permissions:
if (!currentUser.isAuthenticated()) {
UsernamePasswordToken token = new UsernamePasswordToken("lonestarr", "vespa");
token.setRememberMe(true);
try {
currentUser.login(token);
} catch (UnknownAccountException uae) {
log.info("There is no user with username of " + token.getPrincipal());
} catch (IncorrectCredentialsException ice) {
log.info("Password for account " + token.getPrincipal() + " was incorrect!");
} catch (LockedAccountException lae) {
log.info("The account for username " + token.getPrincipal() + " is locked. " +
"Please contact your administrator to unlock it.");
}
// ... catch more exceptions here (maybe custom ones specific to your application?
catch (AuthenticationException ae) {
//unexpected condition? error?
}
}
//say who they are:
//print their identifying principal (in this case, a username):
log.info("User [" + currentUser.getPrincipal() + "] logged in successfully.");
//test a role:
if (currentUser.hasRole("schwartz")) {
log.info("May the Schwartz be with you!");
} else {
log.info("Hello, mere mortal.");
}
//test a typed permission (not instance-level)
if (currentUser.isPermitted("lightsaber:weild")) {
log.info("You may use a lightsaber ring. Use it wisely.");
} else {
log.info("Sorry, lightsaber rings are for schwartz masters only.");
}
//a (very powerful) Instance Level permission:
if (currentUser.isPermitted("winnebago:drive:eagle5")) {
log.info("You are permitted to 'drive' the winnebago with license plate (id) 'eagle5'. " +
"Here are the keys - have fun!");
} else {
log.info("Sorry, you aren't allowed to drive the 'eagle5' winnebago!");
}
//all done - log out!
currentUser.logout();
System.exit(0);
}
}(5)运行结果0 [main] INFO cn.hwd.shiro.main.Tutorial - My First Apache Shiro Application
100 [main] INFO org.apache.shiro.session.mgt.AbstractValidatingSessionManager - Enabling session validation scheduler...
320 [main] INFO cn.hwd.shiro.main.Tutorial - Retrieved the correct value! [aValue]
320 [main] INFO cn.hwd.shiro.main.Tutorial - User [lonestarr] logged in successfully.
320 [main] INFO cn.hwd.shiro.main.Tutorial - May the Schwartz be with you!
320 [main] INFO cn.hwd.shiro.main.Tutorial - You may use a lightsaber ring. Use it wisely.
320 [main] INFO cn.hwd.shiro.main.Tutorial - You are permitted to 'drive' the winnebago with license plate (id) 'eagle5'. Here are the keys - have fun!
相关文章推荐
- Apache Shiro系列三:10分钟入门
- Shiro入门实例
- Apache Shiro入门实例
- shiro入门
- Shiro安全框架入门篇(登录验证实例详解与源码)
- Shiro安全框架入门篇(登录验证实例详解与源码)
- BOS项目练习(权限概述,权限数据模型,shiro入门{bos中应用})
- 使用Shiro入门
- 18.03.09,web学习第七十天,bos第十天,shiro框架入门
- Shiro入门学习三
- shiro 权限控制框架 入门级实例(一)加密、登录验证
- 32、shiro框架入门3.授权
- Shiro入门这篇就够了【Shiro的基础知识、回顾URL拦截】
- Shiro快速入门
- shiro安全框架HelloWord入门篇
- Shiro入门-session管理
- shiro入门
- Shiro入门(1)
- Shiro入门案例
- Apache Shiro 快速入门实例