Android 商品属性SKU选择
2018-03-14 16:11
405 查看
效果图:
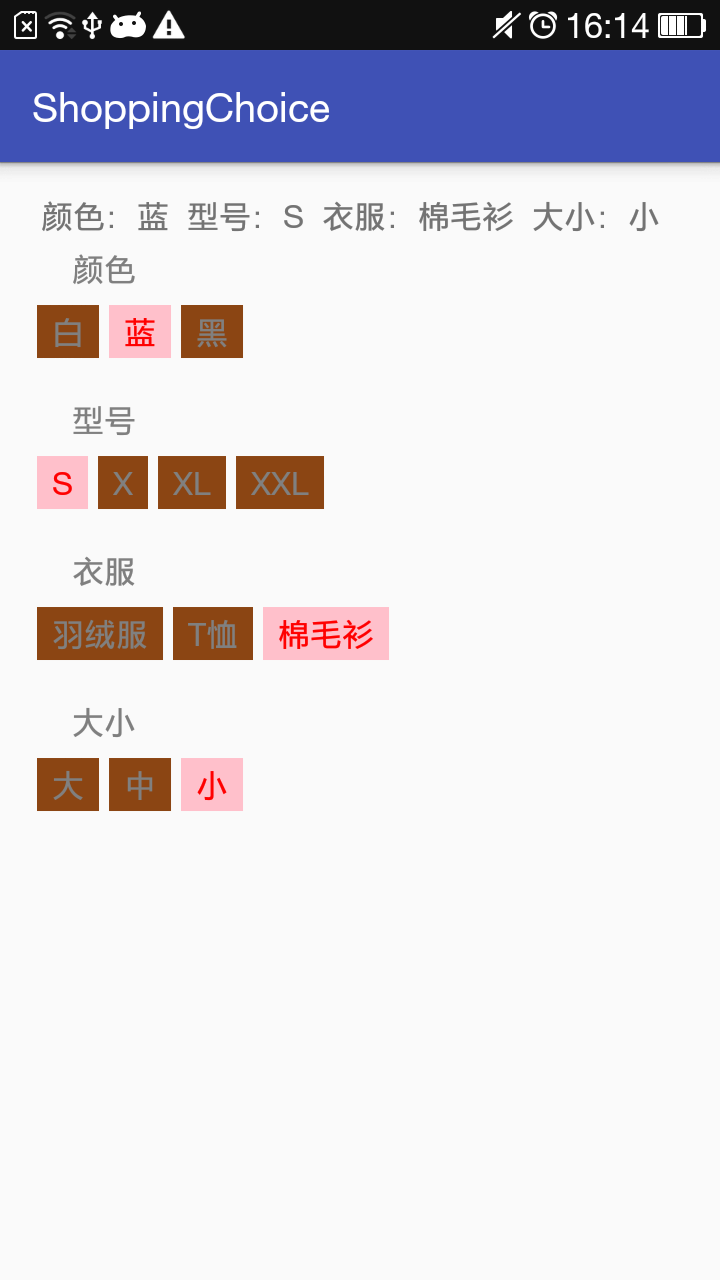
1.继承ViewGrouppublic class SKUViewGroup extends ViewGroup {
public SKUViewGroup(Context context, AttributeSet attrs)
{
super(context, attrs);
}
@Override
protected LayoutParams generateLayoutParams(
LayoutParams p)
{
return new MarginLayoutParams(p);
}
@Override
public LayoutParams generateLayoutParams(AttributeSet attrs)
{
return new MarginLayoutParams(getContext(), attrs);
}
@Override
protected LayoutParams generateDefaultLayoutParams()
{
return new MarginLayoutParams(LayoutParams.MATCH_PARENT,
LayoutParams.MATCH_PARENT);
}
/**
* 负责设置子控件的测量模式和大小 根据所有子控件设置自己的宽和高
*/
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec)
{
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
// 获得它的父容器为它设置的测量模式和大小
int sizeWidth = MeasureSpec.getSize(widthMeasureSpec);
int sizeHeight = MeasureSpec.getSize(heightMeasureSpec);
int modeWidth = MeasureSpec.getMode(widthMeasureSpec);
int modeHeight = MeasureSpec.getMode(heightMeasureSpec);
// 如果是warp_content情况下,记录宽和高
int width = 0;
int height = 0;
/**
* 记录每一行的宽度,width不断取最大宽度
*/
int lineWidth = 0;
/**
* 每一行的高度,累加至height
*/
int lineHeight = 0;
int cCount = getChildCount();
// 遍历每个子元素
for (int i = 0; i < cCount; i++)
{
View child = getChildAt(i);
// 测量每一个child的宽和高
measureChild(child, widthMeasureSpec, heightMeasureSpec);
// 得到child的布局管理器
MarginLayoutParams lp = (MarginLayoutParams) child
.getLayoutParams();
// 当前子空间实际占据的宽度
int childWidth = child.getMeasuredWidth() + lp.leftMargin
+ lp.rightMargin;
// 当前子空间实际占据的高度
int childHeight = child.getMeasuredHeight() + lp.topMargin
+ lp.bottomMargin;
/**
* 如果加入当前child,则超出最大宽度,则的到目前最大宽度给width,类加height 然后开启新行
*/
if (lineWidth + childWidth > sizeWidth)
{
width = Math.max(lineWidth, childWidth);// 取最大的
lineWidth = childWidth; // 重新开启新行,开始记录
// 叠加当前高度,
height += lineHeight;
// 开启记录下一行的高度
lineHeight = childHeight;
} else
// 否则累加值lineWidth,lineHeight取最大高度
{
lineWidth += childWidth;
lineHeight = Math.max(lineHeight, childHeight);
}
// 如果是最后一个,则将当前记录的最大宽度和当前lineWidth做比较
if (i == cCount - 1)
{
width = Math.max(width, lineWidth);
height += lineHeight;
}
}
setMeasuredDimension((modeWidth == MeasureSpec.EXACTLY) ? sizeWidth
: width, (modeHeight == MeasureSpec.EXACTLY) ? sizeHeight
: height);
}
/**
* 存储所有的View,按行记录
*/
private List<List<View>> mAllViews = new ArrayList<>();
/**
* 记录每一行的最大高度
*/
private List<Integer> mLineHeight = new ArrayList<>();
@Override
protected void onLayout(boolean changed, int l, int t, int r, int b)
{
mAllViews.clear();
mLineHeight.clear();
int width = getWidth();
int lineWidth = 0;
int lineHeight = 0;
// 存储每一行所有的childView
List<View> lineViews = new ArrayList<>();
int cCount = getChildCount();
// 遍历所有的孩子
for (int i = 0; i < cCount; i++)
{
View child = getChildAt(i);
MarginLayoutParams lp = (MarginLayoutParams) child
.getLayoutParams();
int childWidth = child.getMeasuredWidth();
int childHeight = child.getMeasuredHeight();
// 如果已经需要换行
if (childWidth + lp.leftMargin + lp.rightMargin + lineWidth > width)
{
// 记录这一行所有的View以及最大高度
mLineHeight.add(lineHeight);
// 将当前行的childView保存,然后开启新的ArrayList保存下一行的childView
mAllViews.add(lineViews);
lineWidth = 0;// 重置行宽
lineViews = new ArrayList<>();
}
/**
* 如果不需要换行,则累加
*/
lineWidth += childWidth + lp.leftMargin + lp.rightMargin;
lineHeight = Math.max(lineHeight, childHeight + lp.topMargin
+ lp.bottomMargin);
lineViews.add(child);
}
// 记录最后一行
mLineHeight.add(lineHeight);
mAllViews.add(lineViews);
int left = 0;
int top = 0;
// 得到总行数
int lineNums = mAllViews.size();
for (int i = 0; i < lineNums; i++)
{
// 每一行的所有的views
lineViews = mAllViews.get(i);
// 当前行的最大高度
lineHeight = mLineHeight.get(i);
// 遍历当前行所有的View
for (int j = 0; j < lineViews.size(); j++)
{
View child = lineViews.get(j);
if (child.getVisibility() == View.GONE)
{
continue;
}
MarginLayoutParams lp = (MarginLayoutParams) child
.getLayoutParams();
//计算childView的Marginleft,top,right,bottom
int lc = left + lp.leftMargin;
int tc = top + lp.topMargin;
int rc =lc + child.getMeasuredWidth();
int bc = tc + child.getMeasuredHeight();
child.layout(lc, tc, rc, bc);
left += child.getMeasuredWidth() + lp.rightMargin
+ lp.leftMargin;
}
left = 0;
top += lineHeight;
}
}
}2.属性选择的适配器public class GoodsAttrsAdapter extends BaseRecyclerAdapter<GoodsAttrsBean.AttributesBean> {
private SKUInterface myInterface;
private SimpleArrayMap<Integer, String> saveClick;
private List<GoodsAttrsBean.StockGoodsBean> stockGoodsList;//商品数据集合
private String[] selectedValue; //选中的属性
private TextView[][] childrenViews; //二维 装所有属性
private final int SELECTED = 0x100;
private final int CANCEL = 0x101;
public GoodsAttrsAdapter(Context ctx, List<GoodsAttrsBean.AttributesBean> list, List<GoodsAttrsBean.StockGoodsBean> stockGoodsList) {
super(ctx, list);
this.stockGoodsList = stockGoodsList;
saveClick = new SimpleArrayMap<>();
childrenViews = new TextView[list.size()][0];
selectedValue = new String[list.size()];
for (int i = 0; i < list.size(); i++) {
selectedValue[i] = "";
}
}
public void setSKUInterface(SKUInterface myInterface) {
this.myInterface = myInterface;
}
@Override
public int getItemLayoutId(int viewType) {
return R.layout.item_skuattrs;
}
private SKUViewGroup vg_skuItem;
@Override
public void bindData(RecyclerViewHolder holder, int position, GoodsAttrsBean.AttributesBean item) {
TextView tv_ItemName = holder.getTextView(R.id.tv_ItemName);
vg_skuItem = (SKUViewGroup) holder.getView(R.id.vg_skuItem);
tv_ItemName.setText(item.getTabName());
List<String> childrens = item.getAttributesItem();
int childrenSize = childrens.size();
TextView[] textViews = new TextView[childrenSize];
for (int i = 0; i < childrenSize; i++) {
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT);
params.setMargins(5, 5, 5, 0);
TextView textView = new TextView(mContext);
textView.setGravity(Gravity.CENTER);
textView.setPadding(15, 5, 15, 5);
textView.setLayoutParams(params);
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.saddlebrown));
textView.setText(childrens.get(i));
textView.setTextSize(16);
textView.setTextColor(ContextCompat.getColor(mContext, R.color.white));
textViews[i] = textView;
vg_skuItem.addView(textViews[i]);
}
childrenViews[position] = textViews;
initOptions();
canClickOptions();
getSelected();
}
private int focusPositionG, focusPositionC;
private class MyOnClickListener implements View.OnClickListener {
//点击操作 选中SELECTED 取消CANCEL
private int operation;
private int positionG;
private int positionC;
public MyOnClickListener(int operation, int positionG, int positionC) {
this.operation = operation;
this.positionG = positionG;
this.positionC = positionC;
}
@Override
public void onClick(View v) {
focusPositionG = positionG;
focusPositionC = positionC;
String value = childrenViews[positionG][positionC].getText().toString();
switch (operation) {
case SELECTED:
saveClick.put(positionG, positionC + "");
selectedValue[positionG] = value;
myInterface.selectedAttribute(selectedValue);
break;
case CANCEL:
saveClick.put(positionG, "");
for (int l = 0; l < selectedValue.length; l++) {
if (selectedValue[l].equals(value)) {
selectedValue[l] = "";
break;
}
}
myInterface.uncheckAttribute(selectedValue);
break;
}
initOptions();
canClickOptions();
getSelected();
}
}
/**
* 初始化选项(不可点击,焦点消失)
*/
private void initOptions() {
for (int y = 0; y < childrenViews.length; y++) {
for (int z = 0; z < childrenViews[y].length; z++) {//循环所有属性
TextView textView = childrenViews[y][z];
textView.setEnabled(false);
textView.setFocusable(false);
textView.setTextColor(ContextCompat.getColor(mContext, R.color.gray));//变灰
}
}
}
/**
* 找到符合条件的选项变为可选
*/
private void canClickOptions() {
for (int i = 0; i < childrenViews.length; i++) {
for (int j = 0; j < stockGoodsList.size(); j++) {
boolean filter = false;
List<GoodsAttrsBean.StockGoodsBean.GoodsInfoBean> goodsInfo = stockGoodsList.get(j).getGoodsInfo();
for (int k = 0; k < selectedValue.length; k++) {
if (i == k || TextUtils.isEmpty(selectedValue[k])) {
continue;
}
if (!selectedValue[k].equals(goodsInfo
.get(k).getTabValue())) {
filter = true;
break;
}
}
if (!filter) {
for (int n = 0; n < childrenViews[i].length; n++) {
TextView textView = childrenViews[i]
;//拿到所有属性TextView
String name = textView.getText().toString();
//拿到属性名称
if (goodsInfo.get(i).getTabValue().equals(name)) {
textView.setEnabled(true);//符合就变成可点击
textView.setFocusable(true); //设置可以获取焦点
//不要让焦点乱跑
if (focusPositionG == i && focusPositionC == n) {
textView.setTextColor(ContextCompat.getColor(mContext, R.color.white));//取消选择后的颜色
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.saddlebrown));
textView.requestFocus();
} else {
textView.setTextColor(ContextCompat.getColor(mContext, R.color.white));
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.saddlebrown));
}
textView.setOnClickListener(new MyOnClickListener(SELECTED, i, n));
}
}
}
}
}
}
/**
* 找到已经选中的选项,让其变红
*/
private void getSelected() {
for (int i = 0; i < childrenViews.length; i++) {
for (int j = 0; j < childrenViews[i].length; j++) {//拿到每行属性Item
TextView textView = childrenViews[i][j];//拿到所有属性TextView
String value = textView.getText().toString();
for (int m = 0; m < selectedValue.length; m++) {
if (selectedValue[m].equals(value)) {
textView.setTextColor(ContextCompat.getColor(mContext, R.color.red));
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.pink));
textView.setOnClickListener(new MyOnClickListener(CANCEL, i, j));
}
}
}
}
}
}Demo下载地址:https://download.csdn.net/download/qq_39735504/10286453
1.继承ViewGrouppublic class SKUViewGroup extends ViewGroup {
public SKUViewGroup(Context context, AttributeSet attrs)
{
super(context, attrs);
}
@Override
protected LayoutParams generateLayoutParams(
LayoutParams p)
{
return new MarginLayoutParams(p);
}
@Override
public LayoutParams generateLayoutParams(AttributeSet attrs)
{
return new MarginLayoutParams(getContext(), attrs);
}
@Override
protected LayoutParams generateDefaultLayoutParams()
{
return new MarginLayoutParams(LayoutParams.MATCH_PARENT,
LayoutParams.MATCH_PARENT);
}
/**
* 负责设置子控件的测量模式和大小 根据所有子控件设置自己的宽和高
*/
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec)
{
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
// 获得它的父容器为它设置的测量模式和大小
int sizeWidth = MeasureSpec.getSize(widthMeasureSpec);
int sizeHeight = MeasureSpec.getSize(heightMeasureSpec);
int modeWidth = MeasureSpec.getMode(widthMeasureSpec);
int modeHeight = MeasureSpec.getMode(heightMeasureSpec);
// 如果是warp_content情况下,记录宽和高
int width = 0;
int height = 0;
/**
* 记录每一行的宽度,width不断取最大宽度
*/
int lineWidth = 0;
/**
* 每一行的高度,累加至height
*/
int lineHeight = 0;
int cCount = getChildCount();
// 遍历每个子元素
for (int i = 0; i < cCount; i++)
{
View child = getChildAt(i);
// 测量每一个child的宽和高
measureChild(child, widthMeasureSpec, heightMeasureSpec);
// 得到child的布局管理器
MarginLayoutParams lp = (MarginLayoutParams) child
.getLayoutParams();
// 当前子空间实际占据的宽度
int childWidth = child.getMeasuredWidth() + lp.leftMargin
+ lp.rightMargin;
// 当前子空间实际占据的高度
int childHeight = child.getMeasuredHeight() + lp.topMargin
+ lp.bottomMargin;
/**
* 如果加入当前child,则超出最大宽度,则的到目前最大宽度给width,类加height 然后开启新行
*/
if (lineWidth + childWidth > sizeWidth)
{
width = Math.max(lineWidth, childWidth);// 取最大的
lineWidth = childWidth; // 重新开启新行,开始记录
// 叠加当前高度,
height += lineHeight;
// 开启记录下一行的高度
lineHeight = childHeight;
} else
// 否则累加值lineWidth,lineHeight取最大高度
{
lineWidth += childWidth;
lineHeight = Math.max(lineHeight, childHeight);
}
// 如果是最后一个,则将当前记录的最大宽度和当前lineWidth做比较
if (i == cCount - 1)
{
width = Math.max(width, lineWidth);
height += lineHeight;
}
}
setMeasuredDimension((modeWidth == MeasureSpec.EXACTLY) ? sizeWidth
: width, (modeHeight == MeasureSpec.EXACTLY) ? sizeHeight
: height);
}
/**
* 存储所有的View,按行记录
*/
private List<List<View>> mAllViews = new ArrayList<>();
/**
* 记录每一行的最大高度
*/
private List<Integer> mLineHeight = new ArrayList<>();
@Override
protected void onLayout(boolean changed, int l, int t, int r, int b)
{
mAllViews.clear();
mLineHeight.clear();
int width = getWidth();
int lineWidth = 0;
int lineHeight = 0;
// 存储每一行所有的childView
List<View> lineViews = new ArrayList<>();
int cCount = getChildCount();
// 遍历所有的孩子
for (int i = 0; i < cCount; i++)
{
View child = getChildAt(i);
MarginLayoutParams lp = (MarginLayoutParams) child
.getLayoutParams();
int childWidth = child.getMeasuredWidth();
int childHeight = child.getMeasuredHeight();
// 如果已经需要换行
if (childWidth + lp.leftMargin + lp.rightMargin + lineWidth > width)
{
// 记录这一行所有的View以及最大高度
mLineHeight.add(lineHeight);
// 将当前行的childView保存,然后开启新的ArrayList保存下一行的childView
mAllViews.add(lineViews);
lineWidth = 0;// 重置行宽
lineViews = new ArrayList<>();
}
/**
* 如果不需要换行,则累加
*/
lineWidth += childWidth + lp.leftMargin + lp.rightMargin;
lineHeight = Math.max(lineHeight, childHeight + lp.topMargin
+ lp.bottomMargin);
lineViews.add(child);
}
// 记录最后一行
mLineHeight.add(lineHeight);
mAllViews.add(lineViews);
int left = 0;
int top = 0;
// 得到总行数
int lineNums = mAllViews.size();
for (int i = 0; i < lineNums; i++)
{
// 每一行的所有的views
lineViews = mAllViews.get(i);
// 当前行的最大高度
lineHeight = mLineHeight.get(i);
// 遍历当前行所有的View
for (int j = 0; j < lineViews.size(); j++)
{
View child = lineViews.get(j);
if (child.getVisibility() == View.GONE)
{
continue;
}
MarginLayoutParams lp = (MarginLayoutParams) child
.getLayoutParams();
//计算childView的Marginleft,top,right,bottom
int lc = left + lp.leftMargin;
int tc = top + lp.topMargin;
int rc =lc + child.getMeasuredWidth();
int bc = tc + child.getMeasuredHeight();
child.layout(lc, tc, rc, bc);
left += child.getMeasuredWidth() + lp.rightMargin
+ lp.leftMargin;
}
left = 0;
top += lineHeight;
}
}
}2.属性选择的适配器public class GoodsAttrsAdapter extends BaseRecyclerAdapter<GoodsAttrsBean.AttributesBean> {
private SKUInterface myInterface;
private SimpleArrayMap<Integer, String> saveClick;
private List<GoodsAttrsBean.StockGoodsBean> stockGoodsList;//商品数据集合
private String[] selectedValue; //选中的属性
private TextView[][] childrenViews; //二维 装所有属性
private final int SELECTED = 0x100;
private final int CANCEL = 0x101;
public GoodsAttrsAdapter(Context ctx, List<GoodsAttrsBean.AttributesBean> list, List<GoodsAttrsBean.StockGoodsBean> stockGoodsList) {
super(ctx, list);
this.stockGoodsList = stockGoodsList;
saveClick = new SimpleArrayMap<>();
childrenViews = new TextView[list.size()][0];
selectedValue = new String[list.size()];
for (int i = 0; i < list.size(); i++) {
selectedValue[i] = "";
}
}
public void setSKUInterface(SKUInterface myInterface) {
this.myInterface = myInterface;
}
@Override
public int getItemLayoutId(int viewType) {
return R.layout.item_skuattrs;
}
private SKUViewGroup vg_skuItem;
@Override
public void bindData(RecyclerViewHolder holder, int position, GoodsAttrsBean.AttributesBean item) {
TextView tv_ItemName = holder.getTextView(R.id.tv_ItemName);
vg_skuItem = (SKUViewGroup) holder.getView(R.id.vg_skuItem);
tv_ItemName.setText(item.getTabName());
List<String> childrens = item.getAttributesItem();
int childrenSize = childrens.size();
TextView[] textViews = new TextView[childrenSize];
for (int i = 0; i < childrenSize; i++) {
LinearLayout.LayoutParams params = new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT);
params.setMargins(5, 5, 5, 0);
TextView textView = new TextView(mContext);
textView.setGravity(Gravity.CENTER);
textView.setPadding(15, 5, 15, 5);
textView.setLayoutParams(params);
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.saddlebrown));
textView.setText(childrens.get(i));
textView.setTextSize(16);
textView.setTextColor(ContextCompat.getColor(mContext, R.color.white));
textViews[i] = textView;
vg_skuItem.addView(textViews[i]);
}
childrenViews[position] = textViews;
initOptions();
canClickOptions();
getSelected();
}
private int focusPositionG, focusPositionC;
private class MyOnClickListener implements View.OnClickListener {
//点击操作 选中SELECTED 取消CANCEL
private int operation;
private int positionG;
private int positionC;
public MyOnClickListener(int operation, int positionG, int positionC) {
this.operation = operation;
this.positionG = positionG;
this.positionC = positionC;
}
@Override
public void onClick(View v) {
focusPositionG = positionG;
focusPositionC = positionC;
String value = childrenViews[positionG][positionC].getText().toString();
switch (operation) {
case SELECTED:
saveClick.put(positionG, positionC + "");
selectedValue[positionG] = value;
myInterface.selectedAttribute(selectedValue);
break;
case CANCEL:
saveClick.put(positionG, "");
for (int l = 0; l < selectedValue.length; l++) {
if (selectedValue[l].equals(value)) {
selectedValue[l] = "";
break;
}
}
myInterface.uncheckAttribute(selectedValue);
break;
}
initOptions();
canClickOptions();
getSelected();
}
}
/**
* 初始化选项(不可点击,焦点消失)
*/
private void initOptions() {
for (int y = 0; y < childrenViews.length; y++) {
for (int z = 0; z < childrenViews[y].length; z++) {//循环所有属性
TextView textView = childrenViews[y][z];
textView.setEnabled(false);
textView.setFocusable(false);
textView.setTextColor(ContextCompat.getColor(mContext, R.color.gray));//变灰
}
}
}
/**
* 找到符合条件的选项变为可选
*/
private void canClickOptions() {
for (int i = 0; i < childrenViews.length; i++) {
for (int j = 0; j < stockGoodsList.size(); j++) {
boolean filter = false;
List<GoodsAttrsBean.StockGoodsBean.GoodsInfoBean> goodsInfo = stockGoodsList.get(j).getGoodsInfo();
for (int k = 0; k < selectedValue.length; k++) {
if (i == k || TextUtils.isEmpty(selectedValue[k])) {
continue;
}
if (!selectedValue[k].equals(goodsInfo
.get(k).getTabValue())) {
filter = true;
break;
}
}
if (!filter) {
for (int n = 0; n < childrenViews[i].length; n++) {
TextView textView = childrenViews[i]
;//拿到所有属性TextView
String name = textView.getText().toString();
//拿到属性名称
if (goodsInfo.get(i).getTabValue().equals(name)) {
textView.setEnabled(true);//符合就变成可点击
textView.setFocusable(true); //设置可以获取焦点
//不要让焦点乱跑
if (focusPositionG == i && focusPositionC == n) {
textView.setTextColor(ContextCompat.getColor(mContext, R.color.white));//取消选择后的颜色
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.saddlebrown));
textView.requestFocus();
} else {
textView.setTextColor(ContextCompat.getColor(mContext, R.color.white));
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.saddlebrown));
}
textView.setOnClickListener(new MyOnClickListener(SELECTED, i, n));
}
}
}
}
}
}
/**
* 找到已经选中的选项,让其变红
*/
private void getSelected() {
for (int i = 0; i < childrenViews.length; i++) {
for (int j = 0; j < childrenViews[i].length; j++) {//拿到每行属性Item
TextView textView = childrenViews[i][j];//拿到所有属性TextView
String value = textView.getText().toString();
for (int m = 0; m < selectedValue.length; m++) {
if (selectedValue[m].equals(value)) {
textView.setTextColor(ContextCompat.getColor(mContext, R.color.red));
textView.setBackgroundColor(ContextCompat.getColor(mContext, R.color.pink));
textView.setOnClickListener(new MyOnClickListener(CANCEL, i, j));
}
}
}
}
}
}Demo下载地址:https://download.csdn.net/download/qq_39735504/10286453
相关文章推荐
- Android 选择商品属性sku
- jquery特效 商品SKU属性规格选择实时联动
- Android多维商品属性SKU选择
- jquery特效 商品SKU属性规格选择实时联动
- android商品属性选择标签控件,可实现自动换行
- 利用AjaxPro2实现商品属性选择(C#)
- 类似于淘宝的sku属性选择动态生成
- Android 动画之淘宝商品选择型号动画
- [ecshop 资料]商品显示 选择属性名称然后标题也加上属性的名称
- ecshop商品属性仿淘宝选择功能
- 商品SKU属性添加、上传图片、生成列表并生成json传给后台(支持IE8、谷歌、火狐等主流浏览器)
- 相关淘宝的名词解释,SPU 关键属性 销售属性 商品属性 非关键属性 SKU AppKey 等
- 商品sku规格选择效果,没有商品的不能选中,选择顺序不影响展示结果
- 前端如何展示商品属性:SKU多维属性状态判断算法的应用-Vue 实现
- Android 购物车选择商品加减和价格合计
- android:高仿京东商品属性筛选(流式布局)
- 商品SKU选择实现
- ecshop商品属性仿淘宝选择功能
- 选择商品属性
- android用sku算法仿淘宝选择颜色分类弹框。