EChat(简易聊天项目)七、保存聊天记录
2018-02-24 22:28
288 查看
(二)使用LitePal数据库保存用户聊天记录
分析:
首先需要进行数据库的创建,需要一张表(自定义类HistoryChat)来保存聊天记录信息,
其次,需要将聊天的记录增加到数据库对应记录中;最后需要对数据库中对应的用户和对应的聊天记录查询,设置查询条件约束(其中包含了对用户和聊天对象和聊天记录的绑定,如点击不同好友是显示的不同的历史信息)等等。
主要部分代码实现:
① 由于之前已有用于存储用户名和密码的LitePal数据库,直接添加对应部分代码
litepal.xml<mapping class="com.example.androidlogin.HistoryChat"></mapping>
新建一个类HistoryChat
② 新建一个用于展示聊天记录的HistoryActivitypublic class HistoryActivity extends AppCompatActivity {
private List<Msg> msgList = new ArrayList<>();
public String username;
public String receiver;
private RecyclerView msgRecyclerView;
private MsgAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.history_layout);
Intent intent = getIntent();
username = intent.getStringExtra("username");
receiver = intent.getStringExtra("receiver");
initMsg();//写入历史记录
msgRecyclerView = (RecyclerView) findViewById(R.id.msg_recycle_view);
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
msgRecyclerView.setLayoutManager(layoutManager);
adapter = new MsgAdapter(msgList);
msgRecyclerView.setAdapter(adapter);
}
//数据库查找对应聊天记录
public void initMsg() {
List<HistoryChat> historylist = DataSupport.where("Sender = ? and Receiver = ?",username,receiver).find(HistoryChat.class);
for (HistoryChat historychat : historylist){
if (historychat.getReceivetext() == null) {
String text = historychat.getSendtext();
String time = historychat.getTime();
Msg msg = new Msg(text + "--" + time, null, Msg.TYPE_SENT);
msgList.add(msg);
}
if(historychat.getReceivetext() != null){
String text1 = historychat.getReceivetext();
String time1 = historychat.getTime();
Msg msg1 = new Msg(text1 + " --" + time1, null, Msg.TYPE_RECEIVED);
msgList.add(msg1);
}
}
}
}
③ 新建的history_layout.xml中添加代码(即原有聊天界面去掉下方发送栏即可) <?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="#d8e0e8">
<android.support.v7.widget.RecyclerView
android:id="@+id/msg_recycle_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"/>
</LinearLayout>
④在MessageActivity中进行代码修改,发送消息和设置初始消息部分添加了将数据增加到数据库中的操作/*
选择好友后进入的聊天界面
*/
public class MessageActivity extends AppCompatActivity {
private List<Msg> msgList = new ArrayList<>();
private EditText inputText;
private Button send;
private Button more;
public String username;
public String receiver;
private ImageView imageView;
//调用系统相册-选择图片
private static final int IMAGE = 1;
private RecyclerView msgRecyclerView;
private MsgAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Intent intent = getIntent();
username = intent.getStringExtra("username");
receiver = intent.getStringExtra("receiver");
//将正在创建的活动添加到活动管理器中
ActivityCollector.addActivity(this);
setContentView(R.layout.message__layout);
Connector.getDatabase();
initMsg();//初始化消息数据
//新建LitePal数据库
inputText = (EditText) findViewById(R.id.input_text);
send = (Button) findViewById(R.id.send);
msgRecyclerView = (RecyclerView) findViewById(R.id.msg_recycle_view);
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
msgRecyclerView.setLayoutManager(layoutManager);
adapter = new MsgAdapter(msgList);
msgRecyclerView.setAdapter(adapter);
send.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String content = inputText.getText().toString();
if (!"".equals(content)){
Msg msg = new Msg(content,null,Msg.TYPE_SENT);
msgList.add(msg);
adapter.notifyItemInserted(msgList.size() - 1);//当有新消息时,刷新RecyclerView中的显示
msgRecyclerView.scrollToPosition(msgList.size() - 1);//将RecyclerView定位到最后一行
inputText.setText("");//清空输入框中的内容
//获取系统当前时间
SimpleDateFormat format = new SimpleDateFormat("yyyy.MM.dd. HH:mm:ss");
Date date = new Date(System.currentTimeMillis());
String time = format.format(date);
//存储数据到数据库
HistoryChat historyChat = new HistoryChat();
historyChat.setReceiver(receiver);
historyChat.setSender(username);
historyChat.setSendtext(content);
historyChat.setTime(time);
historyChat.save();
}
}
});
...
private void initMsg() {
//获取系统当前时间
SimpleDateFormat format = new SimpleDateFormat("yyyy.MM.dd. HH:mm:ss");
Date date = new Date(System.currentTimeMillis());
String time = format.format(date);
Msg msg1 = new Msg("Hello guy.",null, Msg.TYPE_RECEIVED);
msgList.add(msg1);
HistoryChat historyChat = new HistoryChat();
historyChat.setReceiver(receiver);
historyChat.setSender(username);
historyChat.setReceivetext("Hello guy.");
historyChat.setTime(time);
historyChat.save();
Msg msg2 = new Msg("Hello.Who is that?",null, Msg.TYPE_SENT);
msgList.add(msg2);
HistoryChat historyChat1 = new HistoryChat();
historyChat1.setReceiver(receiver);
historyChat1.setSender(username);
historyChat1.setSendtext("Hello.Who is that?");
historyChat1.setTime(time);
historyChat1.save();
Msg msg3 = new Msg("This is Tom. Nice talking to you.",null,Msg.TYPE_RECEIVED);
msgList.add(msg3);
HistoryChat historyChat2 = new HistoryChat();
historyChat2.setReceiver(receiver);
historyChat2.setSender(username);
historyChat2.setReceivetext("This is Tom. Nice talking to you.");
historyChat2.setTime(time);
historyChat2.save();
}
...
}
运行效果(先选择聊天对象)
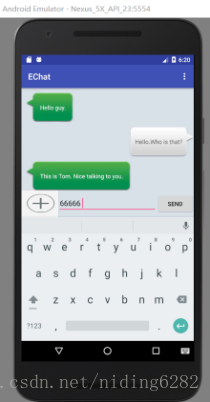
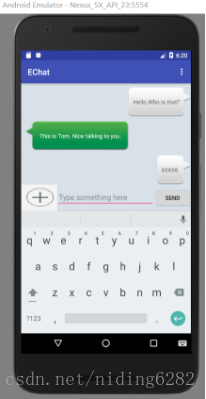
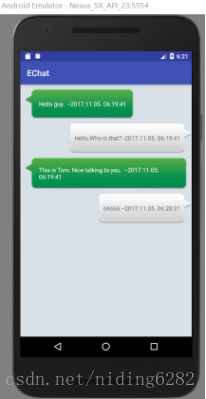
分析:
首先需要进行数据库的创建,需要一张表(自定义类HistoryChat)来保存聊天记录信息,
其次,需要将聊天的记录增加到数据库对应记录中;最后需要对数据库中对应的用户和对应的聊天记录查询,设置查询条件约束(其中包含了对用户和聊天对象和聊天记录的绑定,如点击不同好友是显示的不同的历史信息)等等。
主要部分代码实现:
① 由于之前已有用于存储用户名和密码的LitePal数据库,直接添加对应部分代码
litepal.xml<mapping class="com.example.androidlogin.HistoryChat"></mapping>
新建一个类HistoryChat
/* 聊天记录历史记录实体类 */ public class HistoryChat extends DataSupport{ String sendtext; String receivetext; String Sender; String Receiver; String time; public String getSender() { return Sender; } public void setSender(String sender) { Sender = sender; } public String getReceiver() { return Receiver; } public void setReceiver(String receiver) { Receiver = receiver; } public String getReceivetext() { return receivetext; } public void setReceivetext(String receivetext) { this.receivetext = receivetext; } public String getSendtext() { return sendtext; } public void setSendtext(String sendtext) { this.sendtext = sendtext; } public String getTime() { return time; } public void setTime(String time) { this.time = time; } }
② 新建一个用于展示聊天记录的HistoryActivitypublic class HistoryActivity extends AppCompatActivity {
private List<Msg> msgList = new ArrayList<>();
public String username;
public String receiver;
private RecyclerView msgRecyclerView;
private MsgAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.history_layout);
Intent intent = getIntent();
username = intent.getStringExtra("username");
receiver = intent.getStringExtra("receiver");
initMsg();//写入历史记录
msgRecyclerView = (RecyclerView) findViewById(R.id.msg_recycle_view);
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
msgRecyclerView.setLayoutManager(layoutManager);
adapter = new MsgAdapter(msgList);
msgRecyclerView.setAdapter(adapter);
}
//数据库查找对应聊天记录
public void initMsg() {
List<HistoryChat> historylist = DataSupport.where("Sender = ? and Receiver = ?",username,receiver).find(HistoryChat.class);
for (HistoryChat historychat : historylist){
if (historychat.getReceivetext() == null) {
String text = historychat.getSendtext();
String time = historychat.getTime();
Msg msg = new Msg(text + "--" + time, null, Msg.TYPE_SENT);
msgList.add(msg);
}
if(historychat.getReceivetext() != null){
String text1 = historychat.getReceivetext();
String time1 = historychat.getTime();
Msg msg1 = new Msg(text1 + " --" + time1, null, Msg.TYPE_RECEIVED);
msgList.add(msg1);
}
}
}
}
③ 新建的history_layout.xml中添加代码(即原有聊天界面去掉下方发送栏即可) <?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="#d8e0e8">
<android.support.v7.widget.RecyclerView
android:id="@+id/msg_recycle_view"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"/>
</LinearLayout>
④在MessageActivity中进行代码修改,发送消息和设置初始消息部分添加了将数据增加到数据库中的操作/*
选择好友后进入的聊天界面
*/
public class MessageActivity extends AppCompatActivity {
private List<Msg> msgList = new ArrayList<>();
private EditText inputText;
private Button send;
private Button more;
public String username;
public String receiver;
private ImageView imageView;
//调用系统相册-选择图片
private static final int IMAGE = 1;
private RecyclerView msgRecyclerView;
private MsgAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
Intent intent = getIntent();
username = intent.getStringExtra("username");
receiver = intent.getStringExtra("receiver");
//将正在创建的活动添加到活动管理器中
ActivityCollector.addActivity(this);
setContentView(R.layout.message__layout);
Connector.getDatabase();
initMsg();//初始化消息数据
//新建LitePal数据库
inputText = (EditText) findViewById(R.id.input_text);
send = (Button) findViewById(R.id.send);
msgRecyclerView = (RecyclerView) findViewById(R.id.msg_recycle_view);
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
msgRecyclerView.setLayoutManager(layoutManager);
adapter = new MsgAdapter(msgList);
msgRecyclerView.setAdapter(adapter);
send.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String content = inputText.getText().toString();
if (!"".equals(content)){
Msg msg = new Msg(content,null,Msg.TYPE_SENT);
msgList.add(msg);
adapter.notifyItemInserted(msgList.size() - 1);//当有新消息时,刷新RecyclerView中的显示
msgRecyclerView.scrollToPosition(msgList.size() - 1);//将RecyclerView定位到最后一行
inputText.setText("");//清空输入框中的内容
//获取系统当前时间
SimpleDateFormat format = new SimpleDateFormat("yyyy.MM.dd. HH:mm:ss");
Date date = new Date(System.currentTimeMillis());
String time = format.format(date);
//存储数据到数据库
HistoryChat historyChat = new HistoryChat();
historyChat.setReceiver(receiver);
historyChat.setSender(username);
historyChat.setSendtext(content);
historyChat.setTime(time);
historyChat.save();
}
}
});
...
private void initMsg() {
//获取系统当前时间
SimpleDateFormat format = new SimpleDateFormat("yyyy.MM.dd. HH:mm:ss");
Date date = new Date(System.currentTimeMillis());
String time = format.format(date);
Msg msg1 = new Msg("Hello guy.",null, Msg.TYPE_RECEIVED);
msgList.add(msg1);
HistoryChat historyChat = new HistoryChat();
historyChat.setReceiver(receiver);
historyChat.setSender(username);
historyChat.setReceivetext("Hello guy.");
historyChat.setTime(time);
historyChat.save();
Msg msg2 = new Msg("Hello.Who is that?",null, Msg.TYPE_SENT);
msgList.add(msg2);
HistoryChat historyChat1 = new HistoryChat();
historyChat1.setReceiver(receiver);
historyChat1.setSender(username);
historyChat1.setSendtext("Hello.Who is that?");
historyChat1.setTime(time);
historyChat1.save();
Msg msg3 = new Msg("This is Tom. Nice talking to you.",null,Msg.TYPE_RECEIVED);
msgList.add(msg3);
HistoryChat historyChat2 = new HistoryChat();
historyChat2.setReceiver(receiver);
historyChat2.setSender(username);
historyChat2.setReceivetext("This is Tom. Nice talking to you.");
historyChat2.setTime(time);
historyChat2.save();
}
...
}
运行效果(先选择聊天对象)
相关文章推荐
- EChat(简易聊天项目)五、存储聊天记录中的图片
- EChat(简易聊天项目)二、好友列表实现
- EChat(简易聊天项目)八、Socket实现即时通信(包括部分修改)
- 实现从“环信”下载聊天记录,显示在本地项目页面的功能(三)—— 将解析后的数据保存到本地DB中
- EChat(简易聊天项目)一、登录注册实现
- EChat(简易聊天项目)三、聊天界面UI实现
- EChat(简易聊天项目)四、模拟强制下线
- EChat(简易聊天项目)六、实现记住密码和自动登录
- 修改QQ各版本的默认保存位置(聊天记录)
- lync本地聊天记录修改目录保存的方法
- 终于把聊天记录保存到数据库当中去了,
- 保存图片到本项目data/data下,实现简易照片隐藏功能
- 手动设置QQ聊天记录保存目录
- 修改QQ各版本的默认保存位置(聊天记录)
- 项目记录:登陆( 保存用户名密码 kaptcha验证码 shiro权限管理 RSA加密 非明文保存)
- 请问emesene怎么保存聊天记录?
- 纯Ajax架构的网站 保存qq聊天记录 制成精美电子书
- 如何将QQ聊天记录保存或导入到新安装的QQ里面!
- <转>修改TM2013聊天记录保存目录final版
- 聊天记录保存方式