Java泛型07 : Java自带的泛型Collection、List、Map、Set以及工具类Arrays和Collections
2018-02-23 23:03
766 查看
超级通道: Java泛型学习系列-绪论
本章主要对Java自带的泛型Collection、List、Map和Set进行简单示例。
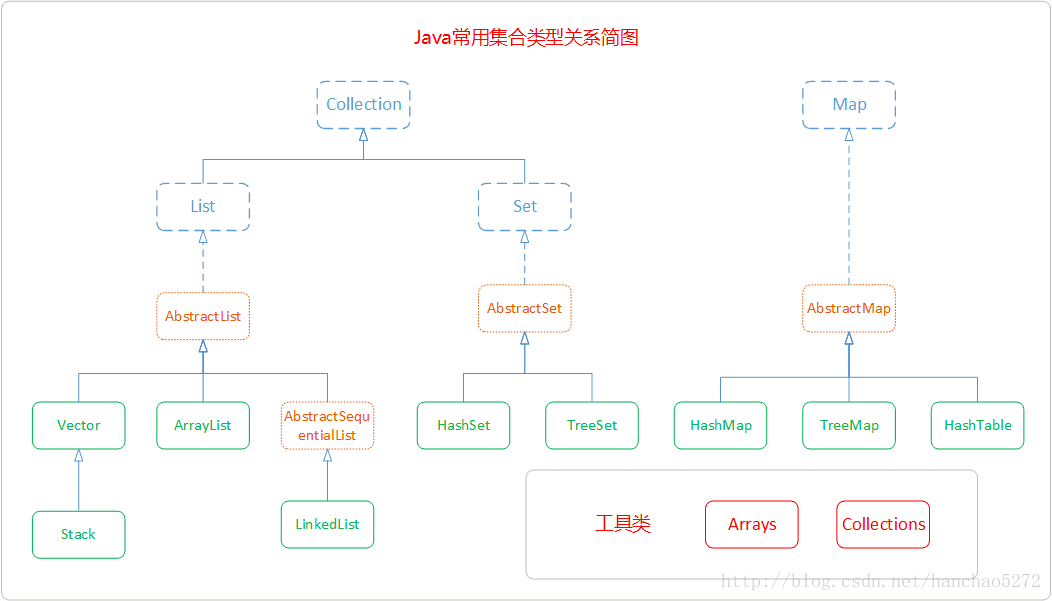
Java在集合类型的定义中使用了大量的泛型,下面对这些常见的类型进行学习。
运行结果:
运行结果:
运行结果:
其实在Java集合框架中,还有更多的泛型类和泛型方法,这里就不一一例句了。
本章主要对Java自带的泛型Collection、List、Map和Set进行简单示例。
1.Java集合
首先回顾一下常用的Java集合类型的相关接口和类之间的关系:Java在集合类型的定义中使用了大量的泛型,下面对这些常见的类型进行学习。
2.List和Set
示例代码:/** * 演示Java自带泛型的集合Collection类型 * Created by 韩超 on 2018/1/30. */ public class CollectionPrintUtils { private final static Logger LOGGER = Logger.getLogger(CollectionPrintUtils.class); /** * <p>Title: 打印集合</p> * @author 韩超@bksx 2018/1/31 13:31 */ public static <E extends Collection> void pringCollection(E collection){ if(null == collection || collection.isEmpty()) { LOGGER.info("无记录"); }else { //java lambda表达式 collection.forEach(LOGGER::info); } } /** * <p>Java泛型在List和Set中的使用</p> * @author hanchao 2018/2/23 22:13 **/ public static void main(String[] args) { //List List<Integer> integerList = new ArrayList<Integer>(); integerList.add(new Integer(1)); integerList.add(2); integerList.add(new Integer(3)); LOGGER.info("integerList的元素如下:"); CollectionPrintUtils.pringCollection(integerList); //Set Set<String > stringSet = new HashSet<String>(); stringSet.add("set1"); stringSet.add("set2"); stringSet.add("set3"); LOGGER.info("stringSet的元素如下"); CollectionPrintUtils.pringCollection(stringSet); } }
运行结果:
2018-02-23 22:57:44 INFO CollectionPrintUtils:37 - integerList的元素如下: 2018-02-23 22:57:44 INFO CollectionPrintUtils:1249 - 1 2018-02-23 22:57:44 INFO CollectionPrintUtils:1249 - 2 2018-02-23 22:57:44 INFO CollectionPrintUtils:1249 - 3 2018-02-23 22:57:44 INFO CollectionPrintUtils:44 - stringSet的元素如下 2018-02-23 22:57:44 INFO CollectionPrintUtils:75 - set3 2018-02-23 22:57:44 INFO CollectionPrintUtils:75 - set2 2018-02-23 22:57:44 INFO CollectionPrintUtils:75 - set1
3.Map
示例代码:/** * 演示Java自带泛型的集合Map类型 * Created by 韩超 on 2018/1/31. */ public class MapPrintUtils { private final static Logger LOGGER = Logger.getLogger(MapPrintUtils.class); /** * <p>Title: 打印Map</p> * @author 韩超@bksx 2018/1/31 14:28 */ public static <E extends Map> void printSet(E map){ if (null == map || map.isEmpty()){ LOGGER.info("当前Map无值!"); }else { for (Object key : map.keySet()){ Object value = map.get(key); LOGGER.info("key:" + key + ",value:" + value); } } } /** * <p>Java泛型在Map中的使用</p> * @author hanchao 2018/2/23 22:14 **/ public static void main(String[] args) { //hashMap Map<String ,String> hashMap = new HashMap<>(); hashMap.put("key1","value1"); hashMap.put(null,"value2"); hashMap.put("key3",null); LOGGER.info("hashMap的元素如下"); MapPrintUtils.printSet(hashMap); //hashTable Map<Integer,Double> hashTable = new Hashtable<>(); hashTable.put(1,2D); hashTable.put(2,3D); hashTable.put(3,4D); LOGGER.info("hashTable的元素如下:"); MapPrintUtils.printSet(hashTable); } }
运行结果:
2018-02-23 22:58:35 INFO MapPrintUtils:40 - hashMap的元素如下 2018-02-23 22:58:35 INFO MapPrintUtils:26 - key:key1,value:value1 2018-02-23 22:58:35 INFO MapPrintUtils:26 - key:null,value:value2 2018-02-23 22:58:35 INFO MapPrintUtils:26 - key:key3,value:null 2018-02-23 22:58:35 INFO MapPrintUtils:48 - hashTable的元素如下: 2018-02-23 22:58:35 INFO MapPrintUtils:26 - key:3,value:4.0 2018-02-23 22:58:35 INFO MapPrintUtils:26 - key:2,value:3.0 2018-02-23 22:58:35 INFO MapPrintUtils:26 - key:1,value:2.0
4.Arrays和Collections
示例代码:/** * <p>集合工具类Collections和Arrays中的泛型示例</p> * @author hanchao 2018/2/23 22:33 **/ public class ArraysAndCollectionsUtils { private static final Logger LOGGER = Logger.getLogger(ArraysAndCollectionsUtils.class); public static void main(String[] args) { LOGGER.info("Arrays工具类中的泛型示例:public static <T> List<T> asList(T... a) {}"); List<? extends Number> numberList = Arrays.asList(1,2D,3L,4.0F); numberList.forEach(LOGGER::info); System.out.println(); LOGGER.info("Collections工具类中的泛型示例:二分查找public static <T>" + " int binarySearch(List<? extends Comparable<? super T>> list, T key) {}"); List<Integer> integerList = new ArrayList<>(); integerList.add(1); integerList.add(2); integerList.add(3); integerList.add(4); LOGGER.info("二分查找:" + Collections.binarySearch(integerList,3)); System.out.println(); LOGGER.info("Collections工具类中的泛型示例:排序public static <T extends Comparable<? super T>> void sort(List<T> list) {}"); List<Double> doubleList = Arrays.asList(2D,1D,3D); LOGGER.info("第一次打印:"); doubleList.forEach(LOGGER::info); LOGGER.info("排序"); Collections.sort(doubleList); LOGGER.info("第二次打印:"); doubleList.forEach(LOGGER::info); System.out.println(); LOGGER.info("Collections工具类中的泛型示例:排序public static <T> void copy(List<? super T> dest, List<? extends T> src) {}"); List<String > stringList = Arrays.asList("A","B","C"); List<String > stringList2 = Arrays.asList("a","b","c","d"); Collections.copy(stringList2,stringList); LOGGER.info(stringList2); } }
运行结果:
2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:18 - Arrays工具类中的泛型示例:public static <T> List<T> asList(T... a) {} 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 1 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 2.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 3 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 4.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:23 - Collections工具类中的泛型示例:二分查找public static <T> int binarySearch(List<? extends Comparable<? super T>> list, T key) {} 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:30 - 二分查找:2 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:33 - Collections工具类中的泛型示例:排序public static <T extends Comparable<? super T>> void sort(List<T> list) {} 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:35 - 第一次打印: 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 2.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 1.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 3.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:37 - 排序 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:39 - 第二次打印: 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 1.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 2.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:3880 - 3.0 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:43 - Collections工具类中的泛型示例:排序public static <T> void copy(List<? super T> dest, List<? extends T> src) {} 2018-02-23 23:00:02 INFO ArraysAndCollectionsUtils:47 - [A, B, C, d]
5.总结
由于这些集合类和工具类提供的静态方法都比较常见,上面没有过多描述。其实在Java集合框架中,还有更多的泛型类和泛型方法,这里就不一一例句了。
相关文章推荐
- JAVA 集合类(Collection)、List、Set、Map、Collections与Arrays、泛型
- JAVA基础学习之 Map集合、集合框架工具类Collections,Arrays、可变参数、List和Set集合框架什么时候使用等(4)
- 黑马程序员——JAVA基础——集合---概述、Collection中共性方法、List、Map、工具类Collections和Arrays
- 黑马程序员——Java语言基础:集合框架(Collection、Map,工具类Collections、Arrays)
- 黑马程序员——6.集合类(String/StringBuffer、List、Set、Map、Collections工具类、Arrays工具类 )
- Java学习日记(九)Collection、泛型、Map集合、Collections&Arrays、增强for语句、可变参数、静态导入
- 黑马程序员_java_集合框架_Collection_List_Set_Map_泛型
- java 集合架构--[Collection] [List] [Set] [Map] [集合工具类]
- List方法及去重,泛型(方法,类,接口),泛型通配符,迭代器,可变参数,set,map,Collections工具类,Comparator
- 3.9 java基础总结集合①LIst②Set③Map④泛型⑤Collections
- java中Collection/Collections;List/Set/Map;ArrayList/Vector/LinkedList;HashSet/HashMap/TreeSet/TreeMap
- java 集合架构--[Collection] [List] [Set] [Map] [集合工具类]
- java 集合架构--[Collection] [List] [Set] [Map] [集合工具类]
- java 集合collection set list map 泛型
- 黑马程序员----Collection和Collections有什么关系?List和Set有什么异同点?Map有哪些常用类,各有什么特点?
- java 集合 list map set collection
- Java -- 容器使用 Set, List, Map, Queue, Collections
- Java基础---泛型、集合框架工具类:collections和Arrays (黑马程序员)
- Map(接口简介、HashMap集合、TreeMap集合、Properties、泛型、Collections工具类、Arrays工具类)
- Java 容器–Collection, List, Set, Map