使用D3.js进行数据可视化
2018-02-13 12:20
501 查看
楼主最近在做一个将特定图结构的数据进行可视化的项目,用到了前段可视化库D3.js,在这里分享一下学习心得。
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
[/code]
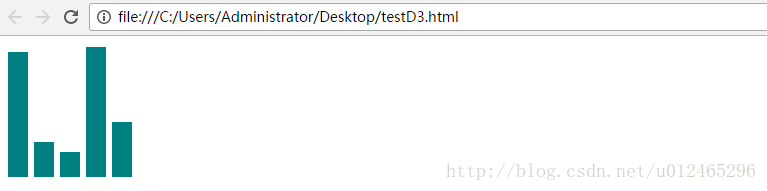
D3库的引用。开头使用
元素选择。D3支持CSS3的选择器语法,可通过tag、class、id等属性对元素进行选择。
数据绑定。 D3的基础原理是将页面中的可视化元素,如段落,层(html元素),圆、路径、线段(SVG元素)与真实数据进行绑定,data方法就是用来绑定元素和数据,该方法会循环遍历数据集中的每一个数据。
enter 为每个待绑定的数据生成一个占位符对象(页面元素),后续可以操作这些对象生成具体的可视化元素。
append方法向一个Dom元素中添加对象,这里页面原本一个DIV层都没有,但是先取得占位符对象(包含对应数据),然后通过添加DIV层,并使用attr和style方法设置该DIV的高度,从而在最后的页面上显示出一幅条形图
需要指出的是,d3.select和d3.selectAll将默认查询整个文档,但是selection.select和selection.selectAll则只在当前selection范围里查找
D3的函数使用也支持连缀用法,多个函数连用时,前一个函数的返回值将作为下一个函数的调用主体,例如
如果参数是name,value键值对形式,则会将selection中的元素的name属性的值设置成value,如果value是常数,那么seletion中所有的元素都将设置成相同的值,如果value是函数的形式,那么这个函数将会获得参数当前元素d和当前元素在集合中的索引作为参数,函数的返回值用来设置每个元素的name属性。如果是name的形式,则将返回该集合中元素中name属性的值。
function中可获得全部参数有:
being passed the current datum (d), the current index (i), and the current group (nodes), with this as the current DOM element.
name指定想设置的class名称,value为true表示添加该class属性,为false表示不分配该class属性,用法与上面类似。但是如何删除已有的class属性?
2
[/code]
[/code]
如果value不为空,则用该value替代选中元素所有的子元素并返回该元素。value为空,则返回该selection中第一个文字内容非空的元素的text content
If the specified type is a string, appends a new element of this type (tag name) as the last child of each selected element, or the next following sibling in the update selection if this is an enter selection.
2
3
4
5
6
7
8
9
10
[/code]
,enter和exit将在下面介绍。指定的data可是是一组任意的数据,数字或者对象。
绑定的顺序由函数key来决定,如果key为空,则绑定顺序为默认按照索引来,即第一个元素绑定到第一个数据,第二个元素绑定到第二个数据,可以通过指定key函数来设置不同的元素绑定顺序。
注意:selection中元素的个数和data数据集中个数并不总是一一对应,可能会出现元素个数较少或者数据集个数较少的情况,为了使元素和数据一一对应,便有了enter 和 exit方法。
为selection绑定的数据集中,尚未绑定dom元素的数据创建dom占位符结点。如果一个selection为空,但它绑定了一个数据集,那么enter方法将为这些数据集中的每一个数据创建一个占位符结点放到selection中,后续的dom操作可以使用该占位符结点。
2
3
4
5
6
7
[/code]
2
3
4
5
6
7
[/code]
选中元素集中存在一些dom元素,没有数据与之绑定,这个方法就将这些元素返回。
enter和exit结合使用,可以很方便的再数据集更新后,快速更新与该数据集绑定的元素集合。例如:
上例中div已经绑定元素[4, 8, 15, 16, 23, 42],此时该数据集发生变化如下,需要重新绑定:
[/code]
这时,enter selection中的元素是为了与1 2 32 这些新数据绑定而产生的占位符结点,而exit selection中的元素则是已删除数据 15 23 42绑定的元素,对新数据我们需要生成新的元素与之绑定,而对于失去绑定数据的元素,则需要清理,如下:
2
[/code]
2
3
4
5
6
7
[/code]To be continued.
QuickStart
<html> <title></title> <script src="https://d3js.org/d3.v4.min.js"></script> <style > div.bar { display: inline-block; width: 20px; height: 75px; /* 后面会覆盖这个高度 */ background-color: teal; margin : 3px; } </style> <body> <script type="text/javascript"> var dataset = [ 25, 7, 5, 26, 11 ]; d3.select("body").selectAll("div")//元素选择 .data(dataset) // 数据绑定 .enter() // 返回占位对象 .append("div") //追加元素 .attr("class", "bar") //设置DOM属性 .style("height", function(d) { var barHeight = d * 5; return barHeight + "px"; }); //设置CSS属性 </script> </body> </html>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
[/code]
D3库的引用。开头使用
<script src = "">引用该可视化库,地址可以是官方服务器提供的版本,也可以把库下载下来,使用本地化路径。
元素选择。D3支持CSS3的选择器语法,可通过tag、class、id等属性对元素进行选择。
数据绑定。 D3的基础原理是将页面中的可视化元素,如段落,层(html元素),圆、路径、线段(SVG元素)与真实数据进行绑定,data方法就是用来绑定元素和数据,该方法会循环遍历数据集中的每一个数据。
enter 为每个待绑定的数据生成一个占位符对象(页面元素),后续可以操作这些对象生成具体的可视化元素。
append方法向一个Dom元素中添加对象,这里页面原本一个DIV层都没有,但是先取得占位符对象(包含对应数据),然后通过添加DIV层,并使用attr和style方法设置该DIV的高度,从而在最后的页面上显示出一幅条形图
常用函数
元素选择
d3.select(selector)
选中与指定选择器字符串匹配的第一个元素,返回单元素选择结果。如果当前文档中没有匹配的元素则返回空的选择。如果有多个元素被选中,只有第一个匹配的元素(在文档遍历次序中)被选中。d3.select(node)
选择并返回指定的节点。d3.selectAll(selector)
选中匹配指定选择器的所有的元素。这些元素会按照文档的遍历顺序(从上到下)选择。如果当前文档中没有匹配的元素则返回空的选择。d3.selectAll(nodes)
选择指定的元素数组。如果你已经有了一些元素的引用这将是很有用的,例如事件监听器中的d3.selectAll(this.childNodes),或者一个全局对象例如document.links。需要指出的是,d3.select和d3.selectAll将默认查询整个文档,但是selection.select和selection.selectAll则只在当前selection范围里查找
D3的函数使用也支持连缀用法,多个函数连用时,前一个函数的返回值将作为下一个函数的调用主体,例如
d3.select("body").selectAll("div"),首先选择了页面中的body元素,然后调用body.selectAll来选择body范围中的所有div元素。
元素修改
selection.attr(name[, value])
用来设置选中元素的属性并返回该selection。如果参数是name,value键值对形式,则会将selection中的元素的name属性的值设置成value,如果value是常数,那么seletion中所有的元素都将设置成相同的值,如果value是函数的形式,那么这个函数将会获得参数当前元素d和当前元素在集合中的索引作为参数,函数的返回值用来设置每个元素的name属性。如果是name的形式,则将返回该集合中元素中name属性的值。
function中可获得全部参数有:
being passed the current datum (d), the current index (i), and the current group (nodes), with this as the current DOM element.
selection.classed(names[, value])
用来设置选中元素的class属性并返回该selection。name指定想设置的class名称,value为true表示添加该class属性,为false表示不分配该class属性,用法与上面类似。但是如何删除已有的class属性?
selection.classed("foo", function() { return Math.random() > 0.5; }); selection.classed("foo bar", true);//同时添加foo和bar属性1
2
[/code]
selection.style(name[, value])
使用设置选中元素的css属性并返回该seletionp.style("width","90px") = <p style="width:90px">1
[/code]
selection.text([value])
设置选中元素的文字内容。如果value不为空,则用该value替代选中元素所有的子元素并返回该元素。value为空,则返回该selection中第一个文字内容非空的元素的text content
selection.append(type)
用来给选中的元素扩展子节点并返回更新后的selection,type可以是string也可以是function。If the specified type is a string, appends a new element of this type (tag name) as the last child of each selected element, or the next following sibling in the update selection if this is an enter selection.
例如,给段落新增div层,可以写成下面形式: d3.selectAll("p").append("div"); d3.selectAll("p").append(function() { return document.createElement("div"); }); d3.selectAll("p").select(function() { return this.appendChild(document.createElement("div")); });1
2
3
4
5
6
7
8
9
10
[/code]
数据绑定
selection.data([data[, key]])
将指定的数据集data和选择的元素集selection进行绑定,并返回更新后的数据集(绑定的数据将存放在元素的_data_属性中),并同时在返回的selection基础上定义了enter selection和exit selection的范围
,enter和exit将在下面介绍。指定的data可是是一组任意的数据,数字或者对象。
绑定的顺序由函数key来决定,如果key为空,则绑定顺序为默认按照索引来,即第一个元素绑定到第一个数据,第二个元素绑定到第二个数据,可以通过指定key函数来设置不同的元素绑定顺序。
注意:selection中元素的个数和data数据集中个数并不总是一一对应,可能会出现元素个数较少或者数据集个数较少的情况,为了使元素和数据一一对应,便有了enter 和 exit方法。
selection.enter()
Returns the enter selection: placeholder nodes for each datum that had no corresponding DOM element in the selection为selection绑定的数据集中,尚未绑定dom元素的数据创建dom占位符结点。如果一个selection为空,但它绑定了一个数据集,那么enter方法将为这些数据集中的每一个数据创建一个占位符结点放到selection中,后续的dom操作可以使用该占位符结点。
例子:从数组中创建div层 js代码 var div = d3.select("body") .selectAll("div") .data([4, 8, 15, 16, 23, 42]) .enter().append("div") .text(function(d) { return d; });1
2
3
4
5
6
7
[/code]
html效果 <div>4</div> <div>8</div> <div>15</div> <div>16</div> <div>23</div> <div>42</div>1
2
3
4
5
6
7
[/code]
selection.exit()
Returns the exit selection: existing DOM elements in the selection for which no new datum was found选中元素集中存在一些dom元素,没有数据与之绑定,这个方法就将这些元素返回。
enter和exit结合使用,可以很方便的再数据集更新后,快速更新与该数据集绑定的元素集合。例如:
上例中div已经绑定元素[4, 8, 15, 16, 23, 42],此时该数据集发生变化如下,需要重新绑定:
div = div.data([1, 2, 4, 8, 16, 32], function(d) { return d; });1
[/code]
这时,enter selection中的元素是为了与1 2 32 这些新数据绑定而产生的占位符结点,而exit selection中的元素则是已删除数据 15 23 42绑定的元素,对新数据我们需要生成新的元素与之绑定,而对于失去绑定数据的元素,则需要清理,如下:
div.enter().append("div").text(function(d) { return d; }); div.exit().remove();1
2
[/code]
页面会变成如下所示: <div>1</div> <div>2</div> <div>4</div> <div>8</div> <div>16</div> <div>32</div>1
2
3
4
5
6
7
[/code]To be continued.
相关文章推荐
- 使用 D3.js 进行简单的数据可视化--折线图
- 使用D3.js进行数据可视化
- 使用wxPython的绘图模块wxPyPlot进行数据可视化
- 用D3.js进行医疗数据可视化 (一)折线图 (Line Chart)
- 使用R进行数据可视化套路之-直方图
- 使用bokeh-scala进行数据可视化
- 利用d3.js对QQ群大数据资料进行可视化分析
- Python进阶(四十)-数据可视化の使用matplotlib进行绘图
- 用D3.js进行医疗数据可视化 (二)图例 (Legend)
- 使用R进行数据可视化套路之-条形图、Cleveland点图、矩阵
- 使用`phy`进行神经网络数据读取及可视化之开发环境准备
- Python进阶(三十九)-数据可视化の使用matplotlib进行绘图分析数据
- 如何使用R进行数据展现?且看使用iris数据可视化实例
- 使用TensorBoard进行数据可视化
- 使用wxPython的绘图模块wxPyPlot进行数据可视化
- 使用R进行数据可视化套路之-多重散点图、连接Mysql获取数据
- 如何使用R进行数据展现?且看使用iris数据可视化实例
- 用D3.js进行医疗数据可视化 (四) 堆积区图 (Stacked Area Chart)
- 使用Python的netCDF4和matplotlib.basemap包进行气象数据的可视化
- 使用Echarts和Ajax动态加载数据进行大数据可视化