unity 程序生成mesh并实现2D山丘地形
2018-02-10 21:49
525 查看
首先看一下最终效果:
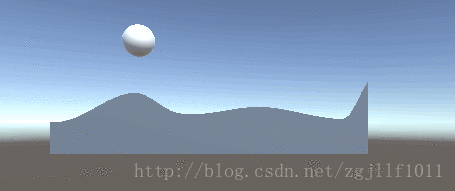
这里我假设读者已经了解并知道如何使用Mesh。如果不懂的话可以百度一下,有很多文章。
实现起来其实非常简单,思路:
每两个顶点为一组,这两个点的x坐标相同,一个在上一个在下,下边的y坐标固定为0,上边的y坐标从曲线编辑器中取值。
然后把生成的mesh赋予物体的MeshCollider。
下面给出完整代码:using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MeshMount : MonoBehaviour {
//每2个定点为一组,此值代表有多少组
const int count = 50;
//每两组顶点的间隔距离,此值越小曲线越平滑
const float pointdis = 0.2f;
Material mat;
MeshCollider mc;
//曲线
public AnimationCurve anim;
// Use this for initialization
void Start () {
mat = Resources.Load<Material>("Shader/MeshMount/Custom_meshshader");
mc = gameObject.AddComponent<MeshCollider>();
MeshRenderer mr = gameObject.AddComponent<MeshRenderer>();
mr.material = mat;
DrawSquare();
}
// Update is called once per frame
void Update () {
}
void DrawSquare()
{
//创建mesh
Mesh mesh = gameObject.AddComponent<MeshFilter>().mesh;
mesh.Clear();
//定义顶点列表
List<Vector3> pointList = new List<Vector3>();
//uv列表
List<Vector2> uvList = new List<Vector2>();
//第一列的前2个点直接初始化好
pointList.Add(new Vector3(0, 0, 0));
pointList.Add(new Vector3(0, 1, 0));
//设置前2个点的uv
uvList.Add(new Vector2(0, 0));
uvList.Add(new Vector2(0,1));
//三角形数组
List<int> triangleList = new List<int>();
for (int i = 0; i < count; i ++){
//计算当前列位于什么位置
float rate = (float)i / (float)count;
//计算当前的顶点
pointList.Add(new Vector3((i + 1)*pointdis, 0, 0));
//这里从曲线函数中取出当点的高度
pointList.Add(new Vector3((i + 1)*pointdis,anim.Evaluate(rate), 0));
//uv直接使用rate即可
uvList.Add(new Vector2(rate, 0));
uvList.Add(new Vector2(rate, 1));
//计算当前2个点与前面2个点组成的2个三角形
int startindex = i * 2;
triangleList.Add(startindex + 0);
triangleList.Add(startindex + 1);
triangleList.Add(startindex + 2);
triangleList.Add(startindex + 3);
triangleList.Add(startindex + 2);
triangleList.Add(startindex + 1);
}
//把最终的顶点和三角形数组赋予mesh;
mesh.vertices = pointList.ToArray();
mesh.triangles = triangleList.ToArray();
mesh.uv = uvList.ToArray();
mesh.RecalculateNormals();
//把mesh赋予MeshCollider
mc.sharedMesh = mesh;
}
}
使用方法:
首先创建一个空物体,把脚本挂到此物体上,然后在Inspector面板中双击anmi,编辑出一个喜欢的曲线形状。注意横轴只有在[0,1]的范围内才有效。
接下来创建一个球体并赋予rigidbode。
最后运行场景就能看到文章最开始的效果了。
这里我假设读者已经了解并知道如何使用Mesh。如果不懂的话可以百度一下,有很多文章。
实现起来其实非常简单,思路:
每两个顶点为一组,这两个点的x坐标相同,一个在上一个在下,下边的y坐标固定为0,上边的y坐标从曲线编辑器中取值。
然后把生成的mesh赋予物体的MeshCollider。
下面给出完整代码:using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MeshMount : MonoBehaviour {
//每2个定点为一组,此值代表有多少组
const int count = 50;
//每两组顶点的间隔距离,此值越小曲线越平滑
const float pointdis = 0.2f;
Material mat;
MeshCollider mc;
//曲线
public AnimationCurve anim;
// Use this for initialization
void Start () {
mat = Resources.Load<Material>("Shader/MeshMount/Custom_meshshader");
mc = gameObject.AddComponent<MeshCollider>();
MeshRenderer mr = gameObject.AddComponent<MeshRenderer>();
mr.material = mat;
DrawSquare();
}
// Update is called once per frame
void Update () {
}
void DrawSquare()
{
//创建mesh
Mesh mesh = gameObject.AddComponent<MeshFilter>().mesh;
mesh.Clear();
//定义顶点列表
List<Vector3> pointList = new List<Vector3>();
//uv列表
List<Vector2> uvList = new List<Vector2>();
//第一列的前2个点直接初始化好
pointList.Add(new Vector3(0, 0, 0));
pointList.Add(new Vector3(0, 1, 0));
//设置前2个点的uv
uvList.Add(new Vector2(0, 0));
uvList.Add(new Vector2(0,1));
//三角形数组
List<int> triangleList = new List<int>();
for (int i = 0; i < count; i ++){
//计算当前列位于什么位置
float rate = (float)i / (float)count;
//计算当前的顶点
pointList.Add(new Vector3((i + 1)*pointdis, 0, 0));
//这里从曲线函数中取出当点的高度
pointList.Add(new Vector3((i + 1)*pointdis,anim.Evaluate(rate), 0));
//uv直接使用rate即可
uvList.Add(new Vector2(rate, 0));
uvList.Add(new Vector2(rate, 1));
//计算当前2个点与前面2个点组成的2个三角形
int startindex = i * 2;
triangleList.Add(startindex + 0);
triangleList.Add(startindex + 1);
triangleList.Add(startindex + 2);
triangleList.Add(startindex + 3);
triangleList.Add(startindex + 2);
triangleList.Add(startindex + 1);
}
//把最终的顶点和三角形数组赋予mesh;
mesh.vertices = pointList.ToArray();
mesh.triangles = triangleList.ToArray();
mesh.uv = uvList.ToArray();
mesh.RecalculateNormals();
//把mesh赋予MeshCollider
mc.sharedMesh = mesh;
}
}
使用方法:
首先创建一个空物体,把脚本挂到此物体上,然后在Inspector面板中双击anmi,编辑出一个喜欢的曲线形状。注意横轴只有在[0,1]的范围内才有效。
接下来创建一个球体并赋予rigidbode。
最后运行场景就能看到文章最开始的效果了。
相关文章推荐
- SilverLight:使用MVVM实现View层在程序运行时自动生成控件并且取得其值
- Unity 2D游戏开发教程之使用脚本实现游戏逻辑
- Unity2D - 6. 生成随机地图 (2) - 脚本控制Tilemap (1) 自动切换不同的sprite
- Unity2D - 6. 生成随机地图 (2) - 脚本控制Tilemap (2) 加载已有房间地图
- 初级3D地形随机生成算法源码(Ogre实现)放出
- ASP.NET程序中实现校验码图像生成
- 实现安卓程序退出后重进自己程序的一个小功能(android,unity)
- 生成画圆程序的实现步骤
- 01:用java实现四则运算题目生成程序
- cocos2dx实现自定义2D地形
- php程序中实现验证码的生成和使用
- unity生成的WP8.1工程的Title本地化实现
- Unity 程序去边框并实现拖拽窗口
- PHP做视频网站,让程序自动实现视频格式转换、设置视频大小、生成视频缩略图
- unity 使用程序生成Texture2D并创建Sprite,制作渐变背景的效果
- php动态网页实现页面静态化 通过在初次被访问时生成html文件保存起来,下次该PHP程序被访问时就直接找到以前被访问过的html页面
- java 二维码生成与解析代码实现,程序源码下载可用
- Unity2D - 4. 实现android虚拟摇杆控制人物移动
- Python脚本生成的exe文件自动升级程序实现方法