【再回首Python之美】【模块 math】math模块的基本使用
2018-02-08 22:50
519 查看
学好数理化,走遍天下都不怕,所以一定要把Python的math模块学好。
math简介
math提供两个数学常量和众多数学函数。这俩常量和众多数学函数都是哪些呢?通过dir(math)一看便知。
使用math模块之前,一定要把模块包括进来,告诉编译器我要开始使用math提供的变量和方法了。import mathmath的两个常量print u"圆周率 math.pi\t= ",math.pi #3.14159265359
print u"自然常数 math.e\t= ",math.e #2.71828182846math的众多函数>>> dir(math)
['__doc__', '__name__', '__package__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos', 'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'hypot', 'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'modf', 'pi', 'pow', 'radians', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'trunc']
>>> math官方手册地址
点击进入下面官方math手册页面,里面包含所有的math 属性,工作当中用到时可以查询使用,很是方便。
https://docs.python.org/2/library/math.html
示例代码#coding:utf-8
#ex_module_math.py
self_file = __file__ #save current file absulote path
import math
print "\n======dump math type======"
print type(math) #<type 'module'>
print "\n======dump math attr list======"
print dir(math)
#['__doc__', '__name__', '__package__', 'acos', 'acosh', 'asin',
#'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos',
#'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs',
#'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'hypot',
#'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p',
#'modf', 'pi', 'pow', 'radians', 'sin', 'sinh', 'sqrt', 'tan',
#'tanh', 'trunc']
print "\n======dump math attr dict======"
print math.__dict__
#{'pow': <built-in function pow>,……,'gamma': <built-in function gamma>}
print "\n===normal introduce to function==="
print u"向上取整 math.ceil(1.001) = ",math.ceil(1.001) #2.0
print u"向上取整 math.ceil(1.999) = ",math.ceil(1.999) #2.0
print u"向下取整 math.floor(1.001) = ",math.floor(1.001) #1.0
print u"向下取整 math.floor(1.999) = ",math.floor(1.999) #1.0
print u"指数运算 math.pow(3,2) = ",math.pow(3,2) #9.0
print u"指数运算 math.pow(4,2) = ",math.pow(4,2) #16.0
print u"指数运算 math.pow(5,2) = ",math.pow(5,2) #25.0
e = math.e
print u"基底为e的对数运算 math.log(e) = ",math.log(e) #1.0
print u"基底为e的对数运算 math.log(e * e) = ",math.log(e * e) #2.0
print u"基底为2的对数运算 math.log(4,2) = ",math.log(4,2) #2.0
print u"基底为2的对数运算 math.log(8,2) = ",math.log(8,2) #3.0
print u"平方根 math.sqrt(4) = ",math.sqrt(4) #2.0
print u"平方根 math.sqrt(9) = ",math.sqrt(9) #3.0
print u"平方根 math.sqrt(16) = ",math.sqrt(16) #4.0
print u"绝对值 math.fabs(+1) = ",math.fabs(+1) #1.0
print u"绝对值 math.fabs(-1) = ",math.fabs(-1) #1.0
print u"绝对值 math.fabs(+2) = ",math.fabs(+2) #2.0
print u"绝对值 math.fabs(-2) = ",math.fabs(-2) #2.0
print u"\n======Angular conversion角度转换======"
#常识 0度(deg) == 0弧度(rad)
#常识 90度(deg) == 1.5707964弧度(rad)
#常识 180度(deg) == 3.1415927弧度(rad)
def deg2rad(deg): #角度转弧度
return math.radians(deg)
def rad2deg(rad): #弧度转角度
return math.degrees(rad)
print "deg2rad(0)\t=",deg2rad(0) #0.0
print "deg2rad(90)\t=",deg2rad(90) #1.57079632679
print "deg2rad(180)\t=",deg2rad(180) #3.14159265359
print u"\n======三角函数======"
#三角函数入参为弧度rad,计算角度的时候,需要先将角度转弧度后计算
print "math.sin(deg2rad(0))\t= ",math.sin(deg2rad(0)) #0.0
print "math.sin(deg2rad(30))\t= ",math.sin(deg2rad(30)) #0.5
print "math.sin(deg2rad(90))\t= ",math.sin(deg2rad(90)) #1.0
print "math.cos(deg2rad(0))\t= ",math.cos(deg2rad(0)) #1.0
print "math.cos(deg2rad(60))\t= ",math.cos(deg2rad(60)) #0.5
print "math.cos(deg2rad(180))\t= ",math.cos(deg2rad(180))#-1.0
print "math.tan(deg2rad(0))\t= ",math.tan(deg2rad(0)) #0.0
print "math.asin(deg2rad(0))\t= ",math.asin(deg2rad(0)) #0.0
print "math.acos(deg2rad(0))\t= ",math.acos(deg2rad(0)) #1.57079632679
print "math.atan(deg2rad(0))\t= ",math.atan(deg2rad(0)) #0.0
print "\n======math's const variable========"
print u"圆周率 math.pi\t= ",math.pi #3.14159265359
print u"自然常数 math.e\t= ",math.e #2.71828182846
print "\nexit %s" % self_file
编译执行
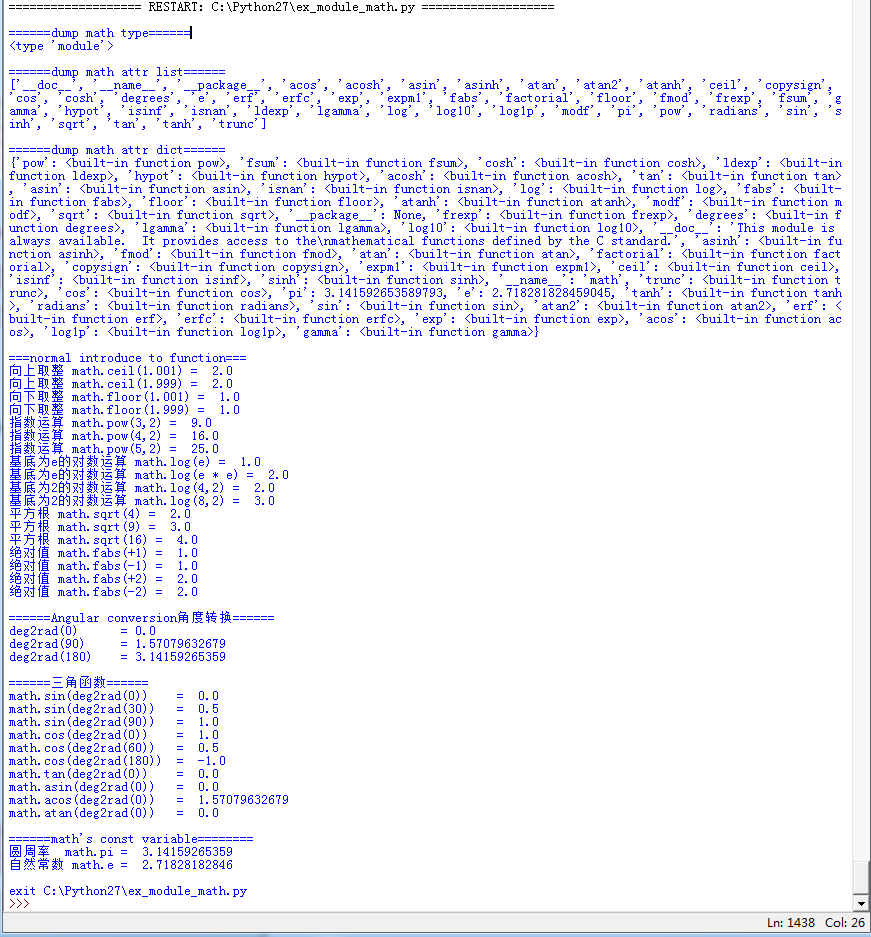
(end)
math简介
math提供两个数学常量和众多数学函数。这俩常量和众多数学函数都是哪些呢?通过dir(math)一看便知。
使用math模块之前,一定要把模块包括进来,告诉编译器我要开始使用math提供的变量和方法了。import mathmath的两个常量print u"圆周率 math.pi\t= ",math.pi #3.14159265359
print u"自然常数 math.e\t= ",math.e #2.71828182846math的众多函数>>> dir(math)
['__doc__', '__name__', '__package__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos', 'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'hypot', 'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'modf', 'pi', 'pow', 'radians', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'trunc']
>>> math官方手册地址
点击进入下面官方math手册页面,里面包含所有的math 属性,工作当中用到时可以查询使用,很是方便。
https://docs.python.org/2/library/math.html
示例代码#coding:utf-8
#ex_module_math.py
self_file = __file__ #save current file absulote path
import math
print "\n======dump math type======"
print type(math) #<type 'module'>
print "\n======dump math attr list======"
print dir(math)
#['__doc__', '__name__', '__package__', 'acos', 'acosh', 'asin',
#'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos',
#'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs',
#'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'hypot',
#'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p',
#'modf', 'pi', 'pow', 'radians', 'sin', 'sinh', 'sqrt', 'tan',
#'tanh', 'trunc']
print "\n======dump math attr dict======"
print math.__dict__
#{'pow': <built-in function pow>,……,'gamma': <built-in function gamma>}
print "\n===normal introduce to function==="
print u"向上取整 math.ceil(1.001) = ",math.ceil(1.001) #2.0
print u"向上取整 math.ceil(1.999) = ",math.ceil(1.999) #2.0
print u"向下取整 math.floor(1.001) = ",math.floor(1.001) #1.0
print u"向下取整 math.floor(1.999) = ",math.floor(1.999) #1.0
print u"指数运算 math.pow(3,2) = ",math.pow(3,2) #9.0
print u"指数运算 math.pow(4,2) = ",math.pow(4,2) #16.0
print u"指数运算 math.pow(5,2) = ",math.pow(5,2) #25.0
e = math.e
print u"基底为e的对数运算 math.log(e) = ",math.log(e) #1.0
print u"基底为e的对数运算 math.log(e * e) = ",math.log(e * e) #2.0
print u"基底为2的对数运算 math.log(4,2) = ",math.log(4,2) #2.0
print u"基底为2的对数运算 math.log(8,2) = ",math.log(8,2) #3.0
print u"平方根 math.sqrt(4) = ",math.sqrt(4) #2.0
print u"平方根 math.sqrt(9) = ",math.sqrt(9) #3.0
print u"平方根 math.sqrt(16) = ",math.sqrt(16) #4.0
print u"绝对值 math.fabs(+1) = ",math.fabs(+1) #1.0
print u"绝对值 math.fabs(-1) = ",math.fabs(-1) #1.0
print u"绝对值 math.fabs(+2) = ",math.fabs(+2) #2.0
print u"绝对值 math.fabs(-2) = ",math.fabs(-2) #2.0
print u"\n======Angular conversion角度转换======"
#常识 0度(deg) == 0弧度(rad)
#常识 90度(deg) == 1.5707964弧度(rad)
#常识 180度(deg) == 3.1415927弧度(rad)
def deg2rad(deg): #角度转弧度
return math.radians(deg)
def rad2deg(rad): #弧度转角度
return math.degrees(rad)
print "deg2rad(0)\t=",deg2rad(0) #0.0
print "deg2rad(90)\t=",deg2rad(90) #1.57079632679
print "deg2rad(180)\t=",deg2rad(180) #3.14159265359
print u"\n======三角函数======"
#三角函数入参为弧度rad,计算角度的时候,需要先将角度转弧度后计算
print "math.sin(deg2rad(0))\t= ",math.sin(deg2rad(0)) #0.0
print "math.sin(deg2rad(30))\t= ",math.sin(deg2rad(30)) #0.5
print "math.sin(deg2rad(90))\t= ",math.sin(deg2rad(90)) #1.0
print "math.cos(deg2rad(0))\t= ",math.cos(deg2rad(0)) #1.0
print "math.cos(deg2rad(60))\t= ",math.cos(deg2rad(60)) #0.5
print "math.cos(deg2rad(180))\t= ",math.cos(deg2rad(180))#-1.0
print "math.tan(deg2rad(0))\t= ",math.tan(deg2rad(0)) #0.0
print "math.asin(deg2rad(0))\t= ",math.asin(deg2rad(0)) #0.0
print "math.acos(deg2rad(0))\t= ",math.acos(deg2rad(0)) #1.57079632679
print "math.atan(deg2rad(0))\t= ",math.atan(deg2rad(0)) #0.0
print "\n======math's const variable========"
print u"圆周率 math.pi\t= ",math.pi #3.14159265359
print u"自然常数 math.e\t= ",math.e #2.71828182846
print "\nexit %s" % self_file
编译执行
(end)
相关文章推荐
- 【再回首Python之美】【模块-random】random模块的基本使用
- python数据持久存储:pickle模块的基本使用
- python数据持久存储:pickle模块的基本使用
- python数据持久存储:pickle模块的基本使用
- MySQL-python模块的基本使用
- python tarfile模块基本使用
- python数据持久存储:pickle模块的基本使用
- 使用Python编写爬虫的基本模块及框架使用指南
- python ORM 模块peewee(二): 数据库使用的基本流程
- Python中atexit模块的基本使用示例
- python数据持久存储:pickle模块的基本使用
- python数据持久存储:pickle模块的基本使用
- 使用Python编写爬虫的基本模块及框架使用指南
- 【window10下python的对elcel表格操作xlrd和xlwt模块的下载与安装及基本的使用】
- python数据持久存储:pickle模块的基本使用
- python数据持久存储:pickle模块的基本使用
- Python程序中用csv模块来操作csv文件的基本使用教程
- python数据持久存储:pickle模块的基本使用
- Python下rrdtool模块的基本使用方法
- python数据持久存储:pickle模块的基本使用