【再回首Python之美】【异常处理】try-except
2018-02-07 11:20
651 查看
使用方法直接跳看:推荐使用的异常处理流程;推荐的内建函数的封装函数
异常处理必要性
为了保证程序的健壮性,将可能出现异常退出的代码用try……except来处理
捕获异常的各种方法
1.捕获所有异常print "\r\n=======try-except========="
try:
open('unexistFile')
except:
print "failed to open." 2.捕获特殊异常,如ctrl+c等
KeyboardInterrupt和SystemExit,是与Exception平级的异常。
print '\r\n======try-except (KeyboardInterupt, SystemExit)======'
try:
while 1:
pass
except (KeyboardInterrupt, SystemExit):#ctrl+c等导致app退出异常
print 'app will exit, handle app exitence.' 3.捕获具体异常,1/0等print "\r\n======try-except ZeroDivisionError,e======="
try:
1/0 #除数为零
except ZeroDivisionError, e:
print 'ZeroDivisionError:',e 4.捕获多个具体的异常#try-except (E1, E2,……En)[,e]:捕获多个异常
print '\r\n======try-except (E1, E2,……En)[,e]======'
try:
1/0
except (NameError,ZeroDivisionError),e:
print repr(e) 5.待追加
简单示例代码#ex_except.py
def dumpFile_withExcept(path):
try:
f = file(path)
print 'Succeed open file'
f.close()
except:
print 'Failed open file, file not exists.'
return False
return True
def dumpFile_nonExcept(path):
f = file(path)
f.close()
#test
dumpFile_withExcept('non-exist.txt')
dumpFile_nonExcept('non-exist.txt')编译执行
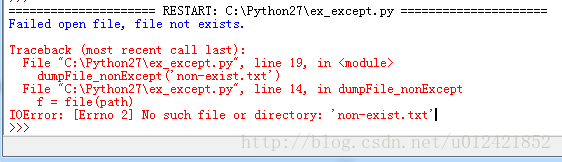
可知
有了try……excepty异常捕捉处理的dumpFile_withExcept函数打开不存在文件时,try抛出异常,然后由except捕获并执行except代码块。
没有异常捕捉处理的dumpFile_nonExcept函数打开不存在文件时,由程序直接退出并给出IOError等信息。
示例代码(追加)#ex_try-except.py
self_file = __file__
#try-except,捕获所有异常
print "\r\n=======try-except========="
try:
open('unexistFile')
except:
print "failed to open."
#try-except + trceback,打印异常堆栈以及错误信息
print "\r\n=======traceback + try-except========="
import traceback #use traceback module
try:
open('unexistFile')
except:
print 'traceback.print_exc():',traceback.print_exc()
print 'traceback.format_exc():\n%s' % traceback.format_exc()
#try-except Exception,e,捕获所有异常
print '\r\n======try-except Exception,e======'
try:
open('unexistFile')
except Exception,e:
print 'ErrorInfo:',e
print 'ErrorInfo:',e.message
print 'ErrorInfo:',str(e)
print "ErrorInfo:",repr(e)
#try-except Exception,(errCode, errMsg),捕获所有异常以及具体错误码
print '\r\n======try-except Exception,(errCode, errMsg)======'
import errno #use errno module
try:
open('unexistFile')
except Exception,(errCode,errMsg):
if errCode == errno.ENOENT:
print 'failed to open, no such file.'
elif errCode == errno.EPERM:
print 'failed to open, permission denied'
else:
print errMsg
#try-except ErrorType, e,捕获具体异常
print '\r\n======try-except (KeyboardInterupt, SystemExit)======'
try:
while 1:
pass
except (KeyboardInterrupt, SystemExit):#ctrl+c等导致app退出异常
print 'app will exit, handle app exitence.'
print "\r\n======try-except NameError,e======="
try:
print unexistVal #访问一个未申明的变量
except NameError, e:
print repr(e)
print "\r\n======try-except ZeroDivisionError,e======="
try:
1/0 #除数为零
except ZeroDivisionError, e:
print 'ZeroDivisionError:',e
print "\r\n======try-except IndexError,e======="
try:
lst = []
print lst[99] #请求的索引超出序列范围
except IndexError,e:
print 'IndexError:',e.message
print "\r\n======try-except KeyError,e======="
try:
adct = {'Tom':25, 'John':18, 'Ann':20}
print adct['Kitty'] #请求一个不存在的字典关键字
except KeyError,e:
print 'KeyError:',e
print "\r\n======try-except IOError,e======="
try:
f = open('unexistFile') #打开一个不存在的文件或目录
except IOError,e:
print 'IOError:',e
print "\r\n======try-except AttributeError,e======="
try:
class Car(object):
pass
car = Car()
print car.color #访问未知的对象属性
except AttributeError, e:
print 'AttributeError:',e
#try-except-……-except,捕获具体异常并具体处理
print '\r\n=====try-except-……-except=========='
try:
1/0
except NameError, e:
print 'NameError:',e
except ZeroDivisionError, e: #由此except抓获1/0抛出的异常
print 'ZeroDivisionError:',e
except IndexError,e:
print 'IndexError:',e
except KeyError,e:
print 'KeyError:',e
except IOError,e:
print 'IOError:',e
except AttributeError, e:
print 'AttributeError:',e
except:#默认except必须作为最后一个except
print 'Unknown Error:',errno
#(start)推荐使用的异常处理流程
print '\r\n=====try-except======'
import errno #use errno module
import traceback #use traceback module
def handleException(errCode, errMsg):
if errCode == errno.ENOENT:
print 'failed to open, no such file.'
elif errCode == errno.EPERM:
print 'failed to open, permission denied'
else:
print errMsg
try:
open('unexistFile')
except Exception,(errCode,errMsg):
print traceback.format_exc()
handleException(errCode, errMsg)
#(end)推荐使用的异常处理流程
#(start)推荐的内建函数的封装函数
print '\r\n======define safe function====='
import errno #use errno module
import traceback #use traceback module
def safe_open(file_path, mode):
try:
ret = open(file_path, mode)
except Exception,(errCode, errMsg):
print traceback.format_exc()
if errCode == errno.ENOENT:
ret = 'ErrorInfo:failed to open, no such file.'
elif errCode == errno.EPERM:
ret = 'ErrorInfo:failed to open, permission denied.'
else:
ret = errMsg
return ret
f = safe_open('unexistFile', 'r')
#(end)推荐的内建函数的封装函数
print '\r\nexit %s' % self_file执行结果:>>>
=================== RESTART: C:\Python27\ex_try-except.py ===================
=======try-except=========
failed to open.
=======traceback + try-except=========
traceback.print_exc():Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 16, in <module>
open('unexistFile')
IOError: [Errno 2] No such file or directory: 'unexistFile'
None
traceback.format_exc():
Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 16, in <module>
open('unexistFile')
IOError: [Errno 2] No such file or directory: 'unexistFile'
======try-except Exception,e======
ErrorInfo: [Errno 2] No such file or directory: 'unexistFile'
ErrorInfo:
ErrorInfo: [Errno 2] No such file or directory: 'unexistFile'
ErrorInfo: IOError(2, 'No such file or directory')
======try-except Exception,(errCode, errMsg)======
failed to open, no such file.
======try-except (KeyboardInterupt, SystemExit)======
app will exit, handle app exitence.
======try-except NameError,e=======
NameError("name 'unexistVal' is not defined",)
======try-except ZeroDivisionError,e=======
ZeroDivisionError: integer division or modulo by zero
======try-except IndexError,e=======
IndexError: list index out of range
======try-except KeyError,e=======
KeyError: 'Kitty'
======try-except IOError,e=======
IOError: [Errno 2] No such file or directory: 'unexistFile'
======try-except AttributeError,e=======
AttributeError: 'Car' object has no attribute 'color'
=====try-except-……-except==========
ZeroDivisionError: integer division or modulo by zero
=====try-except======
Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 132, in <module>
open('unexistFile')
IOError: [Errno 2] No such file or directory: 'unexistFile'
failed to open, no such file.
======define safe function=====
Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 146, in safe_open
ret = open(file_path, mode)
IOError: [Errno 2] No such file or directory: 'unexistFile'
exit C:\Python27\ex_try-except.py
>>>
(end)
异常处理必要性
为了保证程序的健壮性,将可能出现异常退出的代码用try……except来处理
捕获异常的各种方法
1.捕获所有异常print "\r\n=======try-except========="
try:
open('unexistFile')
except:
print "failed to open." 2.捕获特殊异常,如ctrl+c等
KeyboardInterrupt和SystemExit,是与Exception平级的异常。
print '\r\n======try-except (KeyboardInterupt, SystemExit)======'
try:
while 1:
pass
except (KeyboardInterrupt, SystemExit):#ctrl+c等导致app退出异常
print 'app will exit, handle app exitence.' 3.捕获具体异常,1/0等print "\r\n======try-except ZeroDivisionError,e======="
try:
1/0 #除数为零
except ZeroDivisionError, e:
print 'ZeroDivisionError:',e 4.捕获多个具体的异常#try-except (E1, E2,……En)[,e]:捕获多个异常
print '\r\n======try-except (E1, E2,……En)[,e]======'
try:
1/0
except (NameError,ZeroDivisionError),e:
print repr(e) 5.待追加
简单示例代码#ex_except.py
def dumpFile_withExcept(path):
try:
f = file(path)
print 'Succeed open file'
f.close()
except:
print 'Failed open file, file not exists.'
return False
return True
def dumpFile_nonExcept(path):
f = file(path)
f.close()
#test
dumpFile_withExcept('non-exist.txt')
dumpFile_nonExcept('non-exist.txt')编译执行
可知
有了try……excepty异常捕捉处理的dumpFile_withExcept函数打开不存在文件时,try抛出异常,然后由except捕获并执行except代码块。
没有异常捕捉处理的dumpFile_nonExcept函数打开不存在文件时,由程序直接退出并给出IOError等信息。
示例代码(追加)#ex_try-except.py
self_file = __file__
#try-except,捕获所有异常
print "\r\n=======try-except========="
try:
open('unexistFile')
except:
print "failed to open."
#try-except + trceback,打印异常堆栈以及错误信息
print "\r\n=======traceback + try-except========="
import traceback #use traceback module
try:
open('unexistFile')
except:
print 'traceback.print_exc():',traceback.print_exc()
print 'traceback.format_exc():\n%s' % traceback.format_exc()
#try-except Exception,e,捕获所有异常
print '\r\n======try-except Exception,e======'
try:
open('unexistFile')
except Exception,e:
print 'ErrorInfo:',e
print 'ErrorInfo:',e.message
print 'ErrorInfo:',str(e)
print "ErrorInfo:",repr(e)
#try-except Exception,(errCode, errMsg),捕获所有异常以及具体错误码
print '\r\n======try-except Exception,(errCode, errMsg)======'
import errno #use errno module
try:
open('unexistFile')
except Exception,(errCode,errMsg):
if errCode == errno.ENOENT:
print 'failed to open, no such file.'
elif errCode == errno.EPERM:
print 'failed to open, permission denied'
else:
print errMsg
#try-except ErrorType, e,捕获具体异常
print '\r\n======try-except (KeyboardInterupt, SystemExit)======'
try:
while 1:
pass
except (KeyboardInterrupt, SystemExit):#ctrl+c等导致app退出异常
print 'app will exit, handle app exitence.'
print "\r\n======try-except NameError,e======="
try:
print unexistVal #访问一个未申明的变量
except NameError, e:
print repr(e)
print "\r\n======try-except ZeroDivisionError,e======="
try:
1/0 #除数为零
except ZeroDivisionError, e:
print 'ZeroDivisionError:',e
print "\r\n======try-except IndexError,e======="
try:
lst = []
print lst[99] #请求的索引超出序列范围
except IndexError,e:
print 'IndexError:',e.message
print "\r\n======try-except KeyError,e======="
try:
adct = {'Tom':25, 'John':18, 'Ann':20}
print adct['Kitty'] #请求一个不存在的字典关键字
except KeyError,e:
print 'KeyError:',e
print "\r\n======try-except IOError,e======="
try:
f = open('unexistFile') #打开一个不存在的文件或目录
except IOError,e:
print 'IOError:',e
print "\r\n======try-except AttributeError,e======="
try:
class Car(object):
pass
car = Car()
print car.color #访问未知的对象属性
except AttributeError, e:
print 'AttributeError:',e
#try-except-……-except,捕获具体异常并具体处理
print '\r\n=====try-except-……-except=========='
try:
1/0
except NameError, e:
print 'NameError:',e
except ZeroDivisionError, e: #由此except抓获1/0抛出的异常
print 'ZeroDivisionError:',e
except IndexError,e:
print 'IndexError:',e
except KeyError,e:
print 'KeyError:',e
except IOError,e:
print 'IOError:',e
except AttributeError, e:
print 'AttributeError:',e
except:#默认except必须作为最后一个except
print 'Unknown Error:',errno
#(start)推荐使用的异常处理流程
print '\r\n=====try-except======'
import errno #use errno module
import traceback #use traceback module
def handleException(errCode, errMsg):
if errCode == errno.ENOENT:
print 'failed to open, no such file.'
elif errCode == errno.EPERM:
print 'failed to open, permission denied'
else:
print errMsg
try:
open('unexistFile')
except Exception,(errCode,errMsg):
print traceback.format_exc()
handleException(errCode, errMsg)
#(end)推荐使用的异常处理流程
#(start)推荐的内建函数的封装函数
print '\r\n======define safe function====='
import errno #use errno module
import traceback #use traceback module
def safe_open(file_path, mode):
try:
ret = open(file_path, mode)
except Exception,(errCode, errMsg):
print traceback.format_exc()
if errCode == errno.ENOENT:
ret = 'ErrorInfo:failed to open, no such file.'
elif errCode == errno.EPERM:
ret = 'ErrorInfo:failed to open, permission denied.'
else:
ret = errMsg
return ret
f = safe_open('unexistFile', 'r')
#(end)推荐的内建函数的封装函数
print '\r\nexit %s' % self_file执行结果:>>>
=================== RESTART: C:\Python27\ex_try-except.py ===================
=======try-except=========
failed to open.
=======traceback + try-except=========
traceback.print_exc():Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 16, in <module>
open('unexistFile')
IOError: [Errno 2] No such file or directory: 'unexistFile'
None
traceback.format_exc():
Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 16, in <module>
open('unexistFile')
IOError: [Errno 2] No such file or directory: 'unexistFile'
======try-except Exception,e======
ErrorInfo: [Errno 2] No such file or directory: 'unexistFile'
ErrorInfo:
ErrorInfo: [Errno 2] No such file or directory: 'unexistFile'
ErrorInfo: IOError(2, 'No such file or directory')
======try-except Exception,(errCode, errMsg)======
failed to open, no such file.
======try-except (KeyboardInterupt, SystemExit)======
app will exit, handle app exitence.
======try-except NameError,e=======
NameError("name 'unexistVal' is not defined",)
======try-except ZeroDivisionError,e=======
ZeroDivisionError: integer division or modulo by zero
======try-except IndexError,e=======
IndexError: list index out of range
======try-except KeyError,e=======
KeyError: 'Kitty'
======try-except IOError,e=======
IOError: [Errno 2] No such file or directory: 'unexistFile'
======try-except AttributeError,e=======
AttributeError: 'Car' object has no attribute 'color'
=====try-except-……-except==========
ZeroDivisionError: integer division or modulo by zero
=====try-except======
Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 132, in <module>
open('unexistFile')
IOError: [Errno 2] No such file or directory: 'unexistFile'
failed to open, no such file.
======define safe function=====
Traceback (most recent call last):
File "C:\Python27\ex_try-except.py", line 146, in safe_open
ret = open(file_path, mode)
IOError: [Errno 2] No such file or directory: 'unexistFile'
exit C:\Python27\ex_try-except.py
>>>
(end)
相关文章推荐
- Python异常处理try...except、raise
- python中的异常捕获处理机制(try...except...等语句)
- python异常处理try,except,else,finally,raise
- python try except, 异常处理
- Python异常处理try...except...finally raise assert
- (Python)异常处理try...except、raise
- python异常处理try...except
- python异常处理 try.....except
- python try...except....else...finally处理异常
- Python_异常处理try...except、raise
- (Python)异常处理try...except、raise
- python中的异常处理 try..except
- Python异常处理——try...except、主动引发异常(raise)、断言(assert)
- (Python)异常处理try...except、raise
- (Python)异常处理try...except、raise
- 【python学习笔记】Python异常处理raise、try...except、断言assert
- Python学习笔记之错误处理(关键词:错误处理、异常机制、try、except、else、finally、raise)
- 【】Python】异常处理try...except、raise
- Python异常处理try...except、raise
- Python3:异常处理,try...except