python实现图片操作
2018-01-28 15:34
549 查看
前段时间在知乎看到大神用Ruby做的下面这样一张图,觉得非常有意思,所以打算用python实现一下
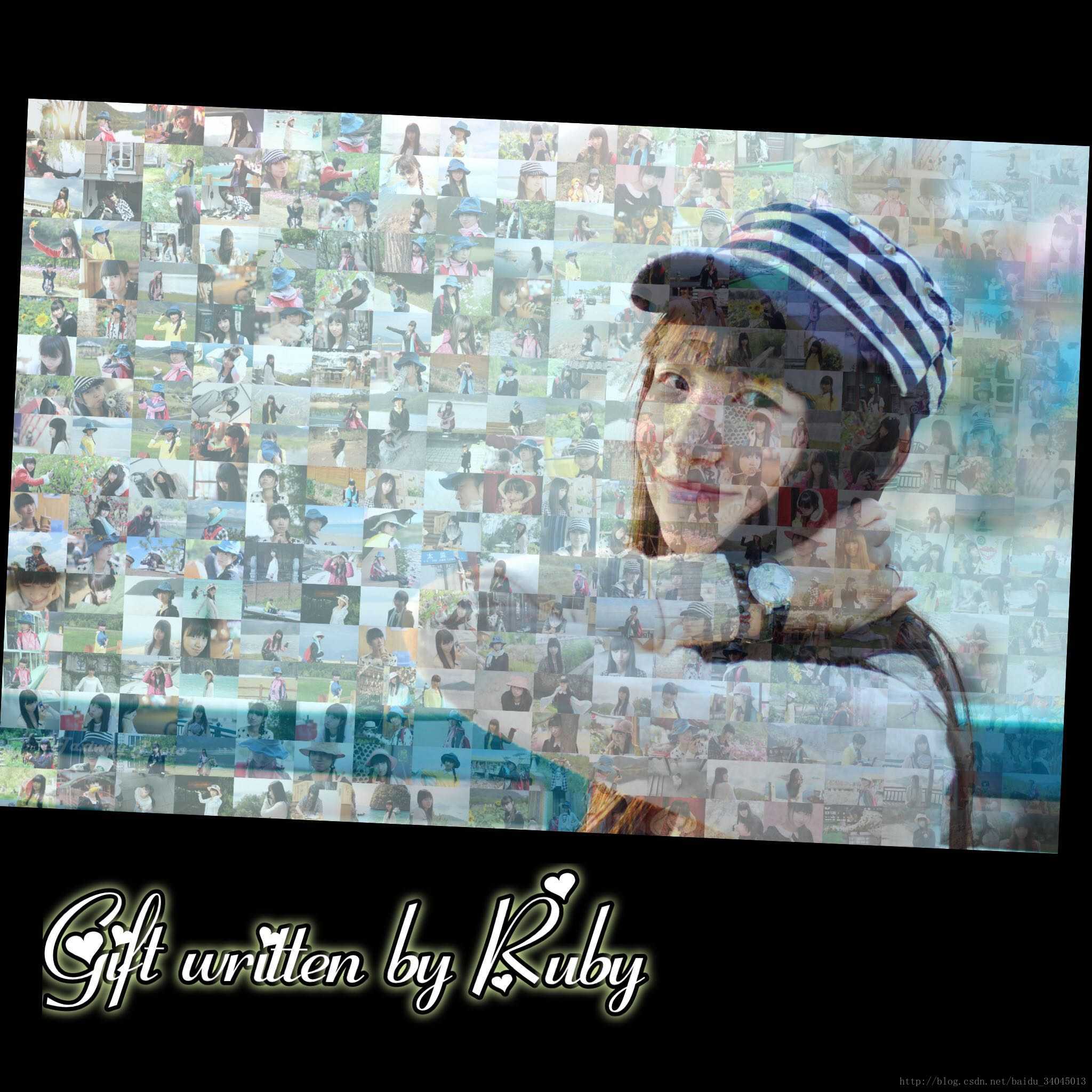
python有图像处理标准库PIL(Python Imaging Library),功能强大,简单易用,可惜只支持2.x,Pillow是在PIL基础上创建的兼容版本,支持python 3.x,同时增加了一些新特性。
打开图片
获取图片大小(返回一个元祖,分别为宽和高)
保存图片
显示图片
新建图像
裁剪图像
复制图像(产生原图像副本,对其操作不影响原图像)
粘贴(对调用的图像对象直接修改)
调整大小
旋转(逆时针,返回旋转后图像,原图像不变)
翻转
获取像素值
写像素值
获取alpha
写alpha
图像复合
1、将img2符合到img1上,返回Image图像(要求size相同,切mode都必须为RGBA)
2、out = img1*(1-alpha)+img2*alpha
3、
图像过滤
需要引入ImageFilter包
高斯模糊
普通模糊
边缘增强
找到边缘
浮雕
轮廓
锐化
平滑
细节
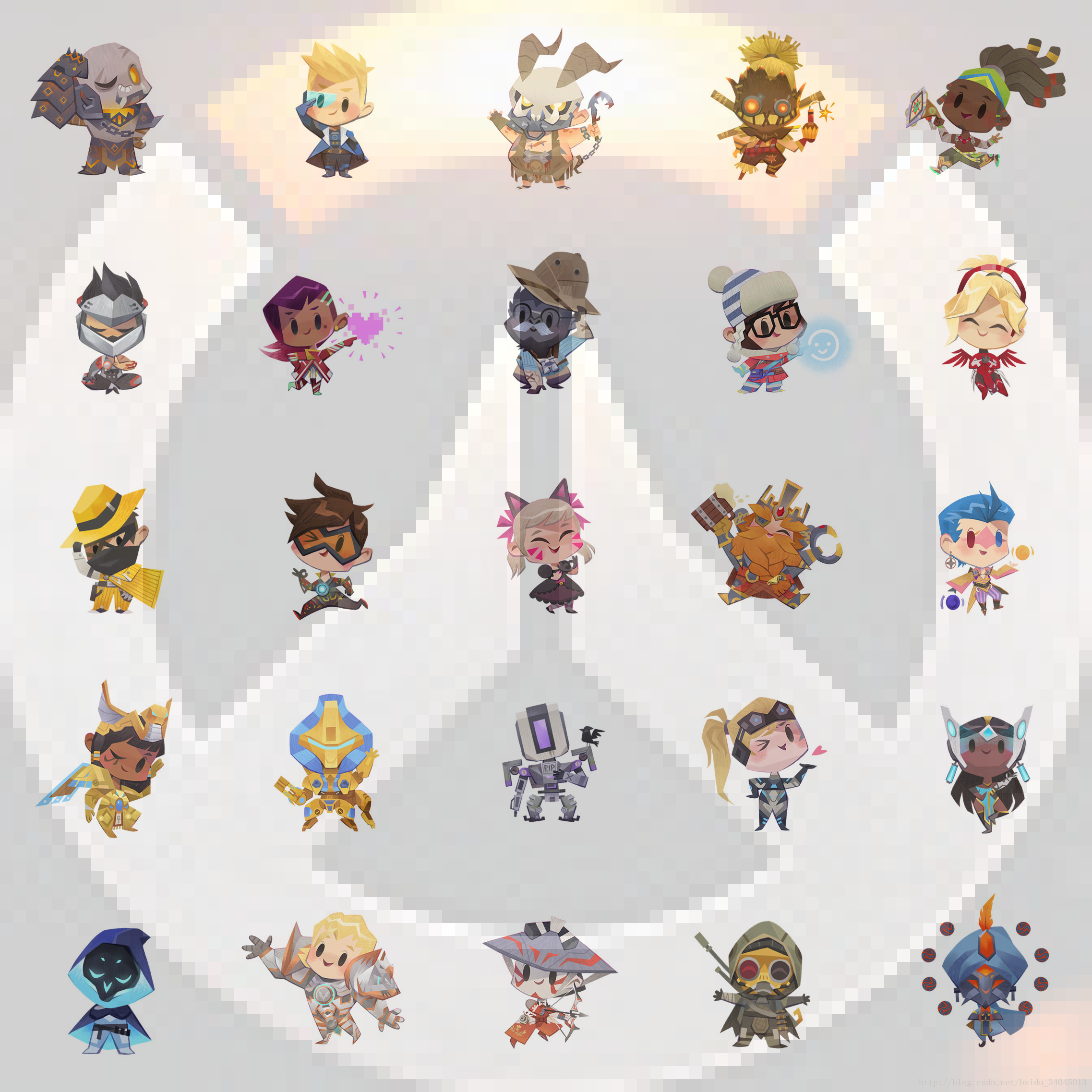
python有图像处理标准库PIL(Python Imaging Library),功能强大,简单易用,可惜只支持2.x,Pillow是在PIL基础上创建的兼容版本,支持python 3.x,同时增加了一些新特性。
安装Pillow
直接在命令行使用pip安装,十分方便pip install Pillow
操作图像
首先要根据需要引入对应的包(Image,ImageColor,ImageFont,ImageFilter等)from PIL import Image
打开图片
image = Image.open(url)
获取图片大小(返回一个元祖,分别为宽和高)
width, height = image.size
保存图片
image.save(url)
显示图片
image.show()
新建图像
newImage = Image.new(mode, (width, height), color)
裁剪图像
cropedImage = image.crop((0, 0, 100, 100))
复制图像(产生原图像副本,对其操作不影响原图像)
copyImage = image.copy()
粘贴(对调用的图像对象直接修改)
copyImage.paste(image, (x, y))
调整大小
resizedIm = image.resize((width, height))
旋转(逆时针,返回旋转后图像,原图像不变)
rotateIm = image.rotate(90)
翻转
image.transpose(Image.FLIP_TOP_LEFT_RIGTH) #水平翻转 image.transpose(Image.FLIP_TOP_TOP_BOTTOM) #垂直翻转
获取像素值
image.getpixel((x,y))
写像素值
image.putpixel((x,y),(r,g,b))
获取alpha
image.getalpha((x,y))
写alpha
image.putalpha((x,y), alpha)
图像复合
1、将img2符合到img1上,返回Image图像(要求size相同,切mode都必须为RGBA)
img3 = Image.alpha_composite(img1, img2)
2、out = img1*(1-alpha)+img2*alpha
img3 = Image.blend(img1, img2, alpha)
3、
img3 = Image.composite(img1, img2, mask)
图像过滤
需要引入ImageFilter包
from PIL import Image, ImageFilter
高斯模糊
im.filter(ImageFilter.GaussianBlur)
普通模糊
im.filter(ImageFilter.BLUR)
边缘增强
im.filter(ImageFilter.EDGE_ENHANCE)
找到边缘
im.filter(ImageFilter.FIND_EDGES)
浮雕
im.filter(ImageFilter.EMBOSS)
轮廓
im.filter(ImageFilter.CONTOUR)
锐化
im.filter(ImageFilter.SHARPEN)
平滑
im.filter(ImageFilter.SMOOTH)
细节
im.filter(ImageFilter.DETAIL)
Demo
# -*- coding: utf-8 -*- """ 将n*n张图片拼接在一起,并覆盖上一张降低透明度的图片 Copyright: 2018/01/27 by 邵明山 """ import os from PIL import Image def makedir(path): if not os.path.exists(path): os.mkdir(path) print("The directory \"" + path + "\" creates success.") else: print("The directory \"" + path + "\" has already existed.") base_path = "base" mask_path = "mask" makedir(base_path) makedir(mask_path) n = int(input("Please enter n, n^2 is the number of the pictures.")) print("Please put n^2 pictures to the forder \"base\".") print("Please put one picture to the forder \"mask\".") os.system("pause") width = int(input("Please enter the width of the final picture.")) height = int(input("Please enter the height of the final picture.")) transparency = int(input("Please enter the transparency% of the mask.")) base_pictures = [] for root, dirs, files in os.walk(os.getcwd()+"\\"+base_path): for file in files: base_pictures.append(os.path.join(root, file)) num = 0 base = Image.new("RGBA", (width, height)) for i in range(0, n): for j in range(0, n): picture = Image.open(base_pictures[num]) temp = picture.resize((int(width/n), int(height/n))) location = (int(i * width / n), int(j * height / n)) base.paste(temp, location) num += 1 if num >= len(base_pictures): break if num >= n*n: break for root, files in os.walk(os.getcwd()+"\\"+mask_path): for file in files: mask_picture = Image.open(mask_path + "\\" + file) mask = mask_picture.resize((width, height)) mask.putalpha(int(transparency/100 * 255)) result = Image.alpha_composite(base, mask) result.save("result.png")
运行结果
源代码
https://github.com/Caesar233/PhotoMixer相关文章推荐
- python3操作微信itchat实现发送图片
- 鼠标右键弹出菜单 上传图片自定义控件 弹出菜单(PopUp 控件) 2 实现数据库插入操作 文件名通过参数传递
- Python 文件操作实现代码
- Python 文件操作实现代码
- Flex与.NET互操作(十五):使用FluorineFx中的字节数组(ByteArray)实现图片上传
- 用python libxml libxslt实现xml操作
- 操作Oracle数据库实现上传图片到Blob类型的字段出现的问题
- Asp.net MVC防止图片盗链的实现方法,通过自定义RouteHandler来操作
- 【Android游戏开发十六】Android Gesture之【触摸屏手势识别】操作!利用触摸屏手势实现一个简单切换图片的功能!
- 【Android游戏开发十六】Android Gesture之【触摸屏手势识别】操作!利用触摸屏手势实现一个简单切换图片的功能!
- js 实现图片预加载(js操作 Image对象属性complete ,事件onload 异步加载图片)
- js 实现图片预加载(js操作 Image对象属性complete ,事件onload 异步加载图片)
- Android开发,实现可多选的图片ListView,便于批量操作
- WINCE6.0下用SilverLight技术实现的图片浏览器(支持手势操作,在模拟器下可以运行)
- Flex与.NET互操作(十五):使用FluorineFx中的字节数组(ByteArray)实现图片上传
- Flex与.NET互操作(十五):使用FluorineFx中的字节数组(ByteArray)实现图片上传
- 【Android游戏开发十六】Android Gesture之【触摸屏手势识别】操作!利用触摸屏手势实现一个简单切换图片的功能!
- Java实现MySQL图片存取操作
- 使用ImageMagick + Jmagick进行图片操作(续2)——实现高质量的图片处理
- 图片移动、鼠标画线----实现GIS地图操作