C语言 链表实现 学生成绩排名
2018-01-25 10:43
441 查看
题 源
本题来自于牛客网:
https://www.nowcoder.com/practice/0383714a1bb749499050d2e0610418b1?tpId=40&tqId=21333&tPage=1&rp=1&ru=/ta/kaoyan&qru=/ta/kaoyan/question-ranking
查找和排序
题目:输入任意(用户,成绩)序列,可以获得成绩从高到低或从低到高的排列,相同成绩都按先录入排列在前的规则处理。
例示:
jack 70
peter 96
Tom 70
smith 67
从高到低 成绩
peter 96
jack 70
Tom 70
smith 67
从低到高
smith 67
Tom 70
jack 70
peter 96
输入多行,先输入要排序的人的个数,然后输入排序方法0(降序)或者1(升序)再分别输入他们的名字和成绩,以一个空格隔开
按照指定方式输出名字和成绩,名字和成绩之间以一个空格隔开
示例1
输入
3
0
fang 90
yang 50
ning 70
输出
fang 90
ning 70
yang 50
C 代 码
CODE BLOCKS上运行结果
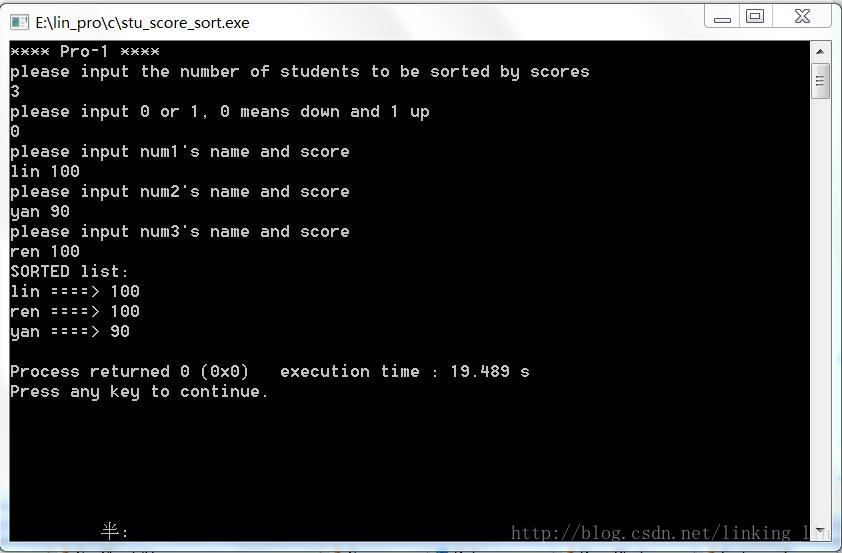
本题来自于牛客网:
https://www.nowcoder.com/practice/0383714a1bb749499050d2e0610418b1?tpId=40&tqId=21333&tPage=1&rp=1&ru=/ta/kaoyan&qru=/ta/kaoyan/question-ranking
题 目 描 述
查找和排序题目:输入任意(用户,成绩)序列,可以获得成绩从高到低或从低到高的排列,相同成绩都按先录入排列在前的规则处理。
例示:
jack 70
peter 96
Tom 70
smith 67
从高到低 成绩
peter 96
jack 70
Tom 70
smith 67
从低到高
smith 67
Tom 70
jack 70
peter 96
输入描述:
输入多行,先输入要排序的人的个数,然后输入排序方法0(降序)或者1(升序)再分别输入他们的名字和成绩,以一个空格隔开
输出描述:
按照指定方式输出名字和成绩,名字和成绩之间以一个空格隔开示例1
输入
3
0
fang 90
yang 50
ning 70
输出
fang 90
ning 70
yang 50
C 代 码
#include <stdio.h> #include <string.h> #include <malloc.h> typedef struct Student { char name[20]; int score; struct Stu *next; }Stu; int count_nodes_of_a_list(Stu * list) { Stu * tmp = list; int count = 0; while(tmp){ count++; tmp = tmp->next; } printf("%d nodes in total\n",count); return count; } void node_print(Stu * node) { printf("%s-->%d\n",node->name,node->score); } Stu * make_a_list(Stu *head, Stu* tmp) { if(tmp != NULL){ tmp->next = head; head = tmp; } return head; } Stu * up(Stu *head,Stu *tmp) { if(head->score == 0){ return tmp; }else if((head->score > tmp->score) || (head->score == tmp->score)){ tmp->next = head; return tmp; }else{ Stu *pre, *cur = head; while(cur && (cur->score< tmp->score || cur->score==tmp->score)){ pre = cur; cur = cur->next; } if(cur == NULL){ pre->next = tmp; }else{ tmp->next = cur; pre->next = tmp; } return head; } } Stu * down(Stu *head,Stu *tmp) { if(head->score == 0){ return tmp; }else if(head->score < tmp->score){ tmp->next = head; return tmp; }else{ Stu *pre, *cur = head; while(cur && ((cur->score > tmp->score) || (cur->score==tmp->score))){ pre = cur; cur = cur->next; } if(cur == NULL){ pre->next = tmp; }else{ tmp->next = cur; pre->next = tmp; } return head; } } int main() { printf("**** Pro-1 ****\n"); printf("please input the number of students to be sorted by scores \n"); int num_of_stu = 0; scanf("%d",&num_of_stu); printf("please input 0 or 1, 0 means down and 1 up \n"); int up_or_down = -1; scanf("%d",&up_or_down); if(!(num_of_stu>0 && (up_or_down==0 || up_or_down==1))){ printf("invalid input\n"); return -1; } Stu *head = (Stu *)malloc(sizeof(Stu)); head->next = NULL; head->score = 0; // node_print(head); for(int i=1;i<=num_of_stu;i++){ Stu *tmp_node = (Stu *)malloc(sizeof(Stu)); // printf("input num %d a84d name:\n",i); // scanf("%s",tmp_node->name); // printf("input num %d score:\n",i); // scanf("%d",&tmp_node->score); printf("please input num%d's name and score\n",i); scanf("%s %d",tmp_node->name,&tmp_node->score); tmp_node->next = NULL; if(1==up_or_down){ head = up(head,tmp_node); } else if(0==up_or_down){ head = down(head,tmp_node); } } Stu *pre, *cur = head; printf("SORTED list:\n"); while(cur){ printf("%s ====> %d\n",cur->name,cur->score); pre = cur; cur = cur->next; free(pre); pre = NULL; } return 0; }
CODE BLOCKS上运行结果
相关文章推荐
- 学生成绩管理系统课程设计(C语言,链表实现)
- C语言链表实现的简易学生成绩管理系统
- JAVA语言 实现简单的学生成绩管理系统(总分+平均分+排名)
- C语言简单用链表实现学生管理系统
- c语言实现学生成绩录入,主要是对指针的运用
- 用基于信息熵的topsis方法实现学生成绩的综合排名
- 链表实现学生成绩管理系统
- C语言实现学生信息管理系统(单链表)
- C语言实现简单学生成绩管理系统
- 数据结构实验二 链式存储结构----单向链表的有关操作(学生成绩信息C语言)
- 单链表实现学生成绩从高到低排序(C语言)
- c语言使用链表编写一个可以实现班级学生管理系统,增加,删除,修改学生信息
- 用单链表实现学生成绩管理
- C语言实现学生成绩管理系统
- 用链表写的学生管理系统 成绩的录入与查询都已经是实现了
- 算法初步--输入学生成绩,输出次数最多的成绩,如果有多个并列,按照从小到大输出(C语言实现)
- 学生成绩管理系统C语言代码实现
- <C语言>使用一个二维数组实现学生姓名管理系统,要求不能使用链表
- C语言实现学生成绩管理系统
- 【C++】单链表实现学生成绩表系统