短信网关post-get-soap请求方式发送验证码(兼容各个短信平台方案)
2018-01-22 10:13
387 查看
短信网关post-get-soap请求方式发送验证码
(兼容各个短信平台方案)
1、短信网关配置参数:
URL
接口请求路径
API
接口传入的参数(为平台所需参数拼接后的结果)
smsText
接収的短信息
smsMob
接収短信的手机号
2、web页面
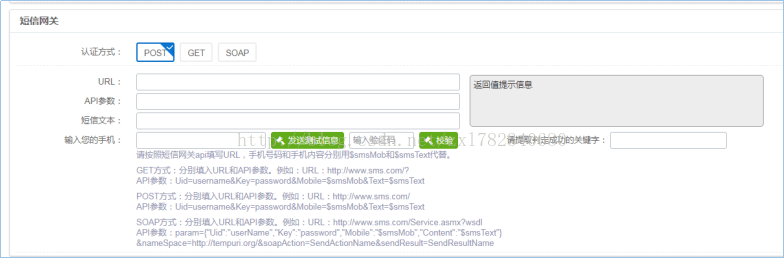
3、三种方式代码实现
A) .POST请求:
页面传入的参数示例:
URL --> https://sms.yunpian.com/v1/sms/send.json
API --> apikey=ed8c99685d9a7bcc3eb23eb4fbff4eca&text=$smsText&mobile=$smsMob
smsMob --> 17631357071
smsText
-->【神州泰岳】您的验证码是{code}
后台处理代码:
/**
* 将请求参数转换为post所识别的格式提交请求
*
@param url接口请求路径
*
@param API接口传入的参数
*
@param smsMob接収短信的手机号
*
@param smsText接収的短信息
*
@param mobile_code随机数(验证码)
*
@return result
返回的文本提示信息
*/
public String post(String url, String API, String smsMob, String smsText, Integer mobile_code){
// 判断链接是否真实
int responseCode =
this.getResponseCode(url);
if(responseCode != 200){
return "URL不是一个真实的链接!";
}
// 将传来的参数与占位串替换
API = API.replace("$smsMob",smsMob).replace("$smsText",smsText).replace("{code}", String.valueOf(mobile_code)).trim();
// 响应结果内容
String result = "";
Map<String, String> params = new HashMap<String, String>();
try{
String[] split = API.split("&");
for (String s : split) {
String[] splitParam = s.split("=");
params.put(splitParam[0], splitParam[1]);
}
result = postRequest(url, params, "UTF-8");
}catch (Exception e) {
e.printStackTrace();
return "API异常!请检查参数格式是否有误!";
}
System.out.println("POST返回值:"+result);
return result;
}
/**
* 基于HttpClient 4.3的通用POST方法
*
@param url 提交的URL
*
@param paramsMap 提交<参数,值>Map
*
@return 提交响应
*/
private String postRequest(String url, Map<String, String> paramsMap,String ENCODING) {
// 创建一个默认的http请求
CloseableHttpClient client = HttpClients.createDefault();
String responseText = "";
CloseableHttpResponse response = null;
try {
// 请求方式为post 若使用get则创建HttpGet()
HttpPost method = new HttpPost(url);
if (paramsMap !=
null) {
// 创建一个NameValuePair数组,用于存储欲传送的参数(常用于发送post请求)
List<NameValuePair> paramList = new ArrayList<NameValuePair>();
for (Map.Entry<String, String> param : paramsMap.entrySet()) {
NameValuePair pair = new BasicNameValuePair(param.getKey(),
param.getValue());
paramList.add(pair);
}
// 设置请求参数
method.setEntity(new UrlEncodedFormEntity(paramList, ENCODING));
}
// 发送请求 返回一个httpResponse
response = client.execute(method);
// 获取响应内容
HttpEntity entity = response.getEntity();
if (entity !=
null) {
responseText = EntityUtils.toString(entity);
}
} catch (Exception e) {
e.printStackTrace();
return "请求URL/API异常!请检查参数格式是否有误!";
} finally {
try {
response.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return responseText;
}
/**
* 获取url状态码
*
@param url
*
@return 状态码
*/
private int getResponseCode(String url){
// 判断url是否为真实的链接
int responseCode = 404;
try {
URL u = new URL(url);
HttpURLConnection connection = (HttpURLConnection)u.openConnection();
responseCode = connection.getResponseCode();
} catch (Exception e1) {
System.out.println("获取http请求状态码异常");
e1.printStackTrace();
}
System.out.println(url+"该链接的状态码为:"+responseCode);
return responseCode;
}
B) .GET请求:
页面传入的参数示例:注:(get请求的url和API之间使用?连接)
URL --> https://sms.yunpian.com/v1/sms/send.json?
API --> apikey=ed8c99685d9a7bcc3eb23eb4fbff4eca&text=$smsText&mobile=$smsMob
smsMob --> 17631357071
smsText
-->【神州泰岳】您的验证码是{code}
后台处理代码:
/**
* 短信网关get认证方式
*
@param url接口请求路径
*
@param API接口传入的参数
*
@param smsMob接収短信的手机号
*
@param smsText接収的短信息
*
@param mobile_code随机数(验证码)
*
@param request调用session将验证码存入作用域用来做后续的验证
*
@return result
返回的文本提示信息
*/
public String get(String url,String API, String smsMob, String smsText, Integer mobile_code) {
int responseCode =
this.getResponseCode(url);
if(responseCode != 200){
return "URL不是一个真实的链接!";
}
url = url+API.replace("$smsMob",smsMob).replace("$smsText",smsText).replace("{code}", String.valueOf(mobile_code)).trim();
responseCode = this.getResponseCode(url);
if(responseCode != 200){
return "请求URL/API异常!请检查参数格式是否有误!";
}
// 创建一个默认的http请求
CloseableHttpClient client = HttpClients.createDefault();
// 响应的文本内容
String responseText = "";
CloseableHttpResponse response = null;
try {
// 创建get请求方式
HttpGet method = new HttpGet(url);
// 发送请求 返回一个httpResponse
response = client.execute(method);
// 获取响应内容
HttpEntity entity = response.getEntity();
if (entity !=
null) {
responseText = EntityUtils.toString(entity);
}
} catch (Exception e) {
e.printStackTrace();
return "请求URL/API异常!请检查参数格式是否有误!";
} finally {
try {
response.close();
} catch (Exception e) {
e.printStackTrace();
}
}
System.out.println("GET返回值:"+responseText);
return responseText;
}
C) .SOAP请求:
页面传入的参数示例:
URL --> http://service2.winic.org:8003/Service.asmx?wsdl
API
--> param={"uid":"C9868175","pwd":"9a659112bbfba19dde0d4fc898095159","tos":"$smsMob","msg":"$smsText"}
&nameSpace=http://tempuri.org/&soapAction=SendMessages&sendResult=SendMessagesResult
smsMob --> 17631357071
smsText
-->【神州泰岳】您的验证码是{code}
后台处理代码:
/**
* 短信网关soap认证方式
*
@param url接口服务地址
*
@param API接口传入的参数
*
@param smsMob接収短信的手机号
*
@param smsText接収的短信息
*
[b]@param mobile_code随机数(验证码)
*
@return result
返回的文本提示信息
*/
public String soap(String url, String API, String smsMob, String smsText, Integer mobile_code){
// 判断链接是否真实
int responseCode =
this.getResponseCode(url);
if(responseCode != 200){
return "URL不是一个真实的链接!";
}
String api = "";
String nameSpace = "";
String soapAction = "";
String sendResult = "";
String[] split = API.split("&");
for (String s : split) {
String[] splitParam = s.split("=");
if("param".equals(splitParam[0])){
api = splitParam[1];
}else if("nameSpace".equals(splitParam[0])){
nameSpace = splitParam[1];
}else if("soapAction".equals(splitParam[0])){
soapAction = splitParam[1];
}else if("sendResult".equals(splitParam[0])){
sendResult = splitParam[1];
}
}
api = api.replace("$smsMob",smsMob).replace("$smsText",smsText).replace("{code}",String.valueOf(mobile_code)).trim();
// 将API中的paramJSON字符串解析为xml文本
StringBuffer xmlParam = new StringBuffer();
try{
JSONObject object = JSONObject.fromObject(api);
Set<?> set = object.keySet();
Iterator<?> it = set.iterator();
while (it.hasNext()) {
Object key = (Object) it.next();
String value = (String) object.get(key);
xmlParam.append("<"+key+">"+value+"</"+key+">\n");
}
}catch(Exception e){
e.printStackTrace();
return "请检查API中param参数是否正确!";
}
String result = sendSms(url,xmlParam.toString(),nameSpace,soapAction,sendResult);
System.out.println("SOAP返回值:"+result);
return result;
}
/**
* 对服务器端返回的XML进行解析
*
@param url 接口服务地址
*
@param api 接口传入的参数
*
@param nameSpace webservice调用服务命名空间
*
@param soapAction 调用服务操作的方法
*
@param sendResult 服务返回结果的方法
*
@return 返回结果的文本信息
*/
private String
sendSms(String url, String xmlParam, String nameSpace, String soapAction, String sendResult) {
try {
Document doc;
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
dbf.setNamespaceAware(true);
DocumentBuilder db = dbf.newDocumentBuilder();
InputStream is = getSoapInputStream(url,xmlParam,nameSpace,soapAction);
StringBuffer sb = new StringBuffer();
if(is!=null){
doc=db.parse(is);
NodeList nl=doc.getElementsByTagName(sendResult);
Node n=nl.item(0);
sb.append(n.getFirstChild().getNodeValue());
is.close();
return sb.toString();
}else {
return "请检查SOAPAction填写是否正确...";
}
} catch (Exception e) {
e.printStackTrace();
return "sendSms异常!请检查参数格式是否有误!";
}
}
/**
* 用户把SOAP请求发送给服务器端,并返回服务器点返回的输入流
*
@param URL 接口服务地址
*
@param xmlParam 接口传入的参数
*
@param nameSpace webservice调用服务命名空间
*
@param soapAction 调用服务操作的方法
*
@return 服务器端返回的输入流,供客户端读取
*
@throws Exception
*/
private InputStream getSoapInputStream(String URL, String xmlParam, String nameSpace, String soapAction)
throws Exception {
try {
String soap = getSoapRequest(xmlParam,nameSpace,soapAction);
if (soap ==
null) {
return null;
}
URL url = new URL(URL);
URLConnection conn = url.openConnection();
conn.setUseCaches(false);
conn.setDoInput(true);
conn.setDoOutput(true);
conn.setRequestProperty("Content-Length", Integer.toString(soap.length()));
conn.setRequestProperty("Content-Type",
"text/xml; charset=utf-8");
// http://tempuri.org/ Web服务唯一的命名空间,以便客户端应用程序在网络上区别于其它服务
conn.setRequestProperty("SOAPAction",nameSpace+soapAction);
OutputStream os = conn.getOutputStream();
OutputStreamWriter osw = new OutputStreamWriter(os,
"utf-8");
osw.write(soap);
osw.flush();
osw.close();
InputStream is = conn.getInputStream();
return is;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
/**
* 获取SOAP的请求头
*
@param xmlParam 接口传入的参数
*
@param nameSpace webservice调用服务命名空间
*
@param soapAction 调用服务操作的方法
*
@return 客户将要发送给服务器的SOAP请求
*/
private String getSoapRequest(String xmlParam, String nameSpace, String soapAction) {
StringBuilder sb = new StringBuilder();
sb.append("<?xml version=\"1.0\" encoding=\"utf-8\"?>"
+ "<soap:Envelope xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\" "
+ "xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" "
+ "xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\">"
+ "<soap:Body>"
+ "<"+ soapAction +" xmlns=\""+ nameSpace +"\">"
+ ""+ xmlParam +""
+ "</"+ soapAction +">"
+ "</soap:Body>"
+ "</soap:Envelope>");
return sb.toString();
}
4、返回值处理
调用接口的返回值不进行处理,直接显示在页面的“返回值提示框”中,根据提示信息,完善网关的配置。若返回值提示的信息表示发送成功,则截取返回值的关键信息,当做发送成功的标识,存储数据库用来做后续的校验。
(兼容各个短信平台方案)
1、短信网关配置参数:
URL
接口请求路径
API
接口传入的参数(为平台所需参数拼接后的结果)
smsText
接収的短信息
smsMob
接収短信的手机号
2、web页面
3、三种方式代码实现
A) .POST请求:
页面传入的参数示例:
URL --> https://sms.yunpian.com/v1/sms/send.json
API --> apikey=ed8c99685d9a7bcc3eb23eb4fbff4eca&text=$smsText&mobile=$smsMob
smsMob --> 17631357071
smsText
-->【神州泰岳】您的验证码是{code}
后台处理代码:
/**
* 将请求参数转换为post所识别的格式提交请求
*
@param url接口请求路径
*
@param API接口传入的参数
*
@param smsMob接収短信的手机号
*
@param smsText接収的短信息
*
@param mobile_code随机数(验证码)
*
@return result
返回的文本提示信息
*/
public String post(String url, String API, String smsMob, String smsText, Integer mobile_code){
// 判断链接是否真实
int responseCode =
this.getResponseCode(url);
if(responseCode != 200){
return "URL不是一个真实的链接!";
}
// 将传来的参数与占位串替换
API = API.replace("$smsMob",smsMob).replace("$smsText",smsText).replace("{code}", String.valueOf(mobile_code)).trim();
// 响应结果内容
String result = "";
Map<String, String> params = new HashMap<String, String>();
try{
String[] split = API.split("&");
for (String s : split) {
String[] splitParam = s.split("=");
params.put(splitParam[0], splitParam[1]);
}
result = postRequest(url, params, "UTF-8");
}catch (Exception e) {
e.printStackTrace();
return "API异常!请检查参数格式是否有误!";
}
System.out.println("POST返回值:"+result);
return result;
}
/**
* 基于HttpClient 4.3的通用POST方法
*
@param url 提交的URL
*
@param paramsMap 提交<参数,值>Map
*
@return 提交响应
*/
private String postRequest(String url, Map<String, String> paramsMap,String ENCODING) {
// 创建一个默认的http请求
CloseableHttpClient client = HttpClients.createDefault();
String responseText = "";
CloseableHttpResponse response = null;
try {
// 请求方式为post 若使用get则创建HttpGet()
HttpPost method = new HttpPost(url);
if (paramsMap !=
null) {
// 创建一个NameValuePair数组,用于存储欲传送的参数(常用于发送post请求)
List<NameValuePair> paramList = new ArrayList<NameValuePair>();
for (Map.Entry<String, String> param : paramsMap.entrySet()) {
NameValuePair pair = new BasicNameValuePair(param.getKey(),
param.getValue());
paramList.add(pair);
}
// 设置请求参数
method.setEntity(new UrlEncodedFormEntity(paramList, ENCODING));
}
// 发送请求 返回一个httpResponse
response = client.execute(method);
// 获取响应内容
HttpEntity entity = response.getEntity();
if (entity !=
null) {
responseText = EntityUtils.toString(entity);
}
} catch (Exception e) {
e.printStackTrace();
return "请求URL/API异常!请检查参数格式是否有误!";
} finally {
try {
response.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return responseText;
}
/**
* 获取url状态码
*
@param url
*
@return 状态码
*/
private int getResponseCode(String url){
// 判断url是否为真实的链接
int responseCode = 404;
try {
URL u = new URL(url);
HttpURLConnection connection = (HttpURLConnection)u.openConnection();
responseCode = connection.getResponseCode();
} catch (Exception e1) {
System.out.println("获取http请求状态码异常");
e1.printStackTrace();
}
System.out.println(url+"该链接的状态码为:"+responseCode);
return responseCode;
}
B) .GET请求:
页面传入的参数示例:注:(get请求的url和API之间使用?连接)
URL --> https://sms.yunpian.com/v1/sms/send.json?
API --> apikey=ed8c99685d9a7bcc3eb23eb4fbff4eca&text=$smsText&mobile=$smsMob
smsMob --> 17631357071
smsText
-->【神州泰岳】您的验证码是{code}
后台处理代码:
/**
* 短信网关get认证方式
*
@param url接口请求路径
*
@param API接口传入的参数
*
@param smsMob接収短信的手机号
*
@param smsText接収的短信息
*
@param mobile_code随机数(验证码)
*
@param request调用session将验证码存入作用域用来做后续的验证
*
@return result
返回的文本提示信息
*/
public String get(String url,String API, String smsMob, String smsText, Integer mobile_code) {
int responseCode =
this.getResponseCode(url);
if(responseCode != 200){
return "URL不是一个真实的链接!";
}
url = url+API.replace("$smsMob",smsMob).replace("$smsText",smsText).replace("{code}", String.valueOf(mobile_code)).trim();
responseCode = this.getResponseCode(url);
if(responseCode != 200){
return "请求URL/API异常!请检查参数格式是否有误!";
}
// 创建一个默认的http请求
CloseableHttpClient client = HttpClients.createDefault();
// 响应的文本内容
String responseText = "";
CloseableHttpResponse response = null;
try {
// 创建get请求方式
HttpGet method = new HttpGet(url);
// 发送请求 返回一个httpResponse
response = client.execute(method);
// 获取响应内容
HttpEntity entity = response.getEntity();
if (entity !=
null) {
responseText = EntityUtils.toString(entity);
}
} catch (Exception e) {
e.printStackTrace();
return "请求URL/API异常!请检查参数格式是否有误!";
} finally {
try {
response.close();
} catch (Exception e) {
e.printStackTrace();
}
}
System.out.println("GET返回值:"+responseText);
return responseText;
}
C) .SOAP请求:
页面传入的参数示例:
URL --> http://service2.winic.org:8003/Service.asmx?wsdl
API
--> param={"uid":"C9868175","pwd":"9a659112bbfba19dde0d4fc898095159","tos":"$smsMob","msg":"$smsText"}
&nameSpace=http://tempuri.org/&soapAction=SendMessages&sendResult=SendMessagesResult
smsMob --> 17631357071
smsText
-->【神州泰岳】您的验证码是{code}
后台处理代码:
/**
* 短信网关soap认证方式
*
@param url接口服务地址
*
@param API接口传入的参数
*
@param smsMob接収短信的手机号
*
@param smsText接収的短信息
*
[b]@param mobile_code随机数(验证码)
*
@return result
返回的文本提示信息
*/
public String soap(String url, String API, String smsMob, String smsText, Integer mobile_code){
// 判断链接是否真实
int responseCode =
this.getResponseCode(url);
if(responseCode != 200){
return "URL不是一个真实的链接!";
}
String api = "";
String nameSpace = "";
String soapAction = "";
String sendResult = "";
String[] split = API.split("&");
for (String s : split) {
String[] splitParam = s.split("=");
if("param".equals(splitParam[0])){
api = splitParam[1];
}else if("nameSpace".equals(splitParam[0])){
nameSpace = splitParam[1];
}else if("soapAction".equals(splitParam[0])){
soapAction = splitParam[1];
}else if("sendResult".equals(splitParam[0])){
sendResult = splitParam[1];
}
}
api = api.replace("$smsMob",smsMob).replace("$smsText",smsText).replace("{code}",String.valueOf(mobile_code)).trim();
// 将API中的paramJSON字符串解析为xml文本
StringBuffer xmlParam = new StringBuffer();
try{
JSONObject object = JSONObject.fromObject(api);
Set<?> set = object.keySet();
Iterator<?> it = set.iterator();
while (it.hasNext()) {
Object key = (Object) it.next();
String value = (String) object.get(key);
xmlParam.append("<"+key+">"+value+"</"+key+">\n");
}
}catch(Exception e){
e.printStackTrace();
return "请检查API中param参数是否正确!";
}
String result = sendSms(url,xmlParam.toString(),nameSpace,soapAction,sendResult);
System.out.println("SOAP返回值:"+result);
return result;
}
/**
* 对服务器端返回的XML进行解析
*
@param url 接口服务地址
*
@param api 接口传入的参数
*
@param nameSpace webservice调用服务命名空间
*
@param soapAction 调用服务操作的方法
*
@param sendResult 服务返回结果的方法
*
@return 返回结果的文本信息
*/
private String
sendSms(String url, String xmlParam, String nameSpace, String soapAction, String sendResult) {
try {
Document doc;
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
dbf.setNamespaceAware(true);
DocumentBuilder db = dbf.newDocumentBuilder();
InputStream is = getSoapInputStream(url,xmlParam,nameSpace,soapAction);
StringBuffer sb = new StringBuffer();
if(is!=null){
doc=db.parse(is);
NodeList nl=doc.getElementsByTagName(sendResult);
Node n=nl.item(0);
sb.append(n.getFirstChild().getNodeValue());
is.close();
return sb.toString();
}else {
return "请检查SOAPAction填写是否正确...";
}
} catch (Exception e) {
e.printStackTrace();
return "sendSms异常!请检查参数格式是否有误!";
}
}
/**
* 用户把SOAP请求发送给服务器端,并返回服务器点返回的输入流
*
@param URL 接口服务地址
*
@param xmlParam 接口传入的参数
*
@param nameSpace webservice调用服务命名空间
*
@param soapAction 调用服务操作的方法
*
@return 服务器端返回的输入流,供客户端读取
*
@throws Exception
*/
private InputStream getSoapInputStream(String URL, String xmlParam, String nameSpace, String soapAction)
throws Exception {
try {
String soap = getSoapRequest(xmlParam,nameSpace,soapAction);
if (soap ==
null) {
return null;
}
URL url = new URL(URL);
URLConnection conn = url.openConnection();
conn.setUseCaches(false);
conn.setDoInput(true);
conn.setDoOutput(true);
conn.setRequestProperty("Content-Length", Integer.toString(soap.length()));
conn.setRequestProperty("Content-Type",
"text/xml; charset=utf-8");
// http://tempuri.org/ Web服务唯一的命名空间,以便客户端应用程序在网络上区别于其它服务
conn.setRequestProperty("SOAPAction",nameSpace+soapAction);
OutputStream os = conn.getOutputStream();
OutputStreamWriter osw = new OutputStreamWriter(os,
"utf-8");
osw.write(soap);
osw.flush();
osw.close();
InputStream is = conn.getInputStream();
return is;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
/**
* 获取SOAP的请求头
*
@param xmlParam 接口传入的参数
*
@param nameSpace webservice调用服务命名空间
*
@param soapAction 调用服务操作的方法
*
@return 客户将要发送给服务器的SOAP请求
*/
private String getSoapRequest(String xmlParam, String nameSpace, String soapAction) {
StringBuilder sb = new StringBuilder();
sb.append("<?xml version=\"1.0\" encoding=\"utf-8\"?>"
+ "<soap:Envelope xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\" "
+ "xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" "
+ "xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\">"
+ "<soap:Body>"
+ "<"+ soapAction +" xmlns=\""+ nameSpace +"\">"
+ ""+ xmlParam +""
+ "</"+ soapAction +">"
+ "</soap:Body>"
+ "</soap:Envelope>");
return sb.toString();
}
4、返回值处理
调用接口的返回值不进行处理,直接显示在页面的“返回值提示框”中,根据提示信息,完善网关的配置。若返回值提示的信息表示发送成功,则截取返回值的关键信息,当做发送成功的标识,存储数据库用来做后续的校验。
相关文章推荐
- IO流、HttpClient、GET方式请求MT发送短信
- JAVA利用第三方平台发送短信验证码
- ajax之使用get方式向服务器发送请求
- PHP发送POST请求的三种方式 分别使用curl file_get_content fsocket 来实现post提交数据
- 发送短信验证码到手机(阿里大于平台) java
- AJAX发送数据之get请求方式
- JAVA利用第三方平台发送短信验证码。
- 手机验证码平台,怎么发送手机验证码,php开发手机验证码短信接口功能
- [iOS开发] 发送验证码的倒计时(采用异步请求的方式)
- c++实现发送http请求通过get方式获取网页源代码
- java发送http get请求的两种方式
- ajax的get 和post方式发送请求
- JAVA实现利用第三方平台发送短信验证码
- Java发送HTTP的POST和GET方式请求
- C#模拟发送http get、post请求的方式
- 【网络】JAVA Socket 实现HTTP与HTTPS客户端发送POST与GET方式请求
- 同步和异步Httpclient通过get和post方式发送请求
- Ajax的GET与POST方式向服务器发送请求
- IE浏览器发送get请求时的缓存问题的解决方式
- ajax通过get方式发送请求