PCL+OpenCV+WebCam实现模拟深度图像实时点云数据采集
2018-01-20 00:00
537 查看
#include <iostream> #include <opencv2/opencv.hpp> #include <pcl/point_types.h> #include <pcl/filters/extract_indices.h> #include <pcl/visualization/cloud_viewer.h> using namespace std; pcl::PointCloud<pcl::PointXYZ>::Ptr MatToPoinXYZ(cv::Mat depthMat) { pcl::PointCloud<pcl::PointXYZ>::Ptr ptCloud (new pcl::PointCloud<pcl::PointXYZ>); // calibration parameters float const fx_d = 5.9421434211923247e+02; float const fy_d = 5.9104053696870778e+02; float const cx_d = 3.3930780975300314e+02; float const cy_d = 2.4273913761751615e+02; unsigned char* p = depthMat.data; for (int i = 0; i<depthMat.rows; i++) { for (int j = 0; j < depthMat.cols; j++) { float z = static_cast<float>(*p); pcl::PointXYZ point; point.z = 0.001 * z; point.x = point.z*(j - cx_d) / fx_d; point.y = point.z *(cy_d - i) / fy_d; ptCloud->points.push_back(point); ++p; } } ptCloud->width = (int)depthMat.cols; ptCloud->height = (int)depthMat.rows; return ptCloud; } pcl::PointCloud<pcl::PointXYZ>::Ptr MatToPoinXYZ2(cv::Mat OpencVPointCloud) { //char pr=100, pg=100, pb=100; pcl::PointCloud<pcl::PointXYZ>::Ptr point_cloud_ptr(new pcl::PointCloud<pcl::PointXYZ>);//(new pcl::pointcloud<pcl::pointXYZ>); for(int i=0;i<OpencVPointCloud.cols;i++) { //std::cout<<i<<endl; pcl::PointXYZ point; point.x = OpencVPointCloud.at<float>(0,i); point.y = OpencVPointCloud.at<float>(1,i); point.z = OpencVPointCloud.at<float>(2,i); point_cloud_ptr -> points.push_back(point); } point_cloud_ptr->width = (int)point_cloud_ptr->points.size(); point_cloud_ptr->height = 1; return point_cloud_ptr; } pcl::PointCloud<pcl::PointXYZRGB>::Ptr MatToPoinXYZRGB(cv::Mat depthMat) { pcl::PointCloud<pcl::PointXYZRGB>::Ptr ptCloud (new pcl::PointCloud<pcl::PointXYZRGB>); // calibration parameters float const fx_d = 5.9421434211923247e+02; float const fy_d = 5.9104053696870778e+02; float const cx_d = 3.3930780975300314e+02; float const cy_d = 2.4273913761751615e+02; unsigned char* p = depthMat.data; for (int i = 0; i<depthMat.rows; i++) { for (int j = 0; j < depthMat.cols; j++) { float z = static_cast<float>(*p); pcl::PointXYZRGB point; point.z = 0.001 * z; point.x = point.z*(j - cx_d) / fx_d; point.y = point.z *(cy_d - i) / fy_d; point.r = depthMat.at<cv::Vec3b>(i,j)[2]; point.g = depthMat.at<cv::Vec3b>(i,j)[1]; point.b = depthMat.at<cv::Vec3b>(i,j)[0]; ptCloud->points.push_back(point); ++p; } } ptCloud->width = (int)depthMat.cols; ptCloud->height = (int)depthMat.rows; return ptCloud; } int main() { pcl::visualization::CloudViewer viewer("Cloud Viewer");//创建viewer对象 cv::VideoCapture cap(0); if(!cap.isOpened()){ std::cerr << "cannot open camera" << std::endl; exit(0); } cv::Mat frame; while (!viewer.wasStopped ()) { cap >> frame; if(frame.empty()){ continue; } viewer.showCloud(MatToPoinXYZRGB(frame)); } return 0; }
程序运行结果如下:
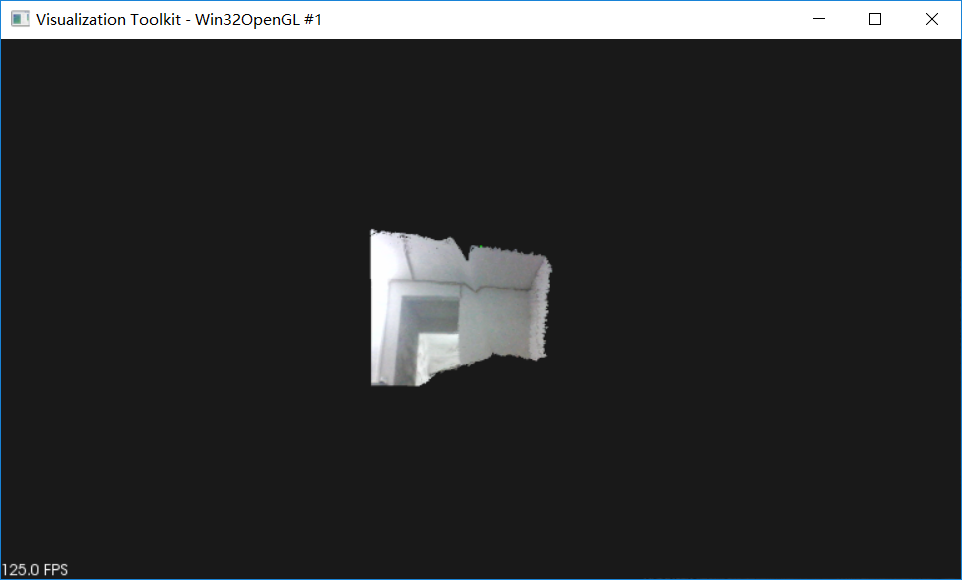
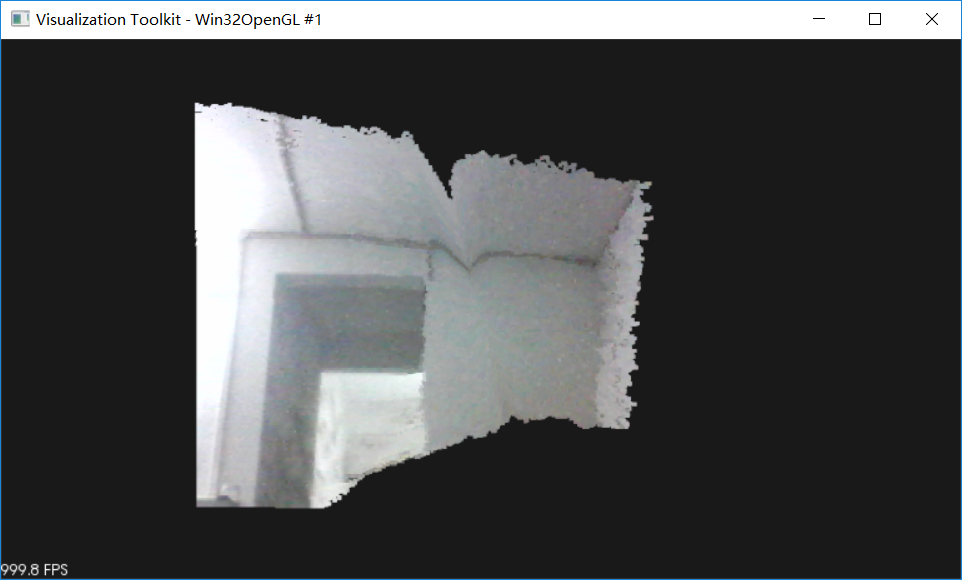
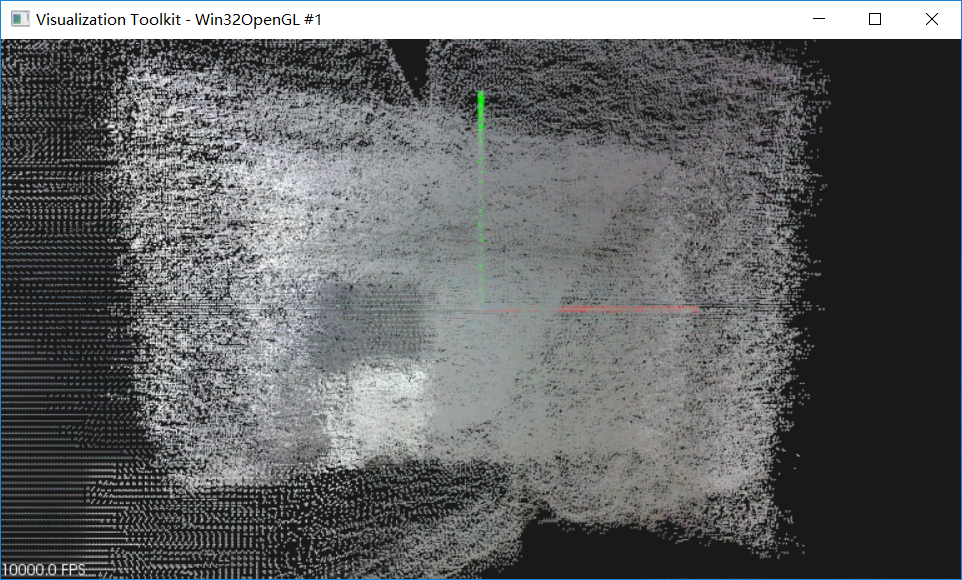
相关文章推荐
- 在pcl用例中获取kinect深度图像数据,并用opencv显示出来
- opencv实现摄像头的实时图像采集与显示
- Halcon+VisualStudio2015使用线程实现大恒水星相机实时图像采集
- javacpp-opencv图像处理之2:实时视频添加图片水印,实现不同大小图片叠加,图像透明度控制,文字和图片双水印
- OpenCV实现运动模糊图像的模拟
- C# 实现 客户端 对实时数据的采集 上传至服务端;在服务端把保存到内存中;供WEB页面调用
- 使用Socket通信实现Silverlight客户端实时数据的获取(模拟GPS数据,地图实时位置)
- 多路视频数据实时采集的软件实现
- 关于OpenCV中利用函数cvConvert实现图像数据类型转换(8U->16S)
- PMODAD1 实现模拟数据的采集 时序篇
- 基于USB的嵌入式CCD图像数据采集系统的实现
- fpga+dp83848实现百兆网络高速数据实时采集
- opencv 开启摄像头实时采集图像
- opencv+directshow实现4个摄像头同步采集图像
- OPENcv从相机采集图像数据-图像处理接口IPLIMAGE结构的指针
- php中CURL实现模拟登录并采集数据
- flume实现kafka到hdfs实时数据采集 - 有负载均衡策略
- Android 使用OPENCV实现图像实时对比
- 利用USB2.0接口的芯片CY7C68013和单片机实现全数字图像的实时采集
- PMODAD1 实现模拟数据的采集 实现篇1