Spring boot中使用Mongodb
2018-01-08 17:12
337 查看
安装
使用Idea新建Spring boot工程时需要选择Mongodb
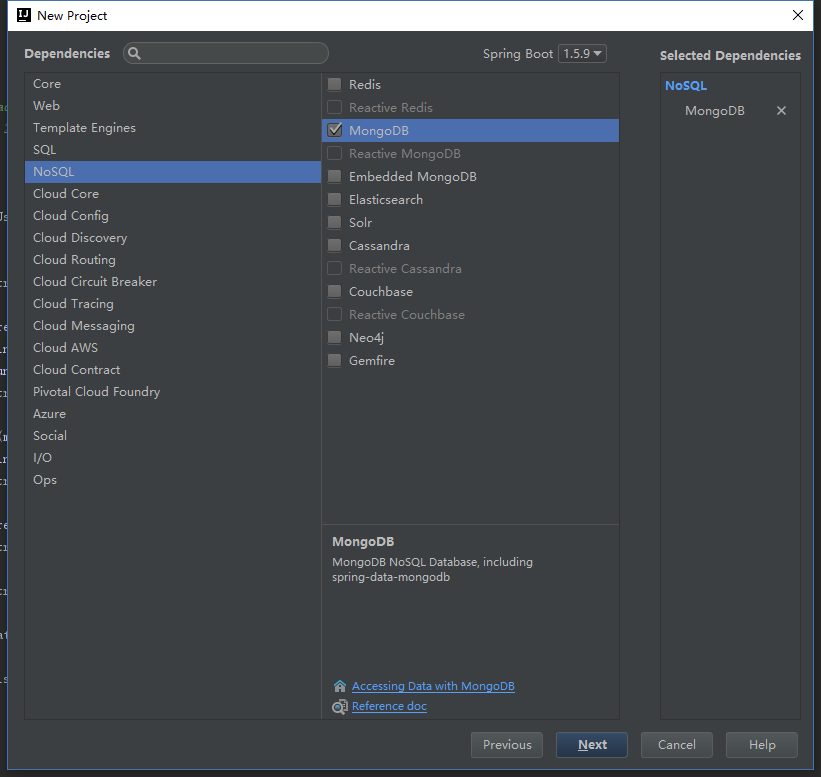
或者在工程中加入依赖
Maven:
Gradle:
目录结构

配置
在application.yml中配置
或者在application.properties配置
其中URL的格式是:mongodb://用户名:用户名@ip地址:端口/数据库
使用
在实体类中加入注解
@Document表示该类为实体类
@Id表示该字段为自动生成的ID
@Indexed表示自动为该字段建立索引
编写查询接口
查询接口实现
测试
测试类
查询出结果即完成
使用Idea新建Spring boot工程时需要选择Mongodb
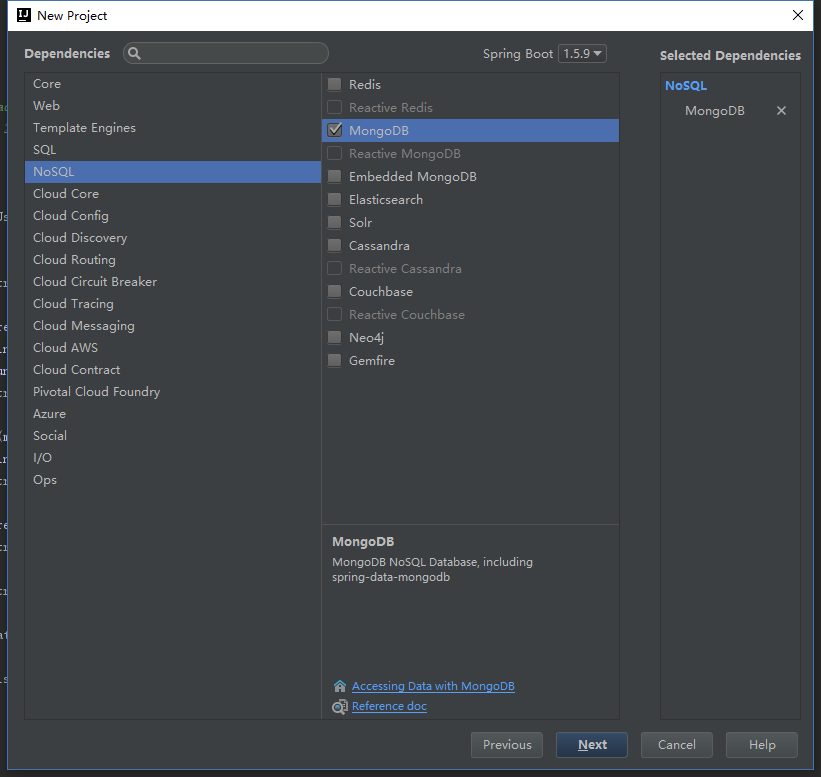
或者在工程中加入依赖
Maven:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-mongodb</artifactId> </dependency>
Gradle:
dependencies { compile('org.springframework.boot:spring-boot-starter-data-mongodb') }
目录结构

配置
在application.yml中配置
spring: data: mongodb: uri: mongodb://test:test@127.0.0.1:27017/test
或者在application.properties配置
spring.data.mongodb.uri=mongodb://test:test@127.0.0.1:27017/test
其中URL的格式是:mongodb://用户名:用户名@ip地址:端口/数据库
使用
在实体类中加入注解
package com.example.demo.domain; import org.springframework.data.annotation.Id; import org.springframework.data.mongodb.core.index.IndexDirection; import org.springframework.data.mongodb.core.index.Indexed; import org.springframework.data.mongodb.core.mapping.Document; import java.io.Serializable; /** * 用户模型 * * @author hackyo * Created on 2017/12/3 11:53. */ @Document public class User implements Serializable { @Id private String id; @Indexed(unique = true, direction = IndexDirection.DESCENDING, dropDups = true) private String username; public String getId() { return id; } public void setId(String id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } }
@Document表示该类为实体类
@Id表示该字段为自动生成的ID
@Indexed表示自动为该字段建立索引
编写查询接口
package com.example.demo.dao; import com.example.demo.domain.User; /** * 用户表操作接口 * * @author hackyo * Created on 2017/12/3 11:53. */ public interface IUserRepository { /** * 通过用户名查找用户 * * @param username 用户名 * @return 用户信息 */ User findByUsername(String username); }
查询接口实现
package com.example.demo.dao.impl; import com.example.demo.dao.IUserRepository; import com.example.demo.domain.User; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.data.mongodb.core.query.Criteria; import org.springframework.data.mongodb.core.query.Query; import org.springframework.stereotype.Repository; /** * 用户表操作接口实现 * * @author hackyo * Created on 2017/12/3 11:53. */ @Repository public class UserRepositoryImpl implements IUserRepository { private MongoTemplate mongoTemplate; @Autowired public UserRepositoryImpl(MongoTemplate mongoTemplate) { this.mongoTemplate = mongoTemplate; } @Override public User findByUsername(String username) { Query query = new Query(Criteria.where("username").is(username)); return mongoTemplate.findOne(query, User.class); } }
测试
测试类
package com.example.demo; import com.example.demo.dao.IUserRepository; import com.example.demo.domain.User; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.test.context.junit4.SpringRunner; @RunWith(SpringRunner.class) @SpringBootTest public class DemoApplicationTests { @Autowired private IUserRepository userRepository; @Test public void contextLoads() { } @Test public void test() { User user = userRepository.findByUsername("test"); System.out.println(user.getId()); } }
查询出结果即完成
相关文章推荐
- springboot(十一):Spring boot中mongodb的使用
- Spring Boot 2.0.0.M7 springBoot-mongodb使用
- springboot(十一):Spring boot中mongodb的使用
- SpringBoot中MongoDB注解概念及使用
- 1.2 使用IntelliJ IDEA搭建Spring-Boot与MongoDB数据的简单登录项目
- Spring boot中mongodb的使用
- SpringBoot中简单使用mongodb
- Spring Boot中使用MongoDB的连接池配置
- SpringBoot中简单使用mongodb
- spring-boot使用mongoDB
- 如何使用MongoDB+Springboot实现分布式ID
- 如何使用MongoDB+Springboot实现分布式ID?
- 基于Springboot使用MongoDB进行简单Gis操作
- Spring Boot中使用MongoDB3.0出现auth failed
- SpringBoot | 第三十一章:MongoDB的集成和使用
- SpringBoot中MongoDB注解概念及使用
- springboot(十一):Spring boot中mongodb的使用
- SpringBoot(十一):Spring boot 中 mongodb 的使用
- MongoDB最简单的入门教程之四:使用Spring Boot操作MongoDB
- springboot(十一):Spring boot中mongodb的使用