第九章 Spring Cloud Feign声明式调用服务
2017-12-31 12:13
996 查看
在Spring Cloud Netflix栈中,各个微服务都是以HTTP接口的形式暴露自身服务的,因此在调用远程服务时就必须使用HTTP客户端。我们可以使用JDK原生的
Client, Spring的
Cloud Feign基于Netflix Feign
实现的,整理Spring Cloud Ribbon
与 Spring CloudHystrix,并且实现了声明式的Web服务客户端定义方式。
项目结构如图:
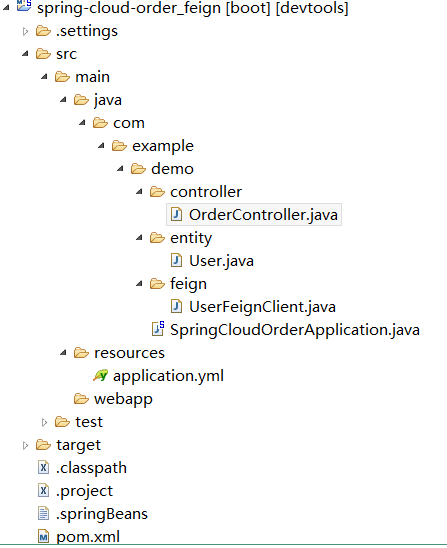
OrderController类
User类
UserFeignClient类
SpringCloudOrderApplication类
application.yml配置
pom.xml配置
URLConnection、Apache的
HttpClient、Netty的异步HTTP
Client, Spring的
RestTemplate。但是,用起来最方便、最优雅的还是要属Feign了。在实际项目中,都是使用声明式调用服务。而不会在客服端和服务端存储2份相同的model和api定义。Feign在RestTemplate的基础上对其封装,由它来帮助我们定义和实现依赖服务接口的定义。Spring
Cloud Feign基于Netflix Feign
实现的,整理Spring Cloud Ribbon
与 Spring CloudHystrix,并且实现了声明式的Web服务客户端定义方式。
项目结构如图:
OrderController类
package com.example.demo.controller; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController; import com.example.demo.entity.User; import com.example.demo.feign.UserFeignClient; //声明式服务 @RestController public class OrderController { @Autowired private UserFeignClient userFeignClient; @GetMapping("/order/{id}") public User findById(@PathVariable Long id) { return this.userFeignClient.findById(id); } @GetMapping("/testPost") public User testPost(User user) { return this.userFeignClient.postUser(user); } @GetMapping("/testGet") public User testGet(User user) { return this.userFeignClient.getUser(user); } }
User类
package com.example.demo.entity; import java.math.BigDecimal; public class User { private Long id; private String username; private String name; private Short age; private BigDecimal balance; public Long getId() { return this.id; } public void setId(Long id) { this.id = id; } public String getUsername() { return this.username; } public void setUsername(String username) { this.username = username; } public String getName() { return this.name; } public void setName(String name) { this.name = name; } public Short getAge() { return this.age; } public void setAge(Short age) { this.age = age; } public BigDecimal getBalance() { return this.balance; } public void setBalance(BigDecimal balance) { this.balance = balance; } }
UserFeignClient类
package com.example.demo.feign; import org.springframework.cloud.netflix.feign.FeignClient; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import com.example.demo.entity.User; ////声明式服务 @FeignClient("spring-cloud-user") // 调用哪个服务的名称 public interface UserFeignClient { @RequestMapping(value = "/user/{id}", method = RequestMethod.GET) public User findById(@PathVariable("id") Long id); // 1. @GetMapping不支持 2. // @PathVariable得设置value @RequestMapping(value = "/testPost", method = RequestMethod.POST) public User postUser(@RequestBody User user); // 该请求不会成功,只要参数是复杂对象,即使指定了是GET方法,feign依然会以POST方法进行发送请求 @RequestMapping(value = "/getUser", method = RequestMethod.GET) public User getUser(User user); }
SpringCloudOrderApplication类
package com.example.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.EnableEurekaClient; import org.springframework.cloud.netflix.feign.EnableFeignClients; /** * 声明式服务 * */ @SpringBootApplication @EnableEurekaClient @EnableFeignClients//表示为Fegin客户端 public class SpringCloudOrderApplication { public static void main(String[] args) { SpringApplication.run(SpringCloudOrderApplication.class, args); } }
application.yml配置
spring: application: name: spring-cloud-order-feign #微服务的名称 server: port: 7906 #微服务端口号 eureka: client: healthcheck: enabled: true # 开启健康检查 serviceUrl: defaultZone: http://user:123456@localhost:8761/eureka #服务eureka的URL地址 instance: prefer-ip-address: true # 解决第一次请求报超时异常的方案: hystrix: command: default: execution: isolation: thread: timeoutInMilliseconds: 5000 # 或者: # hystrix.command.default.execution.timeout.enabled: false # 或者: # 超时的issue:https://github.com/spring-cloud/spring-cloud-netflix/issues/768 # 超时的解决方案: http://stackoverflow.com/questions/27375557/hystrix-command-fails-with-timed-out-and-no-fallback-available # hystrix配置: https://github.com/Netflix/Hystrix/wiki/Configuration#execution.isolation.thread.timeoutInMilliseconds
pom.xml配置
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example.demo</groupId> <artifactId>spring-cloud-order_feign</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>spring-cloud-order_feign</name> <description>spring-cloud-order_feign</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.4.1.RELEASE</version> </parent> <properties> <!-- 文件拷贝时的编码 --> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <!-- 编译时的编码 --> <maven.compiler.encoding>UTF-8</maven.compiler.encoding> <!-- jdk版本 --> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- 注册中心 --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> </dependency> <!-- 这个库让我们可以访问应用的很多信息,包括:/env、/info、/metrics、/health等 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <!-- 添加声明式feign依赖 --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-feign</artifactId> </dependency> <!-- 用于热部署 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> </dependency> </dependencies> <dependencyManagement> <dependencies> <!-- 版本依赖管理,故之后添加依赖无需指定version --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Camden.SR2</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <plugins> <!-- 用以为integration-test提供支持。 --> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
相关文章推荐
- 笔记:Spring Cloud Feign 声明式服务调用
- 【Spring Cloud】--声明式服务调用Feign
- Spring Cloud 入门教程(六): 用声明式REST客户端Feign调用远端HTTP服务
- SpringCloud之声明式服务调用Spring Cloud Feign实例
- Spring Cloud Feign 声明式服务调用
- 干货分享微服务spring-cloud(5.声明式服务调用feign)
- SpringCloud零基础上手(四)——服务发现以及Feign(声明式RESTful服务调用)
- Spring Cloud中声明式服务调用Feign
- 【图文经典版】声明式调用服务SpringCloud之Feign实例讲解
- Spring Cloud 声明式服务调用 Feign
- spring cloud Feign(声明式服务调用)
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- Spring Cloud微服务(7)之Feign服务之间调用
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- Spring Cloud Feign作为HTTP客户端调用远程HTTP服务,feign熔断器
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- 【微服务】之五:轻松搞定SpringCloud微服务-调用远程组件Feign
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- spring cloud : 简化远程调用 (声明式远程调用Feign)
- spring cloud eureka服务调用出现feign.codec.EncodeException: Could not write request: no suitable HttpMessa