Java-NIO(二):缓冲区(Buffer)的数据存取
2017-12-18 16:55
453 查看
缓冲区(Buffer): 一个用于特定基本数据类行的容器。有java.nio包定义的,所有缓冲区都是抽象类Buffer的子类。 Java NIO中的Buffer主要用于与NIO通道进行交互,数据是从通道读入到缓冲区,从缓冲区写入通道中的。 Buffer就像一个数组,可以保存多个相同类型的数据。根据类型不同(boolean除外),有以下Buffer常用子类:ByteBufferCharBufferShortBufferIntBufferLongBufferFloatBufferDoubleBuffer上述Buffer类他们都采用相似的方法进行管理数据,只是各自管理的数据类型不同而已,都是通过以下方法获取一个Buffer对象:
[b] Buffer常用函数测试:[/b]1package com.dx.nios;
static XxxBuffer allocate(int capacity)创建一个容量为capacity的XxxBuffer对象。[b]Buffer中的重要概念:[/b]1)容量(capacity):表示Buffer最大数据容量,缓冲区容量不能为负,并且建立后不能修改。2)限制(limit):第一个不应该读取或者写入的数据的索引,即位于limit后的数据不可以读写。缓冲区的限制不能为负,并且不能大于其容量(capacity)。3)位置(position):下一个要读取或写入的数据的索引。缓冲区的位置不能为负,并且不能大于其限制(limit)。4)标记(mark)与重置(reset):标记是一个索引,通过Buffer中的mark()方法指定Buffer中一个特定的position,之后可以通过调用reset()方法恢复到这个position。 注意:0<=mark<=position<=capacity测试代码:1package com.dx.nios;
2 3 import java.nio.ByteBuffer; 4 5 import org.junit.Test; 6 7 public class BufferTest { 8 9 @Test 10 public void TestBuffer() { 11 ByteBuffer byteBuffer = ByteBuffer.allocate(10); 12 13 System.out.println("------------allocate------------------"); 14 System.out.println(byteBuffer.position()); 15 System.out.println(byteBuffer.limit()); 16 System.out.println(byteBuffer.capacity()); 17 18 19 byteBuffer.put("abcde".getBytes()); 20 21 System.out.println("------------put------------------"); 22 System.out.println(byteBuffer.position()); 23 System.out.println(byteBuffer.limit()); 24 System.out.println(byteBuffer.capacity()); 25 26 byteBuffer.flip(); 27 28 System.out.println("------------flip------------------"); 29 System.out.println(byteBuffer.position()); 30 System.out.println(byteBuffer.limit()); 31 System.out.println(byteBuffer.capacity()); 32 33 } 34 }输出结果:
------------allocate------------------ 0 10 10 ------------put------------------ 5 10 10 ------------flip------------------ 0 5 10分析:

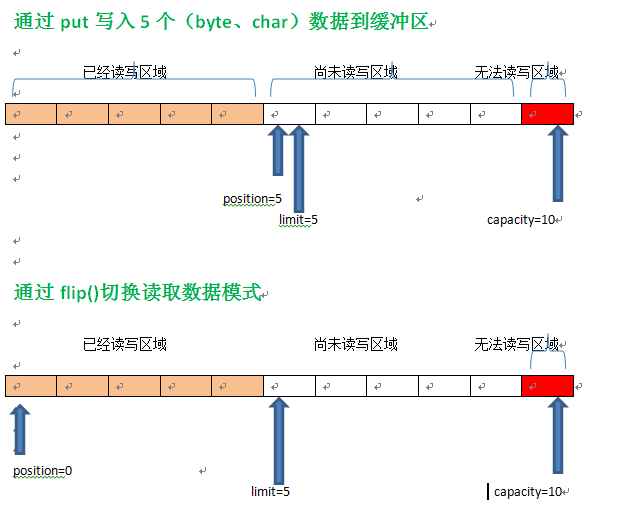
23 import java.nio.ByteBuffer;45 import org.junit.Test;67 public class BufferTest {89 @Test10 public void TestBuffer() {11 // 1.使用allocate()申请10个字节的缓冲区12 ByteBuffer byteBuffer = ByteBuffer.allocate(10);13 System.out.println("------------allocate------------------");14 System.out.println(byteBuffer.position());15 System.out.println(byteBuffer.limit());16 System.out.println(byteBuffer.capacity());1718 // 2.使用put()存放5个字节到缓冲区19 byteBuffer.put("abcde".getBytes());20 System.out.println("------------put------------------");21 System.out.println(byteBuffer.position());22 System.out.println(byteBuffer.limit());23 System.out.println(byteBuffer.capacity());2425 // 3.切换到读取数据模式26 byteBuffer.flip();27 System.out.println("------------flip------------------");28 System.out.println(byteBuffer.position());29 System.out.println(byteBuffer.limit());30 System.out.println(byteBuffer.capacity());3132 // 4.从缓冲区中读取数据33 System.out.println("------------get------------------");34 byte[] bytes = new byte[byteBuffer.limit()];35 byteBuffer.get(bytes);36 System.out.println(new String(bytes, 0, bytes.length));37 System.out.println(byteBuffer.position());38 System.out.println(byteBuffer.limit());39 System.out.println(byteBuffer.capacity());4041 // 5.设置为可重复读取42 System.out.println("------------rewind------------------");43 byteBuffer.rewind();44 System.out.println(byteBuffer.position());45 System.out.println(byteBuffer.limit());46 System.out.println(byteBuffer.capacity());47 byte[] bytes2 = new byte[byteBuffer.limit()];48 byteBuffer.get(bytes2);49 System.out.println(new String(bytes2, 0, bytes2.length));50 System.out.println(byteBuffer.position());51 System.out.println(byteBuffer.limit());52 System.out.println(byteBuffer.capacity());5354 // 6。clear清空缓存区,但是内容没有被清掉,还存在。只不过这些数据状态为被遗忘状态。55 System.out.println("------------clear------------------");56 byteBuffer.clear();57 System.out.println(byteBuffer.position());58 System.out.println(byteBuffer.limit());59 System.out.println(byteBuffer.capacity());60 byte[] bytes3 = new byte[10];61 byteBuffer.get(bytes3);62 System.out.println(new String(bytes3, 0, bytes3.length));63 }64 }输出:1 ------------allocate------------------
2 03 104 105 ------------put------------------6 57 108 109 ------------flip------------------10 011 512 1013 ------------get------------------14 abcde15 516 517 1018 ------------rewind------------------19 020 521 1022 abcde23 524 525 1026 ------------clear------------------27 028 1029 1030 abcde[b]mark与reset的用法:[/b] 1@Test
2 public void testMark() {3 ByteBuffer byteBuffer = ByteBuffer.allocate(1024);4 byteBuffer.put("abcde".getBytes());5 byteBuffer.flip();67 byte[] bytes = new byte[byteBuffer.limit()];8 byteBuffer.get(bytes, 0, 2);9 System.out.println(new String(bytes, 0, bytes.length));1011 System.out.println(byteBuffer.position());12 System.out.println(byteBuffer.limit());13 System.out.println(byteBuffer.capacity());1415 byteBuffer.mark();16 System.out.println("---------mark----------");1718 byteBuffer.get(bytes, 0, 2);19 System.out.println(new String(bytes, 0, bytes.length));2021 System.out.println(byteBuffer.position());22 System.out.println(byteBuffer.limit());23 System.out.println(byteBuffer.capacity());2425 byteBuffer.reset();26 System.out.println("---------reset----------");2728 System.out.println(byteBuffer.position());29 System.out.println(byteBuffer.limit());30 System.out.println(byteBuffer.capacity());31 }打印信息:ab251024---------mark----------cd451024---------reset----------251024java.nio.Buffer.java
/** Copyright (c) 2000, 2013, Oracle and/or its affiliates. All rights reserved.* ORACLE PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.*********************/package java.nio;import java.util.Spliterator;/*** A container for data of a specific primitive type.** <p> A buffer is a linear, finite sequence of elements of a specific* primitive type. Aside from its content, the essential properties of a* buffer are its capacity, limit, and position: </p>** <blockquote>** <p> A buffer's <i>capacity</i> is the number of elements it contains. The* capacity of a buffer is never negative and never changes. </p>** <p> A buffer's <i>limit</i> is the index of the first element that should* not be read or written. A buffer's limit is never negative and is never* greater than its capacity. </p>** <p> A buffer's <i>position</i> is the index of the next element to be* read or written. A buffer's position is never negative and is never* greater than its limit. </p>** </blockquote>** <p> There is one subclass of this class for each non-boolean primitive type.*** <h2> Transferring data </h2>** <p> Each subclass of this class defines two categories of <i>get</i> and* <i>put</i> operations: </p>** <blockquote>** <p> <i>Relative</i> operations read or write one or more elements starting* at the current position and then increment the position by the number of* elements transferred. If the requested transfer exceeds the limit then a* relative <i>get</i> operation throws a {@link BufferUnderflowException}* and a relative <i>put</i> operation throws a {@link* BufferOverflowException}; in either case, no data is transferred. </p>** <p> <i>Absolute</i> operations take an explicit element index and do not* affect the position. Absolute <i>get</i> and <i>put</i> operations throw* an {@link IndexOutOfBoundsException} if the index argument exceeds the* limit. </p>** </blockquote>** <p> Data may also, of course, be transferred in to or out of a buffer by the* I/O operations of an appropriate channel, which are always relative to the* current position.*** <h2> Marking and resetting </h2>** <p> A buffer's <i>mark</i> is the index to which its position will be reset* when the {@link #reset reset} method is invoked. The mark is not always* defined, but when it is defined it is never negative and is never greater* than the position. If the mark is defined then it is discarded when the* position or the limit is adjusted to a value smaller than the mark. If the* mark is not defined then invoking the {@link #reset reset} method causes an* {@link InvalidMarkException} to be thrown.*** <h2> Invariants </h2>** <p> The following invariant holds for the mark, position, limit, and* capacity values:** <blockquote>* <tt>0</tt> <tt><=</tt>* <i>mark</i> <tt><=</tt>* <i>position</i> <tt><=</tt>* <i>limit</i> <tt><=</tt>* <i>capacity</i>* </blockquote>** <p> A newly-created buffer always has a position of zero and a mark that is* undefined. The initial limit may be zero, or it may be some other value* that depends upon the type of the buffer and the manner in which it is* constructed. Each element of a newly-allocated buffer is initialized* to zero.*** <h2> Clearing, flipping, and rewinding </h2>** <p> In addition to methods for accessing the position, limit, and capacity* values and for marking and resetting, this class also defines the following* operations upon buffers:** <ul>** <li><p> {@link #clear} makes a buffer ready for a new sequence of* channel-read or relative <i>put</i> operations: It sets the limit to the* capacity and the position to zero. </p></li>** <li><p> {@link #flip} makes a buffer ready for a new sequence of* channel-write or relative <i>get</i> operations: It sets the limit to the* current position and then sets the position to zero. </p></li>** <li><p> {@link #rewind} makes a buffer ready for re-reading the data that* it already contains: It leaves the limit unchanged and sets the position* to zero. </p></li>** </ul>*** <h2> Read-only buffers </h2>** <p> Every buffer is readable, but not every buffer is writable. The* mutation methods of each buffer class are specified as <i>optional* operations</i> that will throw a {@link ReadOnlyBufferException} when* invoked upon a read-only buffer. A read-only buffer does not allow its* content to be changed, but its mark, position, and limit values are mutable.* Whether or not a buffer is read-only may be determined by invoking its* {@link #isReadOnly isReadOnly} method.*** <h2> Thread safety </h2>** <p> Buffers are not safe for use by multiple concurrent threads. If a* buffer is to be used by more than one thread then access to the buffer* should be controlled by appropriate synchronization.*** <h2> Invocation chaining </h2>** <p> Methods in this class that do not otherwise have a value to return are* specified to return the buffer upon which they are invoked. This allows* method invocations to be chained; for example, the sequence of statements** <blockquote><pre>* b.flip();* b.position(23);* b.limit(42);</pre></blockquote>** can be replaced by the single, more compact statement** <blockquote><pre>* b.flip().position(23).limit(42);</pre></blockquote>*** @author Mark Reinhold* @author JSR-51 Expert Group* @since 1.4*/public abstract class Buffer {/*** The characteristics of Spliterators that traverse and split elements* maintained in Buffers.*/static final int SPLITERATOR_CHARACTERISTICS =Spliterator.SIZED | Spliterator.SUBSIZED | Spliterator.ORDERED;// Invariants: mark <= position <= limit <= capacityprivate int mark = -1;private int position = 0;private int limit;private int capacity;// Used only by direct buffers// NOTE: hoisted here for speed in JNI GetDirectBufferAddresslong address;// Creates a new buffer with the given mark, position, limit, and capacity,// after checking invariants.//Buffer(int mark, int pos, int lim, int cap) { // package-privateif (cap < 0)throw new IllegalArgumentException("Negative capacity: " + cap);this.capacity = cap;limit(lim);position(pos);if (mark >= 0) {if (mark > pos)throw new IllegalArgumentException("mark > position: ("+ mark + " > " + pos + ")");this.mark = mark;}}/*** Returns this buffer's capacity.** @return The capacity of this buffer*/public final int capacity() {return capacity;}/*** Returns this buffer's position.** @return The position of this buffer*/public final int position() {return position;}/*** Sets this buffer's position. If the mark is defined and larger than the* new position then it is discarded.** @param newPosition* The new position value; must be non-negative* and no larger than the current limit** @return This buffer** @throws IllegalArgumentException* If the preconditions on <tt>newPosition</tt> do not hold*/public final Buffer position(int newPosition) {if ((newPosition > limit) || (newPosition < 0))throw new IllegalArgumentException();position = newPosition;if (mark > position) mark = -1;return this;}/*** Returns this buffer's limit.** @return The limit of this buffer*/public final int limit() {return limit;}/*** Sets this buffer's limit. If the position is larger than the new limit* then it is set to the new limit. If the mark is defined and larger than* the new limit then it is discarded.** @param newLimit* The new limit value; must be non-negative* and no larger than this buffer's capacity** @return This buffer** @throws IllegalArgumentException* If the preconditions on <tt>newLimit</tt> do not hold*/public final Buffer limit(int newLimit) {if ((newLimit > capacity) || (newLimit < 0))throw new IllegalArgumentException();limit = newLimit;if (position > limit) position = limit;if (mark > limit) mark = -1;return this;}/*** Sets this buffer's mark at its position.** @return This buffer*/public final Buffer mark() {mark = position;return this;}/*** Resets this buffer's position to the previously-marked position.** <p> Invoking this method neither changes nor discards the mark's* value. </p>** @return This buffer** @throws InvalidMarkException* If the mark has not been set*/public final Buffer reset() {int m = mark;if (m < 0)throw new InvalidMarkException();position = m;return this;}/*** Clears this buffer. The position is set to zero, the limit is set to* the capacity, and the mark is discarded.** <p> Invoke this method before using a sequence of channel-read or* <i>put</i> operations to fill this buffer. For example:** <blockquote><pre>* buf.clear(); // Prepare buffer for reading* in.read(buf); // Read data</pre></blockquote>** <p> This method does not actually erase the data in the buffer, but it* is named as if it did because it will most often be used in situations* in which that might as well be the case. </p>** @return This buffer*/public final Buffer clear() {position = 0;limit = capacity;mark = -1;return this;}/*** Flips this buffer. The limit is set to the current position and then* the position is set to zero. If the mark is defined then it is* discarded.** <p> After a sequence of channel-read or <i>put</i> operations, invoke* this method to prepare for a sequence of channel-write or relative* <i>get</i> operations. For example:** <blockquote><pre>* buf.put(magic); // Prepend header* in.read(buf); // Read data into rest of buffer* buf.flip(); // Flip buffer* out.write(buf); // Write header + data to channel</pre></blockquote>** <p> This method is often used in conjunction with the {@link* java.nio.ByteBuffer#compact compact} method when transferring data from* one place to another. </p>** @return This buffer*/public final Buffer flip() {limit = position;position = 0;mark = -1;return this;}/*** Rewinds this buffer. The position is set to zero and the mark is* discarded.** <p> Invoke this method before a sequence of channel-write or <i>get</i>* operations, assuming that the limit has already been set* appropriately. For example:** <blockquote><pre>* out.write(buf); // Write remaining data* buf.rewind(); // Rewind buffer* buf.get(array); // Copy data into array</pre></blockquote>** @return This buffer*/public final Buffer rewind() {position = 0;mark = -1;return this;}/*** Returns the number of elements between the current position and the* limit.** @return The number of elements remaining in this buffer*/public final int remaining() {return limit - position;}/*** Tells whether there are any elements between the current position and* the limit.** @return <tt>true</tt> if, and only if, there is at least one element* remaining in this buffer*/public final boolean hasRemaining() {return position < limit;}/*** Tells whether or not this buffer is read-only.** @return <tt>true</tt> if, and only if, this buffer is read-only*/public abstract boolean isReadOnly();/*** Tells whether or not this buffer is backed by an accessible* array.** <p> If this method returns <tt>true</tt> then the {@link #array() array}* and {@link #arrayOffset() arrayOffset} methods may safely be invoked.* </p>** @return <tt>true</tt> if, and only if, this buffer* is backed by an array and is not read-only** @since 1.6*/public abstract boolean hasArray();/*** Returns the array that backs this* buffer <i>(optional operation)</i>.** <p> This method is intended to allow array-backed buffers to be* passed to native code more efficiently. Concrete subclasses* provide more strongly-typed return values for this method.** <p> Modifications to this buffer's content will cause the returned* array's content to be modified, and vice versa.** <p> Invoke the {@link #hasArray hasArray} method before invoking this* method in order to ensure that this buffer has an accessible backing* array. </p>** @return The array that backs this buffer** @throws ReadOnlyBufferException* If this buffer is backed by an array but is read-only** @throws UnsupportedOperationException* If this buffer is not backed by an accessible array** @since 1.6*/public abstract Object array();/*** Returns the offset within this buffer's backing array of the first* element of the buffer <i>(optional operation)</i>.** <p> If this buffer is backed by an array then buffer position <i>p</i>* corresponds to array index <i>p</i> + <tt>arrayOffset()</tt>.** <p> Invoke the {@link #hasArray hasArray} method before invoking this* method in order to ensure that this buffer has an accessible backing* array. </p>** @return The offset within this buffer's array* of the first element of the buffer** @throws ReadOnlyBufferException* If this buffer is backed by an array but is read-only** @throws UnsupportedOperationException* If this buffer is not backed by an accessible array** @since 1.6*/public abstract int arrayOffset();/*** Tells whether or not this buffer is* <a href="ByteBuffer.html#direct"><i>direct</i></a>.** @return <tt>true</tt> if, and only if, this buffer is direct** @since 1.6*/public abstract boolean isDirect();// -- Package-private methods for bounds checking, etc. --/*** Checks the current position against the limit, throwing a {@link* BufferUnderflowException} if it is not smaller than the limit, and then* increments the position.** @return The current position value, before it is incremented*/final int nextGetIndex() { // package-privateif (position >= limit)throw new BufferUnderflowException();return position++;}final int nextGetIndex(int nb) { // package-privateif (limit - position < nb)throw new BufferUnderflowException();int p = position;position += nb;return p;}/*** Checks the current position against the limit, throwing a {@link* BufferOverflowException} if it is not smaller than the limit, and then* increments the position.** @return The current position value, before it is incremented*/final int nextPutIndex() { // package-privateif (position >= limit)throw new BufferOverflowException();return position++;}final int nextPutIndex(int nb) { // package-privateif (limit - position < nb)throw new BufferOverflowException();int p = position;position += nb;return p;}/*** Checks the given index against the limit, throwing an {@link* IndexOutOfBoundsException} if it is not smaller than the limit* or is smaller than zero.*/final int checkIndex(int i) { // package-privateif ((i < 0) || (i >= limit))throw new IndexOutOfBoundsException();return i;}final int checkIndex(int i, int nb) { // package-privateif ((i < 0) || (nb > limit - i))throw new IndexOutOfBoundsException();return i;}final int markValue() { // package-privatereturn mark;}final void truncate() { // package-privatemark = -1;position = 0;limit = 0;capacity = 0;}final void discardMark() { // package-privatemark = -1;}static void checkBounds(int off, int len, int size) { // package-privateif ((off | len | (off + len) | (size - (off + len))) < 0)throw new IndexOutOfBoundsException();}}原文地址:http://www.cnblogs.com/yy3b2007com/p/7261025.html
相关文章推荐
- Java-NIO(二):缓冲区(Buffer)的数据存取
- NIO缓冲区(Buffer)的数据存取
- Java nio 学习笔记(一) Buffer(缓冲区)与Channel(通道)的相关知识
- java NIO入门简介 Buffer(缓冲区)与Channel(通道)的相关知识
- Java NIO —— Buffer(缓冲区)
- java.nio.ByteBuffer 类 缓冲区
- java.nio.ByteBuffer 类 缓冲区
- java.nio.ByteBuffer 类 缓冲区
- java.nio.ByteBuffer 类 缓冲区
- Java NIO缓冲区(Buffer)的数据存取
- java.nio.ByteBuffer 类 缓冲区
- java.nio.Buffer缓冲区源码解析
- java.nio.Buffer缓冲区基础
- Java的NIO之详解通道Channel和字节缓冲区ByteBuffer
- Java NIO -- 缓冲区(Buffer)的数据存取
- java nio buffer读取数据乱码问题
- Java nio 学习笔记(一) Buffer(缓冲区)与Channel(通道)的相关知识
- Java nio 学习笔记(一) Buffer(缓冲区)与Channel(通道)的相关知识
- 2. NIO_缓冲区(Buffer)的数据存取
- 【转】java.nio.ByteBuffer 类 缓冲区