Leetcode62-63 Unique Paths
2017-12-11 09:57
387 查看
Unique Paths
题目
A robot is located at the top-left corner of a m x n grid (marked ‘Start’ in the diagram below).The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked ‘Finish’ in the diagram below).
How many possible unique paths are there?
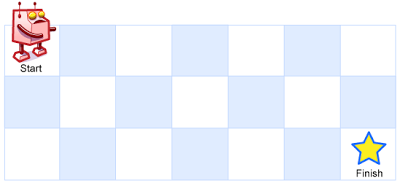
Above is a 3 x 7 grid. How many possible unique paths are there?
Note: m and n will be at most 100.
分析
这是一个关于动态规划的计算路径的问题,一个机器人只可以向下和向右行走,问最终到达终点有多少条路径。可以观察到,对于每一个格子[i, j],从起始位置即[0, 0]到达该点的路径数等于到达[i - 1][j]的路径数加上到达[i][j - 1]的路径数。注意边界情况即可。
因此,定义result[i][j]表示从起始点到当前点的路径数,可以写出状态转移方程如下:
result[0][j] = 1; // j < n result[i][0] = 1; // i < m result[i][j] = result[i - 1][j] + result[i][j - 1]; // 0 < i < m && 0 < j < n
最终的结果即是result[m - 1][n - 1]
代码:
class Solution { public: int uniquePaths(int m, int n) { if (m == 0 || n == 0) return 0; vector<int> temp(n, 0); vector<vector<int>> result(m, temp); for (int i = 0; i < m; i++) { for (int j = 0; j < n; j++) { if (i == 0 && j == 0) { result[i][j] = 1; continue; } int leftRoute = 0, topRoute = 0; if (i - 1 >= 0) { leftRoute = result[i - 1][j]; } if (j - 1 >= 0) { topRoute = result[i][j - 1]; } result[i][j] = leftRoute + topRoute; } } return result[m - 1][n - 1]; } };
改进
因为每一个各自的路径数只依赖与result[i - 1][j] 和 result[i][j - 1]。因此,可以只用一维数组来实现状态的转移。代码如下:
class Solution { public: int uniquePaths(int m, int n) { if (m == 0 || n == 0) return 0; vector<int> result(n, 1); for (int i = 1; i < m; i++) { for (int j = 1; j < n; j++) { result[j] = result[j] + result[j - 1]; } } return result[n - 1]; } };
运行结果
Unique Paths II
题目
Follow up for “Unique Paths”:Now consider if some obstacles are added to the grids. How many unique paths would there be?
An obstacle and empty space is marked as 1 and 0 respectively in the grid.
For example,
There is one obstacle in the middle of a 3x3 grid as illustrated below.
[ [0,0,0], [0,1,0], [0,0,0] ]
The total number of unique paths is 2.
Note: m and n will be at most 100.
分析
题目是从第一道题继承而来,只是,对题目的解增加了限制条件,即引入了障碍,如果一个格子中有障碍物,那么这个格子不可达。延续同一样的思路,略加变化,可以写出状态转移方程如下:
result[i][0] = result[i - 1][0] // i < n result[0][j] = result[0][j - 1] // j < m result[i][j] = obstacleGrid[i][j]? 0 : result[i - 1][j] + result[i][j - 1] // i > 0 && j > 0
代码:
class Solution { public: int uniquePathsWithObstacles(vector<vector<int>>& obstacleGrid) { int m = obstacleGrid.size(), n = obstacleGrid[0].size(); int result[m] ; memset(result, 0, sizeof(int) * m * n); for (int i = 0; i < n; i++) { if (obstacleGrid[0][i] == 1) { break; } result[0][i] = 1; } for (int i = 0; i < m; i++) { if (obstacleGrid[i][0] == 1) { break; } result[i][0] = 1; } for (int i = 1; i < m; i++) { for (int j = 1; j < n; j++) { if (obstacleGrid[i][j] == 1) result[i][j] = 0; else result[i][j] = result[i - 1][j] + result[i][j - 1]; } } return result[m - 1][n - 1]; } };
改进
改进的思路与上一题相同,是对于空间复杂度上的改进,可以只用一维数组来记录路径的信息。因为每一个格子只依赖于[i - 1][j] 和 [i][j - 1]。代码如下:
class Solution { public: int uniquePathsWithObstacles(vector<vector<int>>& obstacleGrid) { int m = obstacleGrid.size(), n = obstacleGrid[0].size(); vector<int> result(n, 0); for (int i = 0; i < n; i++) { if (obstacleGrid[0][i] == 1) break; result[i] = 1; } if (m > 1) { result[0] = obstacleGrid[1][0]? 0 : result[0]; } for (int i = 1; i < m; i++) { for (int j = 0; j < n; j++) { if (obstacleGrid[i][j] == 1) result[j] = 0; else if (j > 0 ) result[j] = result[j] + result[j - 1]; else result[j] = result[j]; } } return result[n - 1]; } };
运行结果
相关文章推荐
- leetcode-62 Unique Paths
- LeetCode 62 Unique Paths 解题报告
- LeetCode 62 - Unique Paths
- 63 leetcode - Unique Paths
- Leetcode:62. Unique Paths
- [LeetCode]: 62: Unique Paths
- LeetCode62——Unique Paths
- 62/63 Unique Paths
- leetcode---62. Unique Paths
- LeetCode_62---Unique Paths
- Leetcode 62 Unique Paths
- LeetCode-62-Unique Paths
- 【LEETCODE】62-Unique Paths
- Leetcode62 Unique Paths
- leetcode(62). Unique Paths
- leetcode 62:Unique Paths
- leetcode || 62、Unique Paths
- LeetCode_62、63、64三题(动态规划)
- [Leetcode 26] 62 Unique Paths
- leetcode 62: Unique Paths