第十五周项目一 - 验证算法之直接插入、希尔、冒泡排序
2017-12-07 10:31
267 查看
【项目1 - 验证算法(1)】
问题描述:用序列{57, 40, 38, 11, 13, 34, 48, 75, 6, 19, 9, 7}作为测试数据,运行并本周视频中所讲过的算法对应 程序,观察运
行结果并深刻领会算法的思路和实现方法:
(1)直接插入排序;(2)希尔排序;(3)冒泡排序
程序及代码:
(1)直接插入排序
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void InsertSort(RecType R[],int n) //对R[0..n-1]按递增有序进行直接插入排序
{
int i,j;
RecType tmp;
for (i=1; i<n; i++)
{
tmp=R[i];
j=i-1; //从右向左在有序区R[0..i-1]中找R[i]的插入位置
while (j>=0 && tmp.key<R[j].key)
{
R[j+1]=R[j]; //将关键字大于R[i].key的记录后移
j--;
}
R[j+1]=tmp; //在j+1处插入R[i]
}
}
int main()
{
int i,n=10;
RecType R[MaxSize];
KeyType a[]= {9,8,7,6,5,4,3,2,1,0};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
InsertSort(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}
运行结果:
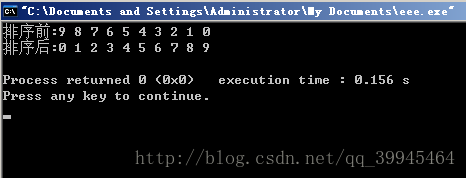
(2)希尔排序
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void ShellSort(RecType R[],int n) //希尔排序算法
{
int i,j,gap;
RecType tmp;
gap=n/2; //增量置初值
while (gap>0)
{
for (i=gap; i<n; i++) //对所有相隔gap位置的所有元素组进行排序
{
tmp=R[i];
j=i-gap;
while (j>=0 && tmp.key<R[j].key)//对相隔gap位置的元素组进行排序
{
R[j+gap]=R[j];
j=j-gap;
}
R[j+gap]=tmp;
j=j-gap;
}
gap=gap/2; //减小增量
}
}
int main()
{
int i,n=11;
RecType R[MaxSize];
KeyType a[]= {16,25,12,30,47,11,23,36,9,18,31};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
ShellSort(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}
运行结果:
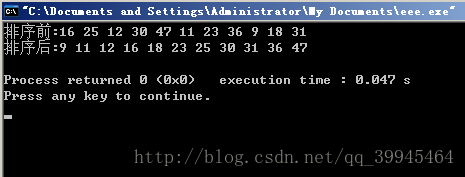
(3)冒泡排序
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void BubbleSort(RecType R[],int n)
{
int i,j,k;
RecType tmp;
for (i=0; i<n-1; i++)
{
for (j=n-1; j>i; j--) //比较,找出本趟最小关键字的记录
if (R[j].key<R[j-1].key)
{
tmp=R[j]; //R[j]与R[j-1]进行交换,将最小关键字记录前移
R[j]=R[j-1];
R[j-1]=tmp;
}
printf("i=%d: ",i);
for (k=0; k<n; k++)
printf("%d ",R[k].key);
printf("\n");
}
}
int main()
{
int i,n=10;
RecType R[MaxSize];
KeyType a[]= {9,8,7,6,5,4,3,2,1,0};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
BubbleSort(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}
运行结果:
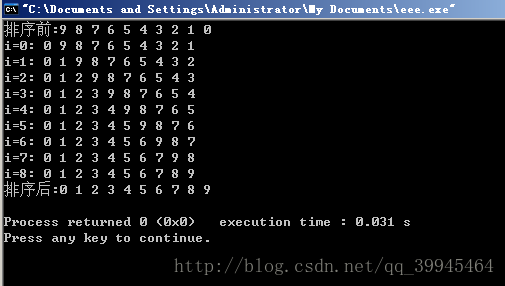
(3)改进后冒泡排序
改进原因:实际操作上,一旦算法中的某一趟比较时不出现任何元素交换,说明已经排好序了。
改进后:一趟冒泡结束后没有交换,则结束排序过程
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void BubbleSort1(RecType R[],int n)
{
int i,j,k,exchange;
RecType tmp;
for (i=0; i<n-1; i++)
{
exchange=0;
for (j=n-1; j>i; j--) //比较,找出最小关键字的记录
if (R[j].key<R[j-1].key)
{
tmp=R[j]; //R[j]与R[j-1]进行交换,将最小关键字记录前移
R[j]=R[j-1];
R[j-1]=tmp;
exchange=1;
}
printf("i=%d: ",i);
for (k=0; k<n; k++)
printf("%d ",R[k].key);
printf("\n");
if (exchange==0) //中途结束算法
return;
}
}
int main()
{
int i,n=10;
RecType R[MaxSize];
KeyType a[]= {0,1,7,2,5,4,3,6,8,9};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
BubbleSort1(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}运行结果:
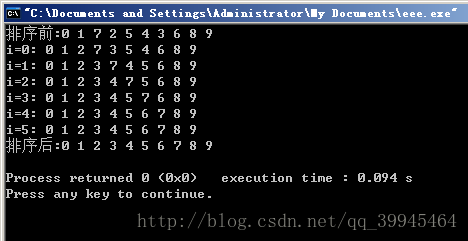
知识点总结:
学习了直接插入排序、希尔排序、冒泡排序算法,并了解了不同排序算法的异同
心得体会:
这三种排序算法比较容易理解,上课时画出排序图,代码也能较好理解
问题描述:用序列{57, 40, 38, 11, 13, 34, 48, 75, 6, 19, 9, 7}作为测试数据,运行并本周视频中所讲过的算法对应 程序,观察运
行结果并深刻领会算法的思路和实现方法:
(1)直接插入排序;(2)希尔排序;(3)冒泡排序
程序及代码:
(1)直接插入排序
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void InsertSort(RecType R[],int n) //对R[0..n-1]按递增有序进行直接插入排序
{
int i,j;
RecType tmp;
for (i=1; i<n; i++)
{
tmp=R[i];
j=i-1; //从右向左在有序区R[0..i-1]中找R[i]的插入位置
while (j>=0 && tmp.key<R[j].key)
{
R[j+1]=R[j]; //将关键字大于R[i].key的记录后移
j--;
}
R[j+1]=tmp; //在j+1处插入R[i]
}
}
int main()
{
int i,n=10;
RecType R[MaxSize];
KeyType a[]= {9,8,7,6,5,4,3,2,1,0};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
InsertSort(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}
运行结果:
(2)希尔排序
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void ShellSort(RecType R[],int n) //希尔排序算法
{
int i,j,gap;
RecType tmp;
gap=n/2; //增量置初值
while (gap>0)
{
for (i=gap; i<n; i++) //对所有相隔gap位置的所有元素组进行排序
{
tmp=R[i];
j=i-gap;
while (j>=0 && tmp.key<R[j].key)//对相隔gap位置的元素组进行排序
{
R[j+gap]=R[j];
j=j-gap;
}
R[j+gap]=tmp;
j=j-gap;
}
gap=gap/2; //减小增量
}
}
int main()
{
int i,n=11;
RecType R[MaxSize];
KeyType a[]= {16,25,12,30,47,11,23,36,9,18,31};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
ShellSort(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}
运行结果:
(3)冒泡排序
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void BubbleSort(RecType R[],int n)
{
int i,j,k;
RecType tmp;
for (i=0; i<n-1; i++)
{
for (j=n-1; j>i; j--) //比较,找出本趟最小关键字的记录
if (R[j].key<R[j-1].key)
{
tmp=R[j]; //R[j]与R[j-1]进行交换,将最小关键字记录前移
R[j]=R[j-1];
R[j-1]=tmp;
}
printf("i=%d: ",i);
for (k=0; k<n; k++)
printf("%d ",R[k].key);
printf("\n");
}
}
int main()
{
int i,n=10;
RecType R[MaxSize];
KeyType a[]= {9,8,7,6,5,4,3,2,1,0};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
BubbleSort(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}
运行结果:
(3)改进后冒泡排序
改进原因:实际操作上,一旦算法中的某一趟比较时不出现任何元素交换,说明已经排好序了。
改进后:一趟冒泡结束后没有交换,则结束排序过程
#include <stdio.h>
#define MaxSize 20
typedef int KeyType; //定义关键字类型
typedef char InfoType[10];
typedef struct //记录类型
{
KeyType key; //关键字项
InfoType data; //其他数据项,类型为InfoType
} RecType; //排序的记录类型定义
void BubbleSort1(RecType R[],int n)
{
int i,j,k,exchange;
RecType tmp;
for (i=0; i<n-1; i++)
{
exchange=0;
for (j=n-1; j>i; j--) //比较,找出最小关键字的记录
if (R[j].key<R[j-1].key)
{
tmp=R[j]; //R[j]与R[j-1]进行交换,将最小关键字记录前移
R[j]=R[j-1];
R[j-1]=tmp;
exchange=1;
}
printf("i=%d: ",i);
for (k=0; k<n; k++)
printf("%d ",R[k].key);
printf("\n");
if (exchange==0) //中途结束算法
return;
}
}
int main()
{
int i,n=10;
RecType R[MaxSize];
KeyType a[]= {0,1,7,2,5,4,3,6,8,9};
for (i=0; i<n; i++)
R[i].key=a[i];
printf("排序前:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
BubbleSort1(R,n);
printf("排序后:");
for (i=0; i<n; i++)
printf("%d ",R[i].key);
printf("\n");
return 0;
}运行结果:
知识点总结:
学习了直接插入排序、希尔排序、冒泡排序算法,并了解了不同排序算法的异同
心得体会:
这三种排序算法比较容易理解,上课时画出排序图,代码也能较好理解
相关文章推荐
- 第十五周项目1—验证算法(直接插入排序)
- 【第十五周项目1】验证算法——直接插入排序
- 第十五周 项目1--验证算法--(2)直接插入排序
- 第十五周--项目4算法验证直接插入排序
- 【第十五周项目1 - 验证算法之直接插入排序】
- 第16周项目1-验证算法(3)冒泡排序 直接插入排序
- 第十五周实践项目1—验证算法(2)直接插入排序
- 第十五周项目1(1)-验证算法(直接插入排序)
- 第十五周项目1-验证算法(2-直接插入排序)
- 第十五周项目1--验证算法--(2)直接插入排序
- 第十五周项目1 验证算法(1)插入排序之直接插入排序
- 第十五周项目1-验证算法(2)-直接插入排序
- 第十五周项目四 验证算法——直接插入排序
- 【第十五周项目1 - 验证算法之直接插入排序】
- 第十五周项目1-验证算法(2)直接插入排序
- 第十五周项目1(2)--验证直接插入排序算法
- 第十五周项目一(3)——验证算法之直接选择排序
- 第16周项目1-验证算法(1)直接插入排序
- 【第十五周项目1】验证算法——冒泡排序
- 第十六周上机实践—项目1(4)—验证算法 冒泡排序 直接选择排序