Python学习(十九)——CSV文件读写
2017-12-04 16:15
447 查看
CSV文件读写
输出:
打开w.csv文件可以看到其中的数据存放形式:
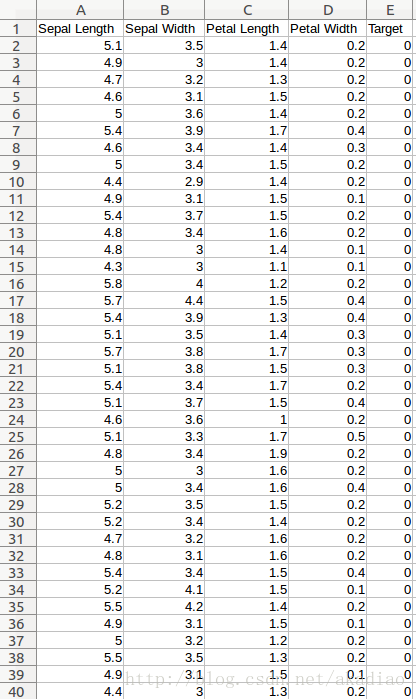
由于直接读取CSV文件内的数据时数据是以字符串类型存放在list内返回的,因此要将读取的list内的字符串转化为float数据,然后转换为矩阵,最后将其分离为data和target,以便于后期对其进行处理:
输出:
写入CSV文件
创建w.csv文件,并将iris数据写入#!/usr/bin/python # -*-coding:utf-8-*- import numpy as np import csv from sklearn.datasets import load_iris iris = load_iris() writer = csv.writer(file('w.csv', 'wb')) # 在首行写入对应数据名称 writer.writerow(['Sepal Length', 'Sepal Width', 'Petal Length', 'Petal Width', 'Target']) # 写入iris数据 for i in xrange(len(iris.data)): l = np.hstack((np.array(iris.data[i]), np.array(iris.target[i]))) writer.writerow(l) print l
输出:
[ 5.1 3.5 1.4 0.2 0. ] [ 4.9 3. 1.4 0.2 0. ] [ 4.7 3.2 1.3 0.2 0. ] [ 4.6 3.1 1.5 0.2 0. ] . . . [ 5.6 2.7 4.2 1.3 1. ] [ 5.7 3. 4.2 1.2 1. ] [ 5.7 2.9 4.2 1.3 1. ]
打开w.csv文件可以看到其中的数据存放形式:
读取CSV文件
读取w.csv数据由于直接读取CSV文件内的数据时数据是以字符串类型存放在list内返回的,因此要将读取的list内的字符串转化为float数据,然后转换为矩阵,最后将其分离为data和target,以便于后期对其进行处理:
# CSV文件读取 import numpy as np import csv l = [] with open('w.csv') as file: lines = csv.reader(file) for line in lines: l.append(line) # 去除第1行,即写入时添加的'Sepal Length', 'Sepal Width', 'Petal Length', 'Petal Width', 'Target' l.remove(l[0]) iris = [] for i in l: # 将list内的字符串转化为float数据 iris.append(map(float, i)) # 转换为矩阵 iris = np.mat(np.array(iris)) # 获得数据 data = iris[:,0:-1] # 获得数据labels target = iris[:,-1] print 'data:\n', data print 'target:\n', target
输出:
data: [[ 5.1 3.5 1.4 0.2] [ 4.9 3. 1.4 0.2] [ 4.7 3.2 1.3 0.2] . . . [ 6.5 3. 5.2 2. ] [ 6.2 3.4 5.4 2.3] [ 5.9 3. 5.1 1.8]] target: [[ 0.] [ 0.] [ 0.] . . . [ 2.] [ 2.] [ 2.]]
相关文章推荐
- python学习之路三(文件读写)
- python文件读写学习
- 文件操作注意事项 分类: python 小练习 python基础学习 open()文件读写 2013-12-03 11:05 532人阅读 评论(0) 收藏
- Python 学习笔记 (6)—— 读写文件
- python 读写 csv文件
- open()文件对象的seek、tell方法详解 分类: python基础学习 open()文件读写 python Module 2013-09-05 09:40 450人阅读 评论(0) 收藏
- 学习 Python 文件的读写
- Python学习笔记--CSV模块读写数据(转)
- Python读写csv文件
- python 学习正则与文件读写 从字符串里面找出 邮箱正则
- Python读写csv文件
- Python学习笔记--文件读写
- Learn Python The Hard Way学习(16) - 读写文件
- 【python学习笔记】pthon3.x中的文件读写操作
- Learn Python The Hard Way学习(16) - 读写文件
- Python读写csv文件
- Python CSV文件处理/读写及With as 用法
- Python读写csv文件
- python学习之路三(文件读写)
- csv模块学习:Python学习笔记—CSV模块读写数据