Python微框架web.py初印象
2017-11-23 13:14
281 查看
环境以及需要安装的软件以及模块
!!!本文注重实际操作以及问题解决,理论自补
对于pip的安装请参阅本人以前写过的博客
一个入门级web.py应用如下:
在命令行中运行以上程序:
好的习惯就是:对于各个项目要有自己的目录,并且易于区分
打开浏览器http://192.168.1.112:1234/,你会看到Hello, world!
模板文件的内容如下:
模板文件写好了,我们就要告诉应用去渲染了
在weeb.py的第一行之下添加:
修改下 URL 配置:
然后修改下 index.GET:
render.halo的halo是模板,也就是html文件的名称!!!
现在访问 /lockey 看看,显示如下:
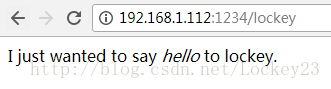
如果不手动输入名字显示如下:
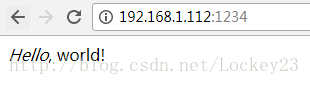
安装数据库驱动模块:
首先你需要创建一个数据库对象:
(根据自己的实际数据库状态,修改用户,密码,链接的数据库。 MySQL用户还需要把 dbn 定义改为 mysql。)
在你的库中创建一个简单的表:
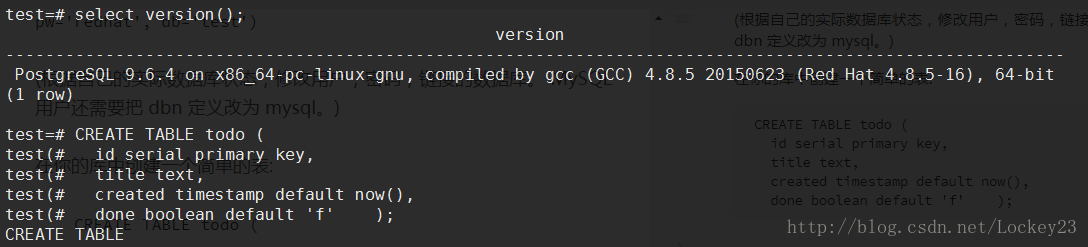
向数据库中插入数据:
我们回来继续编辑weeb.py ,把 index.GET 改成下面的样子,替换整个函数:
然后把URL列表改回来,只保留 /:
像这样编辑并替换 halo.html 的全部内容:
到目前为止weeb.py文件的内容如下:
运行应用然后测试数据库链接以及数据获取:
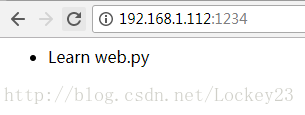
如果在这一步出现问题:
解决方法如下:
from stackoverflow:
通过表单向数据中插入数据:
在 index.html尾部添加:
然后把你的URL列表改为:
(你必须要非常小心那些逗号。如果你省略他们,Python会把所有字符串连接起来,变成 ‘/index/addadd’)
现在添加另一个类用来处理添加请求:
这个时候完整的weeb.py文件看起来是这样的:
模板文件看起来是这样的:
运行然后在浏览器中测试:
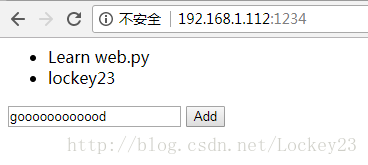
数据库测试:
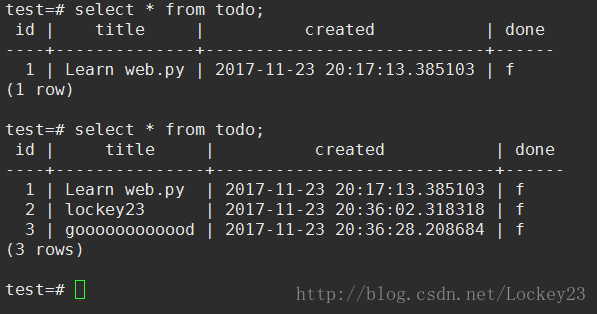
以下为一个示例:
[root@original weeb]# cat weeb.py
[root@original weeb]# cat templates/halo.html
运行测试:
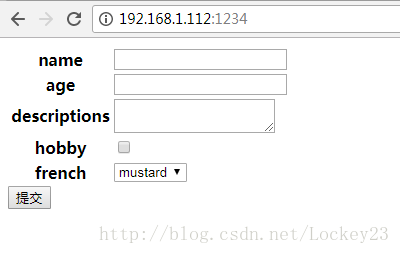
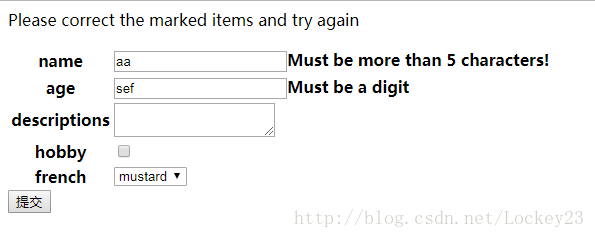
redhat 7.2 x86_64bit,IP:192.168.1.112 postgresql,psycopg2 web.py
!!!本文注重实际操作以及问题解决,理论自补
1、web.py安装测试
pip install web.py
对于pip的安装请参阅本人以前写过的博客
一个入门级web.py应用如下:
import web #导入web.py模块 urls = ( '/', 'index' #这行表示我们要URL/(首页)被一个叫index的类处理。 ) class index: def GET(self): return "Hello, world!" #返回请求 if __name__ == "__main__": app = web.application(urls, globals()) #这会告诉web.py去创建一个基于我们刚提交的URL列表的application。这个application会在这个文件的全局命名空间中查找对应类。 app.run()
在命令行中运行以上程序:
[root@original weeb]# python weeb.py 1234
好的习惯就是:对于各个项目要有自己的目录,并且易于区分
打开浏览器http://192.168.1.112:1234/,你会看到Hello, world!
2、使用模板
给模板新建一个目录(命名为 templates,与应用处于同一目录中),在该目录下新建一个以 .html 结尾的文件,如halo.html模板文件的内容如下:
$def with (name) #表示模板将从这后面取值 $if name: I just wanted to say <em>hello</em> to $name. $else: <em>Hello</em>, world!
模板文件写好了,我们就要告诉应用去渲染了
在weeb.py的第一行之下添加:
render = web.template.render('templates/')
修改下 URL 配置:
'/(.*)', 'index'
然后修改下 index.GET:
def GET(self, name): return render.halo(name)
render.halo的halo是模板,也就是html文件的名称!!!
现在访问 /lockey 看看,显示如下:
如果不手动输入名字显示如下:
3、简单的数据库操作
安装postgresql数据库请参阅本人博客 PostgreSQL Getting Started安装数据库驱动模块:
pip install psycopg2
首先你需要创建一个数据库对象:
db = web.database(dbn='postgres', user='postgres', pw='redhat', db='test')
(根据自己的实际数据库状态,修改用户,密码,链接的数据库。 MySQL用户还需要把 dbn 定义改为 mysql。)
在你的库中创建一个简单的表:
CREATE TABLE todo ( id serial primary key, title text, created timestamp default now(), done boolean default 'f' );
向数据库中插入数据:
INSERT INTO todo (title) VALUES ('Learn web.py');
我们回来继续编辑weeb.py ,把 index.GET 改成下面的样子,替换整个函数:
def GET(self): todos = db.select('todo') return render.index(todos)
然后把URL列表改回来,只保留 /:
'/', 'index',
像这样编辑并替换 halo.html 的全部内容:
$def with (todos) <ul> $for todo in todos: <li id="t$todo.id">$todo.title</li> </ul>
到目前为止weeb.py文件的内容如下:
import web
render = web.template.render('templates/')db = web.database(dbn='postgres', user='postgres', pw='redhat', db='test')urls = (
'/', 'index'
)
app = web.application(urls, globals())
class index:
def GET(self):
todos = db.select('todo')
return render.halo(todos)
if __name__ == "__main__": app.run()
运行应用然后测试数据库链接以及数据获取:
如果在这一步出现问题:
Traceback (most recent call last): File "weeb.py", line 2, in <module> import psycopg2 File "/usr/lib64/python2.7/site-packages/psycopg2/__init__.py", line 50, in <module> from psycopg2._psycopg import ( # noqa ImportError: libpq.so.5: cannot open shared object file: No such file or directory
解决方法如下:
[root@original weeb]# find / -name libpq.so.5 /root/postgresql-9.6.4/src/interfaces/libpq/libpq.so.5 /usr/local/pgsql/lib/libpq.so.5 [root@original weeb]# ln -s /usr/local/pgsql/lib/libpq.so.5 /usr/lib lib/ lib64/ libexec/ [root@original weeb]# ln -s /usr/local/pgsql/lib/libpq.so.5 /usr/lib/libpq.so.5 [root@original weeb]# ln -s /usr/local/pgsql/lib/libpq.so.5 /usr/lib64/libpq.so.5
from stackoverflow:
1: Know the path of libpq.so.5 find / -name libpq.so.5 Output example: /usr/pgsql-9.4/lib/libpq.so.5 If found nothing, check if you have already installed the suitable postgresql-libs for your postgresql version and your OS platform 2: Symbolic link that library in a "well known" library path like /usr/lib: ln -s /usr/pgsql-9.4/lib/libpq.so.5 /usr/lib/libpq.so.5 Attention: If your platform is 64 bit, you MUST also symbolic link to 64 bit libraries path: ln -s /usr/pgsql-9.4/lib/libpq.so.5 /usr/lib64/libpq.so.5 3: Be happy !
通过表单向数据中插入数据:
在 index.html尾部添加:
<form method="post" action="add"> <p><input type="text" name="title" /> <input type="submit" value="Add" /></p> </form>
然后把你的URL列表改为:
'/', 'index','/add', 'add'
(你必须要非常小心那些逗号。如果你省略他们,Python会把所有字符串连接起来,变成 ‘/index/addadd’)
现在添加另一个类用来处理添加请求:
class add: def POST(self): i = web.input() n = db.insert('todo', title=i.title) raise web.seeother('/')
这个时候完整的weeb.py文件看起来是这样的:
import web
render = web.template.render('templates/')db = web.database(dbn='postgres', user='postgres', pw='redhat', db='test')urls = (
'/', 'index','/add', 'add'
)
app = web.application(urls, globals())
class index:
def GET(self):
todos = db.select('todo')
return render.halo(todos)
class add: def POST(self): i = web.input() n = db.insert('todo', title=i.title) raise web.seeother('/')
if __name__ == "__main__": app.run()
模板文件看起来是这样的:
$def with (todos) <ul> $for todo in todos: <li id="t$todo.id">$todo.title</li> </ul><form method="post" action="add"> <p><input type="text" name="title" /> <input type="submit" value="Add" /></p> </form>
运行然后在浏览器中测试:
数据库测试:
4、form模块
详细用法可以参考http://webpy.org/form以下为一个示例:
[root@original weeb]# cat weeb.py
import web
from web import form
render = web.template.render('templates/')
urls = ('/', 'index')
app = web.application(urls, globals())
myform = form.Form(
form.Textbox("name",
form.notnull,
form.regexp('[A-Za-z0-9\-]+', 'Must be alpha or digit!'),
form.Validator('Must be more than 5 characters!', lambda y:len(y)>5)),
form.Textbox("age",
form.notnull,
form.regexp('\d+', 'Must be a digit'),
form.Validator('Must be more than 5', lambda x:int(x)>5)),
form.Textarea('descriptions'),
form.Checkbox('hobby'),
form.Dropdown('french', ['mustard', 'fries', 'wine']))
class index:
def GET(self):
form = myform()
# make sure you create a copy of the form by calling it (line above)
# Otherwise changes will appear globally
return render.halo(form)
def POST(self):
form = myform()
if not form.validates():
return render.halo(form)
else:
# form.d.boe and form['boe'].value are equivalent ways of
# extracting the validated arguments from the form.
return "Grrreat success! name: %s, age: %s" % (form.d.name, form['age'].value)
if __name__=="__main__":
web.internalerror = web.debugerror
app.run()
[root@original weeb]# cat templates/halo.html
$def with (form) <form name="main" method="post"> $if not form.valid: <p class="error">Please correct the marked items and try again</p> $:form.render() <input type="submit" /> </form>
运行测试:
相关文章推荐
- Python Web框架----web.py 0.3 新手指南
- 400行python 教你写个高性能 http服务器+web框架,性能秒胜tornado django webpy uwsgi
- nginx连接uwsgi使用web.py框架构造pythonweb项目
- 简单而直接的Python web 框架:web.py
- 简单而直接的Python web 框架:web.py
- python开发框架(tornado, web.py)
- 安装Python的web.py框架并从hello world开始编程
- 【Python】windows下Eclipse中安装集成webpy框架
- Python - Web框架(web.py)
- python的web.py框架
- python网络编程 webpy框架
- Linux系统上Nginx+Python的web.py与Django框架环境
- python-django开发web框架的setting.py
- Linux系统上Nginx+Python的web.py与Django框架环境
- 简单而直接的Python web 框架:web.py
- 使用Python的web.py框架实现类似Django的ORM查询的教程
- 在python 2.7下面使用webpy框架
- 简单而直接的Python web 框架:web.py
- python web框架企业实战详解(第六期)\第四课时-webpy&django
- Python服务器框架学习——web.py(一)