Android 6.0指纹识别相关API
2017-11-22 15:59
393 查看
本文只对指纹识别相关的API做简单的讲解以及测试,官方文档的相应的API是23,也就是Android6.0才能使用的,但是有些厂商的6.0以下手机也适配了指纹功能,应该有它相应的兼容适配,这里就不做讲解,只讲讲基本的使用。我这里测试用的手机是一加5,Android7.1。
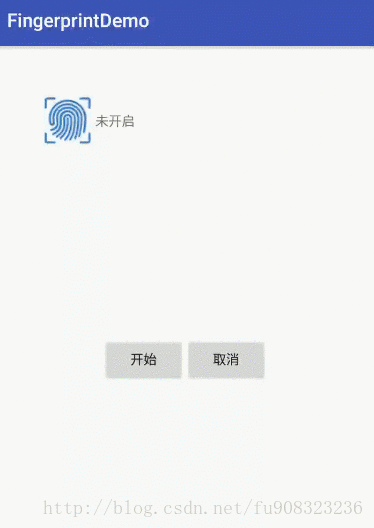
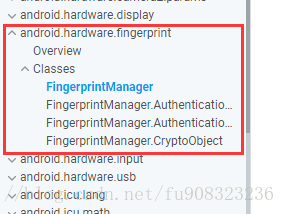
FingerprintManager类中主要对提供了3个方法,也是我们都需要用的,如图:
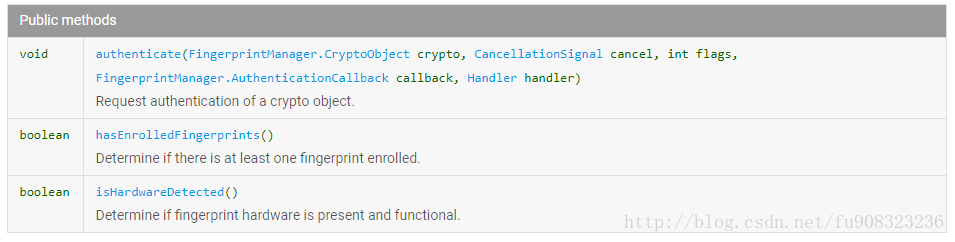
authenticate():开始指纹验证,也就是开启指纹传感器,需要
4000
传入一个回调,用来返回验证的结果。
hasEnrolledFingerprints():判断设备是否录入过指纹,录入过就返回true,反之false。
isHardwareDetected():判断设备是否支持指纹识别,支持为true,不支持为false。
再讲讲authenticate()方法中要传的参数:
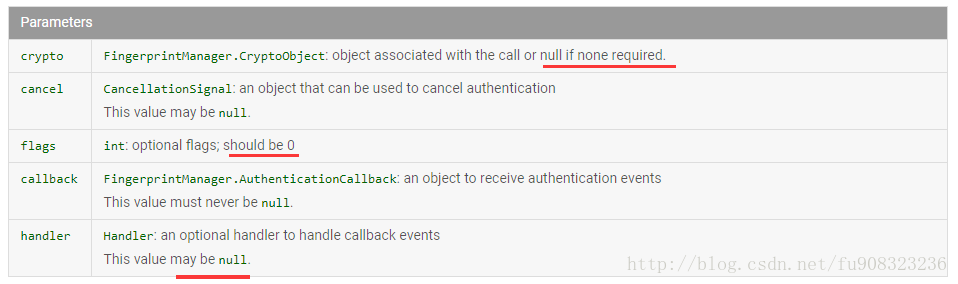
crypto:用来提高安全性的,里面有对指纹加密的操作。本文不讲这个,后面传入null就可以了。
cancel:用来取消指纹识别的,传入CancellationSignal对象。
flags:文档上说应该为0
callback:回调,指纹扫描后的结果都会进入到回调。
handler:传null即可。
下面就来实际演示一下。
后面的大致流程是
判断设备是否支持指纹。
判断设备是否处于安全保护中,比如需要设置解锁的密码锁,图案锁才能使用指纹。这个判断需要使用到KeyguardManager屏幕锁的服务。
判断设备是否录入过指纹,没录入过就当然也不能使用。
开始指纹验证。
首先需要拿到FingerprintManager,这里有两种方法,Google推荐第一种,我们也用第一种
代码
MainActivity.java
布局
activity_main.xml
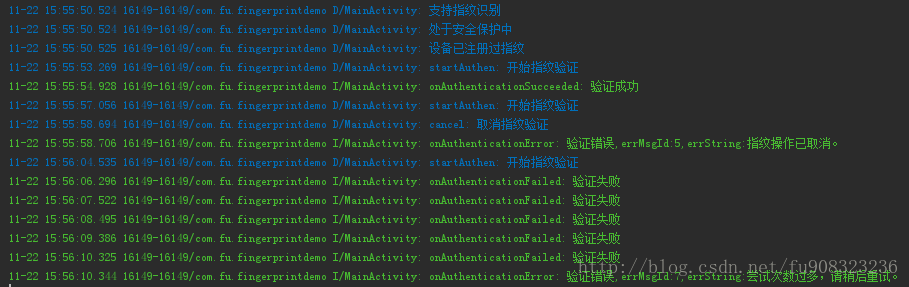
补充说明:
取消的回调会到Error中去。
验证失败指纹传感并没有关闭,可以最多连续验证5次,5次都失败了就会回调到验证错误中,并且会关闭指纹传感器,而且这个时候你把屏幕锁住用指纹也解不开,也会提示尝试次数过多。
效果图
官方文档介绍
与指纹识别相关的类不是很多,只有一个主类FingerprintManager,主类中有3个内部类,如图:FingerprintManager类中主要对提供了3个方法,也是我们都需要用的,如图:
authenticate():开始指纹验证,也就是开启指纹传感器,需要
4000
传入一个回调,用来返回验证的结果。
hasEnrolledFingerprints():判断设备是否录入过指纹,录入过就返回true,反之false。
isHardwareDetected():判断设备是否支持指纹识别,支持为true,不支持为false。
再讲讲authenticate()方法中要传的参数:
crypto:用来提高安全性的,里面有对指纹加密的操作。本文不讲这个,后面传入null就可以了。
cancel:用来取消指纹识别的,传入CancellationSignal对象。
flags:文档上说应该为0
callback:回调,指纹扫描后的结果都会进入到回调。
handler:传null即可。
下面就来实际演示一下。
演示
首先我们需要添加置为识别权限。在6.0以上这个权限是Normal Permissions的,所以不需要运行时申请权限。<uses-permission android:name="android.permission.USE_FINGERPRINT" />
后面的大致流程是
判断设备是否支持指纹。
判断设备是否处于安全保护中,比如需要设置解锁的密码锁,图案锁才能使用指纹。这个判断需要使用到KeyguardManager屏幕锁的服务。
判断设备是否录入过指纹,没录入过就当然也不能使用。
开始指纹验证。
首先需要拿到FingerprintManager,这里有两种方法,Google推荐第一种,我们也用第一种
//方式一:使用v4兼容包中的,Google推荐的 FingerprintManagerCompat fingerprintManagerCompat = FingerprintManagerCompat.from(this); //方式二:使用系统服务 FingerprintManager fingerprintManager = (FingerprintManager) getSystemService(Context.FINGERPRINT_SERVICE);
代码
MainActivity.java
public class MainActivity extends AppCompatActivity { private static final String TAG = "MainActivity"; private TextView tvinfo; private FingerprintManagerCompat fingerprintManagerCompat; private CancellationSignal cancellationSignal; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); this.tvinfo = (TextView) findViewById(R.id.tv_info); //使用v4兼容包中的,Google推荐的 fingerprintManagerCompat = FingerprintManagerCompat.from(this); if (fingerprintManagerCompat.isHardwareDetected()) { //判断设备是否支持指纹识别 Log.d(TAG, "支持指纹识别"); } else { Log.d(TAG, "不支持指纹识别"); } KeyguardManager keyguardManager = (KeyguardManager) getSystemService(Context.KEYGUARD_SERVICE); if (keyguardManager.isKeyguardSecure()) { //判断设备是否处于安全保护中 Log.d(TAG, "处于安全保护中"); } else { Log.d(TAG, "未处于安全保护中"); } if (fingerprintManagerCompat.hasEnrolledFingerprints()) { //判断设备是否已经录入过指纹 Log.d(TAG, "设备已录入指纹"); } else { Log.d(TAG, "设备未录入指纹"); } } /** * 开始指纹验证 * * @param view */ public void startAuthen(View view) { Log.d(TAG, "startAuthen: 开始指纹验证"); tvinfo.setText("开始指纹识别,请按下指纹"); cancellationSignal = new CancellationSignal(); fingerprintManagerCompat.authenticate(null, 0, cancellationSignal, new FingerprintManagerCompat.AuthenticationCallback() { //出现错误的时候会回调,比如:1.取消验证 2.验证了5次都是错误,就会提示验证次数过多 @Override public void onAuthenticationError(int errMsgId, CharSequence errString) { Log.i(TAG, "onAuthenticationError: 验证错误,errMsgId:" + errMsgId + ",errString:" + errString); tvinfo.setText("验证错误," + errString); } @Override public void onAuthenticationHelp(int helpMsgId, CharSequence helpString) { Log.i(TAG, "onAuthenticationHelp: "); } @Override public void onAuthenticationSucceeded(FingerprintManagerCompat.AuthenticationResult result) { Log.i(TAG, "onAuthenticationSucceeded: 验证成功"); tvinfo.setText("验证成功"); } @Override public void onAuthenticationFailed() { Log.i(TAG, "onAuthenticationFailed: 验证失败"); tvinfo.setText("验证失败"); } }, null); } /** * 取消指纹验证 * * @param view */ public void cancel(View view) { if (cancellationSignal != null) { Log.d(TAG, "cancel: 取消指纹验证"); cancellationSignal.cancel(); } } }
布局
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.fu.fingerprintdemo.MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="50dp" android:orientation="horizontal"> <ImageView android:layout_width="60dp" android:layout_height="60dp" android:layout_marginLeft="50dp" android:src="@mipmap/fingerprint" /> <TextView android:id="@+id/tv_info" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:text="未开启" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="200dp" android:gravity="center" android:orientation="horizontal"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="startAuthen" android:text="开始" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="cancel" android:text="取消" /> </LinearLayout> </LinearLayout>
打印的日志
补充说明:
取消的回调会到Error中去。
验证失败指纹传感并没有关闭,可以最多连续验证5次,5次都失败了就会回调到验证错误中,并且会关闭指纹传感器,而且这个时候你把屏幕锁住用指纹也解不开,也会提示尝试次数过多。
相关文章推荐
- Android指纹识别API讲解,一种更快更好的用户体验
- Android开发学习之路-指纹识别api
- Android 6.0指纹识别App开发demo
- Android 6.0使用指纹识别
- Android 6.0指纹识别App开发demo
- Android 6.0+指纹识别心得
- Android 6.0指纹识别App开发Demo
- Android 6.0 指纹识别功能详细分析(郭元歆)
- Android指纹识别功能深入浅出分析到实战(6.0以下系统解决方案)
- Android 6.0指纹识别App开发案例
- Android 6.0 指纹识别功能详细分析
- Android指纹识别深入浅出分析到实战(6.0以下系统适配方案)
- android 6.0 指纹识别调用 Demo
- Android指纹识别深入浅出分析到实战(6.0以下系统适配方案)
- android手机中指纹识别应用相关功能的讲解
- Android 6.0 指纹识别功能学习(一)----阿冬专栏!!!
- Android 6.0指纹识别App开发demo
- Android指纹识别API初试
- 关于android指纹识别兼容6.0以下版本
- Android 6.0指纹识别App开发demo