二级列表实现购物车的效果
2017-11-19 21:06
435 查看
转载的地址:http://blog.csdn.net/feifei20170707/article/details/78051516
效果如下
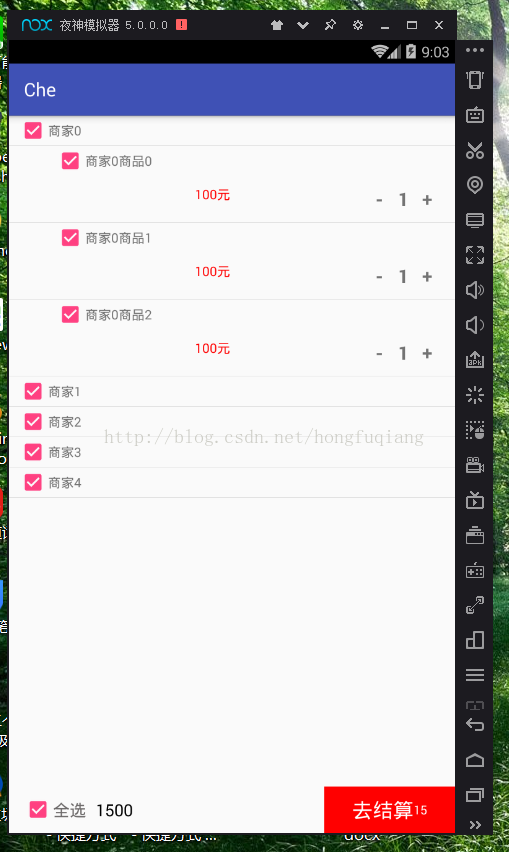
下面就是代码
Manactivity页面
Adapter适配器页面
保存数据和价格的JavaBean
MainActivity页面
Group_item页面
child_item页面
效果如下
下面就是代码
Manactivity页面
public class MainActivity extends AppCompatActivity { private ExpandableListView listview; private MyAdpater adpater; private TextView checked_shop; private TextView price; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); listview = (ExpandableListView) findViewById(R.id.listview); adpater = new MyAdpater(this); listview.setAdapter(adpater); final CheckBox checkAll = (CheckBox) findViewById(R.id.checkAll); price = (TextView) findViewById(R.id.price); checked_shop = (TextView) findViewById(R.id.checked_shop); checkAll.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { //设置商品全部选中 adpater.checkAllShop(checkAll.isChecked()); //计算选中的价格和数量 String shopPrice = adpater.getShopPrice(); //判断商品是否全部选中 boolean b = adpater.selectAll(); String[] split = shopPrice.split(","); price.setText(split[0]); checked_shop.setText(split[1]); checkAll.setChecked(b); } }); adpater.getAdapterData(new MyAdpater.AdapterData() { @Override public void Data(View v, String str, boolean b) { String[] split = str.split(","); price.setText(split[0]); checked_shop.setText(split[1]); checkAll.setChecked(b); } }); checkAll.setChecked(adpater.selectAll()); adpater.notifyDataSetChanged(); } }
Adapter适配器页面
public class MyAdpater extends BaseExpandableListAdapter { private Context context; private String[] group; private String[][] child; private HashMap<Integer, Boolean> groupHashMap; private List<HashMap<Integer, Boolean>> childList; private List<List<Bean>> dataList; public MyAdpater(Context context) { this.context = context; initData(); } private void initData() { group = new String[5]; child = new String[5][]; groupHashMap = new HashMap<>(); childList = new ArrayList<>(); dataList = new ArrayList<>(); for (int i = 0; i < 5; i++) { group[i] = "商家" + i; groupHashMap.put(i, false); String[] strings = new String[3]; HashMap<Integer, Boolean> map = new HashMap<>(); ArrayList<Bean> been = new ArrayList<>(); for (int y = 0; y < 3; y++) { strings[y] = "商家" + i + "商品" + y; map.put(y, false); Bean bean = new Bean("100", "1"); been.add(bean); } child[i] = strings; childList.add(map); dataList.add(been); } } @Override public int getGroupCount() { return group.length; } @Override public int getChildrenCount(int groupPosition) { return child[groupPosition].length; } @Override public Object getGroup(int groupPosition) { return group[groupPosition]; } @Override public Object getChild(int groupPosition, int childPosition) { return child[childPosition]; } @Override public long getGroupId(int groupPosition) { return groupPosition; } @Override public long getChildId(int groupPosition, int childPosition) { return childPosition; } @Override public boolean hasStableIds() { return false; } @Override public View getGroupView(final int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) { GroupViewHolder holder = null; if (convertView == null) { convertView = View.inflate(context, R.layout.group_item, null); holder = new GroupViewHolder(); holder.tv = (TextView) convertView.findViewById(R.id.group_tv); holder.ck = (CheckBox) convertView.findViewById(R.id.group_ck); convertView.setTag(holder); } else { holder = (GroupViewHolder) convertView.getTag(); } holder.tv.setText(group[groupPosition]); holder.ck.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { groupHashMap.put(groupPosition, !groupHashMap.get(groupPosition)); //设置二级列表的选中状态,根据一级列表的状态来改变 setChildCheckAll(); //计算选中的价格和数量 String shopPrice = getShopPrice(); //判断商品是否全部选中 boolean b = selectAll(); adapterData.Data(v, shopPrice, b); } }); holder.ck.setChecked(groupHashMap.get(groupPosition)); return convertView; } @Override public View getChildView(final int groupPosition, final int childPosition, boolean isLastChild, View convertView, ViewGroup parent) { ChildViewHolder holder = null; if (convertView == null) { convertView = View.inflate(context, R.layout.child_item, null); holder = new ChildViewHolder(); holder.tv = (TextView) convertView.findViewById(R.id.child_tv); holder.ck = (CheckBox) convertView.findViewById(R.id.child_ck); holder.jianshao = (TextView) convertView.findViewById(R.id.jianshao); holder.zengjia = (TextView) convertView.findViewById(R.id.zengjia); holder.number = (TextView) convertView.findViewById(R.id.number); convertView.setTag(holder); } else { holder = (ChildViewHolder) convertView.getTag(); } holder.tv.setText(child[groupPosition][childPosition]); holder.ck.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { HashMap<Integer, Boolean> hashMap = childList.get(groupPosition); hashMap.put(childPosition, !hashMap.get(childPosition)); //判断二级列表是否全部选中 ChildisChecked(groupPosition); //计算选中的价格和数量 String shopPrice = getShopPrice(); //判断商品是否全部选中 boolean b = selectAll(); adapterData.Data(v, shopPrice, b); } }); final ChildViewHolder finalHolder = holder; holder.zengjia.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { List<Bean> been = dataList.get(groupPosition); String num = finalHolder.number.getText().toString(); int i = Integer.parseInt(num); ++i; been.get(childPosition).setNumber(i + ""); //计算选中的价格和数量 String shopPrice = getShopPrice(); //判断商品是否全部选中 boolean b = selectAll(); adapterData.Data(v, shopPrice, b); notifyDataSetChanged(); } }); holder.jianshao.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { List<Bean> been = dataList.get(groupPosition); String num = finalHolder.number.getText().toString(); int i = Integer.parseInt(num); if (i > 1) { --i; } been.get(childPosition).setNumber(i + ""); //计算选中的价格和数量 String shopPrice = getShopPrice(); //判断商品是否全部选中 boolean b = selectAll(); adapterData.Data(v, shopPrice, b); notifyDataSetChanged(); } }); holder.number.setText(dataList.get(groupPosition).get(childPosition).getNumber().toString()); holder.ck.setChecked(childList.get(groupPosition).get(childPosition)); return convertView; } @Override public boolean isChildSelectable(int groupPosition, int childPosition) { return false; } class GroupViewHolder { TextView tv; CheckBox ck; } class ChildViewHolder { TextView tv; CheckBox ck; TextView jianshao; TextView zengjia; TextView number; } //设置二级列表的选中状态,根据一级列表的状态来改变 private void setChildCheckAll() { for (int i = 0; i < childList.size(); i++) { HashMap<Integer, Boolean> integerBooleanHashMap1 = childList.get(i); Set<Map.Entry<Integer, Boolean>> entries = integerBooleanHashMap1.entrySet(); for (Map.Entry<Integer, Boolean> entry : entries) { entry.setValue(groupHashMap.get(i)); } } notifyDataSetChanged(); } //判断二级列表是否全部选中 private void ChildisChecked(int groupPosition) { boolean ischecked = true; HashMap<Integer, Boolean> hashMap = childList.get(groupPosition); Set<Map.Entry<Integer, Boolean>> entries = hashMap.entrySet(); for (Map.Entry<Integer, Boolean> entry : entries) { if (!entry.getValue()) { ischecked = false; break; } } groupHashMap.put(groupPosition, ischecked); notifyDataSetChanged(); } //全选 public void checkAllShop(boolean checked) { Set<Map.Entry<Integer, Boolean>> entries = groupHashMap.entrySet(); for (Map.Entry<Integer, Boolean> entry : entries) { entry.setValue(checked); } //调用让二级列表全选的方法 setChildCheckAll(); notifyDataSetChanged(); } //计算价格 public String getShopPrice() { int price = 0; int number = 0; for (int y = 0; y < childList.size(); y++) { HashMap<Integer, Boolean> integerBooleanHashMap1 = childList.get(y); Set<Map.Entry<Integer, Boolean>> entries = integerBooleanHashMap1.entrySet(); for (Map.Entry<Integer, Boolean> entry : entries) { if (entry.getValue()) { Bean bean = dataList.get(y).get(entry.getKey()); price += Integer.parseInt(bean.getPrice()) * Integer.parseInt(bean.getNumber()); number += Integer.parseInt(bean.getNumber()); } } } return price + "," + number; } //编辑一级和二级列表,如果全部选中,全选按钮也选中 public boolean selectAll() { boolean isChecked = true; for (int y = 0; y < childList.size(); y++) { HashMap<Integer, Boolean> hashMap = childList.get(y); Set<Map.Entry<Integer, Boolean>> entries = hashMap.entrySet(); for (Map.Entry<Integer, Boolean> entry : entries) { if (!entry.getValue()) { isChecked = false; break; } } } return isChecked; } private AdapterData adapterData; public interface AdapterData { void Data(View v, String str, boolean b); } public void getAdapterData(AdapterData adapterData) { this.adapterData = adapterData; } }
保存数据和价格的JavaBean
public class Bean { private String price; private String number; public Bean(String price, String number) { this.price = price; this.number = number; } public String getPrice() { return price; } public void setPrice(String price) { this.price = price; } public String getNumber() { return number; } public void setNumber(String number) { this.number = number; } }
MainActivity页面
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <ExpandableListView android:id="@+id/listview" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_weight="1" android:groupIndicator="@null"></ExpandableListView> <RelativeLayout android:layout_width="match_parent" android:layout_height="50dp"> <CheckBox android:id="@+id/checkAll" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_gravity="center_vertical" android:layout_marginLeft="15dp" android:drawablePadding="5dp" android:text="全选" android:textColor="#6b6868" android:textSize="18sp" /> <TextView android:id="@+id/price" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_marginLeft="10dp" android:layout_toRightOf="@id/checkAll" android:gravity="center" android:text="合计:¥0.00" android:textColor="#000000" android:textSize="18sp" /> <LinearLayout android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_alignParentRight="true" android:background="#ff0000"> <TextView android:layout_width="wrap_content" android:layout_height="match_parent" android:background="#ff0000" android:gravity="center" android:paddingLeft="30dp" android:text="去结算" android:textColor="#ffffff" android:textSize="22sp" /> <TextView android:id="@+id/checked_shop" android:layout_width="wrap_content" android:layout_height="match_parent" android:background="#ff0000" android:gravity="center" android:paddingRight="30dp" android:text="(0)" android:textColor="#ffffff" android:textSize="13sp" /> </LinearLayout> </RelativeLayout> </LinearLayout>
Group_item页面
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content"> <CheckBox android:id="@+id/child_ck" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="50dp" /> <TextView android:id="@+id/child_tv" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout> <RelativeLayout android:layout_width="match_parent" android:layout_height="40dp" android:layout_marginTop="10dp"> <TextView android:id="@+id/danjia" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="200dp" android:text="100元" android:textColor="#ff0000" /> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true"> <TextView android:id="@+id/jianshao" android:layout_width="20dp" android:layout_height="30dp" android:gravity="center" android:text="-" android:textSize="20sp" android:textStyle="bold" /> <TextView android:id="@+id/number" android:layout_width="wrap_content" android:layout_height="30dp" android:layout_marginLeft="10dp" android:layout_marginRight="10dp" android:gravity="center" android:text="1" android:textSize="20sp" android:textStyle="bold" /> <TextView android:id="@+id/zengjia" android:layout_width="20dp" android:layout_height="30dp" android:layout_marginRight="20dp" android:gravity="center" android:text="+" android:textSize="20sp" android:textStyle="bold" /> </LinearLayout> </RelativeLayout> </LinearLayout>
child_item页面
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <CheckBox android:id="@+id/group_ck" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="10dp" android:focusable="false" /> <TextView android:id="@+id/group_tv" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
相关文章推荐
- 自定义Adapter实现RecyclerView的可展开二级列表expand效果
- 购物车二级列表的实现,在一级列表基础上,bean类里再添加两个字段(请求本地json数据)
- 二级列表购物车的实现逻辑——wyh
- Andriodjie——二级列表实现购物车
- Andriodjie——二级列表实现购物车
- 使用ExpandListView二级列表实现购物车
- RecyclerView条目跳转+SpringView数据刷新加载+MVP+OKhttp+拦截器+自定义view 实现请求网络数据的二级列表购物车
- android购物车二级列表实现+MVP+Okhttp
- 【深入篇】自定义ExpandableListView,实现二级列表效果
- MVP+OKhttp+拦截器+RecyclerView+自定义view 实现请求网络数据的二级列表购物车
- Andriod购物车实现二级列表(MVP)
- 二级列表实现购物车功能
- 微信小程序 数据 二级,多级列表展示效果实现
- 二级列表实现购物车添加购物车
- Android实现二级列表购物车功能
- jQuery实现带延迟的二级tab切换下拉列表效果
- android 购物车二级列表实现
- Android——二级列表实现购物车
- 查询购物车---二级列表实现
- Android用MVP实现的二级列表购物车