MySQL初步学习4:处理大数据对象
2017-11-01 19:06
253 查看
大数据对象这里主要指CLOB和BLOB两种类型的字段。
CLOB可以存储文本数据,而BLOB中可以存储二进制数据,如图片,电影等。而程序中处理这些大对象数据,必须调用PreparedStatement完成,所有的内容以IO流的方式从大文本字段中保存和读取。
PreparedStatement类中提供了两种方法写入数据
setAsciiStream():文本输入
setBinaryStream():二进制输入
另外,有几种读取数据方式:
getAsciiStream() 获取文本输出流
getBinaryStream() 获取二进制输出流
getClob() 获取CLOB数据
getBlob() 获取BLOB数据
首先在数据库bb中建表如下:
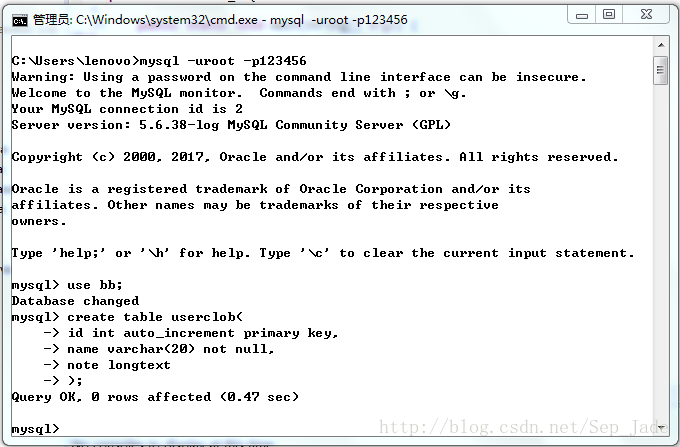
文本文档aa存放路径在”d:\aa.txt”,文本内容:
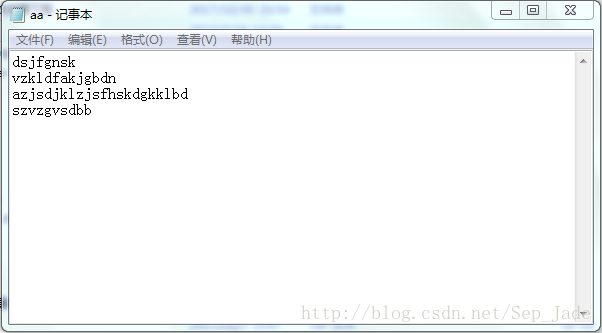
在eclipse中写入大文本数据
读取大文本数据
结果如下:
可以看到,用CLOB读取数据更加简洁。
在数据库建表userblob,方法和上面一样。
将图片写入到数据表中
用到的图片存放到d盘:

在eclipse中编程:
新建一个图片,内容为空,保存在d盘,用于接收数据(也可以程序创建,如下一个例子)。读取内容,将图片信息保存。
使用BLOB读取内容,更加简单:
CLOB可以存储文本数据,而BLOB中可以存储二进制数据,如图片,电影等。而程序中处理这些大对象数据,必须调用PreparedStatement完成,所有的内容以IO流的方式从大文本字段中保存和读取。
PreparedStatement类中提供了两种方法写入数据
setAsciiStream():文本输入
setBinaryStream():二进制输入
另外,有几种读取数据方式:
getAsciiStream() 获取文本输出流
getBinaryStream() 获取二进制输出流
getClob() 获取CLOB数据
getBlob() 获取BLOB数据
1、处理CLOB数据
Clob表示大文本数据,在MySQL中有longtext表示大文本类型,最大保存4G数据。首先在数据库bb中建表如下:
文本文档aa存放路径在”d:\aa.txt”,文本内容:
在eclipse中写入大文本数据
package fileOperation; import java.io.File; import java.io.FileInputStream; import java.io.InputStream; import java.sql.*; public class CLOB_1 { //将文本文件中的内容写入到数据库中 public static void main(String[] args) throws Exception{ Connection con=null; PreparedStatement ps=null; //必须用预处理语句读取数据 Class.forName("com.mysql.jdbc.Driver"); con=DriverManager.getConnection("jdbc:mysql://localhost:3306/bb","root","123456"); ps=con.prepareStatement(" insert into userclob (name,note) values (?,?) "); File f=new File("d:"+File.separator+"aa.txt"); //File.separator表示分隔符,更加通用 InputStream input=new FileInputStream(f); ps.setString(1, "aa"); ps.setAsciiStream(2, input,(int) f.length()); //设置输入流 ps.executeUpdate(); ps.close(); con.close(); } }
读取大文本数据
package fileOperation; import java.io.InputStream; import java.sql.*; import java.util.Scanner; public class CLOB_2 { public static void main(String[] args) throws Exception{ Connection con=null; PreparedStatement ps=null; ResultSet rs=null; Class.forName("com.mysql.jdbc.Driver"); con=DriverManager.getConnection("jdbc:mysql://localhost:3306/bb","root","123456"); ps=con.prepareStatement(" select name,note from userclob where id=? "); ps.setInt(1,1); rs=ps.executeQuery(); while (rs.next()) { String name=rs.getString(1); StringBuffer note=new StringBuffer(); InputStream input = rs.getAsciiStream(2);//接收全部大文本数据 Scanner scan=new Scanner(input); scan.useDelimiter("\r\n"); //将文件换行作为分隔符 while(scan.hasNext()) { note.append(scan.next()).append("\n"); //不断读取数据 } System.out.println("内容为"+note); System.out.println("*****************************************************************"); Clob c=rs.getClob(2); String note1=c.getSubString(1, (int) c.length()); System.out.println("内容为"+note); c.truncate(10); //读取10个长度的内容 System.out.println("读取部分内容: "+c.getSubString(1, (int)c.length()) ); //可以看到,两种方法均可以读取文件中的内容,而下面的这种方法更为简单 } rs.close(); ps.close(); con.close(); } }
结果如下:
内容为dsjfgnsk vzkldfakjgbdn azjsdjklzjsfhskdgkklbd szvzgvsdbb ***************************************************************** 内容为dsjfgnsk vzkldfakjgbdn azjsdjklzjsfhskdgkklbd szvzgvsdbb 读取部分内容: dsjfgnsk
可以看到,用CLOB读取数据更加简洁。
2、处理BLOB对象
BLOB操作与CLOB相似,专门用于存放二进制数据,如电影、图片等。在MySQL中定义了longblob类型变量,最高可以存放4G数据。在数据库建表userblob,方法和上面一样。
将图片写入到数据表中
用到的图片存放到d盘:
在eclipse中编程:
package fileOperation; import java.io.File; import java.io.FileInputStream; import java.io.InputStream; import java.sql.Clob; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.Scanner; public class BLOB_1 { public static void main(String[] args) throws Exception{ Connection con=null; PreparedStatement ps=null; //必须用预处理语句读取数据 Class.forName("com.mysql.jdbc.Driver"); con=DriverManager.getConnection("jdbc:mysql://localhost:3306/bb","root","123456"); ps=con.prepareStatement(" insert into userblob (name,photo) values (?,?) "); File f=new File("d:"+File.separator+"11.gif"); //File.separator表示分隔符 InputStream input=new FileInputStream(f); ps.setString(1, "bb"); ps.setBinaryStream(2,input,(int)f.length()); ps.executeUpdate(); ps.close(); con.close(); } }
新建一个图片,内容为空,保存在d盘,用于接收数据(也可以程序创建,如下一个例子)。读取内容,将图片信息保存。
package fileOperation; import java.io.File; import java.io.FileOutputStream; import java.io.InputStream; import java.sql.*; public class BLOB_2 { public static void main(String[] args) throws Exception{ Connection con=null; PreparedStatement ps=null; ResultSet rs=null; Class.forName("com.mysql.jdbc.Driver"); con=DriverManager.getConnection("jdbc:mysql://localhost:3306/bb","root","123456"); ps=con.prepareStatement(" select name,photo from userblob where id = ? "); ps.setInt(1, 1); rs=ps.executeQuery(); while(rs.next()) { String name = rs.getString(1); InputStream input =rs.getBinaryStream(2); FileOutputStream output = new FileOutputStream(new File("d:"+File.separator+"12.gif")); int temp=0; while((temp=input.read())!=-1) { output.write(temp); } input.close(); output.close(); } rs.close(); ps.close(); con.close(); } }
使用BLOB读取内容,更加简单:
package fileOperation; import java.io.File; import java.io.FileOutputStream; import java.io.InputStream; import java.sql.*; public class BLOB_3 { public static void main(String[] args) throws Exception{ Connection con=null; PreparedStatement ps=null; ResultSet rs=null; Class.forName("com.mysql.jdbc.Driver"); con=DriverManager.getConnection("jdbc:mysql://localhost:3306/bb","root","123456"); ps=con.prepareStatement(" select name,photo from userblob where id = ? "); ps.setInt(1, 1); rs=ps.executeQuery(); while(rs.next()) { String name = rs.getString(1); Blob b=rs.getBlob(2); File f=new File("d:"+File.separator+"13.gif"); if(f.exists()) { f.delete(); } else { f.createNewFile(); } FileOutputStream output = new FileOutputStream(new File("d:"+File.separator+"13.gif")); output.write(b.getBytes(1, (int) b.length())); output.close(); } rs.close(); ps.close(); con.close(); } }
相关文章推荐
- 【JavaEE学习笔记】Hibernate_05_数据类型转换和大对象处理,QBC(junit),DAO接口
- mysql 学习小札(3)-- 数据的处理
- MySQL学习---->第二练:语句初步(SQL概述、数据定义、查询)
- Mysql学习总结(13)——使用JDBC处理MySQL大数据
- Mysql学习总结(13)——使用JDBC处理MySQL大数据
- MySQL学习笔记_13_Linux下C++/C连接MySQL数据库(三) --处理返回数据
- Mysql学习总结(13)——使用JDBC处理MySQL大数据
- JavaWeb学习心得之JDBC处理MySQL大数据
- MySQL学习---->第三练:语句初步(数据更新、视图、数据控制)
- 处理Clob数据(转)关于oracle中大对象处理的一些方法和实例
- BT源代码学习心得(七):跟踪服务器(Tracker)的代码分析(HTTP协议处理对象)
- BT源代码学习心得(七):跟踪服务器(Tracker)的代码分析(HTTP协议处理对象)
- BT源代码学习心得(七):跟踪服务器(Tracker)的代码分析(HTTP协议处理对象)
- c#通过Graphics处理图片以及画图(初步学习1)
- Php+mysql处理大容量数据存储
- 设置 MySql 数据同步及故障处理
- 09月11日学习杂记(MYSQL初步)
- MySQL数据入库时特殊字符处理
- MyGeneration学习笔记(8) :dOOdad提供的数据绑定、特殊函数和事务处理
- ASP中非数据库实现数据对象的定义及处理