SpringCloud使用Feign进行服务调用
2017-10-16 13:28
1001 查看
- Spring Cloud Feign简介
Spring Cloud Feign也是一个基础工具类,它整合了Spring Cloud Ribbon和Spring Cloud Hystrix,除了提供这两者的强大功能以外,它还提供了一种声明式的Web服务客户端定义方式。使用它可以进行服务的消费,但是它的客户端负载平衡仍是通过Ribbon实现的
*- 使用Spring Cloud Feign
创建一个SpringBoot工程,作为服务调用方
1.pom.xml
spring-cloud-starter-feign加入了feign的依赖
2.启动类
@EnableFeignClients:开启Spring Cloud Feign的支持功能
3.服务层
@FeignClient(“hello-service”):制定服务名来绑定服务、
注:服务名不区分大小写
@RequestMapping:指定调用服务中的具体方法
4.Controller层
在方法中调用这个绑定了hello-service服务接口的客户端向该服务发起/hello接口的调用
5.配置类
启动程序以后,在浏览器中输入http://localhost:9001/feign-consumer出现下图:
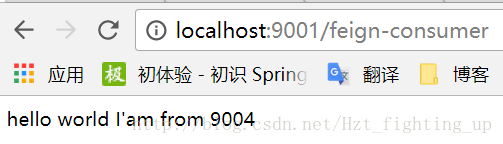
- 提升使用,带参数的请求
在具体业务中的接口会比之前程序的复杂得多,这里介绍一下Feign对集中不同形式参数的绑定方法。有@RequestParam、@RequestHeader、@RequestBody
1.改造服务提供类的Service层,添加几个方法
2.添加一个javabean
3.修改服务调用类的接口
这里在定义各参数绑定时@RequestParam、@RequestHeader等可以指定参数名称的注解,但它们的value不能少,否则会报错,这和SpringMVC中有一点不同
4.在服务调用类Controller层添加一个测试的接口
启动以后访问浏览器:
Spring Cloud Feign也是一个基础工具类,它整合了Spring Cloud Ribbon和Spring Cloud Hystrix,除了提供这两者的强大功能以外,它还提供了一种声明式的Web服务客户端定义方式。使用它可以进行服务的消费,但是它的客户端负载平衡仍是通过Ribbon实现的
*- 使用Spring Cloud Feign
创建一个SpringBoot工程,作为服务调用方
1.pom.xml
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-eureka</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-feign</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency>
spring-cloud-starter-feign加入了feign的依赖
2.启动类
@EnableFeignClients @EnableDiscoveryClient @SpringBootApplication public class FeignConsumerApplication { public static void main(String[] args) { SpringApplication.run(FeignConsumerApplication.class, args); } }
@EnableFeignClients:开启Spring Cloud Feign的支持功能
3.服务层
@FeignClient("hello-service") public interface HelloService { @RequestMapping(value = "/hello", method = RequestMethod.GET) String hello(); }
@FeignClient(“hello-service”):制定服务名来绑定服务、
注:服务名不区分大小写
@RequestMapping:指定调用服务中的具体方法
4.Controller层
@Controller public class ConsumerController { @Autowired private HelloService helloService; @RequestMapping(value = "/feign-consumer", method = RequestMethod.GET) @ResponseBody public String helloConsumer() { return helloService.hello(); } }
在方法中调用这个绑定了hello-service服务接口的客户端向该服务发起/hello接口的调用
5.配置类
server.port=9001 spring.application.name=feign-consumer eureka.client.serviceUrl.defaultZone=http://localhost:1111/eureka/
启动程序以后,在浏览器中输入http://localhost:9001/feign-consumer出现下图:
- 提升使用,带参数的请求
在具体业务中的接口会比之前程序的复杂得多,这里介绍一下Feign对集中不同形式参数的绑定方法。有@RequestParam、@RequestHeader、@RequestBody
1.改造服务提供类的Service层,添加几个方法
@RequestMapping(value = "/hello1", method = RequestMethod.GET) @ResponseBody public String hello(@RequestParam String name) { return "hello " + name; } @RequestMapping(value = "/hello2", method = RequestMethod.GET) @ResponseBody public User hello(@RequestHeader String name, @RequestHeader Integer age) { return new User(name, age); } @RequestMapping(value = "/hello3", method = RequestMethod.POST) @ResponseBody public String hello(@RequestBody User user) { return "Hello " + user.getName() + ", " + user.getAge(); }
2.添加一个javabean
public class User { private String name; private Integer age; public User() { } public User(String name, Integer age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } @Override public String toString() { return "User{" + "name='" + name + '\'' + ", age=" + age + '}'; } }
3.修改服务调用类的接口
@RequestMapping(value = "/hello1", method = RequestMethod.GET) String hello(@RequestParam("name") String name); @RequestMapping(value = "/hello2", method = RequestMethod.GET) User hello(@RequestHeader("name") String name, @RequestHeader("age") Integer age); @RequestMapping(value = "/hello3", method = RequestMethod.POST) String hello(@RequestBody User user);
这里在定义各参数绑定时@RequestParam、@RequestHeader等可以指定参数名称的注解,但它们的value不能少,否则会报错,这和SpringMVC中有一点不同
4.在服务调用类Controller层添加一个测试的接口
@RequestMapping(value = "/feign-consumer2", method = RequestMethod.GET) @ResponseBody public String helloConsumer2() { String s2 = helloService.hello("dayday"); return s2; }
启动以后访问浏览器:
相关文章推荐
- 使用Spring cloud Feign在后台服务之间调用传递Multipart无法传递的问题
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- Spring Cloud(十一)声名式服务调用:Feign的使用 (上)
- SpringCloud 查找调用REST服务使用RestTemplate(ribbon负载)或feign模式 教程源码 火推
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- springcloud使用feign实现服务间条用,参数数据太大,无法调用成功解决方法
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- SpringBoot使用FeignClient进行服务间的调用,传递headers信息
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务的方法(推荐)
- 使用Spring Cloud Feign作为HTTP客户端调用远程HTTP服务
- 3.springcloud中使用Ribbon和Feign调用服务以及服务的高可用
- spring cloud Feign(声明式服务调用)
- Spring Cloud中如何优雅的使用Feign调用接口
- Spring Cloud版——电影售票系统<三>使用Feign实现声明式REST调用
- SpringCloud零基础上手(四)——服务发现以及Feign(声明式RESTful服务调用)