简单的使用POI导出excel
2017-10-15 09:27
447 查看
package org.test; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.util.ArrayList; import java.util.List; import org.apache.poi.hssf.usermodel.HSSFCell; import org.apache.poi.hssf.usermodel.HSSFCellStyle; import org.apache.poi.hssf.usermodel.HSSFFont; import org.apache.poi.hssf.usermodel.HSSFRow; import org.apache.poi.hssf.usermodel.HSSFSheet; import org.apache.poi.hssf.usermodel.HSSFWorkbook; import org.apache.poi.ss.usermodel.CellStyle; import org.apache.poi.ss.usermodel.IndexedColors; import org.apache.poi.xssf.usermodel.XSSFCell; import org.apache.poi.xssf.usermodel.XSSFCellStyle; import org.apache.poi.xssf.usermodel.XSSFFont; import org.apache.poi.xssf.usermodel.XSSFRow; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; /** * Excel导入导出通用工具类 * @author sunzhenyang on 2017-10-10 * */ public class ExcelUtils { private final static String path="F://"; public static void main(String[] args) throws IOException { List<String>[] list = new ArrayList[2]; List<String> lists = new ArrayList<String>(); lists.add("aaaa"); lists.add("bbbb"); lists.add("cccc"); lists.add("dddd"); List<String> lists1 = new ArrayList<String>(); lists1.add("11111"); lists1.add("22222"); lists1.add("33333"); lists1.add("44444"); list[0]=lists; list[1]=lists1; uploadExcel(list); } /** * 导出 * @param list * @throws IOException */ private static void uploadExcel(List<String>[] list) throws IOException { //1、创建workbook工作本,对应一个excel //HSSFWorkbook wb = new HSSFWorkbook(); // xls 格式 == 2003 XSSFWorkbook wb = new XSSFWorkbook(); // xlsx 格式 == 2007 //2、生成一个sheet,对应excel的sheet,参数为excel中sheet显示的名字 XSSFSheet sheet = wb.createSheet("sheet1"); XSSFRow row = sheet.createRow(0); row.setHeight((short) 800);// 设定行的高度 //5、创建row中的单元格,从0开始 XSSFCell cell = row.createCell(0);//我们第一列设置宽度为0,不会显示,因此第0个单元格不需要设置样式 cell.setCellValue("优惠协议表");//设置单元格中内容 第一行第一列 createSheet(wb,sheet,cell,row); //6、输入数据 for (int i = 0; i < list.length; i++) { row = sheet.createRow(i+1); //设置行 for (int j = 0; j < list[i].size(); j++) { //同第五步 cell = row.createCell(j+1);//创建列 cell.setCellValue(list[i].get(j)); //向列中赋值 } } //8、输入excel FileOutputStream os = new FileOutputStream(path+"123abcdefg.xlsx"); wb.write(os); os.close(); System.out.println("导出成功!"); } /** * 创建第一行的标题样式 和 名称 * @param wb * @return */ private static void createSheet(XSSFWorkbook wb,XSSFSheet sheet,XSSFCell cell,XSSFRow row ) { XSSFCellStyle style1 = setStyle(wb); //3、设置sheet中每列的宽度,第一个参数为第几列,0为第一列;第二个参数为列的宽度,可以设置为0。 sheet.setColumnWidth(0, 20*256);//4、生成sheet中一行,从0开始 sheet.setColumnWidth(1, 20*256); sheet.setColumnWidth(2, 20*256); sheet.setColumnWidth(3, 20*256); sheet.setColumnWidth(4, 20*256); cell = row.createCell(1);//从第1个单元格开始,设置每个单元格样式 cell.setCellValue("第一列");//设置单元格中内容 cell.setCellStyle(style1);//设置单元格样式 cell = row.createCell(2);//第二个单元格 cell.setCellValue("第二列"); cell.setCellStyle(style1); cell = row.createCell(3);//第三个单元格 cell.setCellValue("第三列"); cell.setCellStyle(style1); cell = row.createCell(4);//第三个单元格 cell.setCellValue("第四列"); cell.setCellStyle(style1); } /** * 设置样式 * @param wb * @return */ private static XSSFCellStyle setStyle(XSSFWorkbook wb) { //1.5、生成excel中可能用到的单元格样式 //首先创建字体样式 XSSFFont font = wb.createFont();//创建字体样式 font.setFontName("宋体");//使用宋体 font.setFontHeightInPoints((short) 10);//字体大小 font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);// 加粗 //然后创建单元格样式style XSSFCellStyle style1 = wb.createCellStyle(); style1.setFont(font);//将字体注入 style1.setWrapText(true);// 自动换行 style1.setAlignment(HSSFCellStyle.ALIGN_CENTER);// 左右居中 style1.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER);// 上下居中 style1.setFillForegroundColor(IndexedColors.LIGHT_YELLOW.getIndex());// 设置单元格的背景颜色 style1.setFillPattern(CellStyle.SOLID_FOREGROUND); style1.setBorderTop((short) 1);// 边框的大小 style1.setBorderBottom((short) 1); style1.setBorderLeft((short) 1); style1.setBorderRight((short) 1); return style1; } }
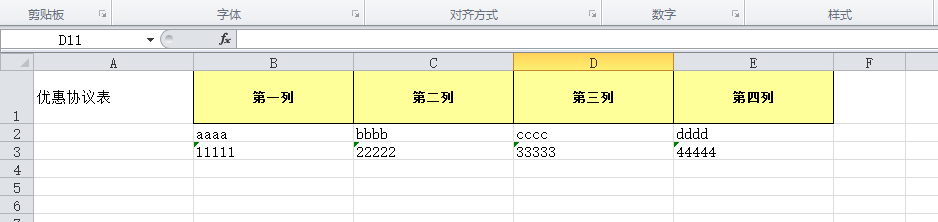
相关文章推荐
- Java-Maven-POI 简单导入导出Excel通用工具,默认使用基于poi实现
- 【JavaWeb开发】使用java实现简单的Excel文件的导入与导出(POI)
- SpringMVC 使用poi导出excel简单小例子
- POI导出Excel简单实现
- 使用poi做excel导出时解决以文本格式存储的数字问题
- 使用poi导出excel
- 使用poi低版本(poi-3.0.1)导出Excel整理
- 使用JDBC+POI把Excel中的数据导出到MySQL
- POI导出到Excel的简单例子
- Java Web利用POI导出Excel简单例子
- 使用poi导出excel
- 使用servlet和poi从服务器导出excel
- 使用POI导出Excel示例
- 使用POI导出excel,完美兼容2003及2007以上版本,购物车原理
- 使用JDBC+POI把Excel中的数据导出到MySQL
- POI导出到Excel的简单例子
- 使用POI导入,导出Excel
- Java使用POI导出到Excel。
- web中使用POI导入导出EXCEL文件的例子