Python学习笔记: Python匿名函数lambda的使用
2017-10-13 16:57
661 查看
定义格式:
lambda
argument1, argument2,...,argumentN :expression using arguments
可见,匿名函数的变量可以有多个,但表达式通常只有一条,因此匿名函数只能使用在比较简单的情况下,后面的表达式会返回结果
(可以无参,调用时不可以在参数表中赋值;后面表达式不能使用未定义的变量,但可以使用匿名函数外定义的变量)
调用:
#直接赋值给一个变量,然后再像一般函数使用
符合定义格式的举例:
>>> t =lambda:
True #一、无参匿名函数,直接返回值
>>> t()
True
>>> t = lambda:
x #二、有参数未定义,报错
>>> t()
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
t()
File "<pyshell#0>", line 1, in <lambda>
t = lambda: x
NameError: name 'x' is not defined
>>> x = 10 #可以在匿名函数外定义变量
>>> t()
10
>>> (lambda x: x)(3) #三、定义后直接给变量赋值
3
>>> (lambda x,y: x+y)(3,4)
7
>>> (lambda s:' '.join(s.split()))("this is\na\ttest") #四、表达式也可以是调用的一个函数
'this is a test'
>>> t = lambda x,y=3: x*y #五、允许参数存在默认值
>>> t(2)
6
大量使用实例,加深理解(采用python3):
例子涉及到一些高级函数的使用,因此比较有参考意义
例01: 字符串联合,有默认值,也可以x=(lambda...)这种格式
>>> t = (lambda
x="A",y="B",z="C":
x+y+z)
>>> t("D")
'DBC'
例02: 和列表联合使用
>>> L = [lambda
x:x**2, lambda
x:x**3, lambda x:x**4]
>>>for
fin L:
...print(f(2))
...
4
8
16
也可以如下面这样调用
>>>print
(L[0](3))
9
例03: 和字典结合使用
>>> dic = {'A':lambda:2*2,'B':lambda:2*4,'C':lambda:2*8}
>>> dic['B']()
8
例04: 求最小值
>>> lower = lambda
x,y: x if x<yelsey
>>> lower(10,11)
10
例05: 和map及list联合使用
>>>import
sys
>>> showall =lambda
x:list(map(sys.stdout.write,x))
#python3中map函数返回的是一个迭代器,通过list函数来变成列表
>>> showall(['Jerry\n','Sherry\n','Alice\n'])
#python3中sys.stdout.write 这个函数输出数据到stdout,同时还有一个返回值,就是字符串的长度
Jerry
Sherry
Alice
[6, 7, 6]
等价于下面
>>> showall = lambda x: [sys.stdout.write(line) for line in x]
>>> showall(('I\t','Love\t','You!'))
ILove
You![2, 5, 4]
例06: 在Tkinter中定义内联的callback函数
import tkinter
from tkinter import Button,mainloop
import sys
x = Button(text='Press me',command=(lambda:sys.stdout.write('Hello,World\n')))
x.pack()
x.mainloop()
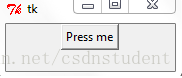
=====================
Hello,World!
Hello,World!
>>>
例07: lambda元组和map联合使用,
>>> out =lambda
*x: sys.stdout.write(' '.join(map(str,x)))
>>> out('This','is','a','book!\n')
This is a book!
16
例08: 判断字符串是否以某个字母开头
>>> print ((lambda
x: x.startswith('B'))('Bob'))
True
-----
>>> Names = ['Anne','Amy',
'Bob', 'David','Carrie',
'Barbara','Zach']
>>> B_Name=filter(lambda
x: x.startswith('B'),Names)
>>> list(B_Name)
#在python2 中直接打印map,filter函数会直接输出结果。但在python3中做了些修改,输出前需要使用list()进行显示转换
['Bob', 'Barbara']
例09: lambda和map联合使用:
>>> squares =list(map(lambda
x:x**2,range(5)))
>>> squares
[0, 1, 4, 9, 16]
例10. lambda和map,filter联合使用:
>>> squares =list(map(lambda
x:x**2,range(10)))
>>> filters =list(filter(lambda
x:x>5 and x<50,squares))
>>> filters
[9, 16, 25, 36, 49]
例11. lambda和sorted联合使用
#按death名单里面,按年龄来排序
#匿名函数的值返回给key,进来排序
>>> death = [ ('James',80),('Alies',60),('Wendy',75)]
>>>sorted(death,key=lambda
age:age[1]) #按照第二个元素,索引为1排序
[('Alies', 60), ('Wendy', 75), ('James', 80)]
例12. lambda和reduce联合使用
>>> from functoolsimport reduce
>>> L = [1,2,3,4]
>>> sum_1 = reduce(lambda x,y:x+y,L)
>>> sum_1
10
例13. 求2-50之间的素数
#素数:只能被1或被自己整除的数
>>> nums =range(2,50)
>>>for
iin nums:
...nums =list(filter(lambda
x:x==i or x % i,nums))
>>> nums
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47]
例14. 求两个列表元素的和
>>> a = [1,2,3,4]
>>> b = [5,6,7,8]
>>>list(map(lambda
x,y:x+y, a,b))
[6, 8, 10, 12]
例15. 求字符串每个单词的长度
>>> sentence ="Welcome To Beijing!"
>>> words = sentence.split()
>>> lengths =list(map(lambda
x:len(x),words))
>>> lengths
[7, 2, 8]
写成一行:
>>>print(list(map(lambda
x:len(x),'Welcome To Beijing!'.split())))
例16. 统计Linux系统挂载点 (不懂,会了以后再来补)
[root@host ~]# mount -v
/dev/mapper/rootVG-root on / type ext3 (rw)
proc on /proc type proc (rw)
sysfs on /sys type sysfs (rw)
devpts on /dev/pts type devpts (rw,gid=5,mode=620)
/dev/mapper/rootVG-tmp on /tmp type ext3 (rw)
/dev/mapper/rootVG-var on /var type ext3 (rw)
/dev/cciss/c0d0p1 on /boot type ext3 (rw)
tmpfs on /dev/shm type tmpfs (rw,size=90%)
>>> import commands
>>> mount = commands.getoutput('mount -v')
>>> lines = mount.splitlines()
>>> point = map(lambda line:line.split()[2],lines)
>>> print point
['/', '/proc', '/sys', '/dev/pts', '/tmp', '/var']
写成一行:
>>> print map(lambda x:x.split()[2],commands.getoutput('mount -v').splitlines())
文中提及的高级函数map、filter、reduce可以参考另一篇文章:
http://blog.csdn.net/bobyuan888/article/details/78181313
本文参考:http://blog.csdn.net/csdnstudent/article/details/40112803
lambda
argument1, argument2,...,argumentN :expression using arguments
可见,匿名函数的变量可以有多个,但表达式通常只有一条,因此匿名函数只能使用在比较简单的情况下,后面的表达式会返回结果
(可以无参,调用时不可以在参数表中赋值;后面表达式不能使用未定义的变量,但可以使用匿名函数外定义的变量)
调用:
#直接赋值给一个变量,然后再像一般函数使用
符合定义格式的举例:
>>> t =lambda:
True #一、无参匿名函数,直接返回值
>>> t()
True
>>> t = lambda:
x #二、有参数未定义,报错
>>> t()
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
t()
File "<pyshell#0>", line 1, in <lambda>
t = lambda: x
NameError: name 'x' is not defined
>>> x = 10 #可以在匿名函数外定义变量
>>> t()
10
>>> (lambda x: x)(3) #三、定义后直接给变量赋值
3
>>> (lambda x,y: x+y)(3,4)
7
>>> (lambda s:' '.join(s.split()))("this is\na\ttest") #四、表达式也可以是调用的一个函数
'this is a test'
>>> t = lambda x,y=3: x*y #五、允许参数存在默认值
>>> t(2)
6
大量使用实例,加深理解(采用python3):
例子涉及到一些高级函数的使用,因此比较有参考意义
例01: 字符串联合,有默认值,也可以x=(lambda...)这种格式
>>> t = (lambda
x="A",y="B",z="C":
x+y+z)
>>> t("D")
'DBC'
例02: 和列表联合使用
>>> L = [lambda
x:x**2, lambda
x:x**3, lambda x:x**4]
>>>for
fin L:
...print(f(2))
...
4
8
16
也可以如下面这样调用
(L[0](3))
9
例03: 和字典结合使用
>>> dic = {'A':lambda:2*2,'B':lambda:2*4,'C':lambda:2*8}
>>> dic['B']()
8
例04: 求最小值
>>> lower = lambda
x,y: x if x<yelsey
>>> lower(10,11)
10
例05: 和map及list联合使用
>>>import
sys
>>> showall =lambda
x:list(map(sys.stdout.write,x))
#python3中map函数返回的是一个迭代器,通过list函数来变成列表
>>> showall(['Jerry\n','Sherry\n','Alice\n'])
#python3中sys.stdout.write 这个函数输出数据到stdout,同时还有一个返回值,就是字符串的长度
Jerry
Sherry
Alice
[6, 7, 6]
等价于下面
>>> showall = lambda x: [sys.stdout.write(line) for line in x]
>>> showall(('I\t','Love\t','You!'))
ILove
You![2, 5, 4]
例06: 在Tkinter中定义内联的callback函数
import tkinter
from tkinter import Button,mainloop
import sys
x = Button(text='Press me',command=(lambda:sys.stdout.write('Hello,World\n')))
x.pack()
x.mainloop()
=====================
Hello,World!
Hello,World!
>>>
例07: lambda元组和map联合使用,
>>> out =lambda
*x: sys.stdout.write(' '.join(map(str,x)))
>>> out('This','is','a','book!\n')
This is a book!
16
例08: 判断字符串是否以某个字母开头
>>> print ((lambda
x: x.startswith('B'))('Bob'))
True
-----
>>> Names = ['Anne','Amy',
'Bob', 'David','Carrie',
'Barbara','Zach']
>>> B_Name=filter(lambda
x: x.startswith('B'),Names)
>>> list(B_Name)
#在python2 中直接打印map,filter函数会直接输出结果。但在python3中做了些修改,输出前需要使用list()进行显示转换
['Bob', 'Barbara']
例09: lambda和map联合使用:
>>> squares =list(map(lambda
x:x**2,range(5)))
>>> squares
[0, 1, 4, 9, 16]
例10. lambda和map,filter联合使用:
>>> squares =list(map(lambda
x:x**2,range(10)))
>>> filters =list(filter(lambda
x:x>5 and x<50,squares))
>>> filters
[9, 16, 25, 36, 49]
例11. lambda和sorted联合使用
#按death名单里面,按年龄来排序
#匿名函数的值返回给key,进来排序
>>> death = [ ('James',80),('Alies',60),('Wendy',75)]
>>>sorted(death,key=lambda
age:age[1]) #按照第二个元素,索引为1排序
[('Alies', 60), ('Wendy', 75), ('James', 80)]
例12. lambda和reduce联合使用
>>> from functoolsimport reduce
>>> L = [1,2,3,4]
>>> sum_1 = reduce(lambda x,y:x+y,L)
>>> sum_1
10
例13. 求2-50之间的素数
#素数:只能被1或被自己整除的数
>>> nums =range(2,50)
>>>for
iin nums:
...nums =list(filter(lambda
x:x==i or x % i,nums))
>>> nums
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47]
例14. 求两个列表元素的和
>>> a = [1,2,3,4]
>>> b = [5,6,7,8]
>>>list(map(lambda
x,y:x+y, a,b))
[6, 8, 10, 12]
例15. 求字符串每个单词的长度
>>> sentence ="Welcome To Beijing!"
>>> words = sentence.split()
>>> lengths =list(map(lambda
x:len(x),words))
>>> lengths
[7, 2, 8]
写成一行:
>>>print(list(map(lambda
x:len(x),'Welcome To Beijing!'.split())))
例16. 统计Linux系统挂载点 (不懂,会了以后再来补)
[root@host ~]# mount -v
/dev/mapper/rootVG-root on / type ext3 (rw)
proc on /proc type proc (rw)
sysfs on /sys type sysfs (rw)
devpts on /dev/pts type devpts (rw,gid=5,mode=620)
/dev/mapper/rootVG-tmp on /tmp type ext3 (rw)
/dev/mapper/rootVG-var on /var type ext3 (rw)
/dev/cciss/c0d0p1 on /boot type ext3 (rw)
tmpfs on /dev/shm type tmpfs (rw,size=90%)
>>> import commands
>>> mount = commands.getoutput('mount -v')
>>> lines = mount.splitlines()
>>> point = map(lambda line:line.split()[2],lines)
>>> print point
['/', '/proc', '/sys', '/dev/pts', '/tmp', '/var']
写成一行:
>>> print map(lambda x:x.split()[2],commands.getoutput('mount -v').splitlines())
文中提及的高级函数map、filter、reduce可以参考另一篇文章:
http://blog.csdn.net/bobyuan888/article/details/78181313
本文参考:http://blog.csdn.net/csdnstudent/article/details/40112803
相关文章推荐
- python3学习笔记:函数作为返回值&匿名函数lambda
- Python学习笔记010——匿名函数lambda
- 【Python学习笔记】函数式编程:匿名函数lambda
- python学习笔记10-匿名函数lambda
- Python学习笔记--匿名函数lambda
- python学习笔记之二:使用字符串
- python2学习笔记 第三章 使用字符串
- Python3.3 学习笔记4 - 函数 - lambda
- Python学习笔记—PyQuery库的使用总结
- python学习--使用 lambda 函数
- 在python中使用lambda来创建匿名函数
- python学习笔记之--os.walk使用
- Python学习笔记(十二):lambda表达式与函数式编程
- python学习笔记3:使用字符串
- Python学习笔记之疑问10:如何使用分隔符连接list中的字符串
- linux 学习笔记 (3) —— 使用python
- python 系统学习笔记(十三)---lambda
- Python 学习笔记【使用元组的注意点】
- Python学习笔记--为什么需要使用__name__=='__main__'
- 我的python学习之路--列表表达式及匿名函数lambda