前备知识 -- Javascript 对象与继承
2017-10-10 08:22
281 查看
很激动能自己开发一款基于canvas的小游戏了,虽然技术实现中很有用到很高深的原理,但是对很多涉及到的知识点都进行了一定程度的深入的学习。那么今天我们就开始进行复习的总结归纳吧!
第一个总结归纳的知识点是,对象。
祭出两周自己觉得很不错的ppt图片:
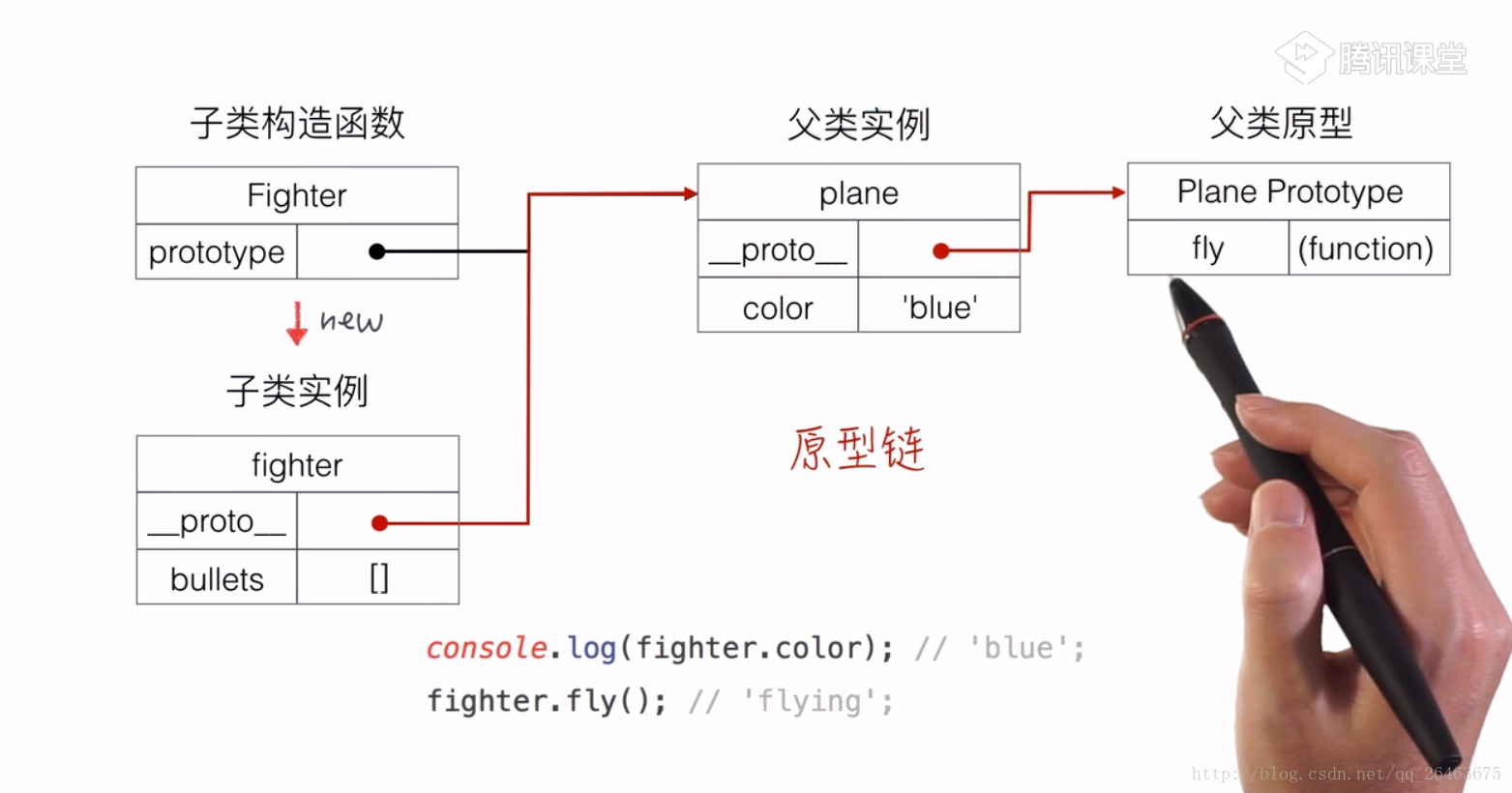
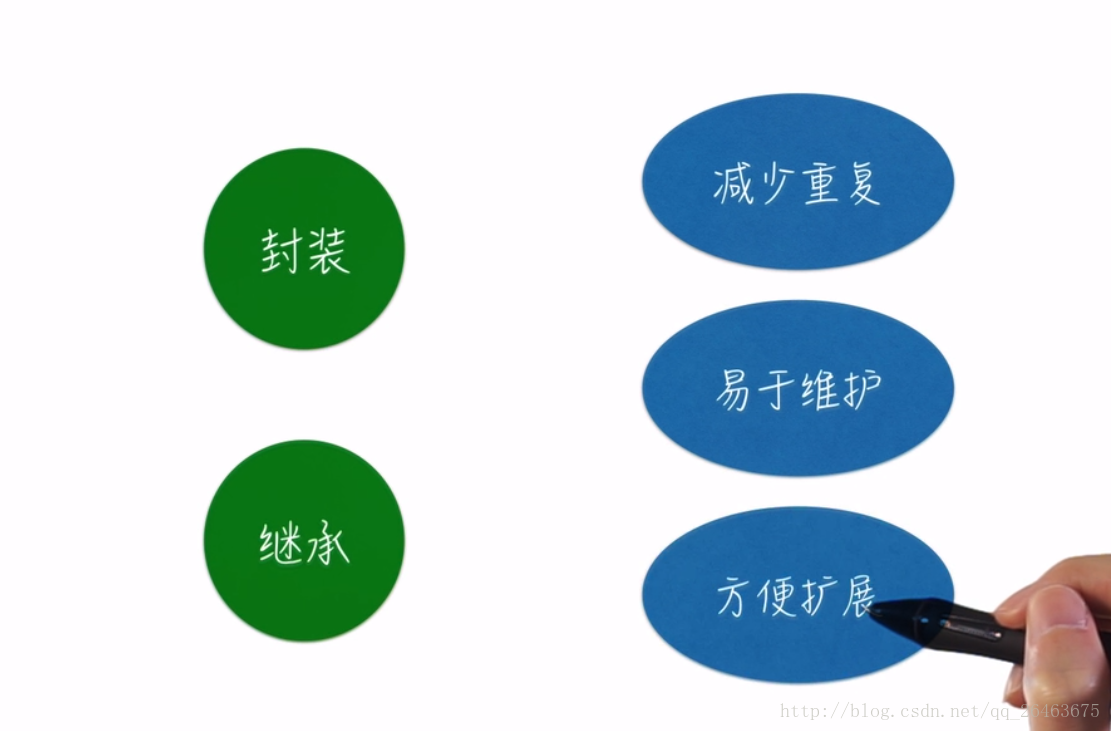
以上是使用了js原生方法函数等生成的对象。在ES6新使用了Class,可以继续了解。
可以说,面向对象编程的方法是很重要的。在游戏设计中,新建对象,渲染对象数据,修改对象数据,重新渲染。
那么下一课,我们将复习的是,如何使用canvas制作动画!
第一个总结归纳的知识点是,对象。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>继承</title> <script src="index.js"></script> </head> <body> <h2>查看控制台</h2> </body> </html>
console.log("it is ok"); //继承:子类具有父类的方法和属性 //父类构造函数 function Plane(color,weight) { this.color = color; this.weight = weight; } Plane.prototype.fly = function() { console.log('flying'); } //子类Fighter 构造函数 function Fighter() { this.buttets = []; } Fighter.prototype = new Plane('blue');//打通原型链 //子类特有方法 Fighter.prototype.shoot = function() { console.log('biu biu biu'< 4000 /span>); } var fighter1 = new Fighter(); console.log(fighter1.color); fighter1.shoot(); //原型链的不足 //1.constructor 指向问题 console.log(fighter1.constructor);//Plane //改进方法 Fighter.prototype.constructor = Fighter; console.log(fighter1.constructor);//Plane //2.属性共享问题 //3.参数 //借用构造函数继承,但是继承不到父类原型链上的方法 function Fighter2(color) { Plane.call(this,color,123); //等等,这里的call函数说明还是没有熟练学到家,还要继续学习call的作用,这里应该是按参数的顺序的吧 this.buttets = []; } var f = new Fighter2('red'); console.log(f); //组合继承 function Fighter3(color) { Plane.call(this,color); //借用构造函数继承实例属性 this.buttets = []; } Fighter3.prototype = new Plane(); Fighter3.prototype.constructor = Fighter3; //继承原型属性和方法 Fighter3.prototype.shoot = function() { console.log('biu biu biu'); } //不足:调用了两次父类的构造函数,父类的属性冗余,例子上color在父类plane中一直被覆盖,是多余的 /* 属性和方法都是从父类继承的(代码复用) 继承的属性是私有的(互补影响) 继承的方法都在原型里(函数复用) */ //最佳实践 /** * Person * @param {String} name */ function Person(name) { this.name = name; } Person.prototype.sayHello = function() { console.log('Hi! My name is ' + this.name + '.'); } /** * TODOS: 改写 Student 类,接收 name 和 grade 属性,并且继承 Person。 * 注意使用下面 inheritPrototype 函数实现继承原型 */ function Student(name, grade) { Person.call(this, name); this.grade = grade; } inheritPrototype(Student, Person); Student.prototype.sayGrade = function() { console.log('I am Grade ' + this.grade + '.'); } // TODOS 完成继承原型的函数 function inheritPrototype(subType, superType){ var prototype = Object.create(superType.prototype); prototype.constructor = subType; subType.prototype = prototype; // var Temp = function() {}; // //为什么要是空函数 // Temp.prototype = superType.prototype; // subType.prototype = new Temp(); // subType.prototype.constructor = subType; } // 可以正确运行下面代码: var student = new Student('Cover', 4); console.log(student.name); // 'Cover' student.sayHello(); // 'Hi! My name is Cover.' student.sayGrade(); // 'I am Grade 4.' student.hasOwnProperty('name'); // true
祭出两周自己觉得很不错的ppt图片:
以上是使用了js原生方法函数等生成的对象。在ES6新使用了Class,可以继续了解。
可以说,面向对象编程的方法是很重要的。在游戏设计中,新建对象,渲染对象数据,修改对象数据,重新渲染。
那么下一课,我们将复习的是,如何使用canvas制作动画!
相关文章推荐
- 前端重点知识整理(JavaScript)四:对象及继承
- JavaScript基础-对象、继承、传值/址基本知识实践
- JavaScript笔记 - 对象继承的几种方式
- javascript面对对象编程 之继承
- Javascript面向对象编程(三):非函数对象的继承
- java基础知识--类和对象、继承(一)
- 由JavaScript中call()方法引发的对面向对象继承机制call的思考
- javascript中用构造函数创建对象以及基类与子类间的继承
- 关于JavaScript的面向对象和继承有利新手学习
- javascript 对象基础 继承机制实例【对象冒充】
- javascript--继承(对象冒充的多重继承)
- 详解JavaScript基础知识(JSON、Function对象、原型、引用类型)
- javascript--继承(对象冒充的多重继承)
- 关于JavaScript的面向对象和继承有利新手学习
- javascript继承对象
- javascript _call2创建对象实现继承
- Javascript之对象的继承
- javascript 对象基础 继承机制实例 call() apply 方法!
- 对JavaScript面对对象#继承的理解
- JavaScript之继承、this问题和对象枚举