使用Newtonsoft.Json进行JSON反序列化操作
2017-10-06 16:25
316 查看
本篇主要介绍两种使用Newtonsoft.Json进行JSON格式文件反序列化的操作。
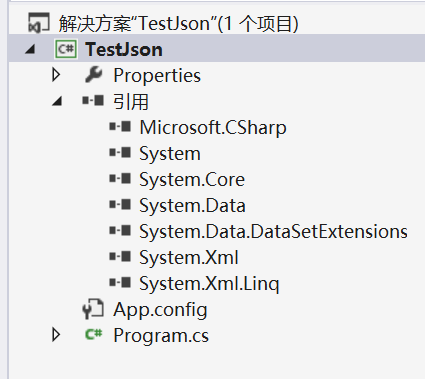
2)添加Newtonsoft.Json引用,并添加类TestTag.cs
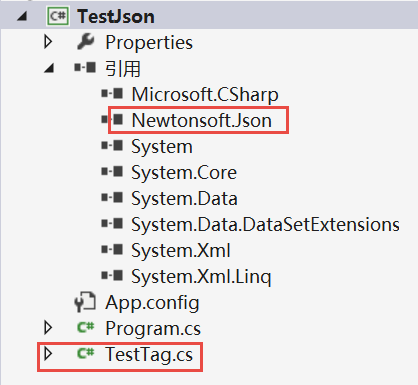
在TestTag类中添加属性:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace TestJson
{
public class TestTag
{
//类中添加以下属性
public int ViewId { get; set; }
public string Name { get; set; }
public bool IsValid { get; set; }
public int SeqNum { get; set; }
}
}
3)在Program主程序中实现反序列化
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace TestJson
{
class Program
{
static void Main(string[] args)
{
//Json对象
string strJson = "[{'ViewId':01,'Name':'Test 1','IsValid':true,'SeqNum':'01'},{'ViewId':02,'Name':'Test 2','IsValid':false,'SeqNum':'02'}]";
List<TestTag> listView = JsonConvert.DeserializeObject<List<TestTag>>(strJson);
foreach (var view in listView)
{
Console.WriteLine("ViewId:{0}", view.ViewId);
Console.WriteLine("Name:{0}", view.Name);
Console.WriteLine("IsValid:{0}", view.IsValid);
Console.WriteLine("SeqNum:{0}", view.SeqNum);
}
Console.ReadKey();
}
}
}
4)输出显示
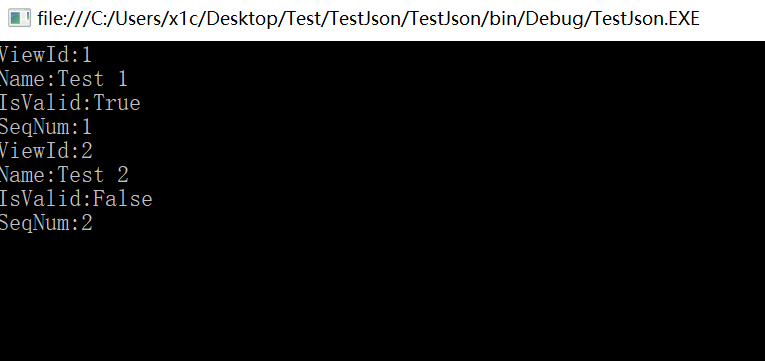
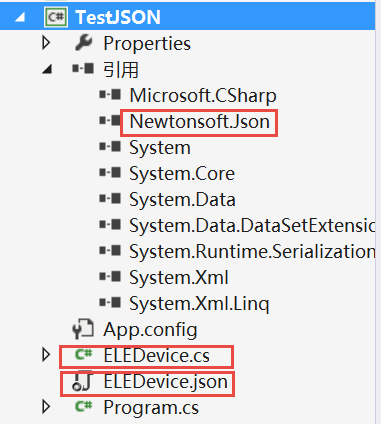
2)输入JSON格式文件(ELEDevice.json)对象
[
{
"ELEName": "ELE1",
"ELEType": "cc25",
"PortName": "COM3",
"BaudRate": 115200
},
{
"ELEName": "ELE2",
"ELEType": "ss70",
"PortName": "COM4",
"BaudRate": 10000
}
]
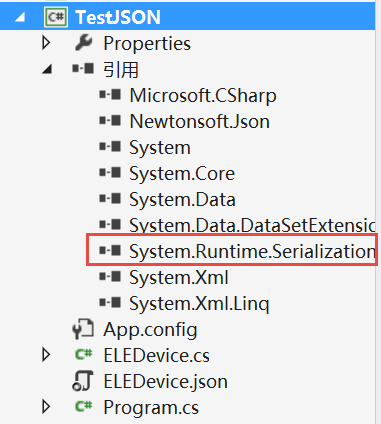
b. 在类添加json反序列化属性
注意:上面代码中序列化类和属性定义之前要添加[DataContract]与[DataMember]
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace TestJSON
{
class Program
{
static void Main(string[] args)
{
List<ELEDevice> devs = ELEDevice.Deserialize(@"..\..\ELEDevice.json");
foreach (var ELE in devs)
{
Console.WriteLine(string.Format("ELEName:{0}", ELE.ELEName));
Console.WriteLine(string.Format("ELEType:{0}", ELE.ELEType));
Console.WriteLine(string.Format("PortName:{0}", ELE.PortName));
Console.WriteLine(string.Format("BaudRate:{0}", ELE.BaudRate));
Console.WriteLine();
}
Console.ReadKey();
}
}
}
1. 直接将程序中string格式的JSON字段进行反序列化
1)在VS中创建TestJSON的控制台应用程序2)添加Newtonsoft.Json引用,并添加类TestTag.cs
在TestTag类中添加属性:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace TestJson
{
public class TestTag
{
//类中添加以下属性
public int ViewId { get; set; }
public string Name { get; set; }
public bool IsValid { get; set; }
public int SeqNum { get; set; }
}
}
3)在Program主程序中实现反序列化
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace TestJson
{
class Program
{
static void Main(string[] args)
{
//Json对象
string strJson = "[{'ViewId':01,'Name':'Test 1','IsValid':true,'SeqNum':'01'},{'ViewId':02,'Name':'Test 2','IsValid':false,'SeqNum':'02'}]";
List<TestTag> listView = JsonConvert.DeserializeObject<List<TestTag>>(strJson);
foreach (var view in listView)
{
Console.WriteLine("ViewId:{0}", view.ViewId);
Console.WriteLine("Name:{0}", view.Name);
Console.WriteLine("IsValid:{0}", view.IsValid);
Console.WriteLine("SeqNum:{0}", view.SeqNum);
}
Console.ReadKey();
}
}
}
4)输出显示
2. 将JSON格式外部文件进行反序列化
1)同步骤1所示,创建工程TestJSON,引入库Newtonsoft.Json,创建类ELEDevice.cs与JSON外部文件ELEDevice.json2)输入JSON格式文件(ELEDevice.json)对象
[
{
"ELEName": "ELE1",
"ELEType": "cc25",
"PortName": "COM3",
"BaudRate": 115200
},
{
"ELEName": "ELE2",
"ELEType": "ss70",
"PortName": "COM4",
"BaudRate": 10000
}
]
3)输入ELEDevice.cs类的属性及反序列化方法对象
a. 添加引用类库b. 在类添加json反序列化属性
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Runtime.Serialization; using Newtonsoft.Json; using System.IO; namespace TestJSON { [DataContract] public class ELEDevice { /// <summary> /// 名称 /// </summary> [DataMember] public string ELEName { get;set;} /// <summary> /// 类型 /// </summary> [DataMember] public string ELEType { get; set; } /// <summary> /// 串口名 /// </summary> [DataMember] public string PortName { get; set; } /// <summary> /// 波特率 /// </summary> [DataMember] public int BaudRate { get; set; } /// <summary> /// 反序列化操作 /// </summary> /// <param name="fileName">外部Json文件</param> /// <returns></returns> public static List<ELEDevice> Deserialize(string fileName) { using(StreamReader fs = new StreamReader(fileName, Encoding.UTF8, true)) { string str = fs. 982d ReadToEnd(); List<ELEDevice> devs = JsonConvert.DeserializeObject<List<ELEDevice>>(str); return devs; } } /// <summary> /// 序列化操作 /// </summary> /// <param name="fileName">外部Json文件</param> /// <param name="devices">序列化对象</param> public static void Serialize(string fileName, List<ELEDevice> devices) { using (StreamWriter fs = new StreamWriter(fileName)) { //将序列化后的Json格式数据写入流中 string str = JsonConvert.SerializeObject(devices, Formatting.Indented); fs.Write(str); } } } }
注意:上面代码中序列化类和属性定义之前要添加[DataContract]与[DataMember]
4)在Program.cs主程序中实现
using System;using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
namespace TestJSON
{
class Program
{
static void Main(string[] args)
{
List<ELEDevice> devs = ELEDevice.Deserialize(@"..\..\ELEDevice.json");
foreach (var ELE in devs)
{
Console.WriteLine(string.Format("ELEName:{0}", ELE.ELEName));
Console.WriteLine(string.Format("ELEType:{0}", ELE.ELEType));
Console.WriteLine(string.Format("PortName:{0}", ELE.PortName));
Console.WriteLine(string.Format("BaudRate:{0}", ELE.BaudRate));
Console.WriteLine();
}
Console.ReadKey();
}
}
}
5)控制台显示输出
相关文章推荐
- mvc 使用Newtonsoft.Json进行序列化json数据
- .Net——使用DataContractJsonSerializer进行序列化及反序列化基本操作
- mvc 使用Newtonsoft.Json进行序列化json数据
- Asp.Net Core中使用Newtonsoft.Json进行序列化处理解决返回值首字母小写
- C#使用NewtonSoft进行Json序列化,设置字段首字母小写方法
- .Net——使用DataContractJsonSerializer进行序列化及反序列化基本操作
- 关于SubSonic3.0插件使用Json反序列化获得的实体进行更新操作时,只能执行添加而不能执行修改(编辑)操作的处理
- .Net——使用DataContractJsonSerializer进行序列化及反序列化基本操作
- 关于SubSonic3.0插件使用Json反序列化获得的实体进行更新操作时,只能执行添加而不能执行修改(编辑)操作的处理
- 使用NewtonSoft.JSON.dll来序列化和发序列化对象
- 使用NewtonSoft.JSON.dll来序列化和反序列化对象
- 使用JavaScriptSerializer进行JSON序列化
- mvc “使用 JSON JavaScriptSerializer 进行序列化或反序列化时出错,字符串的长度超过了为 maxJsonLength 属性设置的值” 解决经历
- 使用 JSON JavaScriptSerializer 进行序列化或反序列化时出错。字符串的长度超过了为 maxJsonLength 属性设置的值
- c#操作json使用newtonsoft.json
- 使用NewtonSoft.JSON.dll来序列化和发序列化对象
- 解决“使用 JSON JavaScriptSerializer 进行序列化或反序列化时出错”的问题
- ASP.NET:使用Newtonsoft.Json序列化和反序列化JSON对象的例子
- 使用NewtonSoft.JSON.dll来序列化和发序列化对象(转载)
- 使用JavaScriptSerializer进行JSON序列化