Android:下拉上滑显示与隐藏导航栏和状态栏
2017-09-30 10:38
393 查看
话不多说先看效果:
小米商店效果图:
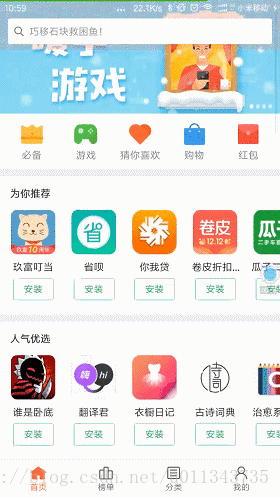
个人实现效果图:
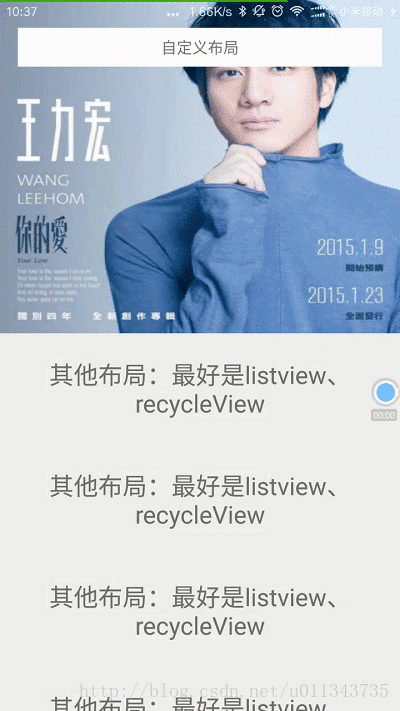
前几天小米手机应用商店更新,看到这样的效果,之后看到其他app也有这样的效果,闲来无事搞一下。
首先怎么实现呢?
思路:不考虑滑动,先要实现全屏,而且上方状态栏显示,之后重写Scrollview,监听滑动事件,当滑动一定距离时不断更新action bar的透明度。
第一步实现全屏:全屏样式实现参考
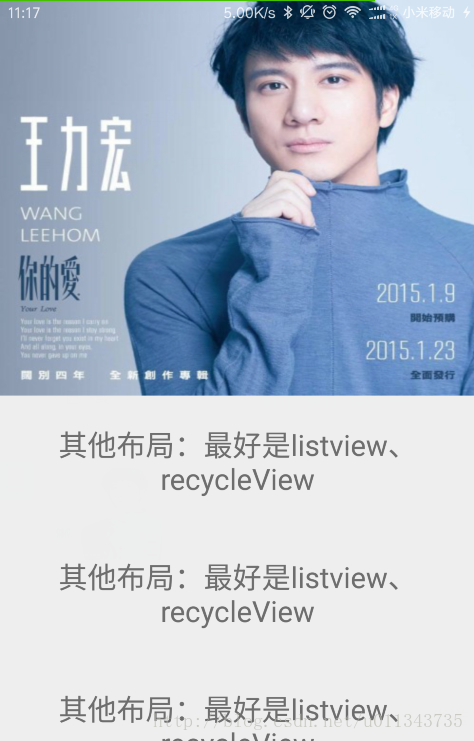
主要代码:
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
然后就是重写ScrollView:
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
布局文件:
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
activity 使用:
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
说一下上方所谓的action bar,这里并没有直接使用actionbar,而是使用自己的布局,因为使用action bar ,就要在activity中对它进行声明设置一些属性,而要实现状态栏透明 这样的效果,代码中已经设置actionbar hide,可能会起冲突,所以在写demo时就直接放弃了actionbar。
这样就大功告成了,如果有说的不正确的地方,欢迎留言一起讨论!
小米商店效果图:
个人实现效果图:
前几天小米手机应用商店更新,看到这样的效果,之后看到其他app也有这样的效果,闲来无事搞一下。
首先怎么实现呢?
思路:不考虑滑动,先要实现全屏,而且上方状态栏显示,之后重写Scrollview,监听滑动事件,当滑动一定距离时不断更新action bar的透明度。
第一步实现全屏:全屏样式实现参考
主要代码:
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); supportRequestWindowFeature(Window.FEATURE_ACTION_BAR_OVERLAY); setContentView(R.layout.activity_nac); if (Build.VERSION.SDK_INT >= 21) { View decorView = getWindow().getDecorView(); int option = View.SYSTEM_UI_FLAG_LAYOUT_HIDE_NAVIGATION | View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN | View.SYSTEM_UI_FLAG_LAYOUT_STABLE; decorView.setSystemUiVisibility(option); getWindow().setNavigationBarColor(Color.TRANSPARENT); getWindow().setStatusBarColor(Color.TRANSPARENT); } ActionBar actionBar = getSupportActionBar(); actionBar.hide(); }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
然后就是重写ScrollView:
import android.content.Context; import android.util.AttributeSet; import android.util.Log; import android.util.LogPrinter; import android.view.MotionEvent; import android.view.View; import android.widget.ScrollView; /** * Created by ZHL on 2016/12/19. */ public class FadingScrollView extends ScrollView { private static String TAG = "-----------FadingScrollView----------"; //渐变view private View fadingView; //滑动view的高度,如果这里fadingHeightView是一张图片, // 那么就是这张图片上滑至完全消失时action bar 完全显示, // 过程中透明度不断增加,直至完全显示 private View fadingHeightView; private int oldY; //滑动距离,默认设置滑动500 时完全显示,根据实际需求自己设置 private int fadingHeight = 500; public FadingScrollView(Context context) { super(context); } public FadingScrollView(Context context, AttributeSet attrs) { super(context, attrs); } public FadingScrollView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); } public void setFadingView(View view){this.fadingView = view;} public void setFadingHeightView(View v){this.fadingHeightView = v;} @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { super.onMeasure(widthMeasureSpec, heightMeasureSpec); if(fadingHeightView != null) fadingHeight = fadingHeightView.getMeasuredHeight(); } @Override protected void onScrollChanged(int l, int t, int oldl, int oldt) { super.onScrollChanged(l, t, oldl, oldt); // l,t 滑动后 xy位置, // oldl lodt 滑动前 xy 位置----- float fading = t>fadingHeight ? fadingHeight : (t > 30 ? t : 0); updateActionBarAlpha( fading / fadingHeight); } void updateActionBarAlpha(float alpha){ try { setActionBarAlpha(alpha); } catch (Exception e) { e.printStackTrace(); } } public void setActionBarAlpha(float alpha) throws Exception{ if(fadingView==null){ throw new Exception("fadingView is null..."); } fadingView.setAlpha(alpha); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
布局文件:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_nac" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.qienanxiang.activity.NacActivity"> <com.qienanxiang.widget.FadingScrollView android:id="@+id/nac_root" android:overScrollMode="never" android:scrollbars="none" android:layout_width="match_parent" android:layout_height="wrap_content"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <ImageView android:id="@+id/nac_image" android:layout_width="match_parent" android:layout_height="300dp" android:scaleType="fitXY" android:src="@mipmap/icon_bg2"/> <TextView android:layout_width="match_parent" android:layout_height="100dp" android:gravity="center" android:textSize="22dp" android:text="其他布局:最好是listview、recycleView"/> <!--...... 建议这里放置listview 或者 recycleView ,LZ为了省事直接放了多个textview来撑起ScrollView。。。--!> </LinearLayout> </com.qienanxiang.widget.FadingScrollView> <FrameLayout android:layout_width="match_parent" android:layout_height="70dp" android:background="@android:color/transparent"> <View android:id="@+id/nac_layout" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@color/colorPrimary"/> <TextView android:layout_marginRight="@dimen/activity_horizontal_margin" android:layout_marginLeft="@dimen/activity_horizontal_margin" android:layout_marginTop="25dp" android:layout_marginBottom="10dp" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:background="#FFFFFF" android:text="自定义布局"/> </FrameLayout> </RelativeLayout>1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
activity 使用:
public class NacActivity extends AppCompatActivity { private View layout; private FadingScrollView fadingScrollView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); supportRequestWindowFeature(Window.FEATURE_ACTION_BAR_OVERLAY); setContentView(R.layout.activity_nac); if (Build.VERSION.SDK_INT >= 21) { View decorView = getWindow().getDecorView(); int option = View.SYSTEM_UI_FLAG_LAYOUT_HIDE_NAVIGATION | View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN | View.SYSTEM_UI_FLAG_LAYOUT_STABLE; decorView.setSystemUiVisibility(option); getWindow().setNavigationBarColor(Color.TRANSPARENT); getWindow().setStatusBarColor(Color.TRANSPARENT); } ActionBar actionBar = getSupportActionBar(); actionBar.hide(); layout = findViewById(R.id.nac_layout); layout.setAlpha(0); fadingScrollView = (FadingScrollView)findViewById(R.id.nac_root); fadingScrollView.setFadingView(layout); fadingScrollView.setFadingHeightView(findViewById(R.id.nac_image)); } }1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
说一下上方所谓的action bar,这里并没有直接使用actionbar,而是使用自己的布局,因为使用action bar ,就要在activity中对它进行声明设置一些属性,而要实现状态栏透明 这样的效果,代码中已经设置actionbar hide,可能会起冲突,所以在写demo时就直接放弃了actionbar。
这样就大功告成了,如果有说的不正确的地方,欢迎留言一起讨论!
相关文章推荐
- Android:下拉上滑显示与隐藏导航栏和状态栏
- android 隐藏状态栏 和导航栏 触碰屏幕边界不被显示
- Android动态控制状态栏以及系统导航栏显示和隐藏
- Android 底部导航栏动态显示和隐藏(上滑,下拉)
- android 状态栏 导航栏隐藏显示控制方法及效果示例
- Android 动态隐藏显示导航栏,状态栏
- Android 动态隐藏显示导航栏,状态栏
- Android App 隐藏标题栏+状态栏+导航栏+获取状态栏的三种方法
- android 命令隐藏导航栏以及显示电量
- android 隐藏导航栏 状态栏 标题栏
- Android下拉上滑显示与隐藏Toolbar另一种实现
- android之隐藏状态栏,全屏显示和隐藏虚拟按键
- Android隐藏状态栏、导航栏
- Android 状态栏的动态显示和隐藏
- SystemUI中状态栏跟导航栏隐藏显示控制方法及效果示例
- 隐藏android系统的状态栏和导航栏
- android 开机后第一次显示锁屏界面时点击紧急呼叫,下拉状态栏,不停的闪屏
- 隐藏android系统的状态栏和导航栏
- Android实现系统状态栏的隐藏和显示功能
- Android App 隐藏标题栏+状态栏+导航栏