Servlet中的service方法
2017-09-21 13:19
197 查看
Servlet中的service方法用于应答浏览器请求,每次请求都会调用该方法。
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
}
}ServletRequest:封装了请求信息,可以从中获取到任何的请求信息。
ServletResponse:封装了响应信息,用于响应用户请求。
这两个接口都是服务器给予实现的,并在服务器调用service方法时传入。
1.ServletRequest:
1.1 获取请求参数:
1.1.1 String getParameter(String name); 根据名字获取值
html:
<!DOCTYPE html>
<html>
<head>
<title>login.html</title>
<meta name="keywords" content="keyword1,keyword2,keyword3">
<meta name="description" content="this is my page">
<meta name="content-type" content="text/html; charset=UTF-8">
<!--<link rel="stylesheet" type="text/css" href="./styles.css">-->
</head>
<body>
<form name="f1" id="f1" action="login" method="post">
<table>
<tr>
<td>Login:</td>
<td><input type="text" name="login" id="login"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password" id="password"></td>
</tr>
<tr>
<td colspan="2"><input type="submit"></td>
</tr>
</table>
</form>
</body>
</html>
xml:
<!-- 配置和映射servlet -->
<servlet>
<!-- servlet注册的名字 -->
<servlet-name>loginServlet</servlet-name>
<!-- servlet的全类名-->
<servlet-class>com.sa.servlet.LoginServlet</servlet-class>
</servlet>
<servlet-mapping>
<!-- 需要和某一个servlet节点的servlet子节点的文本节点一致 -->
<servlet-name>loginServlet</servlet-name>
<!-- 映射具体 的访问路径: / 代表当前web应用的根目录 -->
<url-pattern>/login</url-pattern>
</servlet-mapping> LoginServlet:
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
String login=request.getParameter("login");
String password=request.getParameter("password");
System.out.println("login:"+login);
System.out.println("password:"+password);
}
输出结果:
login:admin
password:123
1.1.2 Map<String, String> getParameterMap();返回请求参数的键值对。
key:参数名,value:参数值,String[] 类型
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
Map<String, String[]> map=request.getParameterMap();
for (String key : map.keySet()) {
System.out.println("key= "+ key + " and value= " + Arrays.toString(map.get(key)));
}
}
输出结果:
key= login and value= [admin]
key= password and value= [123]
key= interesting and value= [Party, Shopping]
1.1.3 Enumeration<String> getParameterNames();返回参数名对应的Enumeration对象。
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO A
4000
uto-generated method stub
//Map<String, String> request.getParameterMap();
//Enumeration<String> request.getParameterNames();
//String[] request.getParameterValues(arg0);
Enumeration<String> enums=request.getParameterNames();
while(enums.hasMoreElements()){
String name=enums.nextElement();
String[] value=request.getParameterValues(name);
System.out.println("name:"+name+",value:"+Arrays.toString(value));
}查看结果:
name:login,value:[admin]
name:password,value:[123]
name:interesting,value:[Playing, Party]
1.1.4 String[] getParameterValues(String name);根据名字获取值数组,例如checkbox
html:
<!DOCTYPE html>
<html>
<head>
<title>login.html</title>
<meta name="keywords" content="keyword1,keyword2,keyword3">
<meta name="description" content="this is my page">
<meta name="content-type" content="text/html; charset=UTF-8">
<!--<link rel="stylesheet" type="text/css" href="./styles.css">-->
</head>
<body>
<form name="f1" id="f1" action="login" method="post">
<table>
<tr>
<td>Login:</td>
<td><input type="text" name="login" id="login"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password" id="password"></td>
</tr>
<tr>
<td>interesting:</td>
<td>
<input type="checkbox" name="interesting" value="Playing"/>Playing
<input type="checkbox" name="interesting" value="TV"/>TV
<input type="checkbox" name="interesting" value="Party"/>Party
<input type="checkbox" name="interesting" value="Sport"/>Sport
<input type="checkbox" name="interesting" value="Shopping"/>Shopping
</td>
</tr>
<tr>
<td colspan="2"><input type="submit"></td>
</tr>
</table>
</form>
</body>
</html>
LoginServlet:
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
String login=request.getParameter("login");
String password=request.getParameter("password");
String[] interesting=request.getParameterValues("interesting");
System.out.println("login:"+login);
System.out.println("password:"+password);
System.out.println("interesting:"+Arrays.toString(interesting));
}输出结果:
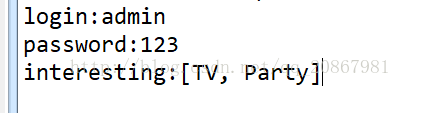
1.2 获取请求的URI:
HttpServletRequest是ServletRequest的子接口,针对于HTTP请求所定义,里面有
大量获取HTTP信息的方法。
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
HttpServletRequest httpServletRequest=(HttpServletRequest) request;
String uri=httpServletRequest.getRequestURI();
System.out.println("uri:"+uri);
}
输出结果:
1.3 获取请求方式:
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
HttpServletRequest httpServletRequest=(HttpServletRequest) request;
String method=httpServletRequest.getMethod();
System.out.println("method:"+method);
}输出结果:
method:POST
2.ServletReponse:
2.1 getWriter():
返回PrintWriter对象,调用该对象的print()方法,把print()中参数打印在网页上。
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
PrintWriter writer=response.getWriter();
writer.println("hello world");
}查看页面:
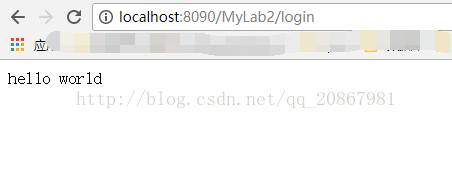
2.2 setContentType():
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
response.setContentType("application/msword");
PrintWriter writer=response.getWriter();
writer.println("hello world");
}设置为内容类型为“application/msword”,这样返回的内容下载下来:
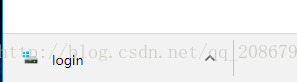
2.3 void sendRedirect(String location):
请求的重定向。(此方法为HttpServletResponse中定义)
在web.xml文件中设置两个WEB应用的初始化参数:user,password.
定义一个login.html,里边定义两个请求字段:user,password,发送请求到loginServlet.
在loginServlet中,获取请求的user,password,比较其和web.xml文件中的初始化参数是否一致,
若一致,则响应: hello,xxx;若不一致,则响应: sorry ,xxx;
html:
<!DOCTYPE html>
<html>
<head>
<title>login.html</title>
<meta name="keywords" content="keyword1,keyword2,keyword3">
<meta name="description" content="this is my page">
<meta name="content-type" content="text/html; charset=UTF-8">
<!--<link rel="stylesheet" type="text/css" href="./styles.css">-->
</head>
<body>
<form name="f1" id="f1" action="login" method="post">
<table>
<tr>
<td>Login:</td>
<td><input type="text" name="login" id="login"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password" id="password"></td>
</tr>
<tr>
<td colspan="2"><input type="submit"></td>
</tr>
</table>
</form>
</body>
</html>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<!-- 配置当前WEB应用的参数 -->
<context-param>
<param-name>user</param-name>
<param-value>sasa</param-value>
</context-param>
<context-param>
<param-name>password</param-name>
<param-value>123</param-value>
</context-param>
<!-- 配置和映射servlet -->
<servlet>
<!-- servlet注册的名字 -->
<servlet-name>loginServlet</servlet-name>
<!-- servlet的全类名-->
<servlet-class>com.sa.servlet.LoginServlet</servlet-class>
</servlet>
<servlet-mapping>
<!-- 需要和某一个servlet节点的servlet子节点的文本节点一致 -->
<servlet-name>loginServlet</servlet-name>
<!-- 映射具体 的访问路径: / 代表当前web应用的根目录 -->
<url-pattern>/login</url-pattern>
</servlet-mapping>
</web-app>LoginServlet:
package com.sa.servlet;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.Arrays;
import java.util.Enumeration;
import java.util.Map;
import javax.servlet.Servlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class LoginServlet implements Servlet {
public LoginServlet(){
}
@Override
public void destroy() {
// TODO Auto-generated method stub
}
@Override
public ServletConfig getServletConfig() {
// TODO Auto-generated method stub
return null;
}
@Override
public String getServletInfo() {
// TODO Auto-generated method stub
return null;
}
@Override
public void init(ServletConfig arg0) throws ServletException {
// TODO Auto-generated method stub
}
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
String user=request.getParameter("login");
String password=request.getParameter("password");
ServletContext servletContext=request.getServletContext();
String user2=servletContext.getInitParameter("user");
String password2=servletContext.getInitParameter("password");
//HttpServletResponse httpServletResponse=(HttpServletResponse) response;
PrintWriter writer=response.getWriter();
if(user.equals(user2)&&password.equals(password2)){
writer.println("hello,"+user);
}else{
writer.println("sorry,"+user);
}
}
}
测试:
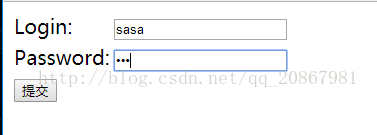
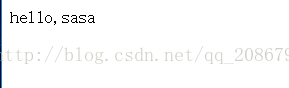
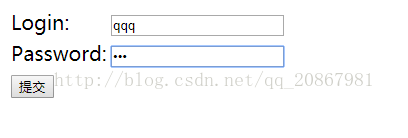
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
}
}ServletRequest:封装了请求信息,可以从中获取到任何的请求信息。
ServletResponse:封装了响应信息,用于响应用户请求。
这两个接口都是服务器给予实现的,并在服务器调用service方法时传入。
1.ServletRequest:
1.1 获取请求参数:
1.1.1 String getParameter(String name); 根据名字获取值
html:
<!DOCTYPE html>
<html>
<head>
<title>login.html</title>
<meta name="keywords" content="keyword1,keyword2,keyword3">
<meta name="description" content="this is my page">
<meta name="content-type" content="text/html; charset=UTF-8">
<!--<link rel="stylesheet" type="text/css" href="./styles.css">-->
</head>
<body>
<form name="f1" id="f1" action="login" method="post">
<table>
<tr>
<td>Login:</td>
<td><input type="text" name="login" id="login"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password" id="password"></td>
</tr>
<tr>
<td colspan="2"><input type="submit"></td>
</tr>
</table>
</form>
</body>
</html>
xml:
<!-- 配置和映射servlet -->
<servlet>
<!-- servlet注册的名字 -->
<servlet-name>loginServlet</servlet-name>
<!-- servlet的全类名-->
<servlet-class>com.sa.servlet.LoginServlet</servlet-class>
</servlet>
<servlet-mapping>
<!-- 需要和某一个servlet节点的servlet子节点的文本节点一致 -->
<servlet-name>loginServlet</servlet-name>
<!-- 映射具体 的访问路径: / 代表当前web应用的根目录 -->
<url-pattern>/login</url-pattern>
</servlet-mapping> LoginServlet:
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
String login=request.getParameter("login");
String password=request.getParameter("password");
System.out.println("login:"+login);
System.out.println("password:"+password);
}
输出结果:
login:admin
password:123
1.1.2 Map<String, String> getParameterMap();返回请求参数的键值对。
key:参数名,value:参数值,String[] 类型
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
Map<String, String[]> map=request.getParameterMap();
for (String key : map.keySet()) {
System.out.println("key= "+ key + " and value= " + Arrays.toString(map.get(key)));
}
}
输出结果:
key= login and value= [admin]
key= password and value= [123]
key= interesting and value= [Party, Shopping]
1.1.3 Enumeration<String> getParameterNames();返回参数名对应的Enumeration对象。
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO A
4000
uto-generated method stub
//Map<String, String> request.getParameterMap();
//Enumeration<String> request.getParameterNames();
//String[] request.getParameterValues(arg0);
Enumeration<String> enums=request.getParameterNames();
while(enums.hasMoreElements()){
String name=enums.nextElement();
String[] value=request.getParameterValues(name);
System.out.println("name:"+name+",value:"+Arrays.toString(value));
}查看结果:
name:login,value:[admin]
name:password,value:[123]
name:interesting,value:[Playing, Party]
1.1.4 String[] getParameterValues(String name);根据名字获取值数组,例如checkbox
html:
<!DOCTYPE html>
<html>
<head>
<title>login.html</title>
<meta name="keywords" content="keyword1,keyword2,keyword3">
<meta name="description" content="this is my page">
<meta name="content-type" content="text/html; charset=UTF-8">
<!--<link rel="stylesheet" type="text/css" href="./styles.css">-->
</head>
<body>
<form name="f1" id="f1" action="login" method="post">
<table>
<tr>
<td>Login:</td>
<td><input type="text" name="login" id="login"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password" id="password"></td>
</tr>
<tr>
<td>interesting:</td>
<td>
<input type="checkbox" name="interesting" value="Playing"/>Playing
<input type="checkbox" name="interesting" value="TV"/>TV
<input type="checkbox" name="interesting" value="Party"/>Party
<input type="checkbox" name="interesting" value="Sport"/>Sport
<input type="checkbox" name="interesting" value="Shopping"/>Shopping
</td>
</tr>
<tr>
<td colspan="2"><input type="submit"></td>
</tr>
</table>
</form>
</body>
</html>
LoginServlet:
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
String login=request.getParameter("login");
String password=request.getParameter("password");
String[] interesting=request.getParameterValues("interesting");
System.out.println("login:"+login);
System.out.println("password:"+password);
System.out.println("interesting:"+Arrays.toString(interesting));
}输出结果:
1.2 获取请求的URI:
HttpServletRequest是ServletRequest的子接口,针对于HTTP请求所定义,里面有
大量获取HTTP信息的方法。
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
HttpServletRequest httpServletRequest=(HttpServletRequest) request;
String uri=httpServletRequest.getRequestURI();
System.out.println("uri:"+uri);
}
输出结果:
uri:/MyLab2/login
1.3 获取请求方式:
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
HttpServletRequest httpServletRequest=(HttpServletRequest) request;
String method=httpServletRequest.getMethod();
System.out.println("method:"+method);
}输出结果:
method:POST
2.ServletReponse:
2.1 getWriter():
返回PrintWriter对象,调用该对象的print()方法,把print()中参数打印在网页上。
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
PrintWriter writer=response.getWriter();
writer.println("hello world");
}查看页面:
2.2 setContentType():
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
response.setContentType("application/msword");
PrintWriter writer=response.getWriter();
writer.println("hello world");
}设置为内容类型为“application/msword”,这样返回的内容下载下来:
2.3 void sendRedirect(String location):
请求的重定向。(此方法为HttpServletResponse中定义)
在web.xml文件中设置两个WEB应用的初始化参数:user,password.
定义一个login.html,里边定义两个请求字段:user,password,发送请求到loginServlet.
在loginServlet中,获取请求的user,password,比较其和web.xml文件中的初始化参数是否一致,
若一致,则响应: hello,xxx;若不一致,则响应: sorry ,xxx;
html:
<!DOCTYPE html>
<html>
<head>
<title>login.html</title>
<meta name="keywords" content="keyword1,keyword2,keyword3">
<meta name="description" content="this is my page">
<meta name="content-type" content="text/html; charset=UTF-8">
<!--<link rel="stylesheet" type="text/css" href="./styles.css">-->
</head>
<body>
<form name="f1" id="f1" action="login" method="post">
<table>
<tr>
<td>Login:</td>
<td><input type="text" name="login" id="login"></td>
</tr>
<tr>
<td>Password:</td>
<td><input type="password" name="password" id="password"></td>
</tr>
<tr>
<td colspan="2"><input type="submit"></td>
</tr>
</table>
</form>
</body>
</html>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0">
<!-- 配置当前WEB应用的参数 -->
<context-param>
<param-name>user</param-name>
<param-value>sasa</param-value>
</context-param>
<context-param>
<param-name>password</param-name>
<param-value>123</param-value>
</context-param>
<!-- 配置和映射servlet -->
<servlet>
<!-- servlet注册的名字 -->
<servlet-name>loginServlet</servlet-name>
<!-- servlet的全类名-->
<servlet-class>com.sa.servlet.LoginServlet</servlet-class>
</servlet>
<servlet-mapping>
<!-- 需要和某一个servlet节点的servlet子节点的文本节点一致 -->
<servlet-name>loginServlet</servlet-name>
<!-- 映射具体 的访问路径: / 代表当前web应用的根目录 -->
<url-pattern>/login</url-pattern>
</servlet-mapping>
</web-app>LoginServlet:
package com.sa.servlet;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.Arrays;
import java.util.Enumeration;
import java.util.Map;
import javax.servlet.Servlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class LoginServlet implements Servlet {
public LoginServlet(){
}
@Override
public void destroy() {
// TODO Auto-generated method stub
}
@Override
public ServletConfig getServletConfig() {
// TODO Auto-generated method stub
return null;
}
@Override
public String getServletInfo() {
// TODO Auto-generated method stub
return null;
}
@Override
public void init(ServletConfig arg0) throws ServletException {
// TODO Auto-generated method stub
}
@Override
public void service(ServletRequest request, ServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
String user=request.getParameter("login");
String password=request.getParameter("password");
ServletContext servletContext=request.getServletContext();
String user2=servletContext.getInitParameter("user");
String password2=servletContext.getInitParameter("password");
//HttpServletResponse httpServletResponse=(HttpServletResponse) response;
PrintWriter writer=response.getWriter();
if(user.equals(user2)&&password.equals(password2)){
writer.println("hello,"+user);
}else{
writer.println("sorry,"+user);
}
}
}
测试:
相关文章推荐
- servlet中的service, doGet, doPost方法的区别和联系
- 模拟Servlet单实例多线程运行service方法
- servlet中service() doGet() doPost() 方法
- [需要补充]javaEE中servlet方法service与doXXX的关系
- 在servlet中出现service()、doGet()和doPost()方法时的执行问题
- myeclipse实现Servlet实例(2) 继承GenericServet类实现,需要重写service方法
- servlet中的service()方法重写与不重写
- HttpServlet的两个Service()方法区别
- servlet中service() doGet() doPost() 方法
- servlet中使用service()方法出现错误:HTTP method POST is not supported by this URL
- 通过源代码分析Servlet的service方法和doXXX方法
- Servlet中通用的service方法
- 通过源代码分析Servlet的service方法和doXXX方法
- 【转载】servlet中service() doGet() doPost() 方法
- springmvc下servlet怎么获取service及调用service的方法(备忘)
- servlet中service() doGet() doPost() 方法
- servlet中init,service方法
- 封装Servlet,跳过service,doPost,doGet直接写方法
- servlet中service方法源码分析
- HTTP Servlet 的service() 方法