java静态代理和动态代理(jdk,cglib)
2017-09-20 16:38
309 查看
一.项目结构:
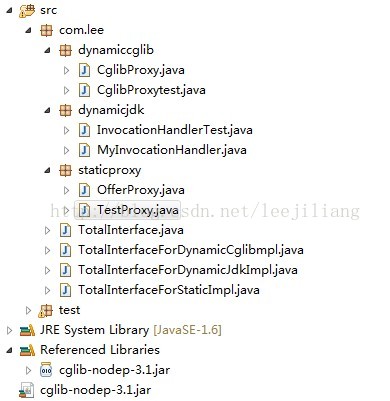
package com.lee;
public interface TotalInterface {
public void method1();
public void method2();
}
二.基础接口(实现类).
1.接口
package com.lee;
public interface TotalInterface {
public void method1();
public void method2();
}2.静态代理实现类:
package com.lee;
public class TotalInterfaceForStaticImpl implements TotalInterface {
@Override
public void method1() {
System.out.println("TotalInterfaceForStaticImpl method1");
}
@Override
public void method2() {
System.out.println("TotalInterfaceForStaticImpl method2");
}
}
3.动态代理实现类(基于JDK)
package com.lee;
public class TotalInterfaceForDynamicJdkImpl implements TotalInterface {
@Override
public void method1() {
System.out.println("TotalInterfaceForDynamicJdkImpl method1");
}
@Override
public void method2() {
System.out.println("TotalInterfaceForDynamicJdkImpl method2");
}
}
4.动态代理实现类(基于cglib),可以不实现任何接口.
package com.lee;
public class TotalInterfaceForDynamicCglibmpl {
public void method1() {
System.out.println("TotalInterfaceForDynamicCglibmpl method1");
}
public void method2() {
System.out.println("TotalInterfaceForDynamicCglibmpl method2");
}
}
三.创建代理类
1.静态代理类:
package com.lee.staticproxy;
import com.lee.TotalInterface;
public class OfferProxy implements TotalInterface {
private TotalInterface delegate;
public OfferProxy(TotalInterface delegate) {
this.delegate = delegate;
}
@Override
public void method1() {
System.out.println("pre");
delegate.method1();
System.out.println("end");
}
@Override
public void method2() {
System.out.println("pre");
delegate.method2();
System.out.println("end");
}
public TotalInterface getDelegate() {
return delegate;
}
public void setDelegate(TotalInterface delegate) {
this.delegate = delegate;
}
}
2.静态代理测试类:
package com.lee.staticproxy;
import com.lee.TotalInterface;
import com.lee.TotalInterfaceForStaticImpl;
public class TestProxy {
public static void main(String[] args) {
TotalInterface offer=new OfferProxy(new TotalInterfaceForStaticImpl());
offer.method1();
offer.method2();
}
}
执行结果:
pre
TotalInterfaceForStaticImpl method1
end
pre
TotalInterfaceForStaticImpl method2
end
3.基于JDK的动态代理
package com.lee.dynamicjdk;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
public class MyInvocationHandler implements InvocationHandler {
private Object target;
public MyInvocationHandler() {
super();
}
public MyInvocationHandler(Object target) {
super();
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args)
throws Throwable {
System.out.println("before "+method.getName());
Object result = method.invoke(target, args);
System.out.println("after "+method.getName());
return result;
}
}
4.测试类
package com.lee.dynamicjdk;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
import com.lee.TotalInterface;
import com.lee.TotalInterfaceForDynamicJdkImpl;
public class InvocationHandlerTest {
public static void main(String[] args) {
TotalInterface ioffer =new TotalInterfaceForDynamicJdkImpl();//被代理类.委托类.
InvocationHandler invocationHandler=new MyInvocationHandler(ioffer);
TotalInterface iofferProxy=(TotalInterface)Proxy.newProxyInstance(ioffer.getClass().getClassLoader(), ioffer.getClass().getInterfaces(), invocationHandler);//代理
iofferProxy.method1();
iofferProxy.method2();
}
}执行结果
before method1
TotalInterfaceForDynamicJdkImpl method1
after method1
before method2
TotalInterfaceForDynamicJdkImpl method2
after method2
5.cglib 代理类
package com.lee.dynamiccglib;
import java.lang.reflect.Method;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
public class CglibProxy implements MethodInterceptor {
@Override
public Object intercept(Object o, Method method, Object[] args,MethodProxy methodProxy) throws Throwable {
System.out.println("before"+methodProxy.getSuperName());
System.out.println(method.getName());
Object result=methodProxy.invokeSuper(o, args);
System.out.println("after"+methodProxy.getSuperName());
return result;
}
}
6.cglib测试类
package com.lee.dynamiccglib;
import com.lee.TotalInterfaceForDynamicCglibmpl;
import net.sf.cglib.proxy.Enhancer;
public class CglibProxytest {
public static void main(String[] args) {
CglibProxy cglibProxy=new CglibProxy();
Enhancer en=new Enhancer();
en.setSuperclass(TotalInterfaceForDynamicCglibmpl.class);
en.setCallback(cglibProxy);
TotalInterfaceForDynamicCglibmpl ioffer=(TotalInterfaceForDynamicCglibmpl)en.create();
ioffer.method1();
ioffer.method2();
}
}
执行结果
beforeCGLIB$method1$0
method1
TotalInterfaceForDynamicCglibmpl method1
afterCGLIB$method1$0
beforeCGLIB$method2$1
method2
TotalInterfaceForDynamicCglibmpl method2
afterCGLIB$method2$1
后续详解.
package com.lee;
public interface TotalInterface {
public void method1();
public void method2();
}
二.基础接口(实现类).
1.接口
package com.lee;
public interface TotalInterface {
public void method1();
public void method2();
}2.静态代理实现类:
package com.lee;
public class TotalInterfaceForStaticImpl implements TotalInterface {
@Override
public void method1() {
System.out.println("TotalInterfaceForStaticImpl method1");
}
@Override
public void method2() {
System.out.println("TotalInterfaceForStaticImpl method2");
}
}
3.动态代理实现类(基于JDK)
package com.lee;
public class TotalInterfaceForDynamicJdkImpl implements TotalInterface {
@Override
public void method1() {
System.out.println("TotalInterfaceForDynamicJdkImpl method1");
}
@Override
public void method2() {
System.out.println("TotalInterfaceForDynamicJdkImpl method2");
}
}
4.动态代理实现类(基于cglib),可以不实现任何接口.
package com.lee;
public class TotalInterfaceForDynamicCglibmpl {
public void method1() {
System.out.println("TotalInterfaceForDynamicCglibmpl method1");
}
public void method2() {
System.out.println("TotalInterfaceForDynamicCglibmpl method2");
}
}
三.创建代理类
1.静态代理类:
package com.lee.staticproxy;
import com.lee.TotalInterface;
public class OfferProxy implements TotalInterface {
private TotalInterface delegate;
public OfferProxy(TotalInterface delegate) {
this.delegate = delegate;
}
@Override
public void method1() {
System.out.println("pre");
delegate.method1();
System.out.println("end");
}
@Override
public void method2() {
System.out.println("pre");
delegate.method2();
System.out.println("end");
}
public TotalInterface getDelegate() {
return delegate;
}
public void setDelegate(TotalInterface delegate) {
this.delegate = delegate;
}
}
2.静态代理测试类:
package com.lee.staticproxy;
import com.lee.TotalInterface;
import com.lee.TotalInterfaceForStaticImpl;
public class TestProxy {
public static void main(String[] args) {
TotalInterface offer=new OfferProxy(new TotalInterfaceForStaticImpl());
offer.method1();
offer.method2();
}
}
执行结果:
pre
TotalInterfaceForStaticImpl method1
end
pre
TotalInterfaceForStaticImpl method2
end
3.基于JDK的动态代理
package com.lee.dynamicjdk;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
public class MyInvocationHandler implements InvocationHandler {
private Object target;
public MyInvocationHandler() {
super();
}
public MyInvocationHandler(Object target) {
super();
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args)
throws Throwable {
System.out.println("before "+method.getName());
Object result = method.invoke(target, args);
System.out.println("after "+method.getName());
return result;
}
}
4.测试类
package com.lee.dynamicjdk;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
import com.lee.TotalInterface;
import com.lee.TotalInterfaceForDynamicJdkImpl;
public class InvocationHandlerTest {
public static void main(String[] args) {
TotalInterface ioffer =new TotalInterfaceForDynamicJdkImpl();//被代理类.委托类.
InvocationHandler invocationHandler=new MyInvocationHandler(ioffer);
TotalInterface iofferProxy=(TotalInterface)Proxy.newProxyInstance(ioffer.getClass().getClassLoader(), ioffer.getClass().getInterfaces(), invocationHandler);//代理
iofferProxy.method1();
iofferProxy.method2();
}
}执行结果
before method1
TotalInterfaceForDynamicJdkImpl method1
after method1
before method2
TotalInterfaceForDynamicJdkImpl method2
after method2
5.cglib 代理类
package com.lee.dynamiccglib;
import java.lang.reflect.Method;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
public class CglibProxy implements MethodInterceptor {
@Override
public Object intercept(Object o, Method method, Object[] args,MethodProxy methodProxy) throws Throwable {
System.out.println("before"+methodProxy.getSuperName());
System.out.println(method.getName());
Object result=methodProxy.invokeSuper(o, args);
System.out.println("after"+methodProxy.getSuperName());
return result;
}
}
6.cglib测试类
package com.lee.dynamiccglib;
import com.lee.TotalInterfaceForDynamicCglibmpl;
import net.sf.cglib.proxy.Enhancer;
public class CglibProxytest {
public static void main(String[] args) {
CglibProxy cglibProxy=new CglibProxy();
Enhancer en=new Enhancer();
en.setSuperclass(TotalInterfaceForDynamicCglibmpl.class);
en.setCallback(cglibProxy);
TotalInterfaceForDynamicCglibmpl ioffer=(TotalInterfaceForDynamicCglibmpl)en.create();
ioffer.method1();
ioffer.method2();
}
}
执行结果
beforeCGLIB$method1$0
method1
TotalInterfaceForDynamicCglibmpl method1
afterCGLIB$method1$0
beforeCGLIB$method2$1
method2
TotalInterfaceForDynamicCglibmpl method2
afterCGLIB$method2$1
后续详解.
相关文章推荐
- java代理(静态代理和jdk动态代理以及cglib代理)
- java代理(静态代理、动态代理)(JDK和cglib)
- Java之代理(jdk静态代理,jdk动态代理,cglib动态代理,aop,aspectj)
- JAVA的代理模式(静态代理、JDK动态代理、cglib动态代理)
- 网摘-java静态代理和动态代理例子(jdk动态代理和cglib动态代理)
- Java静态代理和jdk动态代理、Cglib动态代理
- Java静态代理和jdk动态代理、Cglib动态代理
- Java之代理(jdk静态代理,jdk动态代理,cglib动态代理,aop,aspectj)
- 浅谈Java代理(jdk静态代理、动态代理和cglib动态代理)
- java 静态代理,jdk动态代理,CGLIB动态代理详解
- Java JDK中的静态代理、动态代理&Cglib动态代理
- java代理(静态代理和jdk动态代理以及cglib代理)
- Java之代理(jdk静态代理,jdk动态代理,cglib动态代理,aop,aspectj)
- Java之代理(jdk静态代理,jdk动态代理,cglib动态代理,aop,aspectj)
- java静态代理和动态代理(JDK&cglib)
- java代理(静态代理和jdk动态代理以及cglib代理)
- java静态代理,动态代理(JDK,CGLib)
- Java之代理(jdk静态代理,jdk动态代理,cglib动态代理,aop,aspectj)
- Java之代理(jdk静态代理,jdk动态代理,cglib动态代理,aop,aspectj)
- [教程]Java代理(静态,动态jdk和Cglib)简单应用