ATM:模拟实现一个ATM + 购物商城程序
2017-09-10 23:30
495 查看
额度 15000或自定义
实现购物商城,买东西加入 购物车,调用信用卡接口结账
可以提现,手续费5%
支持多账户登录
支持账户间转账
记录每月日常消费流水
提供还款接口
ATM记录操作日志
提供管理接口,包括添加账户、用户额度,冻结账户等。。。
用户认证用装饰器
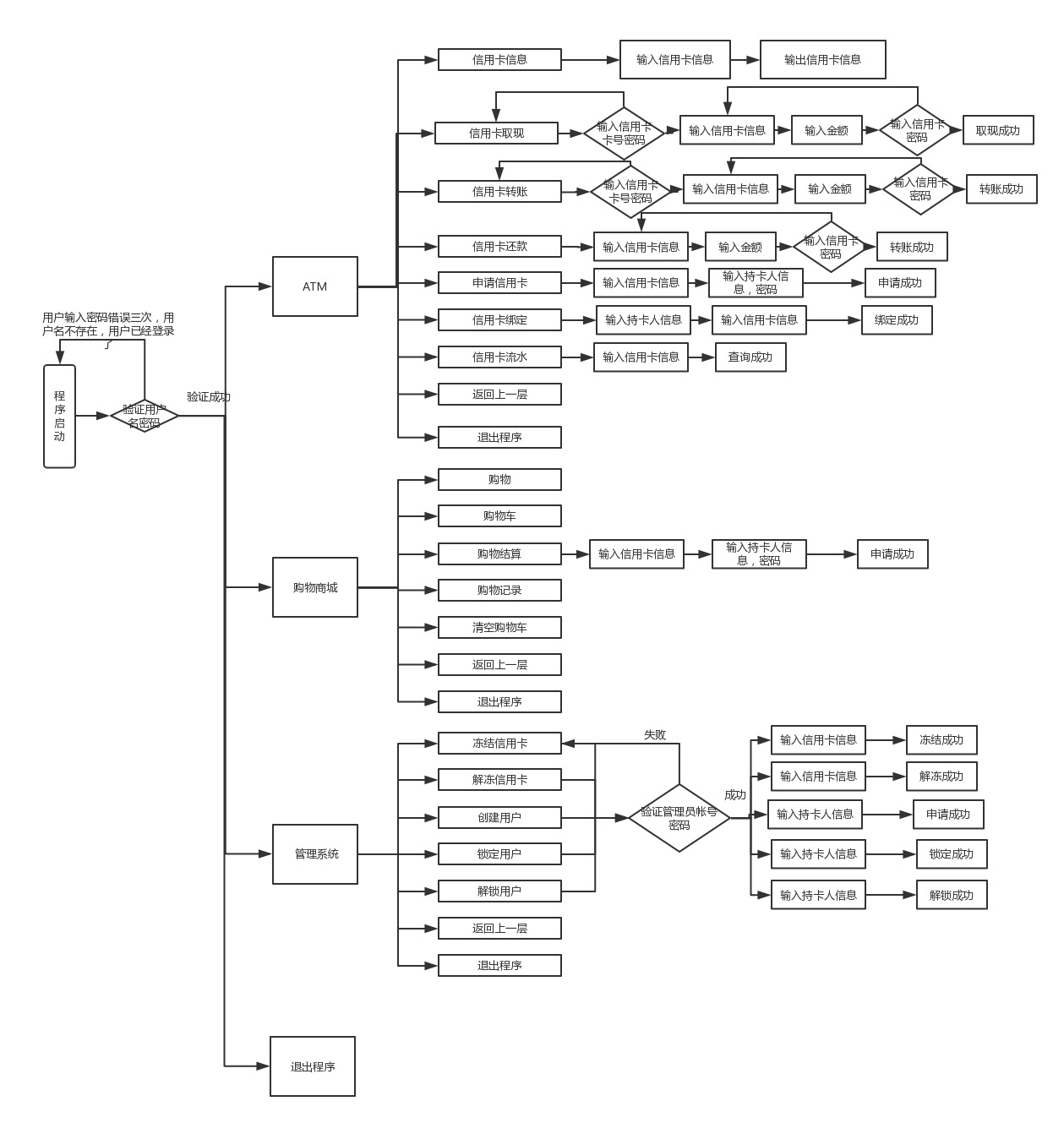
1、开始先运行atm_start.py时执行程序
2、直接到main下,所有用户的密码123,才能进行下一步的操作,管理员默认密码为123,所有信用卡的默认密码为123。所有的密码都为123
3、然后列出ATM、购物商城、管理系统
4、atm的功能列表
1 信用卡信息
2 信用卡取现
3 信用卡转账
4 信用卡还款
5 申请信用卡
6 信用卡绑定
7 信用卡流水
5、购物商城:
1 购物
2 购物车
3 购物结算
4 购物记录
5 清空购物车
6、管理系统:管理系统的默认管理员帐号为admin,密码为123
1 冻结信用卡
2 解冻信用卡
3 创建用户
4 锁定用户
5 解锁用户
atm_start.py
auth.py
creditcard.py
log.py
main.py
shopping.py
user.py
Blacklist_user
creditcard_data
creditcard_record
shopping_car
shopping_list
user_data
实现购物商城,买东西加入 购物车,调用信用卡接口结账
可以提现,手续费5%
支持多账户登录
支持账户间转账
记录每月日常消费流水
提供还款接口
ATM记录操作日志
提供管理接口,包括添加账户、用户额度,冻结账户等。。。
用户认证用装饰器
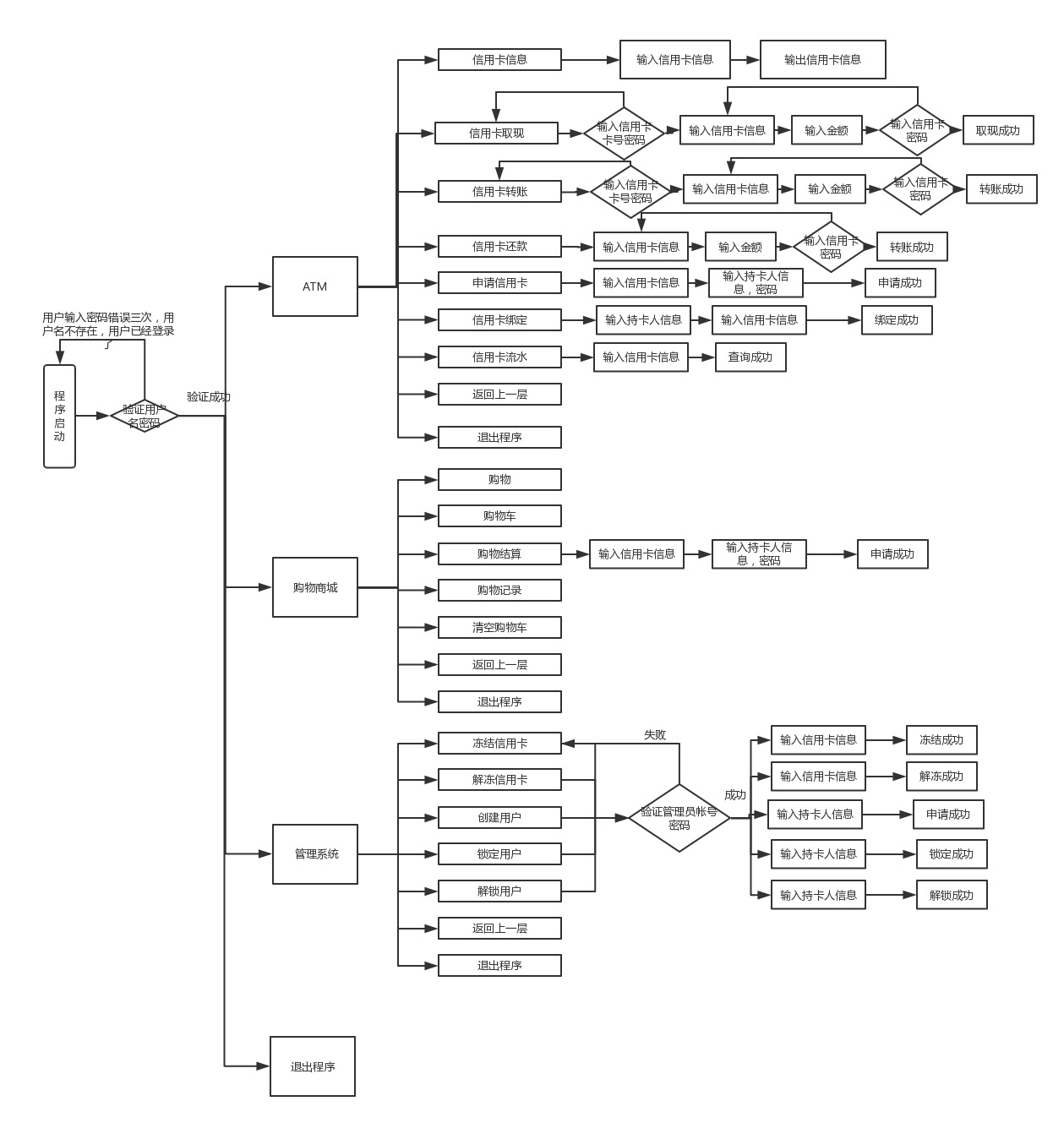
atm作业 ├── atm #ATM主程目录 │ ├── bin #ATM 执行文件 目录 │ │ ├── __init__.py │ │ ├── atm_start.py #ATM 主程序 执行程序 │ │ │ ├── conf #配置文件 │ │ ├── __init__.py │ │ └── settings.py │ ├── core #主要程序逻辑都 在这个目录 里 │ │ ├── __init__.py │ │ ├── auth.py #用户,信用卡,管理员认证模块 │ │ ├── log.py #日志记录模块 │ │ ├── creditcard.py #信用卡模块\转账\还款\取现等 │ │ ├── shopping.py #购物模块\商城\购物车\购物结算等 │ │ ├── main.py #主逻辑交互程序 │ │ └── user.py # 用户模块\创建\锁定\解锁等 │ ├── db #数据库 │ │ ├── __init__.py │ │ ├── Blacklist_user # 用户黑名单文件 │ │ └── user_data #用户文件,用户的各种信息 │ │ └── creditcard_data #信用卡文件,信用卡的各种信息 │ │ └── shopping_car #购物车文件 │ │ └── shopping_list #商品列表文件 │ └── log #日志目录 │ ├── __init__.py └── └── water_record #所有的用户,信用卡交易日志
1、开始先运行atm_start.py时执行程序
2、直接到main下,所有用户的密码123,才能进行下一步的操作,管理员默认密码为123,所有信用卡的默认密码为123。所有的密码都为123
3、然后列出ATM、购物商城、管理系统
4、atm的功能列表
1 信用卡信息
2 信用卡取现
3 信用卡转账
4 信用卡还款
5 申请信用卡
6 信用卡绑定
7 信用卡流水
5、购物商城:
1 购物
2 购物车
3 购物结算
4 购物记录
5 清空购物车
6、管理系统:管理系统的默认管理员帐号为admin,密码为123
1 冻结信用卡
2 解冻信用卡
3 创建用户
4 锁定用户
5 解锁用户
atm_start.py
# !/usr/bin/env python # -*- coding:utf-8 -*- # Author:lzd #ATM程序的执行文件 import os import sys dir = os.path.dirname(os.path.dirname(os.path.abspath(__file__))) #找到路径 sys.path.insert(0,dir+r'\core') # 添加路径 # print(dir) # print(sys.path) #将main.py里面的所有代码封装成main变量 from main import * if __name__ == '__main__': main()
auth.py
# !/usr/bin/env python # -*- coding:utf-8 -*- # Author:lzd #用户认证,信用卡认证,管理员认证模块 import os import json BASE_DIR = os.path.dirname(os.path.dirname(__file__)) user_dic = BASE_DIR + r"/db/user_data" creditcard_dic = BASE_DIR + r"/db/creditcard_data" '''认证装饰器''' def auth(auth_type): def out_wrapper(func): if auth_type == "user_auth": #用户认证 def wrapper(): res = func user_name = input("请输入登录用户名 :").strip() user_pwd = input("请输入登录密码 :").strip() if len(user_name)>0: with open(user_dic,"r") as f: user_data = json.loads(f.read()) if user_name in user_data.keys() and user_pwd == user_data[user_name]["password"]: if user_data[user_name]["locked"] == False: print("[ %s ] 用户认证成功"%(user_name)) return res,user_name else: print("[ %s ] 用户已经被锁定,认证失败"%(user_name)) else: print("[ %s ] 用户或密码错误,认证失败"%(user_name)) else: print("[ %s ] 用户输入不能为空"%(user_name)) return wrapper if auth_type == "creditcard_auth": #信用卡认证 def wrapper(): res = func() with open(creditcard_dic, "r") as f: creditcard_data = json.loads(f.read()) print("当前所有的信用卡卡号%s"%creditcard_data.keys()) creditcard = input("请输入信用卡卡号(6位数字):").strip() password = input("请输入信用卡密码 : ").strip() if creditcard: with open(creditcard_dic,"r") as f: creditcard_data = json.loads(f.read()) if creditcard in creditcard_data.keys() and password == creditcard_data[creditcard]["password"]: if creditcard_data[creditcard]["locked"] == False: print("信用卡 [ %s ] 验证成功"%(creditcard)) return res,creditcard else: print("信用卡 [ %s ]已经被冻结,请使用其他信用卡"%(creditcard)) else: print("信用卡卡账号或密码输入错误") else: print("信用卡账号输入不能为空") return wrapper if auth_type == "admin_auth": #管理员认证 def wrapper(): res = func() admin_dic = {"admin":"admin","passwrod":"123"} while True: admin_name = input("请输入管理员账号 :").strip() admin_pwd = input("请输入密码 :").strip() if admin_name: if admin_name in admin_dic and admin_pwd == admin_dic["passwrod"]: print("管理员账号[%s] 登陆成功。"%(admin_name)) return res,admin_name else: print("账号或密码错误") continue else: print("管理员账号输入不能为空") continue return wrapper return out_wrapper @auth(auth_type="user_auth") def user_auth(): print("用户登录认证".center(40,"-")) return "True" @auth(auth_type="creditcard_auth") def creditcard_auth(): print("信用卡登录认证".center(40,"-")) return "True" @auth(auth_type="admin_auth") def admin_auth(): print("管理员登录认证".center(40,"-")) return "True"
creditcard.py
# !/usr/bin/env python # -*- coding:utf-8 -*- # Author:lzd '''信用卡信息,''' from log import get_logger import json,os BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__))) '''数据库文件相对路径''' user_dic = BASE_DIR + r"/db/user_data" creditcard_dic = BASE_DIR + r"/db/creditcard_data" creditcard_record = BASE_DIR + r"/db/creditcard_record" user_Water = BASE_DIR + r"/log/water_record" logger = get_logger() #日志实例化对象 '''信用卡信息''' def creditcard_data(): while True: with open(creditcard_dic,"r") as f: creditcard_data = json.loads(f.read()) print("当前所有的信用卡%s"%creditcard_data.keys()) choice = input("请输入要查看信息的信用卡账号 '6位数字' :").strip() if choice in creditcard_data.keys(): print("我的信用卡信息".center(50,"-")) print("持卡人:\t[ %s ]\n卡号:\t[ %s ]\n额度:\t[ ¥%s ]\n可用额度:\t[ ¥%s ]\n取现额度:\t[ ¥%s ]" %(creditcard_data[choice]["personinfo"], choice, creditcard_data[choice]["deflimit"], creditcard_data[choice]["limit"], creditcard_data[choice]["limitcash"])) else: print("您输入的信用卡,不存在。") choice = input("返回输入’q':") if choice == "q": break '''查看信用卡流水''' def cat_cred_record(): while True: choice = input("请输入要查看流水记录的信用卡账号:").strip() with open(creditcard_dic) as f1: cred_record = json.loads(f1.read()) if choice: if choice in cred_record.keys(): print("信用卡 [ %s ] 流水记录".center(50, "-") % (choice)) with open(user_Water) as f: for i in f: if choice in i: #print("\33[31;0m信用卡 [ %s ] 还没有进行过消费,去商城买点东西吧\33[0m\n" % (choice)) print(i.strip()) else: print("您输入的信用卡 [ %s ] 不存在"%(choice)) else: print("您输入的信用卡 [ %s ] 不存在"%(choice)) choice = input("返回 'q':") if choice == "q": break '''信用卡还款''' def repayment(): while True: print(" 还款 ".center(40, "-")) with open(creditcard_dic, "r+") as f: creditcard_data = json.loads(f.read()) choice = input("请输入还款的信用卡账号, 返回'q' :").strip() if choice == 'q': break if choice in creditcard_data: money = input("请输入还款金额:").strip() pwd = input("请输入还款密码 :").strip() if pwd == creditcard_data[choice]["password"]: if money.isdigit(): money = int(money) with open(creditcard_dic, "r+") as f: creditcard_data = json.loads(f.read()) limit = creditcard_data[choice]["limit"] limitcash = creditcard_data[choice]["limitcash"] limit += money limitcash += (money//2) creditcard_data[choice]["limit"]=limit creditcard_data[choice]["limitcash"] = limitcash dic = json.dumps(creditcard_data) rapayment_data = [str(choice), "信用卡还款", str(money)] msg = "---".join(rapayment_data) f.seek(0) f.truncate(0) f.write(dic) f.flush() logger.debug(msg) #日志模块 print("信用卡 [ %s ] 还款金额 [ ¥%s ] 还款成功" % (choice, money)) print("信用卡\t[ %s ]\n可用额度:\t[ ¥%s ]\n取现额度:\t[ ¥%s ] " %(choice, creditcard_data[choice]["limit"], creditcard_data[choice]["limitcash"])) else: print("输入金额格式有误") else: print("密码输入错误") else: print("您输入的信用卡不存在") '''信用卡取现''' def takenow(): while True: print(" 取现 ".center(40, "-")) with open(creditcard_dic, "r+") as f: creditcard_data = json.loads(f.read()) choice = input("请输入取现的信用卡账号, 返回'q' :").strip() if choice == 'q': break if choice in creditcard_data: #print(creditcard_data) limit = creditcard_data[choice]["limit"] limitcash = creditcard_data[choice]["limitcash"] takenow = limit // 2 print("信用卡卡号:\t[ %s ]\n信用卡额度:\t[ ¥ %s ]\n取现额度:\t[ ¥ %s ]" % (choice,limit,takenow)) if limit >= limitcash: print("可取现金额为:\t [ ¥%s ]\n" % (limitcash)) cash = input("请输入要取现的金额,收取%5手续费 :").strip() if cash.isdigit(): cash = int(cash) if cash <= limitcash: if cash > 0 : password = input("请输入信用卡[ %s ] 的密码 :" % (choice)).strip() if password and password == creditcard_data[choice]["password"]: limitcash = int(limitcash - (cash * 0.05 + cash)) limit = int(limit - (cash * 0.05 + cash)) creditcard_data[choice]["limit"] = limit creditcard_data[choice]["limitcash"] = limitcash f.seek(0) f.truncate(0) dic = json.dumps(creditcard_data) f.write(dic) takenow_data = [str(choice),"信用卡取现",str(cash),"手续费",str(int(cash*0.05))] msg = "---".join(takenow_data) logger.debug(msg) print("取现成功".center(40,"-")) print("取现金额:\t[%s]\n手续费:\t[%s]" % (cash, cash * 0.05)) else: print("密码输入错误\n") else: print("金额不能为0") else: print("您的取现金额已经超出取现额度了。") else: print("您的信用额度已经小于取现额度,不能取现了") else: print("您输入的信用卡账号 [ %s ] 错误"%(choice)) '''信用卡转账''' def transfer(): while True: print(" 转账 ".center(40, "-")) with open(creditcard_dic, "r+") as f: creditcard_data = json.loads(f.read()) choice = input("请输入信用卡账号, 返回'q' :").strip() if choice == 'q': break if choice in creditcard_data: current_limit = creditcard_data[choice]["limit"] transfer_account = input("请输入转账账号:").strip() if transfer_account.isdigit(): #print("----------") if len(transfer_account) == 6 : if transfer_account in creditcard_data.keys(): money = input("请输入转账金额:").strip() if money.isdigit(): money = int(money) creditcard_pwd = input("请输入信用卡账号密码:") if creditcard_pwd == creditcard_data[choice]["password"]: if money <= current_limit: creditcard_data[choice]["limit"] -= money creditcard_data[choice]["limitcash"] -= money//2 creditcard_data[transfer_account]["limit"] += money creditcard_data[transfer_account]["limitcash"] += money//2 print("转账成功".center(40,"-")) print("转账卡号:\t[ %s ]\n转账金额:\t[ ¥%s ]"%(transfer_account,money)) print("信用卡:\t[ %s ]\t可用额度还剩:\t[ ¥%s ]\n"%(choice, creditcard_data[choice]["limit"])) transfer_data = [str(choice), "信用卡转账", str(money)] msg = "---".join(transfer_data) logger.debug(msg) f.seek(0) f.truncate(0) dic = json.dumps(creditcard_data) f.write(dic) else: print("转账金额不能超过信用额度") else: print("密码输入错误") else: print("请输入数字的金额") else: print("您输入的卡号不存在") else: print("您输入的卡号不存在") else: print("请输入正确的卡号") else: print("您输入的信用卡不存在") '''冻结信用卡''' def lock_creditcard(): while True: print("冻结信用卡".center(50, "-")) with open(creditcard_dic, "r+") as f: creditcard_data = json.loads(f.read()) for key in creditcard_data: if creditcard_data[key]["locked"] == False: print("信用卡 [ %s ]\t\t冻结状态:[未冻结]" % (key)) else: print("信用卡 [ %s ]\t\t冻结状态:[已冻结]" % (key)) choice = input("是否进行信用卡冻结 : 确定 'y' 返回 'q' :").strip() if choice == "q":break if choice == "y": lock_creditcard = input("请输入要冻结的信用卡卡号:").strip() if lock_creditcard in creditcard_data.keys(): if creditcard_data[lock_creditcard]["locked"] == False: creditcard_data[lock_creditcard]["locked"] = True dic = json.dumps(creditcard_data) f.seek(0) f.truncate(0) f.write(dic) print("信用卡 %s 冻结成功\n" % (lock_creditcard)) else: print("信用卡 %s 已经被冻结\n" % (lock_creditcard)) else: print("信用卡 %s 不存在\n" %(lock_creditcard)) '''解除冻结信用卡''' def unlock_creditcard(): while True: print("解冻结信用卡".center(50, "-")) with open(creditcard_dic, "r+") as f: creditcard_data = json.loads(f.read()) for key in creditcard_data: if creditcard_data[key]["locked"] == False: print("信用卡 [ %s ]\t\t冻结状态:[未冻结]" % (key)) else: print("信用卡 [ %s ]\t\t冻结状态:[已冻结]" % (key)) choice = input("是否进行解除信用卡冻结 : 确定 'y' 返回 'q' :").strip() if choice == "q":break if choice == "y": lock_creditcard = input("请输入要冻结的信用卡卡号:").strip() if lock_creditcard in creditcard_data.keys(): if creditcard_data[lock_creditcard]["locked"] == True: creditcard_data[lock_creditcard]["locked"] = False dic = json.dumps(creditcard_data) f.seek(0) f.truncate(0) f.write(dic) print("信用卡 %s 解除冻结成功\n" % (lock_creditcard)) else: print("信用卡 %s 已经解除冻结\n" % (lock_creditcard)) else: print("信用卡 %s 不存在\n" %(lock_creditcard)) '''申请信用卡''' def new_creditcard(limit=15000,locked=False): while True: print("申请信用卡".center(50, "-")) with open(creditcard_dic, "r+") as f: creditcard_data = json.loads(f.read()) for key in creditcard_data: print("系统已有信用卡 【%s】 \t持卡人 【%s】" % (key,creditcard_data[key]["personinfo"])) choice = input("\n\33[34;0m是否申请新的信用卡 确定'y' 返回'q'\33[0m:").strip() if choice == "q":break if choice == "y": creditcard = input("\33[34;0m输入要申请的信用卡卡号(6位数字):\33[0m").strip() if creditcard not in creditcard_data.keys(): if creditcard.isdigit() and len(creditcard) == 6: password = input("\33[34;0m请输入申请的信用卡密码:\33[0m").strip() if len(password) > 0: personinfo = input("\33[34;0m请输入信用卡申请人:\33[0m").strip() if len(personinfo) > 0: creditcard_data[creditcard] = {"creditcard":creditcard, "password":password, "personinfo":personinfo, "limit":limit,"limitcash":limit//2,"locked":locked,"deflimit":limit,} dict = json.dumps(creditcard_data) f.seek(0) f.truncate(0) f.write(dict) print("信用卡:\t[ %s ] 申请成功\n持卡人:\t[ %s ]\n额度:\t[ ¥%s ]\n取现额度:\t[ ¥%s ]"%(creditcard, personinfo, limit, creditcard_data[creditcard]["limitcash"])) else: print("信用卡申请人不能为空\n") else: print("输入的密码不正确\n") else: print("信用卡 %s 卡号不符合规范\n" % (creditcard)) else: print("信用卡 %s 已经存在\n" % (creditcard)) '''信用卡绑定''' def link_creditcard(): while True: print("\33[32;0m修改信用卡绑定\33[0m".center(40, "-")) with open(user_dic, "r+") as f: user_data = json.loads(f.read()) user_name = input("请输入绑定信用卡的用户名 返回 'q' :").strip() if user_name == "q":break if user_name in user_data.keys(): creditcard = user_data[user_name]["creditcard"] if creditcard == 0 : print("当前账号: \t%s"%(user_name)) print("信用卡绑定:\33[31;0m未绑定\33[0m\n") else: print("当前账号: \t%s" %(user_name)) print("绑定的信用卡: %s\n"%(creditcard)) choice = input("\33[34;0m是否要修改信用卡绑定 确定 'y' 返回'q' \33[0m:") if choice == "q":break if choice == "y": creditcard_new = input("\33[34;0m输入新的信用卡卡号(6位数字)\33[0m:").strip() if creditcard_new.isdigit() and len(creditcard_new) ==6: with open(creditcard_dic, "r+") as f1: creditcard_data = json.loads(f1.read()) if creditcard_new in creditcard_data.keys(): user_data[user_name]["creditcard"]=creditcard_new dict = json.dumps(user_data) f.seek(0) f.truncate(0) f.write(dict) print("\33[31;1m信用卡绑定成功\33[0m\n") else: print("\33[31;0m输入信用卡卡号不存在(未发行)\33[0m\n") else: print("\33[31;0m输入信用卡格式错误\33[0m\n") else: print("请选择 ’y‘ 或 ’q‘ ") else: print("您输入的用户 [ %s ] 不存在 ")
log.py
# !/usr/bin/env python # -*- coding:utf-8 -*- # Author:lzd # 日志记录模块 import logging,os BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__))) user_Water = BASE_DIR + r"/log/water_record" '''日志模块''' def get_logger(): #有循环的时候 要放到循环外面 fh = logging.FileHandler(user_Water) # 创建一个文件流,需要给一个文件参数 logger = logging.getLogger() # 获得一个logger对象 #sh = logging.StreamHandler() # 创建一个屏幕流, logger.setLevel(logging.DEBUG) # 设定最低等级debug # 写入文件的中的格式 fm = logging.Formatter("%(asctime)s --- %(message)s") logger.addHandler(fh) # 把文件流添加进来,流向文件 #logger.addHandler(sh) # 把屏幕流添加进来,流向屏幕 fh.setFormatter(fm) # 在文件流添加写入格式 #sh.setFormatter(fm) # 在屏幕流添加写入格式 return logger
main.py
# !/usr/bin/env python # -*- coding:utf-8 -*- # Author:lzd '''主逻辑交互程序''' from auth import * from user import * from creditcard import * from shopping import * '''主页面列表''' def main_list(): msg = [" ATM ", "购物商城", "管理系统", "退出程序 输入 q", ] index = 0 for i in msg: print("\t\t\t\t", index + 1, "\t ", i) index += 1 '''ATM页面列表''' def atm_list(): msg = ["信用卡信息", "信用卡取现", "信用卡转账", "信用卡还款", "申请信用卡", "信用卡绑定", "信用卡流水", "返回上一层 输入 q", "退出程序 输入 exit"] index = 0 for i in msg: print("\t\t\t\t", index + 1, "\t ", i) index += 1 '''购物车页面列表''' def shopp_list(): msg = ["购物", "购物车", "购物结算", "购物记录", "清空购物车", "返回上一层 输入 q", "退出程序 输入 exit"] index = 0 for i in msg: print("\t\t\t\t", index + 1, "\t ", i) index += 1 '''管理员页面列表''' def admin_list(): msg = ["冻结信用卡", "解冻信用卡", "创建用户", "锁定用户", "解锁用户", "返回上一层 输入 q", "退出程序 输入 exit"] index = 0 for i in msg: print("\t\t\t\t", index + 1, "\t ", i) index += 1 '''主函数''' def main(): print("购物商城ATM系统".center(40, "-")) lock() # 三次锁定模块 while True: print("欢迎来到购物商城ATM系统".center(40, "-")) print(" \t\t\t\t ID\t\t信息") main_list() choice = input("请选择 ID :").strip() if choice == "q": print(" bye bye ".center(50, "-")) exit() if choice.isdigit(): choice = int(choice) if choice >= 1 and choice <= 4: if choice == "q": break while True: if choice == 1: print("欢迎来到信用卡中心".center(50, "-")) print(" \t\t\t\t ID\t\tATM信息") atm_list() # 信用卡列表 atm_choice = input("请选择 ATM ID :").strip() if atm_choice == "q": break if atm_choice == "exit": exit() if atm_choice.isdigit(): atm_choice = int(atm_choice) if atm_choice >= 1 and atm_choice <= 7: while True: if atm_choice == 1: creditcard_data() # 信用卡信息模块 break elif atm_choice == 2: creditcard_auth() # 信用卡认证模块 takenow() # 信用卡取现模块 break elif atm_choice == 3: creditcard_auth() # 信用卡认证模块 transfer() # 信用卡转账模块 break elif atm_choice == 4: creditcard_auth() # 信用卡认证模块 repayment() # 信用卡还款模块 break elif atm_choice == 5: new_creditcard(limit=15000, locked=False) # 申请信用卡模块 break elif atm_choice == 6: link_creditcard() # 用户绑定信用卡模块 break elif atm_choice == 7: cat_cred_record() # 查看信用卡流水模块 break else: print("请输入正确的 ID ") else: print("请输入正确的 ID ") elif choice == 2: print("欢迎来到购物中心".center(50, "-")) print(" \t\t\t\t ID\t\t商城信息") shopp_list() # 商城列表 shopp_choice = input("请选择 商城 ID :").strip() if shopp_choice == "q": break if shopp_choice == "exit": exit() if shopp_choice.isdigit(): shopp_choice = int(shopp_choice) if shopp_choice >= 1 and shopp_choice <= 5: while True: if shopp_choice == 1: shopping_mall() # 购物商城模块 break elif shopp_choice == 2: Shopping_car() # 购物车模块 break elif shopp_choice == 3: shopping_pay() # 购物结算模块 break elif shopp_choice == 4: cat_shopp_record() # 查看购物记录模块 break elif shopp_choice == 5: del_shopping_car() # 清空购物车模块 break else: print("请输入正确的 ID ") else: print("请输入正确的 ID ") elif choice == 3: print("欢迎来到管理中心".center(50, "-")) print(" \t\t\t\t ID\t\t操作信息") admin_list() # 管理中心列表 admin_choice = input("请选择 信息 ID :").strip() if admin_choice == "q": break if admin_choice == "exit": exit() if admin_choice.isdigit(): admin_choice = int(admin_choice) if admin_choice >= 1 and admin_choice <= 5: while True: if admin_choice == 1: admin_auth() # 管理员用户验证模块 lock_creditcard() # 冻结信用卡模块 break elif admin_choice == 2: admin_auth() # 管理员用户验证模块 unlock_creditcard() # 解冻信用卡模块 break elif admin_choice == 3: admin_auth() # 管理员用户验证模块 # 创建用户模块 new_user(address="None", locked=False, creditcard=False) break elif admin_choice == 4: admin_auth() # 管理员用户验证模块 lock_user() # 锁定用户模块 break elif admin_choice == 5: admin_auth() # 管理员用户验证模块 unlock_user() # 解锁用户模块 break elif choice == 4: exit()
shopping.py
# !/usr/bin/env python # -*- coding:utf-8 -*- # Author:lzd import json,os from creditcard import link_creditcard from auth import creditcard_auth from log import get_logger BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__))) '''数据库文件相对路径''' shopping_dic = BASE_DIR + r"/db/shopping_record" shopping_lis = BASE_DIR + r"/db/shopping_list" shopping_car = BASE_DIR + r"/db/shopping_car" creditcard_dic = BASE_DIR + r"/db/creditcard_data" user_dic = BASE_DIR + r"/db/user_data" user_Water = BASE_DIR + r"/log/water_record" logger = get_logger() #日志模块实例化对象 '''购物商城''' def shopping_mall(): shopping_list,pro_list = [],[] with open(shopping_lis, "r", encoding="utf-8") as f: for item in f: pro_list.append(item.strip("\n").split()) def pro_inf(): print("\t\t\t\t编号\t商品\t\t价格") for index, item in enumerate(pro_list): print("\t\t\t\t%s\t\t%s\t\t%s" % (index+1, item[0], item[1])) while True: print(("目前商城在售的商品信息").center(50, "-")) pro_inf() choice_id = input("选择要购买的商品编号 '购买 ID' 返回 'q':") if choice_id.isdigit(): choice_id = int(choice_id) if choice_id <= len(pro_list) and choice_id >=0: pro_item = pro_list[choice_id-1] print("商品 [ %s ] 加入购物车 价格 [ ¥%s ] "%(pro_item[0],pro_item[1])) shopping_list.append(pro_item) shopp_data = ["商品",str(pro_item[0]), "价格", str(pro_item[1])] msg = "---".join(shopp_data) logger.debug(msg) else: print("没有相应的编号 请重新输入:") elif choice_id == "q": with open(shopping_car, "r+") as f: list = json.loads(f.read()) list.extend(shopping_list) f.seek(0) f.truncate(0) list = json.dumps(list) f.write(list) break else: print("没有相应的编号 请重新输入:") '''购物车''' def Shopping_car(): while True: with open(shopping_car, "r+") as f: list = json.loads(f.read()) sum = 0 print("购物车信息清单".center(40,"-")) print("id\t商品\t价格") for index,item in enumerate(list): print("%s\t%s\t%s"%(index+1,item[0],item[1])) sum +=int(item[1]) print("商品总额共计: ¥%s"%(sum)) choice = input("请选择要进行的操作 返回 'q' 清空'f':").strip() if choice == "q" :break if choice == "f": del_shopping_car() break '''清空购物车''' def del_shopping_car(): while True: with open(shopping_car, "r+") as f: res = json.loads(f.read()) if res != []: choice = input("是否清空购物车 确定 'y' 返回 'q' :").strip() print("购物车里的商品".center(50, "-")) print(res, "\n") if choice == "q":break if choice == "y": list = json.dumps([]) f.seek(0) f.truncate(0) f.write(list) print("购物车已清空") else: print("请输入正确的指令: ‘y' 或 ’q' ") else: print("您还没有消费过,去商城花点钱把") break '''购物结算''' def shopping_pay(): while True: print("购物结算".center(50, "-"),"\n") with open(shopping_car, "r+") as f: data = json.loads(f.read()) if data != []: print("购物车信息清单".center(50, "-")) print("\t\t\t\t\tid\t商品\t价格") for index, item in enumerate(data): print("\t\t\t\t\t%s\t%s\t%s" % (index + 1, item[0], item[1])) money = sum([int(i[1]) for i in data]) else: print("您还没有消费过,去商城花点钱把") break choice = input("当前商品总额:[ ¥%s ] 是否进行支付 :确定 'y' 返回 'q':" % (money)) if choice == "q": break if choice == "y": creditcard_auth() # 信用卡认证模块 #break user_name = input("请输入结算的用户账号:").strip() with open(user_dic, "r+") as f1: user_data = json.loads(f1.read()) if user_name in user_data.keys(): user_creditcard = user_data[user_name]["creditcard"] if user_creditcard == False: print("账号 %s 未绑定信用卡,请先绑定信用卡" % (user_name)) link_creditcard() #信用卡绑定模块 else: with open(creditcard_dic, "r+") as f2: creditcard_data = json.loads(f2.read()) # print(creditcard_data) # print(user_creditcard) pwd = input("请输入 信用卡[ %s ]支付密码 :" % (creditcard_data[user_creditcard]["creditcard"])) if pwd == creditcard_data[user_creditcard]["password"]: limit = creditcard_data[user_creditcard]["limit"] limit_new = limit - money limit_not = creditcard_data[user_creditcard]["limitcash"] - money // 2 if limit_new >= 0: creditcard_data[user_creditcard]["limit"] = limit_new creditcard_data[user_creditcard]["limitcash"] = limit_not shop_data = [user_name,str(creditcard_data[user_creditcard]["creditcard"]), "信用卡结账", str(money)] msg = "---".join(shop_data) dict = json.dumps(creditcard_data) f2.seek(0) f2.truncate(0) f2.write(dict) logger.debug(msg) print("支付成功-->>>\t购物支付:[ ¥%s ]\t当前额度还剩 : [ ¥%s ]\n" % (money, limit_new)) break else: print("当前信用卡额度 %s元 不足矣支付购物款 可绑定其他信用卡支付\n" % (limit)) else: print("密码错误,请重新输入!!!") continue else: print("您输入的用户不存在") '''查看购物记录''' def cat_shopp_record(): while True: with open(user_dic) as f: user_data = json.loads(f.read()) choice = input("请输入要查看购物记录的用户名:").strip() if choice in user_data.keys(): print("用户 %s 购物记录".center(50, "-")%(choice)) with open(user_Water) as f1: for i in f1: if choice in i: print(i.strip()) #print("\33[31;0m用户 %s 还没有进行过消费\33[0m\n" % (choice)) choice1 = input("返回 'q':") if choice1 == "q": break else: print("您输入的用户名 [ %s ] 不存在"%(choice))
user.py
# !/usr/bin/env python # -*- coding:utf-8 -*- # Author:lzd import json,os BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__))) '''数据库文件相对路径''' user_dic = BASE_DIR + r"/db/user_data" user_Blacklist = BASE_DIR + r"/db/Blacklist_user" '''创建用户''' def new_user(address="None", locked=False, creditcard=False): while True: print("开始创建用户".center(50, "-")) with open(user_dic, "r+") as f: user_data = json.loads(f.read()) for key in user_data: print("系统已有用户 [ %s ]" % (key)) choice = input("是否创建新的用户 确定'y'/返回'q':") if choice == "q": break if choice == "y": user_name = input("请输入要创建的用户名:").strip() user_pwd = input("请输入创建用户的密码 :").strip() if user_name not in user_data.keys(): if len(user_name) > 0 and len(user_pwd) > 0: user_data[user_name] = {"username": user_name, "password": user_pwd, "creditcard": creditcard, "address": address,"status":False,"locked": locked} dic = json.dumps(user_data) with open(user_Blacklist, "r+") as f3: tmpdata = json.loads(f3.read()) tmpdata[user_name]="0" f3.seek(0) f3.truncate(0) f3.write(json.dumps(tmpdata)) f.seek(0) f.truncate(0) f.write(dic) print("用户 %s 创建成功\n" % (user_name)) else: print("输入的用户或密码不能密码为空") else: print("用户 %s 已经存在\n" % (user_name)) '''解锁用户''' def unlock_user(): while True: print("解锁用户".center(50, "-")) with open(user_dic, "r+") as f: user_data = json.loads(f.read()) for key in user_data: if user_data[key]["locked"] == False: print("用户 [ %s ]\t\t锁定状态:[未锁定]" % (key)) else: print("用户 [ %s ]\t\t锁定状态:[已锁定]" % (key)) choice = input("是否进行用户解锁 : 确定 'y' 返回 'q' :").strip() if choice == "q":break if choice == "y": unlock_user = input("请输入要解锁的用户名:").strip() if unlock_user in user_data.keys(): if user_data[unlock_user]["locked"] == True: user_data[unlock_user]["locked"] = False dict = json.dumps(user_data) f.seek(0) f.truncate(0) f.write(dict) print("\33[31;1m用户 %s 解锁成功\33[0m\n" % (unlock_user)) else: print("用户 %s 解锁失败 用户未被锁定" % (unlock_user)) else: print("用户 %s 不存在"%(unlock_user)) '''锁定用户''' def lock_user(): while True: print("锁定用户".center(50, "-")) with open(user_dic, "r+") as f: user_data = json.loads(f.read()) for key in user_data: if user_data[key]["locked"] == False: print("用户 [ %s ]\t\t锁定状态:[未锁定]"%(key)) else: print("用户 [ %s ]\t\t锁定状态:[已锁定]" % (key)) choice = input("是否进行用户锁定 : 确定'y' 返回'q' :") if choice == "q":break if choice == "y": lock_user = input("请输入要锁定的用户名 :") if lock_user in user_data.keys(): if user_data[lock_user]["locked"] == False: user_data[lock_user]["locked"] = True dic = json.dumps(user_data) f.seek(0) f.truncate(0) f.write(dic) print("\33[31;1m用户 %s 锁定成功\33[0m\n" % (lock_user)) else: print("用户 %s 已经锁定\n" % (lock_user)) else: print("用户 %s 不存在\n"%(lock_user)) '''三次锁定''' def lock(): while True: with open(user_Blacklist,"r+") as f: blacklist_data = json.loads(f.read()) print("当前所有的用户:%s"%blacklist_data) user_name = input("请输入登录的用户名 : ").strip() if user_name in blacklist_data.keys(): if blacklist_data[user_name] == 3 : print("您的 %s 用户已经在黑名单中 !!" %(user_name)) continue with open(user_dic,"r+") as f1: user_data = json.loads(f1.read()) if user_name in user_data.keys(): #print(user_data[user_name]["status"]) if user_data[user_name]["status"] == False: if blacklist_data[user_name] != 3: user_pwd = input("请输入用户 [ %s ] 的登录密码: "%(user_name)).strip() if user_pwd and user_pwd == user_data[user_name]["password"]: print("用户 [ %s ] 登陆成功"%(user_name)) user_data[user_name]["status"] = False data = json.dumps(user_data) f1.seek(0) f1.truncate(0) f1.write(data) break else: print("用户 [ %s ] 密码输入错误:"%(user_name)) blacklist_data[user_name] += 1 data = json.dumps(blacklist_data) #print(blacklist_data) f.seek(0) f.truncate(0) f.write(data) else: print("用户 [ %s ] 已被加入黑名单" % (user_name)) data = json.dumps(blacklist_data) f.seek(0) f.truncate(0) f.write(data) else: print("用户 [ %s ] 已经在登录状态" % (user_name)) else: print("用户 [ %s ] 不存在,请重新输入:" % (user_name))
Blacklist_user
{"zhangsan": 1, "zhaosi": 0, "wangwu": 3, "51cto": "0", "53cto": "0"}
creditcard_data
{"123456": {"creditcard": "123456", "password": "123", "personinfo": "\u5510\u50e7", "limit": 12929, "limitcash": 5934, "locked": false, "deflimit": 15000}, "222222": {"creditcard": "222222", "password": "123", "personinfo": "\u732a\u516b\u6212", "limit": 14868, "limitcash": 7428, "locked": false, "deflimit": 15000}, "111111": {"creditcard": "111111", "password": "123", "personinfo": "\u767d\u9f99\u9a6c", "limit": 11721, "limitcash": 5860, "locked": false, "deflimit": 15000}, "123123": {"creditcard": "123123", "password": "123", "personinfo": "51cto", "limit": 15007, "limitcash": 7440, "locked": false, "deflimit": 15000}, "654321": {"creditcard": "654321", "password": "123", "personinfo": "52cto", "limit": 10405, "limitcash": 5203, "locked": false, "deflimit": 15000}, "333333": {"creditcard": "333333", "password": "123", "personinfo": "53cto", "limit": 17000, "limitcash": 8500, "locked": false, "deflimit": 15000}}
creditcard_record
shopping_car
[["l-TV", "999"], ["Book", "99"], ["dell", "1199"], ["Book", "99"], ["iPad", "2199"]]
shopping_list
iPhone 3999 iPad 2199 bike 1999 dell 1199 l-TV 999 Book 99
user_data
{"zhaosi": {"status": false, "username": "zhaosi", "password": "123", "creditcard": "111111", "address": "None", "locked": false}, "zhangsan": {"username": "zhangsan", "password": "123", "creditcard": "123456", "address": "None", "locked": false, "status": false, "creditcard_data": "123123"}, "wangwu": {"username": "wangwu", "password": "123", "creditcard": false, "address": "None", "status": false, "locked": false}, "51cto": {"username": "51cto", "password": "123", "creditcard": "123123", "address": "None", "status": false, "locked": false}, "53cto": {"username": "53cto", "password": "123", "creditcard": "654321", "address": "None", "status": false, "locked": false}}
相关文章推荐
- 模拟实现一个ATM + 购物商城程序
- 模拟实现一个ATM + 购物商城程序
- python 实现(简单的一个购物商城小程序)
- 一个用java模拟ATM操作的小程序
- WCF技术剖析之二十七: 如何将一个服务发布成WSDL[基于HTTP-GET的实现](提供模拟程序)
- 利用集合实现一个简单的购物商城
- c语言:模拟实现一个输入密码自动取款的程序
- AutoIT模拟实现一个简单的供销存的程序
- 【操作系统】进程程序替换之模拟实现一个简易的Shell
- 用C语言控制台程序模拟一个ATM 机存取款流程
- 最近在编一个通过代理实现网页模拟点击和POST的Internet程序
- WCF技术剖析之二十七: 如何将一个服务发布成WSDL[基于WS-MEX的实现](提供模拟程序)
- java中集合的运用,实现一个简单的购物程序
- 【C语言】没事可以试试这个小程序,使用文件操作,模拟实现一个简单的文件拷贝工具!
- c语言:模拟实现一个输入密码自动取款的程序
- Java 小程序:实现一个购物流程的功能
- WCF技术剖析之二十七: 如何将一个服务发布成WSDL[基于HTTP-GET的实现](提供模拟程序)
- 如何实现用Java编写程序,设计一个模拟电梯运行的类
- WCF技术剖析之二十七: 如何将一个服务发布成WSDL[基于WS-MEX的实现](提供模拟程序)
- 微信小程序 - 实现一个移动端小商城