UIAlertView、UIActionSheet、UIAlertController使用
2017-09-10 00:00
1046 查看
(一)UIAlertView
1.默认样式
// UIAlertView iOS8开始 被废弃 - (void)defaultAlert { // iOS8被废弃 UIAlertView *alert = [[UIAlertView alloc] initWithTitle:nil message:@"测试" delegate:self cancelButtonTitle:@"取消" otherButtonTitles:@"查看",@"评论", nil]; // 因为是默认样式,所以这里可以不用写这句代码 alert.alertViewStyle = UIAlertViewStyleDefault; [alert show]; } // 需要遵守协议UIAlertViewDelegate来响应点击事件 #pragma mark - UIActionSheetDelegate - (void)actionSheet:(UIActionSheet *)actionSheet clickedButtonAtIndex:(NSInteger)buttonIndex { NSLog(@"%ld",buttonIndex); }
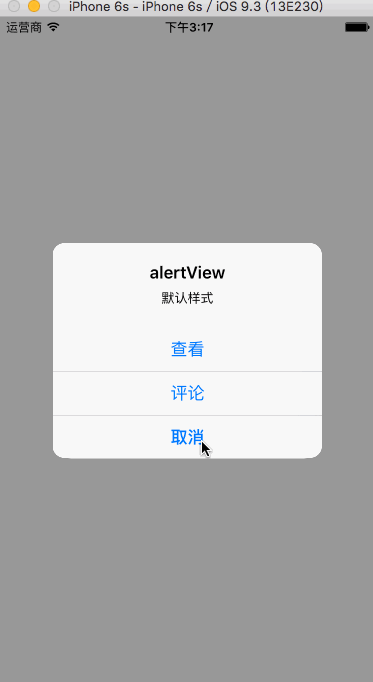
defaultAlert.gif
其中,"查看"的buttonIndex == 1,"评论"的buttonIndex == 2,"取消"的buttonIndex == 0
2.带有明文输入框
- (void)plainText { // iOS8被废弃 UIAlertView *alert = [[UIAlertView alloc] initWithTitle:nil message:@"输入姓名" delegate:self cancelButtonTitle:@"取消" otherButtonTitles:@"确定", nil]; alert.alertViewStyle = UIAlertViewStylePlainTextInput; [alert show]; } #pragma mark - UIActionSheetDelegate - (void)actionSheet:(UIActionSheet *)actionSheet clickedButtonAtIndex:(NSInteger)buttonIndex { NSLog(@"%ld",buttonIndex); }
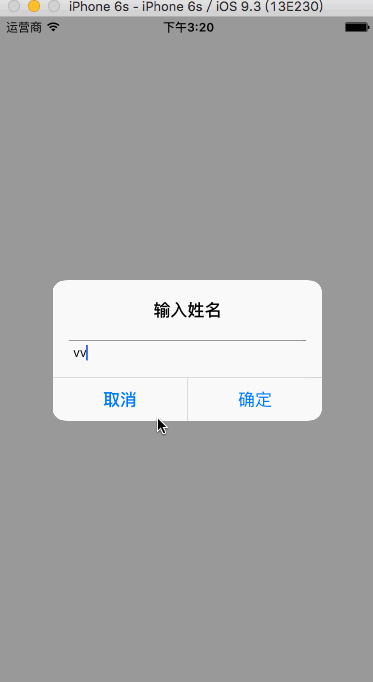
plainTextAlert.gif
3.带有密文输入框
- (void)secureText { // iOS8被废弃 UIAlertView *alert = [[UIAlertView alloc] initWithTitle:nil message:@"输入姓名" delegate:self cancelButtonTitle:@"取消" otherButtonTitles:@"确定", nil]; alert.alertViewStyle = UIAlertViewStyleSecureTextInput; [alert show]; } #pragma mark - UIActionSheetDelegate - (void)actionSheet:(UIActionSheet *)actionSheet clickedButtonAtIndex:(NSInteger)buttonIndex { NSLog(@"%ld",buttonIndex); }
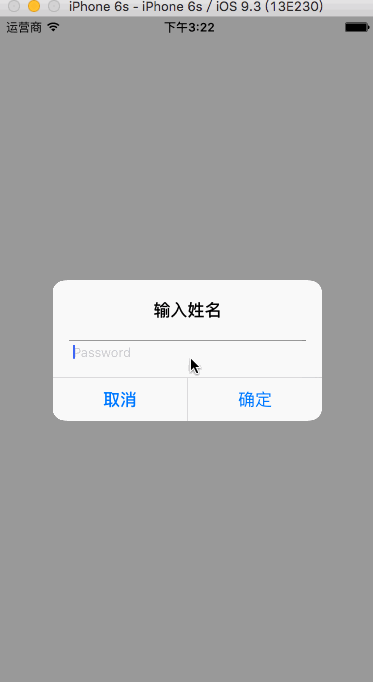
secureTextAlert.gif
4.带有登录和密码输入框
- (void)loginAndPassword { // iOS8被废弃 UIAlertView *alert = [[UIAlertView alloc] initWithTitle:nil message:@"登录" delegate:self cancelButtonTitle:@"取消" otherButtonTitles:@"登录",@"注册", nil]; alert.alertViewStyle = UIAlertViewStyleLoginAndPasswordInput; [alert show]; }
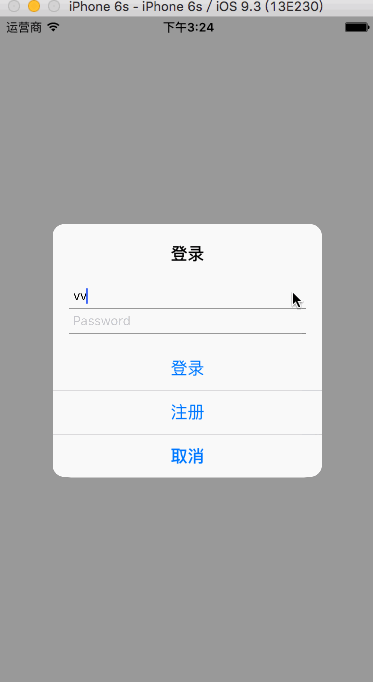
loginAndPwdTextAlert.gif
(二)UIActionSheet
- (void)actionSheet { // iOS8被废弃 UIActionSheet *sheet = [[UIActionSheet alloc] initWithTitle:nil delegate:self cancelButtonTitle:@"取消" destructiveButtonTitle:nil otherButtonTitles:@"查看",@"评论", nil]; [sheet showInView:self.view]; } #pragma mark - UIActionSheetDelegate - (void)actionSheet:(UIActionSheet *)actionSheet clickedButtonAtIndex:(NSInteger)buttonIndex { NSLog(@"%ld",buttonIndex); }
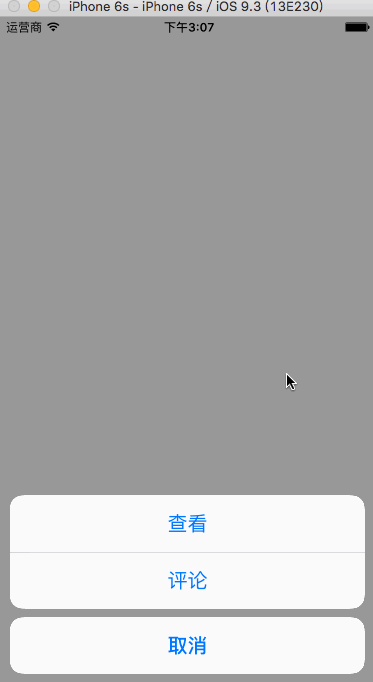
actionSheet.gif
(三)UIAlertController
UIAlertController从iOS8.0开始被使用。旨在替代UIAlertView和UIActionSheet这两个控件。所以UIAlertController有一个
preferredStyle属性属性,该属性是个
UIAlertControllerStyle类型的枚举值,其值如下:
typedef NS_ENUM(NSInteger, UIAlertControllerStyle) { UIAlertControllerStyleActionSheet = 0, UIAlertControllerStyleAlert } NS_ENUM_AVAILABLE_IOS(8_0);
不难发现,UIAlertControllerStyle的枚举值分别是
UIAlertControllerStyleActionSheet和
UIAlertControllerStyleAlert,如果UIAlertController对象的preferredStyle属性取值为UIAlertControllerStyleActionSheet,那么其作用和效果就相当于iOS8中被废弃的UIActionSheet。
想反,如果UIAlertController对象的preferredStyle属性取值为UIAlertControllerStyleAlert,那么其作用和效果就相当于iOS8中被废弃的UIAlertView。
我们知道,使用UIAlertView和UIActionSheet需要遵守对应的协议并实现相应的方法,因为UIAlertView和UIActionSheet采用delegate的方式处理事件。而UIAlertController则采用灵活的block方式处理事件。所以使用UIAlertController不用再遵守某个协议,只需将响应事件的代码写在block中即可。这一点,让我们想起了NSURLConnection和NSURLsession。
1.UIAlertControllerStyleActionSheet样式
- (void)actionSheet { UIAlertController *actionSheetController = [UIAlertController alertControllerWithTitle:nil message:nil preferredStyle:UIAlertControllerStyleActionSheet]; UIAlertAction *showAllInfoAction = [UIAlertAction actionWithTitle:@"查看" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) { }]; UIAlertAction *pickAction = [UIAlertAction actionWithTitle:@"评论" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) { }]; UIAlertAction *cancelAction = [UIAlertAction actionWithTitle:@"取消" style:UIAlertActionStyleCancel handler:^(UIAlertAction * _Nonnull action) { }]; [actionSheetController addAction:cancelAction]; [actionSheetController addAction:commentAction]; [actionSheetController addAction:showAllInfoAction]; [self presentViewController:actionSheetController animated:YES completion:nil]; }
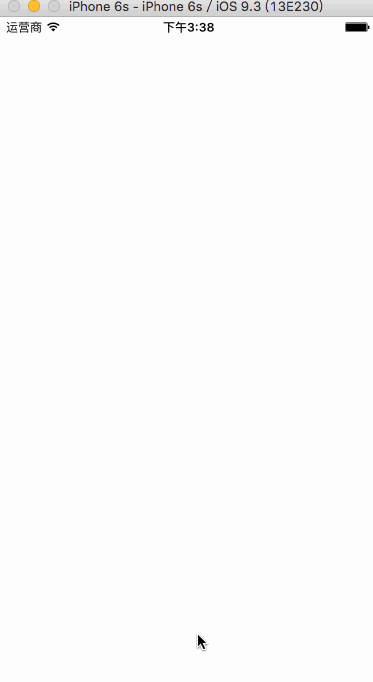
actionSheetStyle.gif
2.UIAlertControllerStyleAlert样式
2.1.默认样式
UIAlertController *actionSheetController = [UIAlertController alertControllerWithTitle:nil message:nil preferredStyle:UIAlertControllerStyleAlert]; UIAlertAction *showAllInfoAction = [UIAlertAction actionWithTitle:@"查看" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) { }]; UIAlertAction *commentAction = [UIAlertAction actionWithTitle:@"评论" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) { }]; UIAlertAction *cancelAction = [UIAlertAction actionWithTitle:@"取消" style:UIAlertActionStyleCancel handler:^(UIAlertAction * _Nonnull action) { }]; [actionSheetController addAction:cancelAction]; [actionSheetController addAction:commentAction]; [actionSheetController addAction:showAllInfoAction]; [self presentViewController:actionSheetController animated:YES completion:nil];
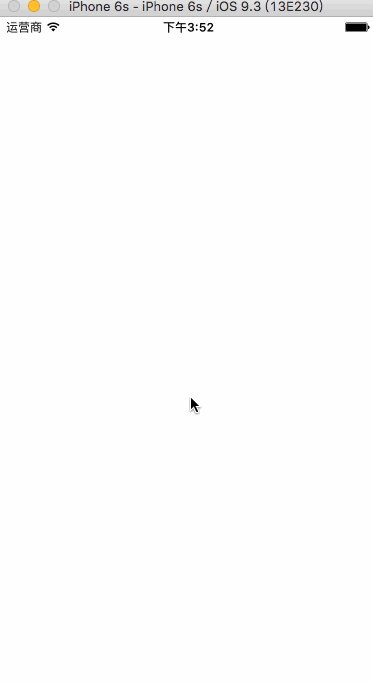
alertStyle.gif
文本框样式
- (void)alertStyleWithTextField { UIAlertController *actionSheetController = [UIAlertController alertControllerWithTitle:nil message:@"输入姓名" preferredStyle:UIAlertControllerStyleAlert]; [actionSheetController addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField) { textField.placeholder = @"请输入姓名"; }]; UIAlertAction *determineAction = [UIAlertAction actionWithTitle:@"确定" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) { }]; UIAlertAction *cancelAction = [UIAlertAction actionWithTitle:@"取消" style:UIAlertActionStyleCancel handler:^(UIAlertAction * _Nonnull action) { }]; [actionSheetController addAction:determineAction]; [actionSheetController addAction:cancelAction]; [self presentViewController:actionSheetController animated:YES completion:nil]; }
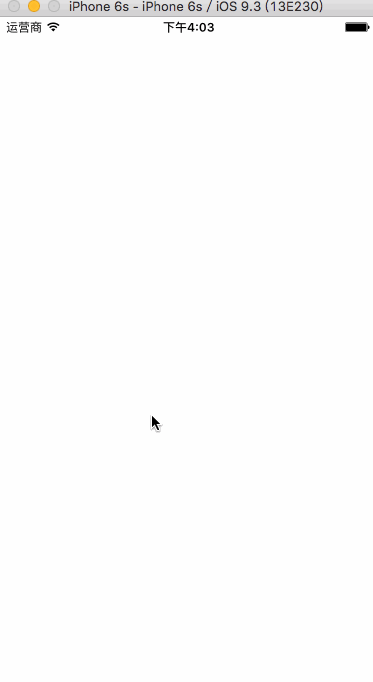
alertTextFieldStyle.gif
2.3.登录模式
- (void)alertStyleWithTwoTextField { UIAlertController *actionSheetController = [UIAlertController alertControllerWithTitle:nil message:@"登录" preferredStyle:UIAlertControllerStyleAlert]; [actionSheetController addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField) { textField.placeholder = @"账号"; }]; [actionSheetController addTextFieldWithConfigurationHandler:^(UITextField * _Nonnull textField) { textField.placeholder = @"密码"; textField.secureTextEntry = YES; }]; UIAlertAction *determineAction = [UIAlertAction actionWithTitle:@"确定" style:UIAlertActionStyleDefault handler:^(UIAlertAction * _Nonnull action) { }]; UIAlertAction *cancelAction = [UIAlertAction actionWithTitle:@"取消" style:UIAlertActionStyleCancel handler:^(UIAlertAction * _Nonnull action) { }]; [actionSheetController addAction:determineAction]; [actionSheetController addAction:cancelAction]; [self presentViewController:actionSheetController animated:YES completion:nil]; }
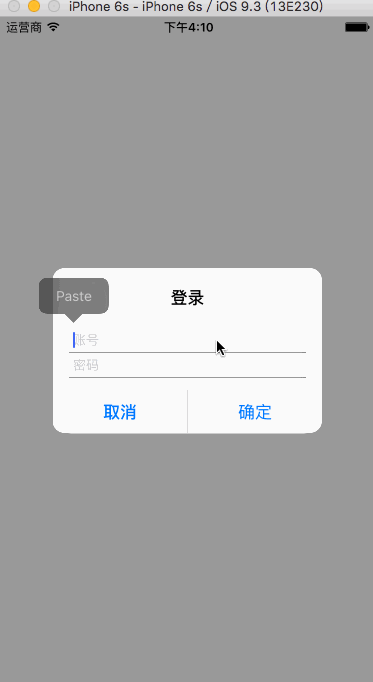
alertTwoTextFieldStyle.gif
demo地址: https://github.com/nlgb/AlertAndActionSheetDemo
文/VV木公子(简书作者)
PS:如非特别说明,所有文章均为原创作品,著作权归作者所有,转载转载请联系作者获得授权,并注明出处,所有打赏均归本人所有!
如果您是iOS开发者,或者对本篇文章感兴趣,请关注本人,后续会更新更多相关文章!敬请期待!
相关文章推荐
- 谈谈改变 UIAlertView 和 UIActionSheet 的颜色 iOS8及以上应该使用的方式
- UIAlertController的使用,代替UIAlertView和UIActionSheet
- iOS UIAlertView 和 UIActionSheet 的使用
- IOS学习笔记(九)之UIAlertView(警告视图)和UIActionSheet(操作表视图)基本概念和使用方法
- UIAlertView提示控件和UIActionSheet的使用
- IOS学习笔记(九)之UIAlertView(警告视图)和UIActionSheet(操作表视图)基本概念和使用方法
- iOS学习笔记—— UIAlertView 和 UIActionSheet 的使用
- UIAlertController的使用(ios9.0后代替UIAlertView与UIActionSheet)
- UIAlertControl的使用对比与UIAlertView和UIActionSheet
- 2.1 UIAlertView,UIActionSheet,UIDatePicker,UIPickerView使用的简单总结
- UIAlertView,UIActionSheet的使用
- iOS:简单使用UIAlertVIew和UIActionSheet
- iOS8中提示框的使用UIAlertController(UIAlertView和UIActionSheet)
- 4.ScrollView常用属性,常用代理方法,图片轮播器,UIAlertView/UIActionSheet
- IOS笔记之UIKit_UIAlertView、UIActionSheet
- IOS基础UI之(五)UIAlertView、UIActionSheet和UIAlertController详解
- UIActionSheet/ UIAlaterView/UIAlertController
- UIActionSheet,UIAlertView技术分享
- Swift UIAlertView/UIActionSheet