tensorflow之简单卷积神经网络(CNN)搭建
2017-09-03 11:32
549 查看
Local Response Nomalization在前面文章中采用softmax完成了无隐藏层的浅层神经网络,用于对mnist数据集(https://my.oschina.net/chkui/blog/888346)分类,通过1000次迭代,识别率大于90%。本篇文章采用cnn搭建来实现对mnist数据集的分类,增加了卷积运算。参看书籍《tensorflow实战》
1.卷积神经网络
CNN最大的特点就是在于卷积的权值共享,利用空间结构减少学习的参数量,防止过拟合的同时减少计算量。在卷积神经网络中,第一个卷积层直接接受图像像素级的输入,卷积之后传给后面的网络,每一层的卷积操作相当于滤波器,对图像进行特征提取,原则上可保证尺度,平移和旋转不变性。
一般的卷积网络包含一下操作:
(1)卷积。图像通过不同卷积核卷积并且加偏置(bias),提取局部特征,每一个卷积核产生一幅新的2D图像。
(2)非线性激活。对卷积输出结果进行非线性激活函数处理,以前常用Sigmoid函数,现在常用ReLU函数。
(3)池化(Pooling)。降采样操作,包括平均池化和最大值池化,一般选择最大值池化,保留最显著特征,提升模型容畸变能力。
(4)完成以上操作之后,就完成了最常见的卷积层。当然也可以加一个LRN(Local Response Nomalization,局部响应归一化)层,目前流行Trick和Batch Nomalization等。
卷积神经网络的好处在于,参数数量只与滤波器数目和卷积核大小有关,与输入图像尺寸无关。总结一下CNN的要点:局部连接,权值共享,池化(降采样)。
2.LeNet5
LeNet5
是最早的卷积神经网络之一,具有以下特点:
(1)每个卷积层包含:卷积(提取空间特征),池化(Average Pooling)和非线性激活函数(Tanh或Sigmoid)。
(2)采用多层感知器 (Multi-layer Perceptron,MLP)作为最后分类器。
(3)层与层之间的稀疏连接减少计算复杂度。
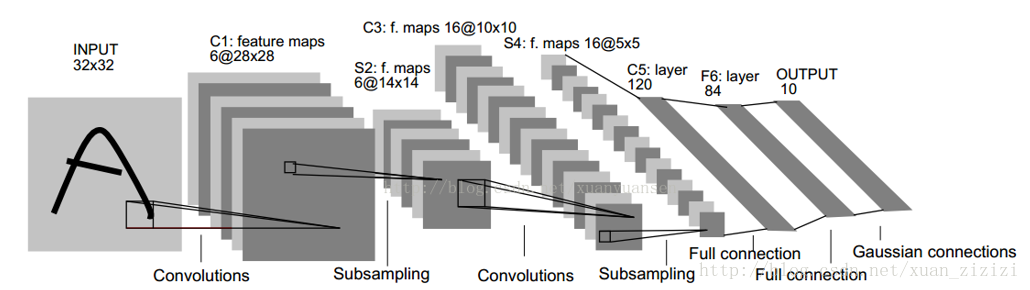
这里有必要说一下卷积前后的尺寸变化计算规则:
输出宽高:outputw outputh
图像宽高:imagew imageh
padding的像素数:pad
步长:stride
卷积核大小:kernelsize
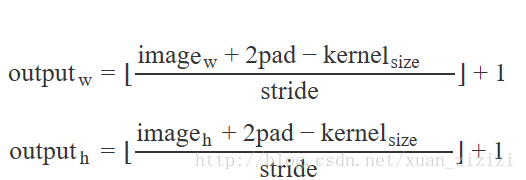
2.两层卷积网络代码实现
这里采用两层卷积网络进行基础的CNN搭建,输入为mnist数据集。涉及到需要定义的函数为:定义权值,定义偏置,定义卷积,定义pooling,定义精确度计算
3.保存
1.卷积神经网络
CNN最大的特点就是在于卷积的权值共享,利用空间结构减少学习的参数量,防止过拟合的同时减少计算量。在卷积神经网络中,第一个卷积层直接接受图像像素级的输入,卷积之后传给后面的网络,每一层的卷积操作相当于滤波器,对图像进行特征提取,原则上可保证尺度,平移和旋转不变性。
一般的卷积网络包含一下操作:
(1)卷积。图像通过不同卷积核卷积并且加偏置(bias),提取局部特征,每一个卷积核产生一幅新的2D图像。
(2)非线性激活。对卷积输出结果进行非线性激活函数处理,以前常用Sigmoid函数,现在常用ReLU函数。
(3)池化(Pooling)。降采样操作,包括平均池化和最大值池化,一般选择最大值池化,保留最显著特征,提升模型容畸变能力。
(4)完成以上操作之后,就完成了最常见的卷积层。当然也可以加一个LRN(Local Response Nomalization,局部响应归一化)层,目前流行Trick和Batch Nomalization等。
卷积神经网络的好处在于,参数数量只与滤波器数目和卷积核大小有关,与输入图像尺寸无关。总结一下CNN的要点:局部连接,权值共享,池化(降采样)。
2.LeNet5
LeNet5
是最早的卷积神经网络之一,具有以下特点:
(1)每个卷积层包含:卷积(提取空间特征),池化(Average Pooling)和非线性激活函数(Tanh或Sigmoid)。
(2)采用多层感知器 (Multi-layer Perceptron,MLP)作为最后分类器。
(3)层与层之间的稀疏连接减少计算复杂度。
这里有必要说一下卷积前后的尺寸变化计算规则:
输出宽高:outputw outputh
图像宽高:imagew imageh
padding的像素数:pad
步长:stride
卷积核大小:kernelsize
2.两层卷积网络代码实现
这里采用两层卷积网络进行基础的CNN搭建,输入为mnist数据集。涉及到需要定义的函数为:定义权值,定义偏置,定义卷积,定义pooling,定义精确度计算
#encoding=utf-8 import tensorflow as tf from tensorflow.examples.tutorials.mnist import input_data mnist = input_data.read_data_sets('MNIST_data',one_hot=True) ##定义权值 def weight_variable(shape): initial = tf.truncated_normal(shape,stddev=0.1) return tf.Variable(initial) ##定义偏置 def bias_variable(shape): initial = tf.constant(0.1,shape=shape) return tf.Variab c8b5 le(initial) ##定义卷积 def conv2d(x,W): #srtide[1,x_movement,y_,movement,1]步长参数说明 return tf.nn.conv2d(x,W,strides = [1,1,1,1],padding='SAME') #x为输入,W为卷积参数,[5,5,1,32]表示5*5的卷积核,1个channel,32个卷积核。strides表示模板移动步长,SAME和VALID两种形式的padding,valid抽取出来的是在原始图片直接抽取,结果比原始图像小,same为原始图像补零后抽取,结果与原始图像大小相同。 ##定义pooling def max_pool_2x2(x): #ksize strides return tf.nn.max_pool(x,ksize=[1,2,2,1],strides=[1,2,2,1],padding='SAME') ##定义计算准确度的功能 def compute_accuracy(v_xs,v_ys): global prediction y_pre = sess.run(prediction,feed_dict={xs:v_xs, keep_prob:1}) correct_prediction = tf.equal(tf.argmax(y_pre,1),tf.argmax(v_ys,1)) accuracy = tf.reduce_mean(tf.cast(correct_prediction,tf.float32)) result = sess.run(accuracy,feed_dict={xs:v_xs,ys:v_ys,keep_prob:1}) return result ##输入 xs = tf.placeholder(tf.float32,[None,784])#28*28 ys = tf.placeholder(tf.float32,[None,10])#10个输出 keep_prob = tf.placeholder(tf.float32) x_image = tf.reshape(xs,[-1,28,28,1]) #-1代表样本数不定,28*28大小的图片,1表示通道数 #print(x_image.shape) ##卷积层conv1 W_conv1 = weight_variable([5,5,1,32])#第一层卷积:卷积核大小5x5,1个颜色通道,32个卷积核 b_conv1 = bias_variable([32])#第一层偏置 h_conv1 = tf.nn.relu(conv2d(x_image,W_conv1)+b_conv1)#第一层输出:输出的非线性处理28x28x32 h_pool1 = max_pool_2x2(h_conv1)#输出为14x14x32 ##卷积层conv2 W_conv2 = weight_variable([5,5,32,64]) b_conv2 = bias_variable([64]) h_conv2 = tf.nn.relu(conv2d(h_pool1,W_conv2)+b_conv2) h_pool2 = max_pool_2x2(h_conv2)#输出为7x7x64 ##全连接层,隐含层的节点个数为1024 W_fc1 = weight_variable([7*7*64,1024]) b_fc1 = bias_variable([1024]) h_pool2_flat = tf.reshape(h_pool2,[-1,7*7*64]) #将2D图像变成1D数据[n_samples,7,7,64]->>[n_samples,7*7*64] h_fc1 = tf.nn.relu(tf.matmul(h_pool2_flat,W_fc1)+b_fc1)#非线性激活函数 h_fc1_drop = tf.nn.dropout(h_fc1,keep_prob)#防止过拟合 ##softmaxe层 W_fc2 = weight_variable([1024,10]) b_fc2 = bias_variable([10]) prediction = tf.nn.softmax(tf.matmul(h_fc1_drop,W_fc2)+b_fc2) #输出误差loss,算法cross_entropy+softmax就可以生成分类算法 cross_entropy = tf.reduce_mean(-tf.reduce_sum(ys*tf.log(prediction),reduction_indices=[1])) train_step = tf.train.AdamOptimizer(1e-4).minimize(cross_entropy) sess = tf.Session() #重要的初始化变量操作 sess.run(tf.initialize_all_variables()) for i in range(1000): batch_xs,batch_ys = mnist.train.next_batch(100)#从下载好的数据集提取100个数据,mini_batch sess.run(train_step,feed_dict={xs:batch_xs,ys:batch_ys,keep_prob:0.5}) if i%50 == 0: print(compute_accuracy(mnist.test.images, mnist.test.labels))
3.保存
#encoding=utf-8 import tensorflow as tf import numpy as np #save file #记得定义的时候形状和类型一样 W = tf.Variable(np.arange(6).reshape((2,3)),dtype=tf.float32,name='weights') b = tf.Variable(np.arange(3).reshape((1,3)),dtype=tf.float32,name='biases') saver = tf.train.Saver() with tf.Session() as sess: saver.restore(sess,"my_net/save_net.ckpt") print("weights:",sess.run(W)) print("weights:",sess.run(b))
相关文章推荐
- TensorFlow基础教程:搭建卷积神经网络CNN
- CNN 卷积神经网络TensorFlow简单实现
- 【Tensorflow】实现简单的卷积神经网络CNN实际代码
- TensorFlow搭建CNN卷积神经网络
- Tensorflow学习:简单实现卷积神经网络(CNN)
- 用Tensorflow搭建CNN卷积神经网络,实现MNIST手写数字识别
- tensorflow_CNN 卷积神经网络_简单教程
- 03:一文全解:使用Tensorflow搭建卷积神经网络CNN识别手写数字图片
- Deep Learning-TensorFlow (10) CNN卷积神经网络_ TFLearn 快速搭建深度学习模型
- tensorflow实现简单的卷积神经网络CNN
- TensorFlow-5实现简单的卷积神经网络CNN
- TensorFlow (2) CNN卷积神经网络_ TFLearn 快速搭建深度学习模型
- Tensorflow体验: 搭建 3D CNN
- Tensorflow体验: 搭建 3D CNN
- Deep Learning-TensorFlow (9) CNN卷积神经网络_《TensorFlow实战》及经典网络模型(下)
- tensorflow程序-最简单的CNN模型
- (Tensorflow之十一)cnn卷积神经网络LeNet-5模型以及卷积核的计算
- 深度学习之卷积神经网络CNN及tensorflow代码实现示例
- Deep Learning-TensorFlow (12) CNN卷积神经网络_ Network in Network 学习笔记
- 简单卷积神经网络的tensorflow实现