WPF窗体关闭/放大/缩小按钮禁用、隐藏的实现
2017-08-31 00:00
483 查看
摘要: 实现WPF中window窗体关闭按钮禁用,放大按钮禁用。整理网上一些大神分享的方法,并附上实验得出结果。
今天接到同事的一个需求,想把wpf中window窗体的关闭按钮禁用,他要在页面中写一个添加按钮点击函数调用this.close();来关闭窗体。
二话不说去百度了,然后找到一堆方法,实验结果都不相同,也出现一些问题。下面先放正确的方法:
借助user32.dll的API,disable关闭按钮(效果如下图):
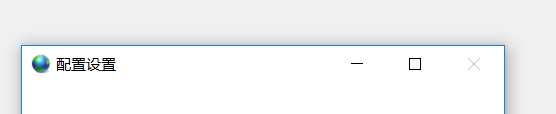
在页面的.xaml.cs文件window类中添加如下代码:
然后再window类的Loaded函数中添加如下代码:

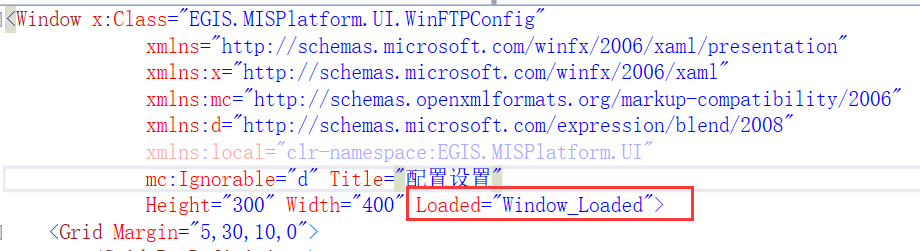
放大按钮禁用
直接在window中设置属性 ResizeMode="CanMinimize"即可。

彻底删除关闭按钮(效果如图)

这个方法会将页面logo,放大、缩小、关闭全部隐藏。
代码如下:
在window类里面添加:
然后在类的Loaded函数中添加:
同上一个方法差不多,使用自定义类来实现,重用它并将它定义为窗口的附加属性,实现效果如下:
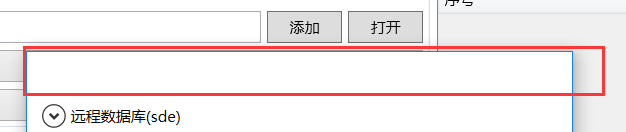
窗体上logo、标题、放大、缩小、关闭全都隐藏了。
代码如下:(新建一个类文件)
然后在window页面中使用:
直接重写onlosing事件(缺点需要在任务管理器中才能关闭窗口)不推荐
在window类中添加:
我找到的差不多就这些方法了。下面是参考文
http://www.cnblogs.com/khler/archive/2009/11/26/1611446.html https://ask.helplib.com/220145 http://blog.csdn.net/gywtzh0889/article/details/47728569
今天接到同事的一个需求,想把wpf中window窗体的关闭按钮禁用,他要在页面中写一个添加按钮点击函数调用this.close();来关闭窗体。
二话不说去百度了,然后找到一堆方法,实验结果都不相同,也出现一些问题。下面先放正确的方法:
借助user32.dll的API,disable关闭按钮(效果如下图):
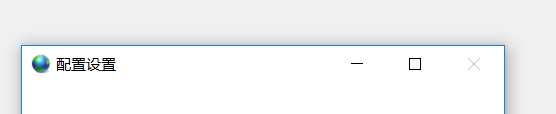
在页面的.xaml.cs文件window类中添加如下代码:
[DllImport("USER32.DLL", CharSet = CharSet.Unicode)] private static extern IntPtr GetSystemMenu(IntPtr hWnd, UInt32 bRevert); [DllImport("USER32.DLL ", CharSet = CharSet.Unicode)] private static extern UInt32 RemoveMenu(IntPtr hMenu, UInt32 nPosition, UInt32 wFlags); private const UInt32 SC_CLOSE = 0x0000F060; private const UInt32 MF_BYCOMMAND = 0x00000000;
然后再window类的Loaded函数中添加如下代码:
var hwnd = new WindowInteropHelper(this).Handle; //获取window的句柄 IntPtr hMenu = GetSystemMenu(hwnd, 0); RemoveMenu(hMenu, SC_CLOSE, MF_BYCOMMAND);

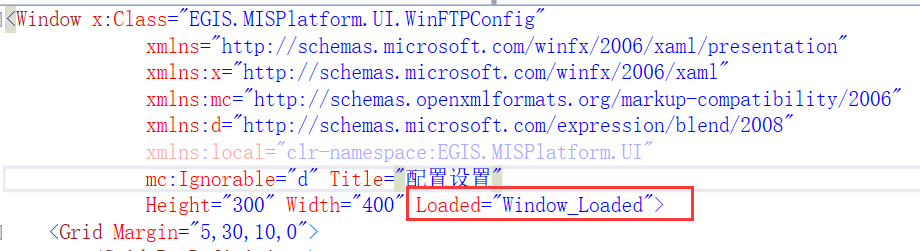
放大按钮禁用
直接在window中设置属性 ResizeMode="CanMinimize"即可。

彻底删除关闭按钮(效果如图)

这个方法会将页面logo,放大、缩小、关闭全部隐藏。
代码如下:
在window类里面添加:
private const int GWL_STYLE = -16; private const int WS_SYSMENU = 0x80000; [DllImport("user32.dll", SetLastError = true)] private static extern int GetWindowLong(IntPtr hWnd, int nIndex); [DllImport("user32.dll")] private static extern int SetWindowLong(IntPtr hWnd, int nIndex, int dwNewLong);
然后在类的Loaded函数中添加:
var hwnd = new WindowInteropHelper(this).Handle; SetWindowLong(hwnd, GWL_STYLE, GetWindowLong(hwnd, GWL_STYLE) & ~WS_SYSMENU);
同上一个方法差不多,使用自定义类来实现,重用它并将它定义为窗口的附加属性,实现效果如下:
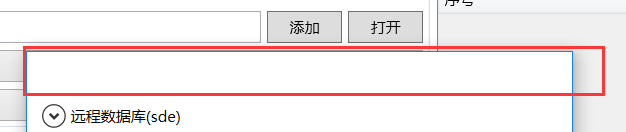
窗体上logo、标题、放大、缩小、关闭全都隐藏了。
代码如下:(新建一个类文件)
public class WindowBehavior { private static readonly Type OwnerType = typeof(WindowBehavior); #region HideCloseButton (attached property) public static readonly DependencyProperty HideCloseButtonProperty = DependencyProperty.RegisterAttached( "HideCloseButton", typeof(bool), OwnerType, new FrameworkPropertyMetadata(false, new PropertyChangedCallback(HideCloseButtonChangedCallback))); [AttachedPropertyBrowsableForType(typeof(Window))] public static bool GetHideCloseButton(Window obj) { return (bool)obj.GetValue(HideCloseButtonProperty); } [AttachedPropertyBrowsableForType(typeof(Window))] public static void SetHideCloseButton(Window obj, bool value) { obj.SetValue(HideCloseButtonProperty, value); } private static void HideCloseButtonChangedCallback(DependencyObject d, DependencyPropertyChangedEventArgs e) { var window = d as Window; if (window == null) return; var hideCloseButton = (bool)e.NewValue; if (hideCloseButton && !GetIsHiddenCloseButton(window)) { if (!window.IsLoaded) { window.Loaded += HideWhenLoadedDelegate; } else { HideCloseButton(window); } SetIsHiddenCloseButton(window, true); } else if (!hideCloseButton && GetIsHiddenCloseButton(window)) { if (!window.IsLoaded) { window.Loaded -= ShowWhenLoadedDelegate; } else { ShowCloseButton(window); } SetIsHiddenCloseButton(window, false); } } #region Win32 imports private const int GWL_STYLE = -16; private const int WS_SYSMENU = 0x80000; [DllImport("user32.dll", SetLastError = true)] private static extern int GetWindowLong(IntPtr hWnd, int nIndex); [DllImport("user32.dll")] private static extern int SetWindowLong(IntPtr hWnd, int nIndex, int dwNewLong); #endregion private static readonly RoutedEventHandler HideWhenLoadedDelegate = (sender, args) => { if (sender is Window == false) return; var w = (Window)sender; HideCloseButton(w); w.Loaded -= HideWhenLoadedDelegate; }; private static readonly RoutedEventHandler ShowWhenLoadedDelegate = (sender, args) => { if (sender is Window == false) return; var w = (Window)sender; ShowCloseButton(w); w.Loaded -= ShowWhenLoadedDelegate; }; private static void HideCloseButton(Window w) { var hwnd = new WindowInteropHelper(w).Handle; SetWindowLong(hwnd, GWL_STYLE, GetWindowLong(hwnd, GWL_STYLE) & ~WS_SYSMENU); } private static void ShowCloseButton(Window w) { var hwnd = new WindowInteropHelper(w).Handle; SetWindowLong(hwnd, GWL_STYLE, GetWindowLong(hwnd, GWL_STYLE) | WS_SYSMENU); } #endregion #region IsHiddenCloseButton (readonly attached property) private static readonly DependencyPropertyKey IsHiddenCloseButtonKey = DependencyProperty.RegisterAttachedReadOnly( "IsHiddenCloseButton", typeof(bool), OwnerType, new FrameworkPropertyMetadata(false)); public static readonly DependencyProperty IsHiddenCloseButtonProperty = IsHiddenCloseButtonKey.DependencyProperty; [AttachedPropertyBrowsableForType(typeof(Window))] public static bool GetIsHiddenCloseButton(Window obj) { return (bool)obj.GetValue(IsHiddenCloseButtonProperty); } private static void SetIsHiddenCloseButton(Window obj, bool value) { obj.SetValue(IsHiddenCloseButtonKey, value); } #endregion }
然后在window页面中使用:
<Window x:Class="WafClient.Presentation.Views.SampleWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:model="clr-namespace:EGIS.MISPlatform.Model;assembly=EGIS.MISPlatform" ResizeMode="NoResize" model:WindowBehavior.HideCloseButton="True" . . . </Window>
直接重写onlosing事件(缺点需要在任务管理器中才能关闭窗口)不推荐
在window类中添加:
protected override void OnClosing(System.ComponentModel.CancelEventArgs e) { e.Cancel = true; }
我找到的差不多就这些方法了。下面是参考文
http://www.cnblogs.com/khler/archive/2009/11/26/1611446.html https://ask.helplib.com/220145 http://blog.csdn.net/gywtzh0889/article/details/47728569
相关文章推荐
- WPF窗体最大化、最小化、关闭按钮的隐藏和禁用
- android webview 实现放大缩小,隐藏按钮控件
- 如何实现“WinForm窗体禁用关闭按钮”方法功能及源代码
- 窗体最大化、最小化、隐藏、关闭功能及程序退出功能的按钮实现
- C# 实现关闭按钮隐藏窗体而不退出
- 百度地图显示/隐藏放大缩小按钮
- cocos2dx lua实现按钮的放大和缩小功能
- canvas画一个图片,并实现点击按钮上移下移左移右移放大缩小
- 禁用WPF窗体的最大化按钮
- winForm窗体关闭按钮实现托盘后台运行(类似QQ托盘区运行)
- 实现一个简单的按钮实例-上下左右,左旋转,右旋转,放大缩小。
- 【转载】winForm窗体关闭按钮 实现提示选择,托盘后台运行或退出(类似QQ托盘区运行)
- Windows Forms中禁用窗体的关闭按钮
- android webview 实现放大缩小 隐藏控件问题
- 禁用winform窗体上的关闭按钮
- 实现隐藏窗体而非关闭的方法
- WPF中如何禁用/去除窗口右上角的关闭按钮
- flex实现窗口关闭时放大和缩小的效果 Zoom
- WinForm窗体禁用关闭按钮
- winform 窗体禁用关闭按钮的三种方法