Python爬虫——漫画下载
2017-08-25 23:13
393 查看
在前面的分享中,我们已经知道了如何利用PhantomJS来下载网页中动态加载的图片。本次分享的目标是,下载动漫网页中的漫画,示例网址如下:http://comic.kukudm.com/comiclist/43/ .
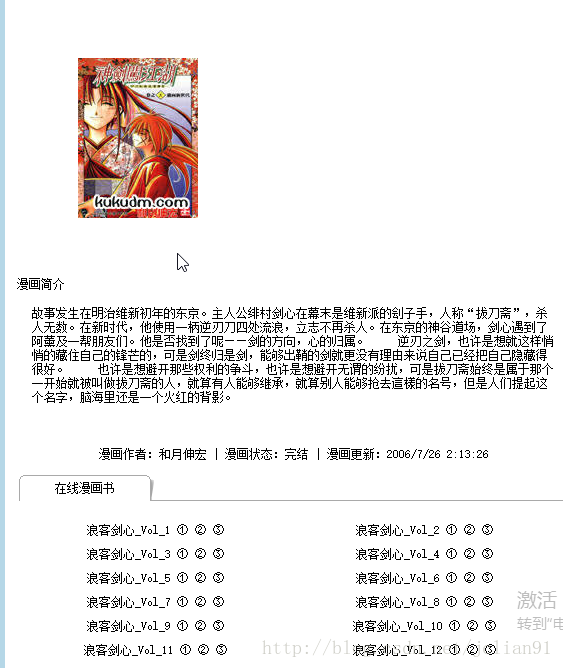
分析上面的网页,如果要将页面中的漫画都下载下来,那么首先必须要分析每卷漫画的网址,具体代码如下,其中url_lst为每卷漫画的网址,file_lst为每卷漫画的名字。
接着需要对每卷漫画进行下载,可以利用for循环遍历url_lst.利用PhantomJS先分析出每卷漫画总的图片数,利用网址的变化规律(就是网址前面的部分不变,数字部分即为图片的编号)去下载每一页的漫画。还有一个比较重要的问题,就是如何解决urlretrieve下载不完全的问题,这个已在上一篇的分享中讲过。具体实现的代码如下:
这样我们就能愉快地在这个网站上下载自己喜欢的动漫啦~笔者的测试如下:

附上全部代码,欢迎大家测试,也欢迎大家交流~~如有不足之处,还请批评指正^o^~
分析上面的网页,如果要将页面中的漫画都下载下来,那么首先必须要分析每卷漫画的网址,具体代码如下,其中url_lst为每卷漫画的网址,file_lst为每卷漫画的名字。
def get_url_lst(url): url_lst = [] file_lst = [] # 讀取網頁 html = urllib.request.urlopen(url) content = html.read() html.close() #網頁解析 soup = BeautifulSoup(content, "lxml") table = soup.find_all(id="comiclistn") for tr in table[0].children: count = 0 for x in tr: if isinstance(x, bs4.element.Tag): if count == 0: file_lst.append(x.string) if count == 1: url_lst.append(x['href']) count += 1 return url_lst, file_lst
接着需要对每卷漫画进行下载,可以利用for循环遍历url_lst.利用PhantomJS先分析出每卷漫画总的图片数,利用网址的变化规律(就是网址前面的部分不变,数字部分即为图片的编号)去下载每一页的漫画。还有一个比较重要的问题,就是如何解决urlretrieve下载不完全的问题,这个已在上一篇的分享中讲过。具体实现的代码如下:
for i in range(len(url_lst)): #獲取這一頁總的圖片數量 browser = webdriver.PhantomJS() browser.set_page_load_timeout(30) # 最大等待时间为30s #当加载时间超过30秒后,自动停止加载该页面 try: browser.get(url_lst[i]) except (TimeoutException,socket.timeout): browser.execute_script('window.stop()') source = browser.page_source total_page = int(source[source.find('共')+1:source.find('页')]) browser.quit() #创建新文件夹 os.mkdir(os.path.join("J:\\comic",file_lst[i])) #遍历图片的网址 for j in range(1,1+total_page): browser = webdriver.PhantomJS() browser.set_page_load_timeout(30) # 最大等待时间为30s #当加载时间超过30秒后,自动停止加载该页面 try: browser.get(url_lst[i].replace('/1.htm','/%d.htm'%j)) except (TimeoutException,socket.timeout): browser.execute_script('window.stop()') source = browser.page_source soup = BeautifulSoup(source,'lxml') #获取图片的下载地址,设置图片名称 image = soup.find('img') url =image.get('src') image_name = "J:\\comic\%s\pic_%d.jpg"%(file_lst[i],j) #解决下载不完全问题,重新尝试五次后仍未下载完即为下载失败 try: urllib.request.urlretrieve(url,image_name) print("%s,第%d张图片下载完毕!"%(file_lst[i],j),time.ctime()) except (socket.timeout, AttributeError): count = 1 while count <= 5: try: urllib.request.urlretrieve(url,image_name) print("%s,第%d张图片下载完毕!"%(file_lst[i],j),time.ctime()) break except (socket.timeout, AttributeError): err_info = 'Reloading for %d time'%count if count == 1 else 'Reloading for %d times'%count print(err_info) count += 1 if count > 5: with open('J:\\comic\%s\error.txt'%file_lst[i],'a') as f: f.write("%s,第%d张图片下载失败!\n"%(file_lst[i],j)) browser.quit()
这样我们就能愉快地在这个网站上下载自己喜欢的动漫啦~笔者的测试如下:
附上全部代码,欢迎大家测试,也欢迎大家交流~~如有不足之处,还请批评指正^o^~
# -*- coding: utf-8 -*-
import os
import bs4
import time
import socket
import urllib
import urllib.request
from bs4 import BeautifulSoup
from selenium import webdriver
from selenium.common.exceptions import TimeoutException
def get_url_lst(url): url_lst = [] file_lst = [] # 讀取網頁 html = urllib.request.urlopen(url) content = html.read() html.close() #網頁解析 soup = BeautifulSoup(content, "lxml") table = soup.find_all(id="comiclistn") for tr in table[0].children: count = 0 for x in tr: if isinstance(x, bs4.element.Tag): if count == 0: file_lst.append(x.string) if count == 1: url_lst.append(x['href']) count += 1 return url_lst, file_lst
def main():
socket.setdefaulttimeout(45)
url = 'http://comic.kukudm.com/comiclist/43/'
url_lst, file_lst = get_url_lst(url)
print('Begin downloading...',time.ctime())
for i in range(len(url_lst)):
#獲取這一頁總的圖片數量
browser = webdriver.PhantomJS()
browser.set_page_load_timeout(30) # 最大等待时间为30s
#当加载时间超过30秒后,自动停止加载该页面
try:
browser.get(url_lst[i])
except (TimeoutException,socket.timeout):
browser.execute_script('window.stop()')
source = browser.page_source
total_page = int(source[source.find('共')+1:source.find('页')])
browser.quit()
#创建新文件夹
os.mkdir(os.path.join("J:\\comic",file_lst[i]))
#遍历图片的网址
for j in range(1,1+total_page):
browser = webdriver.PhantomJS()
browser.set_page_load_timeout(30) # 最大等待时间为30s
#当加载时间超过30秒后,自动停止加载该页面
try:
browser.get(url_lst[i].replace('/1.htm','/%d.htm'%j))
except (TimeoutException,socket.timeout):
browser.execute_script('window.stop()')
source = browser.page_source
soup = BeautifulSoup(source,'lxml')
#获取图片的下载地址,设置图片名称
image = soup.find('img')
url =image.get('src')
image_name = "J:\\comic\%s\pic_%d.jpg"%(file_lst[i],j)
##解决下载不完全问题,重新尝试五次后仍未下载完即为下载失败
try:
urllib.request.urlretrieve(url,image_name)
print("%s,第%d张图片下载完毕!"%(file_lst[i],j),time.ctime())
except (socket.timeout, AttributeError):
count = 1
while count <= 5:
try:
urllib.request.urlretrieve(url,image_name)
print("%s,第%d张图片下载完毕!"%(file_lst[i],j),time.ctime())
break
except (socket.timeout, AttributeError):
err_info = 'Reloading for %d time'%count if count == 1 else 'Reloading for %d times'%count
print(err_info)
count += 1
if count > 5:
with open('J:\\comic\%s\error.txt'%file_lst[i],'a') as f:
f.write("%s,第%d张图片下载失败!\n"%(file_lst[i],j))
browser.quit()
main()
相关文章推荐
- python实现爬虫下载漫画示例
- python学习笔记(14)--爬虫下载漫画图片修改版
- python实现爬虫下载漫画示例
- python学习笔记(11)--爬虫下载漫画图片
- Python简单知乎爬虫--爬取页面的图片并下载到本地
- python自学笔记(8)--Python简单爬虫从网站上下载图片和用第三方库request实现百度翻译
- python中使用网络爬虫下载图片
- python --网页爬虫,文本处理,科学计算,机器学习,数据挖掘资料+附带工具包下载+tensorflow
- python爬虫实战:利用scrapy,短短50行代码下载整站短视频
- python爬虫:selenuim+phantomjs模拟浏览器操作,用BeautifulSoup解析页面,用requests下载文件
- Python爬虫之urllib简单下载内容
- 使用python进行爬虫下载指定网站的图片
- Python小爬虫-自动下载三亿文库文档
- python 网络爬虫爬取落网 期刊内容 下载图片 音乐
- python爬虫:下载百度贴吧图片(多页)学习笔记
- Python协程并发 爬虫下载
- Python开发爬虫爬取百度百科词条信息(源码下载)
- 基于Python的scrapy框架的广州天气爬虫源码下载