文本编辑器
2017-08-18 00:23
162 查看
这是之前学习swing窗体技术时写的一个文本编辑器,现在写到博客里。
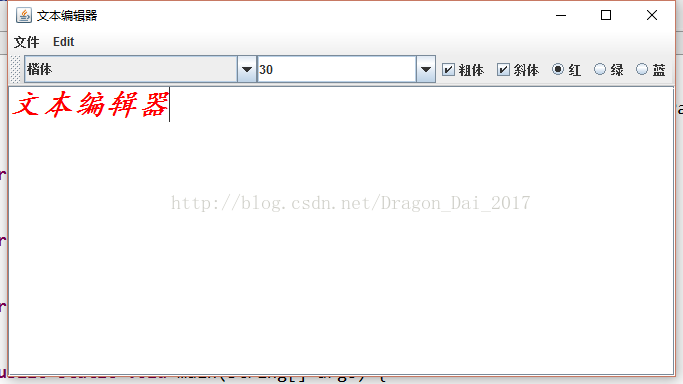
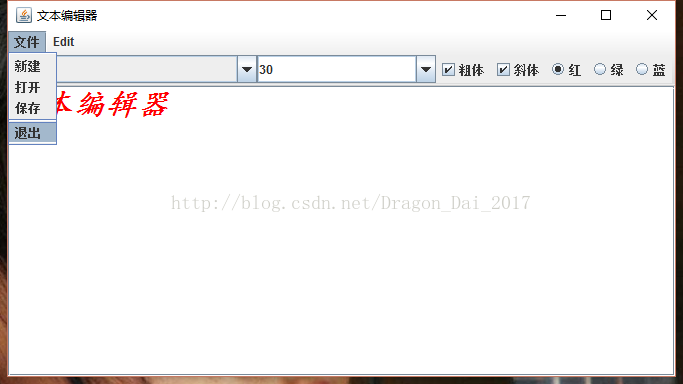
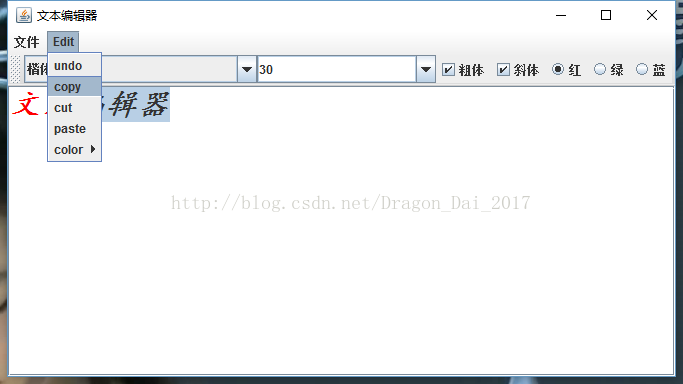
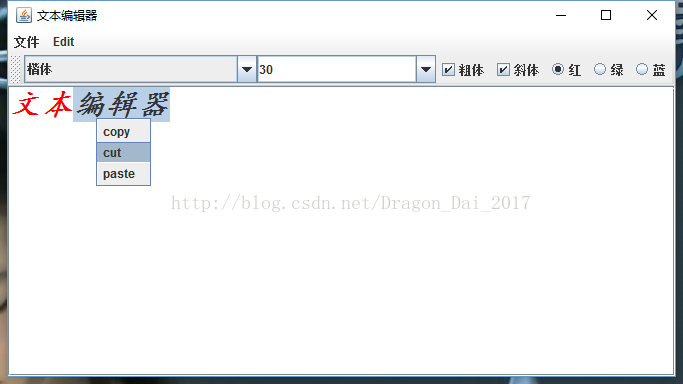
代码:
代码:
package cn.hncu.swing; import java.awt.BorderLayout; import java.awt.Color; import java.awt.Dimension; import java.awt.Font; import java.awt.GraphicsEnvironment; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.File; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import javax.swing.ButtonGroup; import javax.swing.JCheckBox; import javax.swing.JComboBox; import javax.swing.JFileChooser; import javax.swing.JFrame; import javax.swing.JMenu; import javax.swing.JMenuBar; import javax.swing.JMenuItem; import javax.swing.JOptionPane; import javax.swing.JPopupMenu; import javax.swing.JRadioButton; import javax.swing.JRadioButtonMenuItem; import javax.swing.JScrollPane; import javax.swing.JTextArea; import javax.swing.JToolBar; public class EditorJFrame extends JFrame implements ActionListener { private JTextArea text; private JComboBox<String>comName,comSize; private JCheckBox checkBold,checkItalic; private JRadioButton radioColors[]; private Color colors[]=new Color[]{Color.red,Color.green,Color.blue};; private String colorStr[]={"红","绿","蓝"}; private JRadioButtonMenuItem itemColors[]; private File oldFile=null; public EditorJFrame(){ super("文本编辑器"); //setLocationRelativeTo(null);//使窗口位于中间 Dimension dim=getToolkit().getScreenSize(); setBounds(dim.width/4, dim.height/4, dim.width/2, dim.height/2); setDefaultCloseOperation(EXIT_ON_CLOSE); getContentPane().setLayout(new BorderLayout());//默认为边布局,也可以不设 text=new JTextArea("welcom 欢迎"); getContentPane().add(new JScrollPane(text),BorderLayout.CENTER); //工具条,,添加方式跟菜单条差不多 JToolBar toolbar=new JToolBar(); getContentPane().add(toolbar,BorderLayout.NORTH); //设置字体名 GraphicsEnvironment ga=GraphicsEnvironment.getLocalGraphicsEnvironment();//返回一个GraphicEnvironment对象 String[] strName=ga.getAvailableFontFamilyNames();//返回字体所有系列名称的字符串数组 comName=new JComboBox<String>(strName); comName.setSelectedIndex(0); comName.addActionListener(this); comName.setEditable(false);//默认是false //comName.setSelectedItem("幼圆");//bug,在给JComBox设置值时,相当于用户单击该组件,激发一次单击事件,从而导致事件单击事件中,在设置该JComboBox之前,没有开内存的变量,发生空指针异常 toolbar.add(comName); //设置字体大小 String[] strSize={"20","30","40","50","60","70"}; comSize=new JComboBox<String>(strSize); comSize.addActionListener(this); comSize.setEditable(true); toolbar.add(comSize); //设置字体形式 checkBold =new JCheckBox("粗体"); checkItalic =new JCheckBox("斜体"); checkBold.addActionListener(this); checkItalic.addActionListener(this); toolbar.add(checkBold); toolbar.add(checkItalic); //设置字体颜色 radioColors=new JRadioButton[colors.length]; ButtonGroup group=new ButtonGroup(); for(int i=0;i<colorStr.length;i++){ radioColors[i]=new JRadioButton(colorStr[i]); group.add(radioColors[i]); toolbar.add(radioColors[i]); radioColors[i].addActionListener(this); } //初始化 comName.setSelectedItem("幼圆"); comSize.setSelectedItem("30"); radioColors[0].setSelected(true); text.setForeground(colors[0]); addMyMenu(); addPopMenu(); setVisible(true); } private void addPopMenu() { final JPopupMenu popMenu=new JPopupMenu(); JMenuItem itemCopy=new JMenuItem("copy"); JMenuItem itemCut=new JMenuItem("cut"); JMenuItem itemPaste=new JMenuItem("paste"); itemCopy.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { copy(); } }); itemCut.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { cut(); } }); itemPaste.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent e) { paste(); } }); popMenu.add(itemCopy); popMenu.add(itemCut); popMenu.add(itemPaste); text.add(popMenu); text.addMouseListener(new MouseAdapter() { public void mouseClicked(MouseEvent e) { if(e.getButton()==MouseEvent.BUTTON3){ popMenu.show(text, e.getX()+10, e.getY()+10); } } }); } private void addMyMenu() { JMenuBar menubar=new JMenuBar(); //add(menubar); this.setJMenuBar(menubar); JMenu menuFile=new JMenu("文件"); menubar.add(menuFile); JMenuItem itemNew=new JMenuItem("新建"); itemNew.setActionCommand("new"); JMenuItem itemOpen=new JMenuItem("打开"); itemOpen.setActionCommand("open"); JMenuItem itemSave=new JMenuItem("保存"); itemSave.setActionCommand("save"); JMenuItem itemExit=new JMenuItem("退出"); itemExit.setActionCommand("exit"); menuFile.add(itemNew); menuFile.add(itemOpen); menuFile.add(itemSave); menuFile.addSeparator();//添加分割线 menuFile.add(itemExit); JMenu menuEdit=new JMenu("Edit"); menubar.add(menuEdit); JMenuItem itemUndo=new JMenuItem("undo"); JMenuItem itemCopy=new JMenuItem("copy"); JMenuItem itemCut=new JMenuItem("cut"); JMenuItem itemPaste=new JMenuItem("paste"); itemSave.addActionListener(this); itemOpen.addActionListener(this); itemNew.addActionListener(this); itemCopy.addActionListener(this); itemCut.addActionListener(this); itemPaste.addActionListener(this); itemExit.addActionListener(this); menuEdit.add(itemUndo); menuEdit.add(itemCopy); menuEdit.add(itemCut); menuEdit.add(itemPaste); JMenu menuColor=new JMenu("color"); itemColors=new JRadioButtonMenuItem[colorStr.length]; ButtonGroup group=new ButtonGroup(); for(int i=0;i<colorStr.length;i++){ itemColors[i]=new JRadioButtonMenuItem(colorStr[i]); group.add(itemColors[i]); menuColor.add(itemColors[i]); itemColors[i].addActionListener(this); } itemColors[0].setSelected(true); menuEdit.add(menuColor); } boolean isFilter=false; public void actionPerformed(ActionEvent e) { if(e.getSource() instanceof JComboBox||e.getSource() instanceof JCheckBox){ String name=(String) comName.getSelectedItem(); String sizeStr=(String) comSize.getSelectedItem(); if(isFilter && e.getSource()==comSize){ isFilter=false; return; } try { int size=Integer.parseInt(sizeStr); int style=0; if(checkBold.isSelected()){ if(checkItalic.isSelected()){ style=style|Font.BOLD; style=style|Font.ITALIC; }else{ style=style|Font.BOLD; } }else{ if(checkItalic.isSelected()){ style=style|Font.ITALIC; } } Font font=new Font(name, style, size); text.setFont(font); if(e.getSource()==comSize){ int i=comSize.getItemCount(); for(int j=0;j<i;j++){ if(size<Integer.parseInt(comSize.getItemAt(j))){ comSize.insertItemAt(size+"", j); break; } if(size==Integer.parseInt(comSize.getItemAt(j))){ return; } } } //JConBox的一个特征,当用户输入数据回车时(相当于给组合框setSelectItem),用户会触发一次单击事件,JComBobox内部会触发一次单击事件 } catch (NumberFormatException e1) { JOptionPane.showMessageDialog(this, sizeStr+"为非法字体大小"); isFilter=true; } } if(e.getSource() instanceof JRadioButton||e.getSource() instanceof JRadioButtonMenuItem){ for(int i=0;i<colorStr.length;i++){ if(e.getSource()==radioColors[i]){ text.setForeground(colors[i]); itemColors[i].setSelected(true); } if(e.getSource()==itemColors[i]){ text.setForeground(colors[i]); radioColors[i].setSelected(true); } } } if(e.getSource() instanceof JMenuItem){ if(e.getActionCommand().equals("copy")){ copy(); }else if(e.getActionCommand().equals("cut")){ cut(); }else if(e.getActionCommand().equals("paste")){ paste(); }else if(e.getActionCommand().equals("new")){ text.setText(""); oldFile=null; }else if(e.getActionCommand().equals("exit")){ System.exit(0); } if(e.getActionCommand().equals("open")){ openFile(); } if(e.getActionCommand().equals("save")){ if(oldFile==null){ saveAsFile(); }else{ try { BufferedWriter bw=new BufferedWriter(new FileWriter(oldFile)); bw.write(text.getText()); bw.close(); } catch (IOException e1) { e1.printStackTrace(); } } } } } private void saveAsFile() { JFileChooser jfc=new JFileChooser(new File(".")); jfc.setDialogTitle("另存为"); int chooser=jfc.showSaveDialog(this); if(chooser==JFileChooser.APPROVE_OPTION){ File file=jfc.getSelectedFile(); String filePath=file.getPath(); try { BufferedWriter bw=new BufferedWriter(new FileWriter(new File(filePath),true)); String string=text.getText(); String str[]=string.split("\n"); c916 int lineCount=text.getLineCount(); boolean isFirst=true; for(int i=0;i<lineCount;i++){ if(isFirst){ isFirst=false; }else{ bw.newLine(); } bw.write(str[i]); } bw.close(); } catch (IOException e) { e.printStackTrace(); } } } private void openFile(){ JFileChooser jfc=new JFileChooser(new File(".")); int chooser=jfc.showOpenDialog(this); if(chooser==JFileChooser.APPROVE_OPTION){ //System.out.println("选中"); oldFile=jfc.getSelectedFile(); try { BufferedReader br=new BufferedReader(new FileReader(oldFile)); String str=null; boolean isFirst=true; while ((str=br.readLine())!=null) { if(isFirst){ isFirst=false; }else{ text.append("\r\n"); } text.append(str); } br.close(); } catch (FileNotFoundException e1) { e1.printStackTrace(); } catch (IOException e1) { e1.printStackTrace(); } }else if(chooser==JFileChooser.CANCEL_OPTION){ JOptionPane.showMessageDialog(this, "你点击了取消"); }else if(chooser==JFileChooser.ERROR_OPTION){ JOptionPane.showMessageDialog(this, "发生错误", "错误", JOptionPane.WARNING_MESSAGE); } } private void copy() { text.copy(); } private void cut() { text.cut(); } private void paste() { text.paste(); } public static void main(String[] args) { new EditorJFrame(); } }
相关文章推荐
- FCKeditor文本编辑器的使用方法
- Visual Studio 2008 菜单:工具+选项+文本编辑器+HTML+格式,选中“键入时插入属性值引号”
- ckeditor3.5.3+ckfinder for java 2.1.1,嵌入文本编辑器,实现上传文件
- 为NBArticle更换文本编辑器
- 三个iOS开源文本编辑器EGOTextView、BCTextView、JTextView
- Qt之文本编辑器(一)
- Asp.Net 文本编辑器(FCKeditor)的介绍、安装及使用
- 文本编辑器制作(2):TextField方案
- 使用百度Ueditor在线文本编辑器心得
- [AHOI2006]Editor文本编辑器Splay Pascal
- 文本编辑器
- iframe 文本编辑器
- ASP.NET2.0 文本编辑器FCKeditor的冰冷之心
- Centos vi 文本编辑器
- linux之文本编辑器
- 装了个个人感觉最好的文本编辑器Sublime Text 3.实时记录。
- 高效文本编辑器vim之基础入门
- paip.提升用户体验---论文本编辑器的色彩方案
- 使用kindeditor文本编辑器
- Extjs4中的Form之文本编辑器htmleditor的使用