python函数: 内置函数
2017-08-14 00:00
597 查看
http://blog.csdn.net/pipisorry/article/details/44755423
python build-in functions - https://docs.python.org/release/3.4.2/library/functions.html]
[https://docs.python.org/release/2.7.2/library/functions.html]
[定位Python built-in函数的源码实现]
皮皮blog
数学运算
abs(-5) # 取绝对值,也就是5
round(number, ndigits=None) # 四舍五入取整,或者小数保留ndigits位小数,round(2.6) 也就是3.0
pow(2, 3) # 相当于2**3,如果是pow(2, 3, 5),相当于2**3 % 5
cmp(2.3, 3.2) # 比较两个数的大小
divmod(9,2) # 返回除法结果和余数
max([1,5,2,9]) # 求最大值
min([9,2,-4,2]) # 求最小值
sum([2,-1,9,12]) # 求和
如果你想计算 x 的 y 次方,以 z 为模,那么你可以这么写:
但是当 x=1234567, y=4567676, z=56 的时候我的电脑足足跑了 64 秒!
不要用 ** 和 % 了,使用 pow(x,y,z) 吧!这个例子可以写成pow(1234567,4567676,56) ,只用了 0.034 秒就出了结果!
你完全可以使用一句 cmp(x, y) 来替代。
float(2) # 转换为浮点数 float
float(str)
Note: python ValueError: could not convert string to float.原因:数据中存在空字符串'',导致无法转换成float。
long("23") # 转换为长整数 long integer
str(2.3) # 转换为字符串 string
complex(3, 9) # 返回复数 3 + 9i
ord("A") # "A"字符对应的数值
chr(65) # 数值65对应的字符
unichr(65) # 数值65对应的unicode字符
bool(0) # 转换为相应的真假值,在Python中,0相当于False
在Python中,下列对象都相当于False:[], (),{},0, None,0.0,''
bin(56) # 返回一个字符串,表示56的二进制数
hex(56) # 返回一个字符串,表示56的十六进制数
oct(56) # 返回一个字符串,表示56的八进制数
list((1,2,3)) # 转换为表 list
tuple([2,3,4]) # 转换为定值表 tuple
slice(5,2,-1) # 构建下标对象 slice
dict(a=1,b="hello",c=[1,2,3]) # 构建词典 dictionary
类型转换
int(str,base) :str为base进制,默认转换为十进制整型
>>>int('11',2) 3
函数str() 用于将值转化为适于人阅读的形式,而repr() 转化为供解释器读取的形式(如果没有等价的语法,则会发生SyntaxError 异常)
某对象没有适于人阅读的解释形式的话, str() 会返回与repr()等同的值。很多类型,诸如数值或链表、字典这样的结构,针对各函数都有着统一的解读方式。字符串和浮点数,有着独特的解读方式。
>>>s = 'Hello, world.'
>>>str(s)
'Hello, world.'
>>>repr(s)
"'Hello, world.'" # The repr() of a string adds string quotes and backslashes
序列操作
all([True, 1, "hello!"]) # 是否所有的元素都相当于True值
any(["", 0, False, [], None]) # 是否有任意一个元素相当于True值
sorted([1,5,3]) # 返回正序的序列,也就是[1,3,5]
reversed([1,5,3]) # 返回反序的序列,也就是[3,5,1]
更简便的写法是:
更简便的写法是:
py2中该函数原型为:sorted(iterable,cmp,key,reverse)
参数解释:
iterable指定要排序的list或者iterable;
cmp为带两个参数的比较函数,指定排序时进行比较的函数,可以指定一个函数或者lambda函数;(从上面看cmp应该是在py3中弃用了)
key 是带一个参数的比较函数;
reverse升降序选择,为False或者True(降序);
axis:指定轴进行排序;
Note:
1 list直接排序的通常用法:list.sort(axis = None, key=lambdax:x[1],reverse = True)
2 对dict对象排序sorted(dict)返回的只是dict.keys()的排序结果,也就是sorted(dict)相当于sorted(dict.keys()),dict.keys()是可迭代对象,返回列表。sorted(dict({1: 2})) 返回 [1]。
例子:
(1)用cmp函数排序
>>>list1 = [('david', 90), ('mary',90), ('sara',80),('lily',95)]
>>>sorted(list1,cmp = lambda x,y: cmp(x[1],y[1]))
[('sara', 80), ('david', 90), ('mary', 90), ('lily', 95)]
(2)用key函数排序
>>>list1 = [('david', 90), ('mary',90), ('sara',80),('lily',95)]
>>>sorted(list1,key = lambda list1: list1[1])
[('sara', 80), ('david', 90), ('mary', 90), ('lily', 95)]
(3)用reverse排序
>>>sorted(list1,reverse = True)
[('sara', 80), ('mary', 90), ('lily', 95), ('david', 90)]
(4)用operator.itemgetter函数排序
>>>from operator import itemgetter
>>>sorted(list1, key=itemgetter(1))
[('sara', 80), ('david', 90), ('mary', 90), ('lily', 95)]
>>>sorted(list1, key=itemgetter(0))
[('david', 90), ('lily', 95), ('mary', 90), ('sara', 80)]
(5)多级排序
>>>sorted(list1, key=itemgetter(0,1))
[('david', 90), ('lily', 95), ('mary', 90), ('sara', 80)]
[由 sort 中 key 的用法浅谈 python]
[https://wiki.python.org/moin/HowTo/Sorting/]
[Python中的sorted函数以及operator.itemgetter函数]
示例
通过内建函数“sorted”来实现:让其通过序列长度来从小到大排序
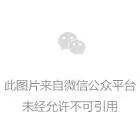
创建一个新的元组(s,t),并把它作为“sorted”的第一个参数。我们将从“sorted”得到一个返回的列表。因为我们使用内置的“len”函数作为“key”参数,如果s更短,“sorted”将返回[s,t], 如果t更短,将返回 [t,s]。
operator.itemgetter函数
operator模块提供的itemgetter函数用于获取对象的哪些维的数据,参数为一些序号(即需要获取的数据在对象中的序号)。
a = [1,2,3]
>>>b=operator.itemgetter(1) //定义函数b,获取对象的第1个域的值
>>>b(a)
2
>>>b=operator.itemgetter(1,0) //定义函数b,获取对象的第1个域和第0个的值
>>>b(a)
(2, 1)
Note:operator.itemgetter函数获取的不是值,而是定义了一个函数,通过该函数作用到对象上才能获取值。
hasattr(me, "test") # 检查me对象是否有test属性
getattr(me, "test") # 返回test属性
setattr(me, "test", new_test) # 将test属性设置为new_test
delattr(me, "test") # 删除test属性
isinstance(me, Me) # me对象是否为Me类生成的对象 (一个instance)
issubclass(Me, object) # Me类是否为object类的子类
当你想检验一个对象的类型的时候,第一个想到的应该是使用 type() 函数。
或者你可以这么写:
编译,执行
repr(me) # 返回对象的字符串表达
compile("print('Hello')",'test.py','exec') # 编译字符串成为code对象
eval("1 + 1") # 解释字符串表达式。参数也可以是compile()返回的code对象
exec("print('Hello')") # 解释并执行字符串,print('Hello')。参数也可以是compile()返回的code对象
import ast
my_list = ast.literal_eval(expr)
来代替以下这种操作:
expr = "[1, 2, 3]"
my_list = eval(expr)
皮皮blog
输入输出
input("Please input:") # 等待输入
print("Hello World!)
文本文件的输入输出 open()
变量
globals() # 返回全局命名空间,比如全局变量名,全局函数名
locals() # 返回局部命名空间
Note:使用 locals() 时要注意是它将包括 所有 的局部变量,它们可能比你想访问的要多。也包括传入函数的参数。
>>>foo = [1, 2, 3, 4]
>>>dir(foo)
['__add__', '__class__', '__contains__',
'__delattr__', '__delitem__', '__delslice__', ...,
'extend', 'index', 'insert', 'pop', 'remove',
'reverse', 'sort']
[python模块导入及属性]
range()
range好像只能生成整数类型的range,但是可以使用np.arange(0,1,0.1)来生成float类型的range。
噢,不用那么麻烦!你可以使用 enumerate() 来提高可读性。
利用enumerate()函数,可以在每次循环中同时得到下标和元素
实际上,enumerate()在每次循环中,返回的是一个包含两个元素的定值表(tuple),两个元素分别赋予index和char
enumerate函数还可以接收第二个参数。
>>>list(enumerate('abc', 1))
[(1, 'a'), (2, 'b'), (3, 'c')]
或者这么写:
如果你想得到倒序的话加上 * 操作符就可以了。
Note: zip函数中的参数len不同,则只取len短的为准
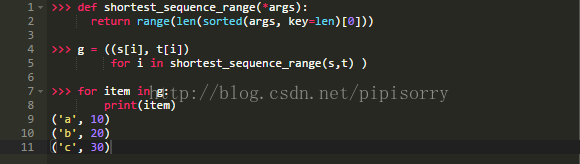
[用列表解析实现‘zip" ]
每次循环时,从各个序列分别从左到右取出一个元素,合并成一个tuple,然后tuple的元素赋予给a,b,c
zip()函数的功能,就是从多个列表中,依次各取出一个元素。每次取出的(来自不同列表的)元素合成一个元组,合并成的元组放入zip()返回的列表中。zip()函数起到了聚合列表的功能。
使用zip分组相邻列表项
皮皮blog
这样会为启动一个服务器。
使用C风格的大括号代替Python缩进来表示作用域
from __future__ import braces
[on_true] if [expression] else [on_false]
x, y = 50, 25
small = x if x < y else y
from:http://blog.csdn.net/pipisorry/article/details/44755423
ref: [Python build-in functions]
Nifty Python tricks
Python built-in functions are awesome. Use them!
Python: Tips, Tricks and Idioms
30 Python Language Features and Tricks You May Not Know About
python内置函数
Python内置(built-in)函数随着python解释器的运行而创建。在Python的程序中,你可以随时调用这些函数,不需要定义。python build-in functions - https://docs.python.org/release/3.4.2/library/functions.html]
[https://docs.python.org/release/2.7.2/library/functions.html]
[定位Python built-in函数的源码实现]
皮皮blog
python常用内置函数
数学运算
abs(-5) # 取绝对值,也就是5round(number, ndigits=None) # 四舍五入取整,或者小数保留ndigits位小数,round(2.6) 也就是3.0
pow(2, 3) # 相当于2**3,如果是pow(2, 3, 5),相当于2**3 % 5
cmp(2.3, 3.2) # 比较两个数的大小
divmod(9,2) # 返回除法结果和余数
max([1,5,2,9]) # 求最大值
min([9,2,-4,2]) # 求最小值
sum([2,-1,9,12]) # 求和
pow(x,y[,z])
返回 x 的 y 次幂(如果 z 存在的话则以z 为模)。如果你想计算 x 的 y 次方,以 z 为模,那么你可以这么写:
mod = (x ** y) % z
但是当 x=1234567, y=4567676, z=56 的时候我的电脑足足跑了 64 秒!
不要用 ** 和 % 了,使用 pow(x,y,z) 吧!这个例子可以写成pow(1234567,4567676,56) ,只用了 0.034 秒就出了结果!
cmp(x,y)
比较两个对象 x 和 y 。 x<y 的时候返回负数,x==y 的时候返回 0,x>y 的时候返回正数。def compare(x,y): if x < y: return -1 elif x == y: return 0 else: return 1
你完全可以使用一句 cmp(x, y) 来替代。
类型转换
int("5") # 转换为整数 integerfloat(2) # 转换为浮点数 float
float(str)
Note: python ValueError: could not convert string to float.原因:数据中存在空字符串'',导致无法转换成float。
long("23") # 转换为长整数 long integer
str(2.3) # 转换为字符串 string
complex(3, 9) # 返回复数 3 + 9i
ord("A") # "A"字符对应的数值
chr(65) # 数值65对应的字符
unichr(65) # 数值65对应的unicode字符
bool(0) # 转换为相应的真假值,在Python中,0相当于False
在Python中,下列对象都相当于False:[], (),{},0, None,0.0,''
bin(56) # 返回一个字符串,表示56的二进制数
hex(56) # 返回一个字符串,表示56的十六进制数
oct(56) # 返回一个字符串,表示56的八进制数
list((1,2,3)) # 转换为表 list
tuple([2,3,4]) # 转换为定值表 tuple
slice(5,2,-1) # 构建下标对象 slice
dict(a=1,b="hello",c=[1,2,3]) # 构建词典 dictionary
类型转换
int(str,base) :str为base进制,默认转换为十进制整型>>>int('11',2) 3
python repr() \str() 函数
将任意值转为字符串:将它传入repr() 或str() 函数。函数str() 用于将值转化为适于人阅读的形式,而repr() 转化为供解释器读取的形式(如果没有等价的语法,则会发生SyntaxError 异常)
某对象没有适于人阅读的解释形式的话, str() 会返回与repr()等同的值。很多类型,诸如数值或链表、字典这样的结构,针对各函数都有着统一的解读方式。字符串和浮点数,有着独特的解读方式。
>>>s = 'Hello, world.'
>>>str(s)
'Hello, world.'
>>>repr(s)
"'Hello, world.'" # The repr() of a string adds string quotes and backslashes
序列操作
all([True, 1, "hello!"]) # 是否所有的元素都相当于True值any(["", 0, False, [], None]) # 是否有任意一个元素相当于True值
sorted([1,5,3]) # 返回正序的序列,也就是[1,3,5]
reversed([1,5,3]) # 返回反序的序列,也就是[3,5,1]
all(iterable)
如果可迭代的对象(数组,字符串,列表等,下同)中的元素都是 true (或者为空)的话返回 True 。_all = True for item in iterable: if not item: _all = False break if _all: # do stuff
更简便的写法是:
if all(iterable): # do stuff
any(iterable)
如果可迭代的对象中任何一个元素为 true 的话返回 True 。如果可迭代的对象为空则返回False 。_any = False for item in iterable: if item: _any = True break if _any: # do stuff
更简便的写法是:
if any(iterable): # do stuff
sorted(iterable,key,reverse)
Python内置的排序函数sorted可以对iterable对象进行排序,Return a new sorted list from the items in iterable.,官网文档 [py3: sorted(iterable[, key][, reverse])] [py2: sorted(iterable[, cmp[, key[, reverse]]])]。py2中该函数原型为:sorted(iterable,cmp,key,reverse)
参数解释:
iterable指定要排序的list或者iterable;
cmp为带两个参数的比较函数,指定排序时进行比较的函数,可以指定一个函数或者lambda函数;(从上面看cmp应该是在py3中弃用了)
key 是带一个参数的比较函数;
reverse升降序选择,为False或者True(降序);
axis:指定轴进行排序;
Note:
1 list直接排序的通常用法:list.sort(axis = None, key=lambdax:x[1],reverse = True)
2 对dict对象排序sorted(dict)返回的只是dict.keys()的排序结果,也就是sorted(dict)相当于sorted(dict.keys()),dict.keys()是可迭代对象,返回列表。sorted(dict({1: 2})) 返回 [1]。
例子:
(1)用cmp函数排序
>>>list1 = [('david', 90), ('mary',90), ('sara',80),('lily',95)]
>>>sorted(list1,cmp = lambda x,y: cmp(x[1],y[1]))
[('sara', 80), ('david', 90), ('mary', 90), ('lily', 95)]
(2)用key函数排序
>>>list1 = [('david', 90), ('mary',90), ('sara',80),('lily',95)]
>>>sorted(list1,key = lambda list1: list1[1])
[('sara', 80), ('david', 90), ('mary', 90), ('lily', 95)]
(3)用reverse排序
>>>sorted(list1,reverse = True)
[('sara', 80), ('mary', 90), ('lily', 95), ('david', 90)]
(4)用operator.itemgetter函数排序
>>>from operator import itemgetter
>>>sorted(list1, key=itemgetter(1))
[('sara', 80), ('david', 90), ('mary', 90), ('lily', 95)]
>>>sorted(list1, key=itemgetter(0))
[('david', 90), ('lily', 95), ('mary', 90), ('sara', 80)]
(5)多级排序
>>>sorted(list1, key=itemgetter(0,1))
[('david', 90), ('lily', 95), ('mary', 90), ('sara', 80)]
[由 sort 中 key 的用法浅谈 python]
[https://wiki.python.org/moin/HowTo/Sorting/]
[Python中的sorted函数以及operator.itemgetter函数]
示例
通过内建函数“sorted”来实现:让其通过序列长度来从小到大排序
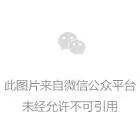
创建一个新的元组(s,t),并把它作为“sorted”的第一个参数。我们将从“sorted”得到一个返回的列表。因为我们使用内置的“len”函数作为“key”参数,如果s更短,“sorted”将返回[s,t], 如果t更短,将返回 [t,s]。
operator.itemgetter函数
operator模块提供的itemgetter函数用于获取对象的哪些维的数据,参数为一些序号(即需要获取的数据在对象中的序号)。a = [1,2,3]
>>>b=operator.itemgetter(1) //定义函数b,获取对象的第1个域的值
>>>b(a)
2
>>>b=operator.itemgetter(1,0) //定义函数b,获取对象的第1个域和第0个的值
>>>b(a)
(2, 1)
Note:operator.itemgetter函数获取的不是值,而是定义了一个函数,通过该函数作用到对象上才能获取值。
类,对象,属性
# define class class Me(object): def test(self): print "Hello!" def new_test(): print "New Hello!" me = Me()
hasattr(me, "test") # 检查me对象是否有test属性
getattr(me, "test") # 返回test属性
setattr(me, "test", new_test) # 将test属性设置为new_test
delattr(me, "test") # 删除test属性
isinstance(me, Me) # me对象是否为Me类生成的对象 (一个instance)
issubclass(Me, object) # Me类是否为object类的子类
isinstance(object, classinfo)
如果 object 参数是 classinfo 参数的一个实例或者子类(直接或者间接)的话返回 True 。当你想检验一个对象的类型的时候,第一个想到的应该是使用 type() 函数。
if type(obj) == type(dict): # do stuff elif type(obj) == type(list): # do other stuff ...
或者你可以这么写:
if isinstance(obj, dict): # do stuff elif isinstance(obj, list): # do other stuff ...
编译,执行
repr(me) # 返回对象的字符串表达compile("print('Hello')",'test.py','exec') # 编译字符串成为code对象
eval("1 + 1") # 解释字符串表达式。参数也可以是compile()返回的code对象
exec("print('Hello')") # 解释并执行字符串,print('Hello')。参数也可以是compile()返回的code对象
对Python表达式求值eval和literal_eval
我们都知道eval函数,但是我们知道literal_eval函数么?import ast
my_list = ast.literal_eval(expr)
来代替以下这种操作:
expr = "[1, 2, 3]"
my_list = eval(expr)
皮皮blog
其它常用函数
输入输出
input("Please input:") # 等待输入print("Hello World!)
文本文件的输入输出 open()
变量
globals() # 返回全局命名空间,比如全局变量名,全局函数名locals() # 返回局部命名空间
Local函数
想让代码看起来更加简明,可以利用 Python 的内建函数 locals() 。它返回的字典对所有局部变量的名称与值进行映射。def test(c): a = {} a[0] = 3 b = 4 print(locals()) if __name__ == '__main__': test(8) {'c': 8, 'b': 4, 'a': {0: 3}}
Note:使用 locals() 时要注意是它将包括 所有 的局部变量,它们可能比你想访问的要多。也包括传入函数的参数。
基本数据类型
type() dir() len()对象自检dir()
在Python 中你可以通过dir() 函数来检查对象。正如下面这个例子:>>>foo = [1, 2, 3, 4]
>>>dir(foo)
['__add__', '__class__', '__contains__',
'__delattr__', '__delitem__', '__delslice__', ...,
'extend', 'index', 'insert', 'pop', 'remove',
'reverse', 'sort']
[python模块导入及属性]
循环设计
range() enumerate() zip()range()
range好像只能生成整数类型的range,但是可以使用np.arange(0,1,0.1)来生成float类型的range。枚举函数enumerate(iterable [,start=0])
如果你以前写过 C 语言,那么你可能会这么写:for iin range(len(list)): # do stuff with list[i], for example, print it print i, list[i]
噢,不用那么麻烦!你可以使用 enumerate() 来提高可读性。
for i, item in enumerate(list): # so stuff with item, for example print it print i, item
利用enumerate()函数,可以在每次循环中同时得到下标和元素
S = 'abcdef' for (index,char) in enumerate(S): print index print char
实际上,enumerate()在每次循环中,返回的是一个包含两个元素的定值表(tuple),两个元素分别赋予index和char
enumerate函数还可以接收第二个参数。
>>>list(enumerate('abc', 1))
[(1, 'a'), (2, 'b'), (3, 'c')]
zip([iterable,])
这个函数返回一个含元组的列表,具体请看例子。l1 = ('You gotta', 'the') l2 = ('love', 'built-in') out = [] if len(l1) == len(l2): for iin range(len(l1)): out.append((l1[i], l2[i])) # out = [('You gotta', 'love'), ('the', 'built-in)]
或者这么写:
l1 = ['You gotta', 'the'] l2 = ['love', 'built-in'] out = zip(l1, l2) # [('You gotta', 'love'), ('the', 'built-in)]
如果你想得到倒序的话加上 * 操作符就可以了。
print zip(*out) # [('You gotta', 'the'), ('love', 'built-in')]
Note: zip函数中的参数len不同,则只取len短的为准
用列表解析实现zip
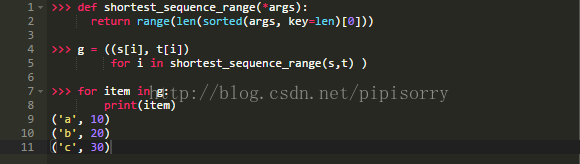
[用列表解析实现‘zip" ]
Zipping and unzipping lists and iterables
>>>a= [1, 2, 3] >>>b = ['a', 'b', 'c'] >>>z = zip(a, b) >>>z [(1, 'a'), (2, 'b'), (3, 'c')] >>>zip(*z) [(1, 2, 3), ('a', 'b', 'c')]
多个序列的zip
如果你多个等长的序列,然后想要每次循环时从各个序列分别取出一个元素,可以利用zip()方便地实现:ta = [1,2,3] tb = [9,8,7] tc = ['a','b','c'] for (a,b,c) in zip(ta,tb,tc): print(a,b,c)
每次循环时,从各个序列分别从左到右取出一个元素,合并成一个tuple,然后tuple的元素赋予给a,b,c
zip()函数的功能,就是从多个列表中,依次各取出一个元素。每次取出的(来自不同列表的)元素合成一个元组,合并成的元组放入zip()返回的列表中。zip()函数起到了聚合列表的功能。
x = [1,23,45] print(x) y = [8,43,74] print(y) z = [3,34,39] print(z) print(list(zip(x,y,z))) [(1, 8, 3), (23, 43, 34), (45, 74, 39)]
使用zip分组相邻列表项
>>>a
=
[
1
,
2
,
3
,
4
,
5
,
6
]
>>>
# Using iterators
>>>group_adjacent
=
lambda
a, k:
zip
(
*
([
iter
(a)]
*
k))
>>>group_adjacent(a,
3
)
[(
1
,
2
,
3
), (
4
,
5
,
6
)]
>>>group_adjacent(a,
2
)
[(
1
,
2
), (
3
,
4
), (
5
,
6
)]
>>>group_adjacent(a,
1
)
[(
1
,), (
2
,), (
3
,), (
4
,), (
5
,), (
6
,)]
>>>
# Using slices
>>>
from
itertools
import
islice
>>>group_adjacent
=
lambda
a, k:
zip
(
*
(islice(a, i,
None
, k)
for
i
in
range
(k)))
>>>group_adjacent(a,
3
)
[(
1
,
2
,
3
), (
4
,
5
,
6
)]
>>>group_adjacent(a,
2
)
[(
1
,
2
), (
3
,
4
), (
5
,
6
)]
>>>group_adjacent(a,
1
)
[(
1
,), (
2
,), (
3
,), (
4
,), (
5
,), (
6
,)]
使用zip & iterators实现推拉窗(n-grams)
>>>
from
itertools
import
islice
>>>
def
n_grams(a, n):
...z
=
(islice(a, i,
None
)
for
i
in
range
(n))
...
return
zip
(
*
z)
...
>>>a
=
[
1
,
2
,
3
,
4
,
5
,
6
]
>>>n_grams(a,
3
)
[(
1
,
2
,
3
), (
2
,
3
,
4
), (
3
,
4
,
5
), (
4
,
5
,
6
)]
>>>n_grams(a,
2
)
[(
1
,
2
), (
2
,
3
), (
3
,
4
), (
4
,
5
), (
5
,
6
)]
>>>n_grams(a,
4
)
[(
1
,
2
,
3
,
4
), (
2
,
3
,
4
,
5
), (
3
,
4
,
5
,
6
)]
使用zip反相字典对象
>>>m
=
{
"a"
:
1
,
"b"
:
2
,
"c"
:
3
,
"d"
:
4
}
>>>
zip
(m.values(), m.keys())
[(
1
,
"a"
), (
3
,
"c"
), (
2
,
"b"
), (
4
,
"d"
)]
>>>mi
=
dict
(
zip
(m.values(), m.keys()))
>>>mi
{
1
:
"a"
,
2
:
"b"
,
3
:
"c"
,
4
:
"d"
}
循环对象
iter()函数对象
map() filter() reduce()皮皮blog
简单服务器
你是否想要快速方便的共享某个目录下的文件呢?# Python2 python -m SimpleHTTPServer # Python 3 python3 -m http.server
这样会为启动一个服务器。
使用C风格的大括号代替Python缩进来表示作用域
from __future__ import braces三元运算
三元运算是if-else 语句的快捷操作,也被称为条件运算。这里有几个例子可以供你参考,它们可以让你的代码更加紧凑,更加美观。[on_true] if [expression] else [on_false]
x, y = 50, 25
small = x if x < y else y
from:http://blog.csdn.net/pipisorry/article/details/44755423
ref: [Python build-in functions]
Nifty Python tricks
Python built-in functions are awesome. Use them!
Python: Tips, Tricks and Idioms
30 Python Language Features and Tricks You May Not Know About
相关文章推荐
- Python学习笔记014——迭代工具函数 内置函数zip()
- Day3 - Python基础3 函数、递归、内置函数
- Python函数篇(3)-内置函数、文件处理
- Python标准库:内置函数hasattr() getattr() setattr() 函数使用方法详解
- Python函数篇(3)-内置函数、文件处理
- python练习,函数,内置函数,递归,程序运行顺序测试
- Python-Day3知识点——深浅拷贝、函数基本定义、内置函数
- python 函数定义和内置函数isinstance以及数据类型检查type的使用
- Python函数篇(3)-内置函数、文件处理
- Python函数篇(3)-内置函数、文件处理
- Python内置函数相详解——sorted()函数
- Python函数篇(3)-内置函数、文件处理
- Python内置函数-reduce()函数
- python函数: 内置函数
- Python学习笔记(三)函数初识和内置函数
- python函数基础(2)-----内置函数、作用域、闭包、递归
- Python函数篇(3)-内置函数、文件处理
- 基于python内置函数与匿名函数详解
- python3中可选参数的灵活运用/内置函数与函数的区别
- Python-老男孩-01_基础_文件IO_函数_yield_三元_常用内置函数_反射_random_md5_序列化_正则表达式_time