python内置函数的简单使用和介绍
2017-08-05 13:06
585 查看
""" 内置函数的简单使用和介绍 参考链接:https://docs.python.org/3/library/functions.html
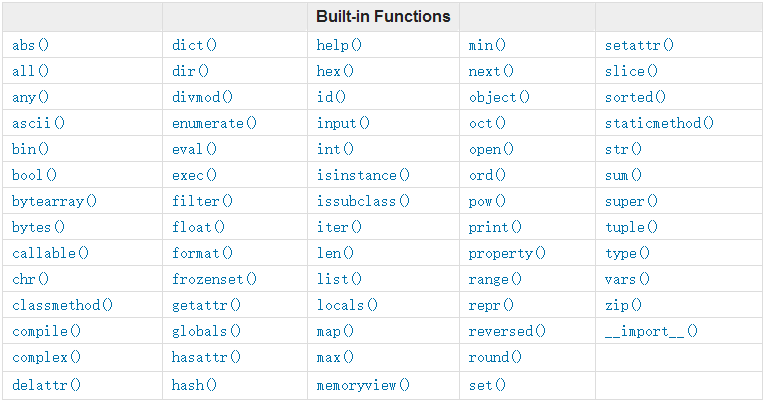
""" 1. abs() # 绝对值 """
n = abs(-10) print (n) # 10
""" 2. all() # 全为真,输出Ture , 则输出Flase any() # 只要有真,输出Ture,则输出Flase 0,None,"",[],(),{} # False """
n1 = all([1,2,3,[],None]) print (n1) # False n2 = any([1,0,"",[]]) print (n2) # True
""" 3. ascii() 自动执行对象的__repr__方法 """
class Foo: def __repr__(self): return "444" n = ascii(Foo()) print (n) # 444
""" 4. bin() # 将十进制转为二进制 0b 表示二进制 oct() # 将十进制转为八进制 0o 表示八进制 hex() # 将十进制转为十六进制 0x 表示十六进制 """
print (bin(5)) print (oct(9)) print (hex(27)) # 0b101 # 0o11 # 0x1b
""" 5. bool() 布尔值 0,None,"",[],(),{} 表示False """ """ 6. bytes() utf8 编码,一个汉字3个字节 gbk 编码,一个汉字2个字节 一个字节 == 8位 str() 字节转化为字符串 """
# 将字符串转换为字节类型,系统中的表现形式为16进制 # bytes(字符串,编码格式) s = "中国" n3 = bytes(s,encoding="utf-8") print (n3) # b'\xe4\xb8\xad\xe5\x9b\xbd' n4 = bytes(s,encoding="gbk") print (n4) # b'\xd6\xd0\xb9\xfa' n5 = str(b'\xd6\xd0\xb9\xfa',encoding="gbk") print (n5) # 中国 n6 = str(b'\xe4\xb8\xad\xe5\x9b\xbd',encoding="utf-8") print (n6) # 中国
""" 7. callable() 检测传递的值是否可以调用 """
def f1(): pass f1() f2 = 123 print (callable(f1)) # True print (callable(f2)) # False
""" 8. chr() ord() 输出 ASCII的对应关系,chr()输出十进制位置的字符,ord()输出字符在ASCii表的位置 """
print (chr(65)) # A print (ord("A")) # 65 # 实例1:生成6位数随机密码,纯字母 # import random # li = [] # for i in range(6): # temp = chr(random.randrange(65,91)) # li.append(temp) # print (li) # result = "".join(li) # print (result) # 实例2:生成8位数随机密码,带数字,字母 import random li = [] for i in range(8): r = random.randrange(0,6) # 让生成数字的位置随机 if r == 2 or r == 5: num = random.randrange(0,10) li.append(str(num)) else: temp = random.randrange(65,91) c = chr(temp) li.append(c) result = "".join(li) print (result) # 3E4JVHF8
""" 9. compile() # 编译,将字符串编译成python代码 eval() # 执行表达式,并且获取结果 exec() # 执行python代码 注:eval有返回值,exec没返回值 python hello.py 过程: 1.读取文件内容open,str 到内存 2.python,把字符串 -> 编译 -> 特殊代码 3.执行代码 """
s = "print('hello,python~')" r = compile(s,"<string>","exec") exec (r) # hello,python~ ss = "8*8+5" print (eval(ss)) # 69
""" 10. dir() help() 快速获取模块,对象提供的功能 """
print (dir(tuple)) print (help(tuple))
""" 11. divmod() 得到商和余数 """
n1,n2 = divmod(96,10) print (n1,n2) # 9 6
""" 12. isinstance() 用于判断,对象是否是某个类的实例 """
s1 = "hello" r = isinstance(s1,str) print (r) # True r1 = isinstance(s1,list) print (r1) # Flase
""" 13. filter() # 函数返回值为Ture,将元素添加结果中 # filter 循环第二个参数,让每个循环元素执行函数,如果函数返回值为Ture,表示函数合法 map() # 将函数返回值添加结果中 # (函数,可迭代的对象(可以for循环)) """
# 示例1 def f1(args): result = [] for item in args: if item > 22: result.append(item) return result li = [11,22,33,44,55,66,77,88] ret = f1(li) print (ret) # [33, 44, 55, 66, 77, 88] # 优化示例1 def f2(a): if a > 22: return True ls1 = [11,22,33,44,55,66,77] res1 = filter(f2,ls1) print(list(res1)) # [33, 44, 55, 66, 77] # 知识扩展,lambda 函数 res2 = filter(lambda x:x > 22,ls1) print (res2) # 返回一个filter object # <filter object at 0x000000E275771748> print (list(res2)) # [33, 44, 55, 66, 77] # 示例2 def f1(args): result = [] for i in args: result.append(i + 100) return result lst1 = [11,22,33,44,55,66] rest = f1(lst1) print (list(rest)) # [111, 122, 133, 144, 155, 166] # 优化示例2,,,map函数 def f2(a): return a + 100 lst2 = [11,22,33,44,55,66] result1 = map(f2,lst2) print (list(result1)) # [111, 122, 133, 144, 155, 166] # 优化示例2,map函数+lambda函数 lst3 = [11,22,33,44,55,66] result2 = map(lambda a:a+100,lst3) print (list(result2)) # [111, 122, 133, 144, 155, 166]
""" 14. globals() # 所有的全局变量 locals() # 所有的局部变量 """
name = "python" def show(): a = 123 b = 456 print (locals()) print (globals()) show() # {'a': 123, 'b': 456} # {'result': '3VZ8B1V0', 'res1': <filter object at 0x000000ECFE24B5C0>, 'lst3': [11, 22, 33, 44, 55, 66], '__package__': None, 'f2': <function f2 at 0x000000ECFFCB9268>, 'ss': '8*8+5', 'n2': 6, 'n3': b'\xe4\xb8\xad\xe5\x9b\xbd', 'temp': 86, 's1': 'hello', 'n': '444', 'name': 'python', 'i': 7, 'rest': [111, 122, 133, 144, 155, 166], '__spec__': None, 'lst1': [11, 22, 33, 44, 55, 66], 'r1': False, 'num': 0, '__builtins__': <module 'builtins' (built-in)>, 'n6': '中国', 'random': <module 'random' from 'C:\\Users\\xieshengsen\\AppData\\Local\\Programs\\Python\\Python35\\lib\\random.py'>, 's': "print('hello,python~')", '__file__': 'D:/PycharmProjects/高级自动化/模块学习/内置函数_v1.py', 'f1': <function f1 at 0x000000ECFFCBF510>, 'result2': <map object at 0x000000ECFE25EC88>, 'show': <function show at 0x000000ECFFCBF2F0>, 'ret': [33, 44, 55, 66, 77, 88], '__cached__': None, 'li': [11, 22, 33, 44, 55, 66, 77, 88], '__doc__': '\n内置函数的简单使用和介绍\n参考链接:https://docs.python.org/3/library/functions.html\n', 'c': 'V', 'result1': <map object at 0x000000ECFE260D30>, 'Foo': <class '__main__.Foo'>, 'n1': 9, 'n5': '中国', 'r': True, '__loader__': <_frozen_importlib_external.SourceFileLoader object at 0x000000ECFDFEA898>, 'ls1': [11, 22, 33, 44, 55, 66, 77], 'lst2': [11, 22, 33, 44, 55, 66], 'res2': <filter object at 0x000000ECFE24B4A8>, 'n4': b'\xd6\xd0\xb9\xfa', '__name__': '__main__'}
""" 15. hash() 生成一个hash值(字符串) """
has = "python" print (hash(has)) # 839578881833832098
""" 16. len() 输出对象的长度 """
print(len("python")) # 6 print (len("长城")) # python3, python2 输出长度为6 (python3按字符,python2按字节) # 2
""" 17. max() # 最大值 min() # 最小值 sun() # 求和 """
lit = [11,22,33,44,55] print (max(lit)) print (min(lit)) print (sum(lit)) # 55 # 11 # 165
""" 18. pow() 求指数 """
print(pow(2,10)) # 1024
""" 19. reverse() 反转 """
lit1 = [11,22,33,44,55,66] print (list(reversed(lit1))) # [66, 55, 44, 33, 22, 11]
""" 20. round() 四舍五入求值 """
print (round(1.4)) print (round(1.8)) # 1 # 2
""" 21. sorted() 排序 等同于列表的sort """
lit2 = [12,32,1,3,4,34,11,5] print (list(sorted(lit2))) # [1, 3, 4, 5, 11, 12, 32, 34] lit2.sort() print (lit2) # [1, 3, 4, 5, 11, 12, 32, 34]
""" 22. zip() """
l1 = ["hello",11,22,33] l2 = ["world",44,55,66] l3 = ["python",77,88,99] l4 = zip(l1,l2,l3) # print (list(l4)) # # [('hello', 'world', 'python'), (11, 44, 77), (22, 55, 88), (33, 66, 99)] temp1 = list(l4)[0] print (temp1[0]) ret1 = " ".join(temp1) print (ret1) # hello world python
相关文章推荐
- 简单介绍Python2.x版本中的cmp()方法的使用
- 【Python Oracle】使用cx_Oracle 连接oracle的简单介绍
- Python经常使用内置函数介绍【filter,map,reduce,apply,zip】
- 简单介绍python-nmap 模块的使用
- 简单介绍使用Python解析并修改XML文档的方法
- python写红包的原理流程包含random,lambda其中的使用和见简单介绍
- 简单介绍Python2.x版本中的cmp()方法的使用
- 简单介绍Python中的JSON使用
- python之pandas简单介绍及使用
- python使用sqlite简单介绍
- 目前大家对Python都有一个共识,就是他对测试非常有用,自动化测试里Python用途也很广,但是Python到底怎么进行自动化测试呢?今天就简单的向大家介绍一下怎么使用Python进行自动化测试
- 【python】Redis介绍及简单使用
- 【Python Oracle】使用cx_Oracle 连接oracle的简单介绍
- 【Python Oracle】使用cx_Oracle 连接oracle的简单介绍
- 简单介绍Python中的decode()方法的使用
- xgboost+python参数介绍的简单使用
- 简单介绍Python中的decode()方法的使用
- 简单介绍使用Python解析并修改XML文档的方法
- python内置函数:lambda、map、filter简单介绍
- 简单介绍Python中的readline()方法的使用